The trimToSize() method is used to trim the capacity of an ArrayList to its current size. In this post, we are going to discuss the trimToSize() method in detail.
- Method declaration – public void trimToSize()
- What does it do? It is used to trim the capacity of an ArrayList to its current size. So, after using the trimToSize() method, the capacity of the ArrayList becomes equal to the size of the ArrayList
- What does it return? It does not return anything as its return type is void
A bit about capacity and size
Let’s create an ArrayList.
ArrayList<String> al = new ArrayList<String>();
Now, this is an empty ArrayList whose size is 0 which can be confirmed by using the size() method but the capacity of this ArrayList is 10. ArrayList assigns a default capacity of 10 to every ArrayList unless we explicitly assign it by using the parameterized constructor at the time of creating the ArrayList.
Whenever new elements are added to the list, the size increases but capacity remains the same. But, when the capacity becomes equal to that of the size and new elements need to be added to the list, then capacity has to be increased further to accommodate new elements.
So, we can say that the capacity >= size of an ArrayList. Now, where does trimToSize() come into the picture?
- If we want to make capacity equal to that of the size of ArrayList, there we can use the trimToSize() method.
- And, if we are sure that no new elements will be added to the ArrayList afterward, then we can use trimToSize() to free up the space acquired by the capacity.
Code example
public class Codekru {
public static void main(String[] args) throws Exception {
ArrayList<String> al = new ArrayList<String>();
al.add("first");
al.add("second");
al.add("third");
al.trimToSize(); // making capacity = size = 3
System.out.println("Size of ArrayList: " + al.size());
}
}
Output –
Size of ArrayList: 3
Before using the trimToSize() method
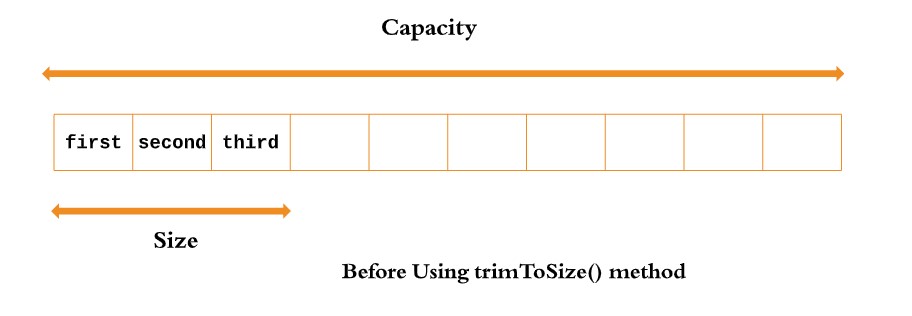
After using the trimToSize() method
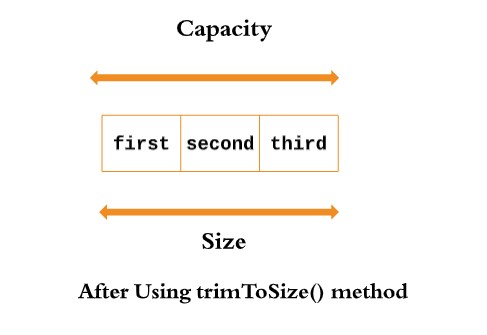
The What If scenarios
What if we use the trimToSize() method on a null ArrayList?
It will throw a NullPointerException.
public class Codekru {
public static void main(String[] args) throws Exception {
ArrayList<String> al = new ArrayList<String>();
al = null;
al.trimToSize();
}
}
Output –
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "java.util.ArrayList.trimToSize()" because "al" is null
What if we use the trimToSize() method on an empty ArrayList?
An empty ArrayList has a default capacity of 10. So, after using the trimToSize() method on it, the capacity of the list would be reduced to 0.
public class Codekru {
public static void main(String[] args) throws Exception {
ArrayList<String> al = new ArrayList<String>();
al.trimToSize(); // this will reduce the capacity to 0
System.out.println("Reduced the capacity to 0");
}
}
Output –
Reduced the capacity to 0
Please visit this link if you want to know more about the ArrayList class of java and its other functions or methods.
Hope you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.