One of the core components of Go is its control structures. This includes the if-else statement, which allows developers to implement conditional statements in their code. This post will discuss the Go language’s decision-making capabilities using if-else statements.
There are multiple combinations of if-else statements that can be used and we will go through each of them one by one.
if statement
This is the most simple statement in the decision-making capabilities of the Go language. It states that if the condition is true, execute the condition contained within the if block.
Syntax of if statement
if condition{
// code
}
Here the condition represents a boolean expression and will return either true or false.
- If the condition returns true, it will execute the code within the if block.
- And if the condition returns false, it will not execute the code within the if block.
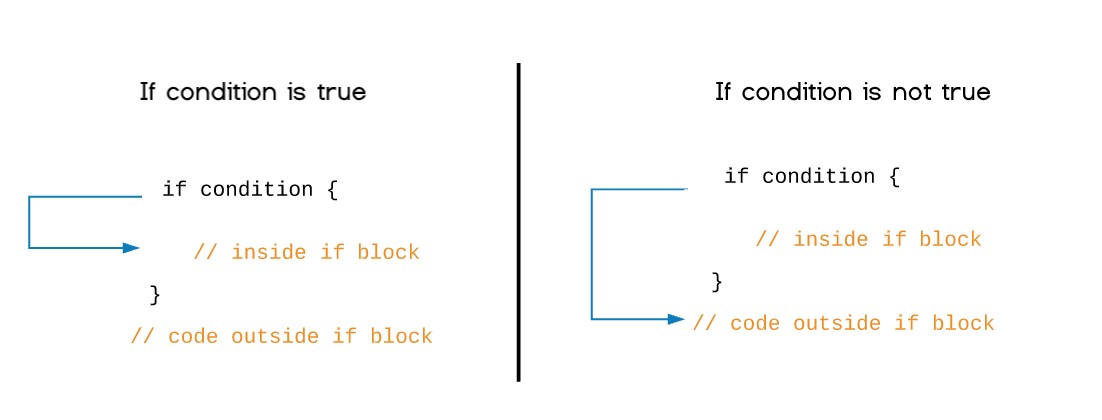
Code example 1
package main
import (
"fmt"
)
func main() {
var height int = 170
if height > 150 {
fmt.Println("Inside if block")
}
fmt.Println("Outside if block")
}
Output –
Inside if block
Outside if block
Here we can see that the if block is executed because the condition was satisfied, and then code outside of the if block is executed.
Code example 2
package main
import (
"fmt"
)
func main() {
var height int = 170
if height < 150 {
fmt.Println("Inside if block")
}
fmt.Println("Outside if block")
}
Output –
Outside if block
We can see here that as the condition failed, the code inside the if block didn’t execute and moved on to the part outside of the if block.
if…else statement
Well, if block code would only be executed if the boolean expression evaluates to true but if it doesn’t, then it will continue with the rest of the code without executing the if block.
But what if want to execute some piece of code if and only if the condition under if-statement is evaluated to false, this is where if…else statement comes into the picture.
Syntax of if-else statements
if condition{
// if block code
} else{
// else block code
}
A very important note: We have written the else keyword in the same line where we closed the if block with a curly brace ( } ). It is important to do so because if we had written the else keyword in the next line, shown below, it would have thrown an error.
if condition{
// if block code
}
else{ // this will give an error, so don't write it like this
//else block code
}
- So, if the condition under the if statement evaluates to true, then the if block will be executed.
- Otherwise, if the condition evaluates to false, then the else block will be executed.

Code example 1
Here we will write a code where the if statement condition will evaluate to true and thus if block will be executed and else block will be ignored.
package main
import (
"fmt"
)
func main() {
var height int = 170
if height > 150 {
fmt.Println("Inside if block")
} else {
fmt.Println("Inside else block")
}
fmt.Println("Outside if else block")
}
Output –
Inside if block
Outside if else block
Code example 2
Here we will fail the if condition and then will see whether the else block will be executed or not.
package main
import (
"fmt"
)
func main() {
var height int = 130
if height > 150 {
fmt.Println("Inside if block")
} else {
fmt.Println("Inside else block")
}
fmt.Println("Outside if else block")
}
Output –
Inside else block
Outside if else block
Here we can see that the if block is not executed because the condition has failed but instead the else block was executed and then the rest of the code.
nested if…else statement
We can also use an if-else statement inside another if-else statement, and this is known as a nested if-else statement.
Below is the simplest syntax of the nested if-else statement, but we can take it up to any number of levels.
if condition {
if condition{
// some code
} else{
// some code
}
}
Code example
package main
import (
"fmt"
)
func main() {
var height int = 170
if height > 150 {
if height < 200 {
fmt.Println("Height is between 150 and 200") // this if-block will be executed
} else {
fmt.Println("Height is greater than 200")
}
}
fmt.Println("Outside nested if else block")
}
Output –
Height is between 150 and 200
Outside nested if else block
Here the height was 170, so it passes the condition inside first if statement ( height > 150 ), then it enters the corresponding if block and again encounters an if statement whose condition also evaluates to true ( height < 200 ), so, it enters in that if block to execute its code and so the corresponding else block is ignored. Afterward, the flow comes out of the nested if-else statements to execute other parts of the program.
if…else…if ladder
The simplest syntax of an if…else…if ladder
if condition1 {
// some code
} else if condition2 {
// some code
} else{
// some code
}
We can have as many chains as we want, there is no limit here.
- The flow will start from top to bottom, so it first checks the condition in the first if statement, and if it evaluates to true then that if block will be executed and all other else…if blocks will be ignored.
- But if the condition evaluates to false, then the flow will go to the next else if statement to check its condition and if the condition evaluates to true, then the corresponding block will be executed and all other blocks will be ignored.
- And If no condition evaluates to true, then the last else block will be executed.
Code example
package main
import (
"fmt"
)
func main() {
var height int = 170
if height < 100 {
fmt.Println("Height is less than 100")
} else if height > 100 && height < 200 {
fmt.Println("Height is between than 100 and 200")
} else {
fmt.Println("Height is greater than 200")
}
fmt.Println("Outside if...else...if ladder")
}
Output –
Height is between than 100 and 200
Outside if...else...if ladder
Well, this is it for if…else statements. But we have a question in mind.
In other programming languages, it is required to surround the if statement’s condition with parentheses, but you might have noticed that we hadn’t done it here in our programs.
Well, it is up to you whether you want to add the parentheses. But the Go language doesn’t want you to add the parentheses as much as possible, so, even if you added the parentheses in your code and then run the go fmt command on your file, you will notice that it will remove your parentheses unless you are using them to set an order of operations.
Hope you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.