In the previous post, we learned how to make our own custom Linked list, and in this post, we will be learning about the inbuilt linked list class in Java, which is a part of the Java Collection framework. This Linked list class is present in java.util package.
First, let’s learn about what is a linked list –
- A linked list is a linear data structure that is used to store elements at non-contiguous memory locations
- A linked list is made up of nodes and each node is made up of two things
- Data
- Address
- Data contains the actual value that is to be stored in the linked list
- And the Address will contain the address of the next node or the previous node, depending on whether it is a singly linked list or a doubly linked list
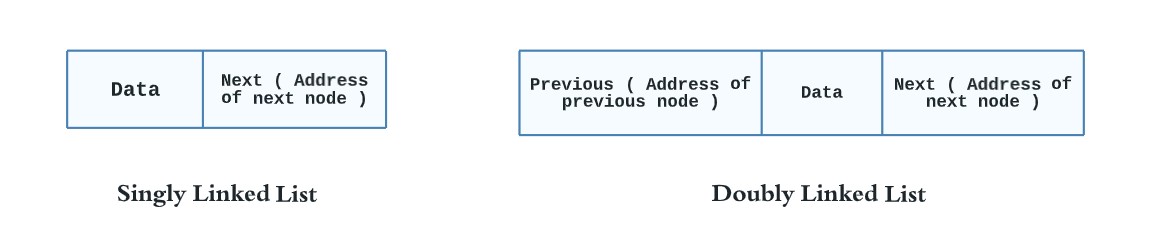
There are three types of linked lists in general that we would like to point out here –
- Singly-linked list – A node only has a reference to the next node in a singly linked list. So, we can only move in one direction in a singly linked list.

- Doubly linked list – A node references both the previous and the subsequent node in a doubly linked list, except the head node or the first node, which only has a reference to the next node in the linked list. So, we can move in both directions in a doubly linked list.

- Circular linked list – A circular linked list is a singly linked list, with the difference that the last node points back to the first(head) node.

The inbuilt linked list class is a doubly-linked list, which means it can move in either direction to perform various operations. There are other features of Linked List in java –
- It maintains the insertion order.
- It is non-synchronized.
Declaration of Linked List class
public class LinkedList<E>
extends AbstractSequentialList<E>
implements List<E>, Deque<E>, Cloneable, java.io.Serializable
Now, here you can see that it extends the AbstractSequentialList class and implements the List, Deque interfaces. As List and Deque internally extends the Collection interface, the Linked List class also has to override the unimplemented methods of the Collection interface.
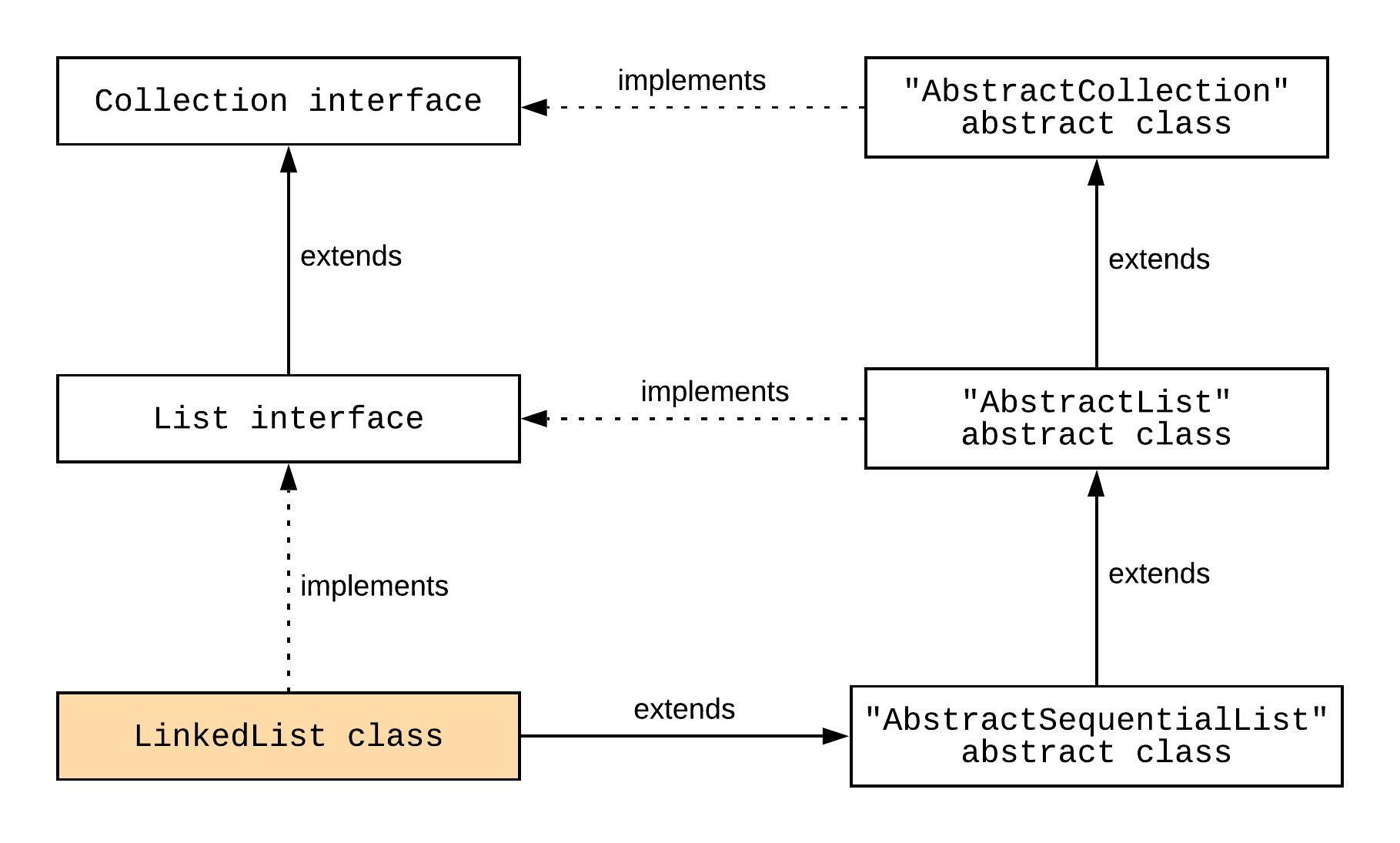
How to make Linked List Objects or call its constructors
There are two ways of creating a Linked list class object, one is by using the default constructor, and another one is by using the parameterized constructor.
The default constructor of the Linked List class –
It will create an empty linked list.
/**
* Constructs an empty list.
*/
public LinkedList() {
}
The parameterized constructor of the Linked List class –
It will take the collection as the argument.
/**
* Constructs a list containing the elements of the specified
* collection, in the order they are returned by the collection's
* iterator.
*
* @param c the collection whose elements are to be placed into this list
* @throws NullPointerException if the specified collection is null
*/
public LinkedList(Collection<? extends E> c) {
this();
addAll(c);
}
Now to make a linked list, we can use the default constructor as shown below –
LinkedList<String> linkedList = new LinkedList<String>();
