This post will discuss the numerous ways using which we can know whether a checkbox is checked or not.
- Using isSelected() method
- By using getAttribute() method
- and using the JavascriptExecutor
Let’s look at all of the ways one by one.
Using isSelected() method
We have written a different article on the isSelected() method, discussing it in detail. But in a nutshell, the isSelected() method can be used on a checkbox, radio button, or a select option to see if they are selected or not.
Let’s see this with an example now. We have highlighted one checkbox, which is checked in the below image, and now we will use the isSelected() method t see whether the checkbox is checked or not.
You will be able to find the element on this page – https://testkru.com/Elements/Checkboxes.
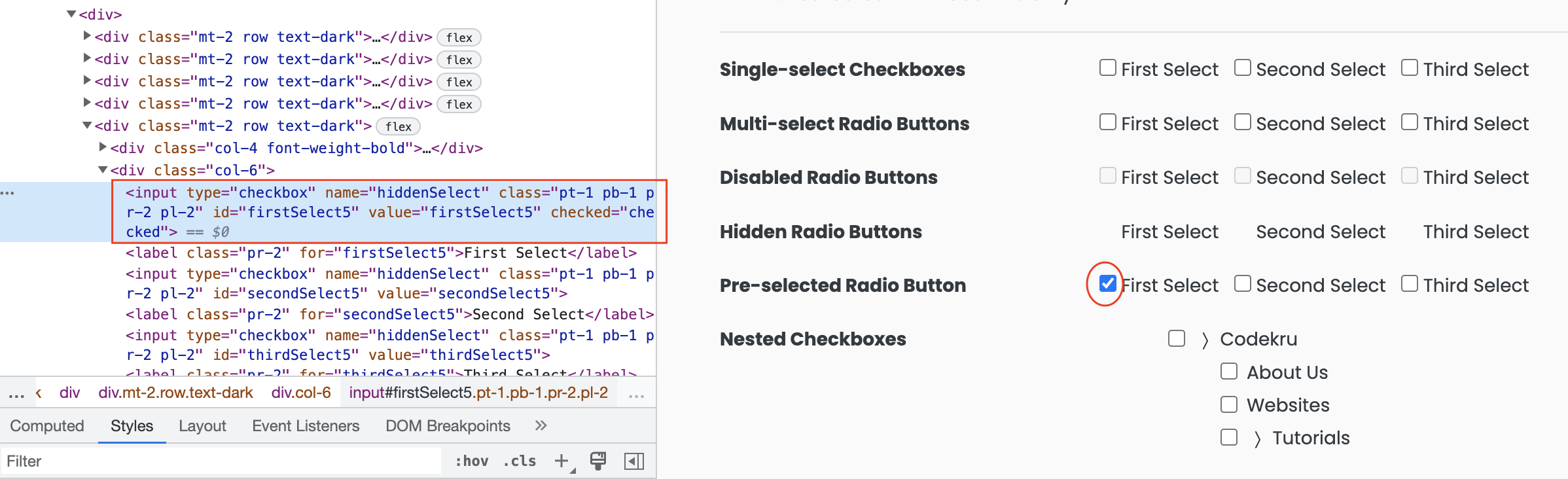
- First, we will find the element using the id attribute. Here the id of our element is firstSelect5.
- And then, we can use the isSelected() method on the element.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Checkboxes");
WebElement element = driver.findElement(By.id("firstSelect5"));
System.out.println("Is checkbox checked: " + element.isSelected());
}
}
Output –
Is checkbox checked: true
We can see that isSelected() method returned true because the checkbox was checked. If the checkbox isn’t checked, it would have returned false.
Now, let’s see how we can now achieve the same thing with the getAttribute() method.
Using getAttribute() method
We have also written a detailed post on the getAttribute() method. Please go through that article to learn about the getAttribute() method. It is used to get an element’s property and attribute value.
There is a checked attribute in HTML that will return true if a checkbox is checked and false if not. We will get the checked attribute’s value using the getAttribute() method to know if a checkbox is checked or not.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Checkboxes");
WebElement element = driver.findElement(By.id("firstSelect5"));
System.out.println("Is checkbox checked: " + element.getAttribute("checked"));
}
}
Output –
Is checkbox checked: true
There is one more way to get the checked attribute value, and that is by using the JavascriptExecutor.
Using JavascriptExecutor
JavascriptExecutor helps execute the javascript code in Selenium. The below script will help get the checked attribute value.
return arguments[0].checked
where arguments[0] is the element for which we want to check whether the checkbox is checked or not.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Checkboxes");
WebElement element = driver.findElement(By.id("firstSelect5"));
JavascriptExecutor jse = (JavascriptExecutor) driver;
System.out.println("Is checkbox checked: "+jse.executeScript("return arguments[0].checked", element));
}
}
Output –
Is checkbox checked: true
This is it. We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.