In this post, we are going to reverse any given array using go language.
Input arr = { 43, 12, 45, 76, 56 }
Output arr = { 56, 76, 45, 12, 43 }
Now, there are many ways to do so. We are going to discuss a few of them in this post and will also write about their respective time complexities.
- Reverse the array by using another array.
- And second is, reverse an array by swapping the elements in an iteartive way.
- And third is, reverse the array by swapping the elements using recursive approach.
Reverse the array by using another array
- Making a copy of the array that you want to reverse.
- Now, copy the last element of the copied array at the first element location of the original array.
- Then, copy the second last element of the copied array at the second element location of the original array.
- Repeat this until the array is fully iterated.
package main
import "fmt"
func main() {
arr := [...]int{43, 12, 45, 76, 56} // original array
copied_arr := arr // making the copy of the original array
lengthOfTheArray := len(arr) // finding the length of the array using len function
fmt.Println("Printing the original array:", arr)
// iterating over the array
for i := 0; i < lengthOfTheArray; i++ {
arr[i] = copied_arr[lengthOfTheArray-i-1]
}
fmt.Println("Printing the reversed array:", arr)
}
Output –
Printing the original array: [43 12 45 76 56]
Printing the reversed array: [56 76 45 12 43]
Space complexity : O(n)
Time complexity : O(1)
Reverse the array by swapping the elements
Here, instead of using another array, we will be swapping the elements within the original array itself and it will help us in improving our space complexity.
- Swap first and the last element of the array.
- Then, swap second and second last element of the array.
- Repeat this, until we have reached the middle of the array.
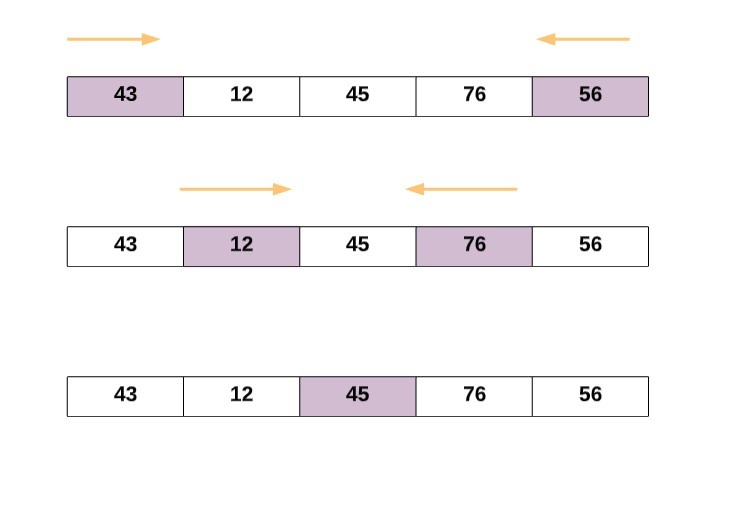
- Here to achieve this, we will be taking two variables, start index and an end index.
- Now, the start index will have the first location of the array ( 0th position ) and the end index will have the last location of the array ( array’s length – 1 ).
- And after each successful swap, we will do start++ and end– till start < end condition fails ( middle of the array ).
package main
import "fmt"
func main() {
arr := [...]int{43, 12, 45, 76, 56} // original array
start := 0
end := len(arr) - 1
fmt.Println("Printing the original array:", arr)
// iterating over the array
for start < end {
// swapping the elements present at start and end indexes
temp := arr[start]
arr[start] = arr[end]
arr[end] = temp
start++
end--
}
fmt.Println("Printing the reversed array:", arr)
}
Output –
Printing the original array: [43 12 45 76 56]
Printing the reversed array: [56 76 45 12 43]
Space complexity : O(1)
Time complexity : O(n)
Reverse the array by swapping the elements recursively
We will be using the pointers to reverse the array recursively. We will pass a pointer into the function to swap the elements by accessing the memory place directly, so, that the array retains the changes even after executing the recursive function.
package main
import "fmt"
func reverse(arr *[5]int, start int, end int) {
if start <= end {
// swapping the elements present at start and end indexes
temp := (*arr)[start]
(*arr)[start] = (*arr)[end]
(*arr)[end] = temp
reverse(arr, start+1, end-1)
}
}
func main() {
arr := [...]int{43, 12, 45, 76, 56} // original array
start := 0
end := len(arr) - 1
fmt.Println("Printing the original array:", arr)
reverse(&arr, start, end)
fmt.Println("Printing the reversed array:", arr)
}
Output –
Printing the original array: [43 12 45 76 56]
Printing the reversed array: [56 76 45 12 43]
Hope you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.