Here, <String> is telling the linked list class that it will contain elements of type String. Here we can use any class, but don’t try to use primitive like int, float, double, etc., as it will throw you an error 😛
Linked list class methods
There are many helper methods in Linked last to facilitate various things. Some of them are listed below –
codekru.com/…/linked-list-contains-and-containsall-method
Function Name (Method name) | What it does |
---|---|
add(E e) | Appends the specified element to the end of this list. |
add(int index, E element) | Inserts the specified element at the specified position in this list. Shifts the element currently at that position (if any) and any subsequent elements to the right (adds one to their indices). |
addAll(Collection c) | Appends all of the elements in the specified collection to the end of this list, in the order that the specified collection’s iterator returns them. The behavior of this operation is undefined if the specified collection is modified while the operation is in progress. |
addAll(int index, Collection c) | Inserts all of the elements in the specified collection into this list, starting at the specified position. Shifts the element currently at that position (if any) and any subsequent elements to the right (increases their indices). |
addFirst(E e) | Inserts the specified element at the beginning of this list. |
addLast(E e) | Appends the specified element to the end of this list. This method is equivalent to the add method. |
clear() | Removes all of the elements from this list. The list will be empty after this call returns. |
clone() | Returns a shallow copy of the linked list (The elements themselves are not cloned.) |
contains(Object o) | Returns true if the list contains the specified element; otherwise false. |
containsAll(Collection c) | This implementation iterates over the specified collection, checking each element returned by the iterator, in turn, to see if it’s contained in this collection. If all elements are so contained true is returned, otherwise, false. |
descendingIterator() | Adapter to provide descending iterators via ListItr.previous. It came in Java 1.6 and it will iterate in the reverse direction of element insertion in the Linked List. |
element() | Retrieves, but does not remove, the list’s head (first element). |
equals(Object o) | Compares the specified object with the list for equality. Returns true if and only if the specified object is also a list, both lists have the same size, and all corresponding pairs of elements in the two lists are equal. |
get(int index) | Returns the element at the specified position in the list. |
getFirst() | Returns the first element in this list. |
getLast() | Returns the last element in this list. |
indexOf(Object o) | Returns the index of the first occurrence of the specified element in the list, or -1 if the list does not contain the element. |
isEmpty() | Returns true if the list is empty. otherwise false. |
iterator() | Returns an iterator over the elements in this list. |
lastIndexOf(Object o) | Returns the index of the last occurrence of the specified element in the list, or -1 if the list does not contain the element. |
offer(E e) | Adds the specified element as the tail (last element) of this list. |
