The numberOfLeadingZeros() is a static method of the Integer wrapper class that counts the number of zero bits preceding the highest-order (“leftmost”) one-bit in the two’s complement binary representation. In this post, we are going to look at the numberOfLeadingZeros() method in detail.
- Method declaration – public static int numberOfLeadingZeros(int i)
- What does it do? – It will count the number of zero bits preceding the highest-order (“leftmost”) one-bit in the two’s complement binary representation of the int value passed in the argument.
- It will return 32 if the number passed in the argument has no 1-bit set in the 2’s complement of the binary representation, or we can say the number passed in the argument is 0
- What does it return? It will return the count of the number of zero bits preceding the highest-order (“leftmost”) one-bit in the two’s complement binary representation of the int value passed in the argument.
Code Example
public class Codekru {
public static void main(String[] args) {
int i = 21332;
System.out.println("No. of zero bits preceding the leftmost 1-bit in 2's complement representation: "
+ Integer.numberOfLeadingZeros(i));
}
}
Output –
No. of zero bits preceding the leftmost 1-bit in 2's complement representation: 17
Here, the leading zero bits are counted concerning the 32-bit representation. The 2’s complement binary representation of 21332 is 00000000000000000101001101010100. And so, the number of zeros preceding the leftmost 1 bit is 15.
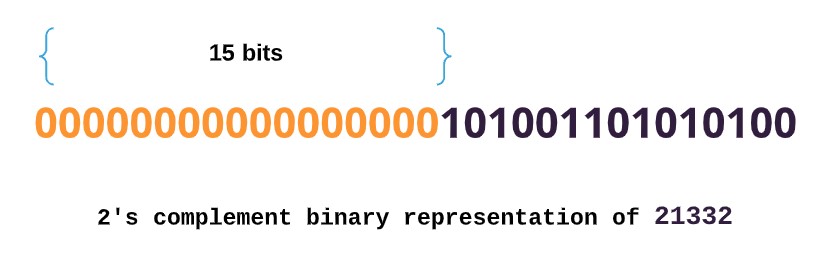
How to calculate 2’s complement of a negative number?
- Positive numbers are just represented by their binary strings but negative numbers are represented by using 2’s complement
- To find the 2’s complement of a number, we first have to know how many bits we are working with. In our case, the number of bits is 32
- Let’s try to find the 2’s complement of the number -30
- For this, we first have to write the binary representation of the number 30
0000 0000 0000 0000 0000 0000 0001 1110
- Invert the binary digits
1111 1111 1111 1111 1111 1111 1110 0001
- Now, add one to it
1111 1111 1111 1111 1111 1111 1110 0010
The What If Scenarios
Q – What if we pass 0 into the numberOfLeadingZeros() argument?
The function will return 32 as the number of one bit is zero and all of the bits will be counted as leading bits.
public class Codekru {
public static void main(String[] args) {
System.out.println("No. of zero bits preceding the leftmost 1-bit in 2's complement representation: "
+ Integer.numberOfLeadingZeros(0));
}
}
Output –
No. of zero bits preceding the leftmost 1-bit in 2's complement representation: 32
Q – What if we pass a negative number into the numberOfLeadingZeros() argument?
In this case, numberOfLeadingZeros() would return 0 as the leftmost bit of the 2’s complement representation of a negative number is 1. And so, there will be no 0 bits preceding the 1 bit in case of negative numbers.
public class Codekru {
public static void main(String[] args) {
int i = -30;
System.out.println("No. of zero bits preceding the leftmost 1-bit in 2's complement representation: "
+ Integer.numberOfLeadingZeros(i));
}
}
Output –
No. of zero bits preceding the leftmost 1-bit in 2's complement representation: 0
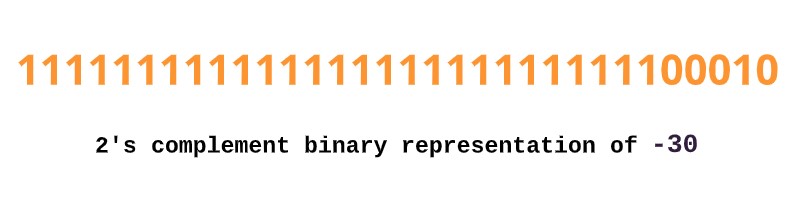
Please visit this link if you want to know more about the Integer wrapper class of java and its other functions or methods.
Hope you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.