In this post, we will discuss how we can ignore the test cases in TestNG. TestNG helps us ignore the cases annotated with @Test annotation, and we can ignore these cases at various levels.
- First, Ignore only a test method or test case.
- Second, Ignore all cases within a class and its subclasses.
- And the third is, Ignore all cases within a package and its subpackages.
Let’s look at the above points one by one.
Ignore only a test method or test case
This can be achieved using @Test(enabled = false) or @Ignore annotation on the test case or method.
import org.testng.Assert;
import org.testng.annotations.Ignore;
import org.testng.annotations.Test;
public class CodekruTest {
@Test @Ignore
public void test1() {
System.out.println("Excecuting test1");
Assert.assertTrue(true);
}
@Test
public void test2() {
System.out.println("Excecuting test2");
Assert.assertTrue(true);
}
@Test(enabled = false)
public void test3() {
System.out.println("Excecuting test3");
Assert.assertTrue(true);
}
}
Output –
Excecuting test2
PASSED: test2
===============================================
Default test
Tests run: 1, Failures: 0, Skips: 0
===============================================
So, both ( test1 and test3 ) were ignored, and only the test2 method was run and executed.
Ignore all cases within a class and its subclasses
This can also be achieved by using the @Ignore annotation. So, if we place @Ignore annotation on a class, then the cases within that class and its subclasses will be ignored. So, let’s see this with an example.
Note: @Ignore annotation has a higher priority than individual @Test method annotations. When @Ignore is placed on a class, all the tests in that class will be disabled, even if we place @Test( enabled = true) on a Test case.
package Test;
import org.testng.Assert;
import org.testng.annotations.Ignore;
import org.testng.annotations.Test;
@Ignore
public class CodekruTest {
@Test
public void test1() {
System.out.println("Excecuting test1");
Assert.assertTrue(true);
}
@Test
public void test2() {
System.out.println("Excecuting test2");
Assert.assertTrue(true);
}
@Test(enabled = true)
public void test3() {
System.out.println("Excecuting test3");
Assert.assertTrue(true);
}
}
Output –
===============================================
Default test
Tests run: 0, Failures: 0, Skips: 0
===============================================
===============================================
Default suite
Total tests run: 0, Failures: 0, Skips: 0
===============================================
[TestNG] No tests found. Nothing was run
So, here we can see that none of the cases was executed. Now. Let’s make a subclass(CodekruTestSubclass) of the above class(CodekruTest), try to execute the subclass test cases, and see what happens.
import org.testng.Assert;
import org.testng.annotations.Ignore;
import org.testng.annotations.Test;
class CodekruTestSubclass extends CodekruTest{
@Test
public void test1() {
System.out.println("Excecuting test1 of subclass");
Assert.assertTrue(true);
}
@Test
public void test2() {
System.out.println("Excecuting test2 of subclass");
Assert.assertTrue(true);
}
}
@Ignore
public class CodekruTest {
@Test
public void test1() {
System.out.println("Excecuting test1");
Assert.assertTrue(true);
}
@Test
public void test2() {
System.out.println("Excecuting test2");
Assert.assertTrue(true);
}
@Test(enabled = true)
public void test3() {
System.out.println("Excecuting test3");
Assert.assertTrue(true);
}
}
Output –
===============================================
Default test
Tests run: 0, Failures: 0, Skips: 0
===============================================
===============================================
Default suite
Total tests run: 0, Failures: 0, Skips: 0
===============================================
[TestNG] No tests found. Nothing was run
Here, the cases of subclass didn’t execute because we have used @Ignore annotation on the parent class.
Ignore all cases within a package and its subpackages
We can also ignore the test cases in a package and its subpackages. But, we can’t just put the @Ignore annotation at the top of our package, as shown in the below code. Instead, we have to make another package-info.java file and add the package-level annotation.
@Ignore // this is wrong and is throwing an error
package Test;
import org.testng.Assert;
import org.testng.annotations.Test;
public class CodekruTest {
@Test
public void test1() {
System.out.println("Excecuting test1");
Assert.assertTrue(true);
}
}
How to create a package-info.java file? As eclipse won’t allow you to make a package-info class file.
- Select New -> Package.
- Now, tick the checkbox package-info.java
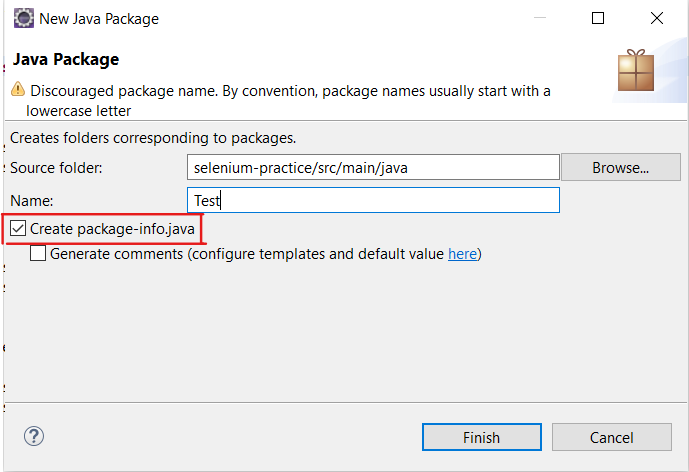
This will create a package-info.java file in the package mentioned above. So, now in this file, we can write @Ignore on the Test package as shown below.
@org.testng.annotations.Ignore
package Test;
So, all cases in this package and its sub packages will now be ignored.
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.