offerFirst(E e) | Inserts the specified element at the front of this list. |
offerLast(E e) | Inserts the specified element at the end of this list. |
peek() | Retrieves, but does not remove, the head (first element) of this list. |
peekFirst() | Retrieves, but does not remove, the first element of this list, or returns null if this list is empty. |
peekLast() | Retrieves, but does not remove, the last element of this list, or returns null if this list is empty. |
poll() | Retrieves and removes the head (first element) of this list. |
pollFirst() | Retrieves and removes the first element of this list, or returns null if this list is empty. |
pollLast() | Retrieves and removes the last element of this list, or returns null if this list is empty. |
pop() | Pops an element from the stack represented by this list. In other words, removes and returns the first element of this list. This method is equivalent to removeFirst(); |
push(E e) | Pushes an element onto the stack represented by this list. In other words, inserts the element at the front of this list. This method is equivalent to addFirst(E e); |
remove() | Retrieves and removes the head (first element) of this list. |
remove(int index) | Removes the element at the specified position in this list. Shifts any subsequent elements to the left (subtracts one from their indices). |
remove(Object o) | Removes the first occurrence of the specified element from this list, if it is present. If this list does not contain the element, it is unchanged |
removeAll(Collection c) | This implementation iterates over this collection, checking each element returned by the iterator, in turn, to see if it’s contained in the specified collection. If it’s so contained, it’s removed from this collection with the iterator’s remove method. |
removeFirst() | Removes and returns the first element from this list. |
removeFirstOccurrence(Object o) | Removes the first occurrence of the specified element in the list |
removeLast() | Removes and returns the last element from this list. |
removeLastOccurrence(Object o) | Removes the last occurrence of the specified element in the list. |
set(int index, E element) | Replaces the element at the specified position in this list with the specified element. |
size() | Returns the number of elements in this list. |
sort(Comparator c) | Sorts this list according to the order induced by the specified comparator. The sort is stable: this method must not reorder equal elements. |
toArray() | Returns an array containing all of the elements in this list in proper sequence (from first to the last element). |
toArray(T[] a) | Returns an array containing all of the elements in this list in proper sequence (from first to the last element); the runtime type of the returned array is that of the specified array. If the list fits in the specified array, it is returned therein. Otherwise, a new array is allocated with the runtime type of the specified array and the size of this list. |
toString() | Returns a string representation of the list. Adjacent elements are separated by the characters “, ” (comma and space) |
Hope you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.