The decode() method is a static method of the Integer class that converts a string into an Integer instance. This post will look at the decode() method in detail and the difference between the decode() method and the valueOf() method of the Integer class.
- Method declaration – public static Integer decode(String nm)
- What does it do? – This static method can convert a string into an Integer instance representing that string value. This function also accepts hexadecimal and octal strings along with the normal decimal strings. We will cover the examples for each of those.
- What does it return? It will return an instance of the Integer class representing the string value passed in the argument.
Decoding the decimal strings
The decimal string should only contain the decimal characters and the optional positive or negative sign at the start. If it contains any other character, the decode() method will throw a NumberFormatException.
public class Codekru {
public static void main(String[] args) {
String s1 = "123";
String s2 = "-123";
String s3 = "+123";
System.out.println("Integer representing string s1: " + Integer.decode(s1));
System.out.println("Integer representing string s2: " + Integer.decode(s2));
System.out.println("Integer representing string s3: " + Integer.decode(s3));
}
}
Output –
Integer representing string s1: 123
Integer representing string s2: -123
Integer representing string s3: 123
Decoding the HexaDecimal strings
We can also pass the Hexadecimal strings to the decode function, which will be decoded using the radix as 16. We can pass the Hexadecimal strings in the below formats.
1) Starting with "0x", Eg. 0x123
2) Starting with "0X", Eg. 0X123
3) Starting with "#", Eg. #123
All of the above formats will be treated as Hexadecimal strings by the decode() function.
public class Codekru {
public static void main(String[] args) {
String s1 = "0x123";
String s2 = "0X123";
String s3 = "#123";
System.out.println("Integer representing string s1: " + Integer.decode(s1));
System.out.println("Integer representing string s2: " + Integer.decode(s2));
System.out.println("Integer representing string s3: " + Integer.decode(s3));
}
}
Output –
Integer representing string s1: 291
Integer representing string s2: 291
Integer representing string s3: 291
The “123” string is converted using the radix 16, and the result was 291. The below representation will help in further clarification of the same.
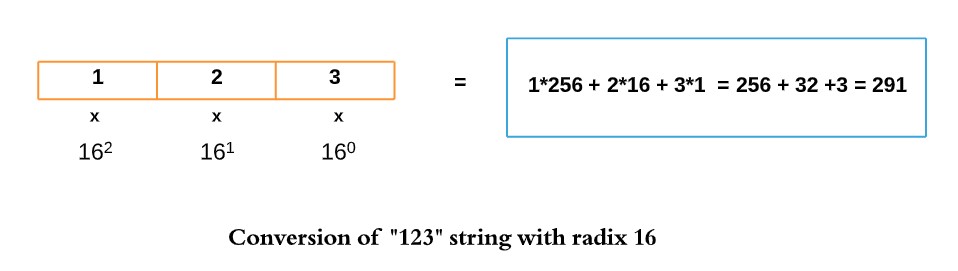
Decoding the Octal strings
The octal strings must start from “0” ( zero ), and the radix used for converting them would be 8.
public class Codekru {
public static void main(String[] args) {
String s1 = "0123";
// 1*(8^2) + 2*(8^1) + 3*(8^0) = 64+16+3 = 83
System.out.println("Integer representing string s1: " + Integer.decode(s1));
}
}
Output –
Integer representing string s1: 83
Internal implementations of the decode() method
public static Integer decode(String nm) throws NumberFormatException {
int radix = 10;
int index = 0;
boolean negative = false;
Integer result;
if (nm.isEmpty())
throw new NumberFormatException("Zero length string");
char firstChar = nm.charAt(0);
// Handle sign, if present
if (firstChar == '-') {
negative = true;
index++;
} else if (firstChar == '+')
index++;
// Handle radix specifier, if present
if (nm.startsWith("0x", index) || nm.startsWith("0X", index)) {
index += 2;
radix = 16;
}
else if (nm.startsWith("#", index)) {
index ++;
radix = 16;
}
else if (nm.startsWith("0", index) && nm.length() > 1 + index) {
index ++;
radix = 8;
}
if (nm.startsWith("-", index) || nm.startsWith("+", index))
throw new NumberFormatException("Sign character in wrong position");
try {
result = Integer.valueOf(nm.substring(index), radix);
result = negative ? Integer.valueOf(-result.intValue()) : result;
} catch (NumberFormatException e) {
// If number is Integer.MIN_VALUE, we'll end up here. The next line
// handles this case, and causes any genuine format error to be
// rethrown.
String constant = negative ? ("-" + nm.substring(index))
: nm.substring(index);
result = Integer.valueOf(constant, radix);
}
return result;
}
The What If Scenarios
Q – What If we pass an empty string within the decode() function argument?
Here we will get a NumberFormatException as illustrated by the below program.
public class Codekru {
public static void main(String[] args) {
String s1 = "";
System.out.println("Integer representing string s1: " + Integer.decode(s1));
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: Zero length string
at java.base/java.lang.Integer.decode(Integer.java:1407)
Q – What If we place a negative sign after the prefixes( “0x-123” ) for HexaDecimal strings?
public class Codekru {
public static void main(String[] args) {
String s1 = "0x-123";
System.out.println("Integer representing string s1: " + Integer.decode(s1));
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: Sign character in wrong position
at java.base/java.lang.Integer.decode(Integer.java:1431)
It would have worked fine if we had used “-0x123” instead of “0x-123”.
What is the difference between decode() vs valueOf() methods?
- valueOf() method does not accept the prefixes “0x”,”0X”,”#”, and “0” for Hexadecimal and octal strings, whereas the decode() method does. If we try to pass the string with these prefixes into the valueOf() method, then we will get a NumberFormatException
- decode() method internally uses the valueOf() method to convert the string into the corresponding Integer instance.
Please visit this link to learn more about the Integer wrapper class of java and its other functions or methods.
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.