This post will discuss how we can get the coordinates of a web element on a webpage. An element is part of a rectangular box, so when we say that these are the XY coordinates of an element, this represents the top left-hand corner of that rectangle.
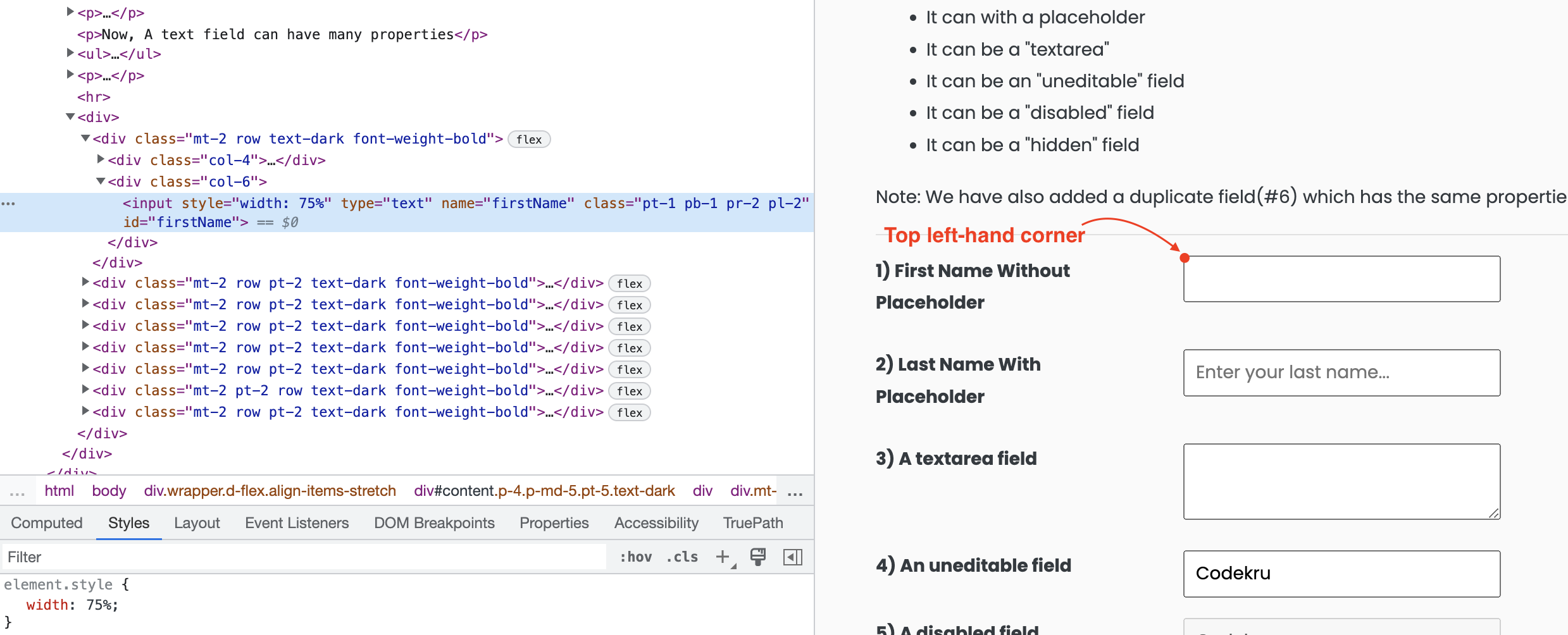
We can find the top left-hand corner of the element using several ways.
Let’s have a look at them one by one.
Using getLocation() method
We have discussed the getLocation() method in a different post where we wrote about the getLocation() method in detail. To summarize it, getLocation() returns us a Point class object which provides us a getX() and getY() methods to get the X and Y coordinates of the element.
Let’s get the XY coordinates of the highlighted element in the below image. You can also check it for yourself on this page – https://testkru.com/Elements/TextFields.

import org.openqa.selenium.By;
import org.openqa.selenium.Point;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("firstName"));
Point point = element.getLocation();
System.out.println("x coordinate: " + point.getX());
System.out.println("y coordinate: " + point.getY());
}
}
Output –
x coordinate: 532
y coordinate: 427
Using getRect() method
We have also written a detailed article on the getRect() method. getRect() method returns a Rectangle class object representing the rectangle box that encompasses the element.
Rectangle class also provides the getX() and getY() methods to know about an element’s X and Y coordinates.
import org.openqa.selenium.By;
import org.openqa.selenium.Rectangle;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("firstName"));
Rectangle rect = element.getRect();
System.out.println("x coordinate: " + rect.getX());
System.out.println("y coordinate: " + rect.getY());
}
}
Output –
x coordinate: 532
y coordinate: 427
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.