In our previous article, we have written about the maven goals, plugins, phases, and lifecycle. In this post, we will dig deeper into the maven build lifecycle.
A build lifecycle is a well-defined sequence of phases to execute the goals associated with each phase. A phase is a step in the build lifecycle. There are three standard maven lifecycles –
Clean lifecycle
Running “mvn clean” will invoke the clean lifecycle of maven. It consists of three phases –
- pre-clean
- clean
- post-clean
The clean’s plugin clean goal is bound to the clean phase in the clean lifecycle. But directly executing the clean:clean goal will not run the pre-clean or the post-clean phase of the clean lifecycle.
Now, you might be wondering what does the clean phase even does?
The clean phase deletes the output of a build by deleting the build directory. By default, it is {base_dir}/target directly as defined by the Super POM unless you have customized it.
Why would we need the pre-clean or post-clean phase?
Sometimes, we might have to archive the build directory before deleting it, or we might have to echo a notification before or after cleaning the project. The pre-clean and post-clean phases can help us in achieving the same.
Now, let’s try to echo the text messages while executing each phase of the clean lifecycle. We can echo the text messages by using the maven antrun plugin, which we will also be using in our example. We will have to execute the command “mvn post-clean” because the post-clean phase will execute pre-clean and clean phases before executing the post-clean phase. So, in this way, all phases would be executed.
Below is our pom.xml file.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.website.codekru</groupId>
<artifactId>DemoProject</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>DemoProject</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-antrun-plugin</artifactId>
<version>1.1</version>
<executions>
<execution>
<id>id.pre-clean</id>
<phase>pre-clean</phase>
<goals>
<goal>run</goal>
</goals>
<configuration>
<tasks>
<echo>Executing pre-clean phase</echo>
</tasks>
</configuration>
</execution>
<execution>
<id>id.clean</id>
<phase>clean</phase>
<goals>
<goal>run</goal>
</goals>
<configuration>
<tasks>
<echo>Executing clean phase</echo>
</tasks>
</configuration>
</execution>
<execution>
<id>id.post-clean</id>
<phase>post-clean</phase>
<goals>
<goal>run</goal>
</goals>
<configuration>
<tasks>
<echo>Executing post-clean phase</echo>
</tasks>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
After running the “mvn post-clean” command, we will see the below output in the terminal.

We can also delete the additional files and the build directory contents while executing the clean phase. Let’s try to delete all the files under a custom-made folder named “testFolder“.
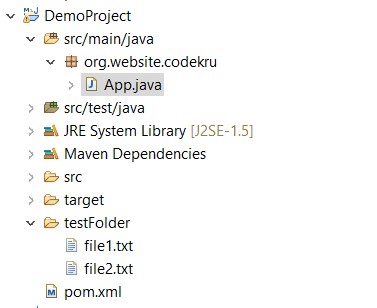
Below is our pom.xml file, which is used to delete the files inside the “testFolder” directory and the build directory files.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.website.codekru</groupId>
<artifactId>DemoProject</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>DemoProject</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-clean-plugin</artifactId>
<version>3.2.0</version>
<type>maven-plugin</type>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<artifactId>maven-clean-plugin</artifactId>
<version>3.2.0</version>
<configuration>
<filesets>
<fileset>
<directory>testFolder</directory>
<includes>
<include>*</include>
</includes>
</fileset>
</filesets>
</configuration>
</plugin>
</plugins>
</build>
</project>
Now, run the “mvn clean” command, and the files under testFolder will also be deleted.

Now, let’s move on to our next build lifecycle.
Default lifecycle
This is the most familiar and primary lifecycle of maven. It starts with the validate phase and ends with the deploy phase.
Phase | Description |
---|---|
validate | It validates whether the project is correct or not, and all necessary information is available to complete a build |
initialize | It initializes the build state, for example, set properties or creating directories |
generate-sources | It generates any source code for inclusion in the compilation |
process-sources | It processes the source code, for example, to filter any values |
generate-resources | It generates the resources for inclusion in the package. |
process-resources | It copies and processes the resources into the destination directory, ready for packaging. |
compile | It compiles the source code of the project. |
process-classes | It post-processes the generated files from compilation, for example, to do bytecode enhancement on Java classes. |
generate-test-sources | It generates any test source code for inclusion in compilation. |
process-test-sources | It processes the test source code, for example, to filter any values. |
generate-test-resources | It creates the resources for testing. |
process-test-resources | It copies and processes the resources into the test destination directory. |
test-compile | It compiles the test source code into the test destination directory |
process-test-classes | It post-processes the generated files from test compilation, for example, to do bytecode enhancement on Java classes. |
test | It runs tests using a suitable unit testing framework. These tests should not require the code to be packaged or deployed. |
prepare-package | It performs any operations necessary to prepare a package before the actual packaging. This often results in an unpacked, processed version of the package. |
package | It packages the compiled code in its distributable format, such as a JAR. |
pre-integration-test | It performs actions required before integration tests are executed. This may involve things such as setting up the required environment. |
integration-test | It processes and deploys the package if necessary into an environment where integration tests can be run. |
post-integration-test | It performs actions required after integration tests have been executed. This may include cleaning up the environment. |
verify | It runs any checks to verify the package is valid and meets quality criteria. |
install | It installs the package into the local repository for use as a dependency in other projects locally. |
deploy | It is done in an integration or release environment and copies the final package to the remote repository for sharing with other developers and projects. |
Running a phase will execute all the phases up to that phase. For Example, running “mvn install” would run all phases up to the install phase.
Each phase may be linked with zero or more plugin goals, and executing a particular phase is equivalent to running that phase’s plugin goals. E.g., executing the install phase is like executing the “install:install” goal. But running the “mvn install” and “mvn install:install” commands is not the same because “mvn install” will execute all of the phases up to the install phase. Thus all goals associated with each phase would also be executed, but “mvn install:install” will execute this specific goal only.
Below is the list of some most commonly remembered phases and the goals attached to them.
Phase | plugin:goal |
---|---|
process-resources | resources:resources |
compile | compiler:compile |
process-test-resources | resources:testResources |
test-compile | compiler:testCompile |
test | surefire:test |
package | ejb:ejb or ejb3:ejb3 or jar:jar or par:par or rar:rar or war:war |
install | install:install |
deploy | deploy:deploy |
If you want to know about plugins and goals, please look at this article. And now, without further ado, let’s move on to our lifecycle.
Site lifecycle
Maven does more than build software artifacts from projects. It can also generate project documentation and reports about the project. Project documentation and site generation have a specific lifecycle dedicated to them which consists of four phases.
- pre-site
- site
- post-site
- site-deploy
Plugin goals attached to the site lifecycle phases are –
- site ( site:site)
- site-deploy ( site:deploy )
The type of packaging usually does not alter the site lifecycle since packaging types are concerned primarily with artifact creation, not with the type of site generated.
We can generate a site from the maven project by running the “mvn site” command.
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.