A Pointer is a variable that stores the address of any other variable. We can get the address of the variables by using the & ( an ampersand ) operator, which is also Go’s address of operator.
And why do we need pointers? It is a manual overhead to remember the address where the variable value is stored, so, instead of remembering the address, Golang provides us with an easy way of getting those addresses using pointers. Those addresses are stored as hexadecimal values as shown in the below program.
package main
import "fmt"
func main() {
var variable int = 8
fmt.Println("variable value:", variable) //printing the value of the variable
fmt.Println("address:", &variable) // printing the address of the variable where the value is stored
}
Output –
variable value: 8
address: 0xc000012088
And now, what are these “addresses”? Well, suppose you have to find a house or a shop? How will you find it out? Using an address, right? In the same way, the computer sets aside the address for each variable, which contains the value within. And the values that represent the address of a variable are known as the pointers because they point to the variable’s location.
We will look at the below points in our post.
- Pointer Types in Golang
- How to declare a pointer in Golang
- Getting and changing the variable’s value using a pointer
Let’s look at each point one by one.
Pointer Types
The pointer type would be written as a * ( asterisk ) symbol, followed by the type of variable to which the pointer will point. For example, the type of the pointer int would be written as *int ( we can read it as a pointer to int ).
We can use the reflect package TypeOf method to find the type of the pointers for various data types variables.
package main
import (
"fmt"
"reflect"
)
func main() {
var variable1 int = 8
var variable2 float64 = 13
fmt.Println("address:", reflect.TypeOf(&variable1)) // printing the type of pointer for data type "int"
fmt.Println("address:", reflect.TypeOf(&variable2)) // printing the type of pointer for data type "float64"
}
Output –
address: *int
address: *float64
Declaring a pointer
We can declare a variable that will hold the pointers as easily as declaring a normal variable.
package main
import (
"fmt"
)
func main() {
var var1 int = 8
var pointerToVar1 *int = &var1 // declaring a variable to hold a pointer to an int
fmt.Println("address of var1:", pointerToVar1)
}
Output –
address of var1: 0xc000012088
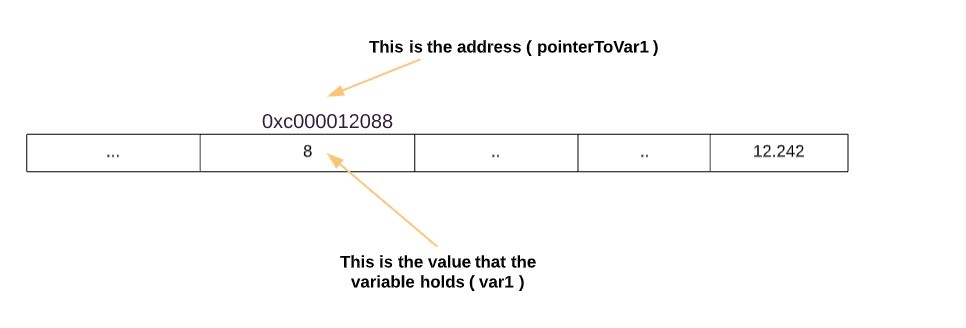
Declaring a pointer using the shorthand declaration
We can declare and initialize the pointer using the shorthand declaration ( := ). Here, the compiler will automatically detect the pointer type based on the variable’s data type, which eventually the pointer will point to.
package main
import (
"fmt"
)
func main() {
var var1 int = 8
pointerToVar1 := &var1 // using shorthand declaration here
fmt.Println("address of var1:", &var1)
fmt.Println("value of pointerToVar1", pointerToVar1)
}
Output –
address of var1: 0xc0000ac058
value of pointerToVar1 0xc0000ac058
Getting and changing the variable’s value using a pointer
How to get the variable’s value the pointer is referring to?
- We can get the variable’s value by typing the * ( asterisk ) operator right before the pointer, So, to get the value at pointerToVar1, we would write *pointerToVar1.
- * ( asterisk ) is also called the dereferencing operator, or we can also read the * operator as “value at” ( this is not an official term ). So, *pointerToVar1 would be read as value at pointerToVar1.
package main
import (
"fmt"
)
func main() {
var var1 int = 8
var pointerToVar1 *int = &var1 // declaring a variable to hold a pointer to an int
fmt.Println("address of var1:", pointerToVar1) // this will print the address
fmt.Println("value at pointerToVar1:", *pointerToVar1) // this will print the variable's value
}
Output –
address of var1: 0xc000012088
value at pointerToVar1: 8
How to change the variable’s value using pointers?
We can easily update the variable’s value using the pointers. Changing the value present at the address using the pointers is changing the variable’s value itself, as shown in the below program.
package main
import (
"fmt"
)
func main() {
var var1 int = 8
pointerToVar1 := &var1 // using shorthand declaration here
fmt.Println("value at pointerToVar1 before:", *pointerToVar1)
*pointerToVar1 = 15
fmt.Println("value at pointerToVar1 now:", *pointerToVar1)
fmt.Println("value of var1:", var1)
}
Output –
value at pointerToVar1 before: 8
value at pointerToVar1 now: 15
value of var1: 15
What is the default value or zero value of a pointer in golang?
The default value or zero value of a pointer is nil.
package main
import (
"fmt"
)
func main() {
var pointer *int
fmt.Println("pointer value:", pointer)
}
Output –
pointer value: <nil>
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.