Sometimes we might need to send emails not by using traditional logging into an account and sending it but via code. This post will discuss how we can send emails using the SMTP server in Java.
We will use a Gmail Account, using which we will send emails via SMTP. Google has changed its policies to access Google accounts, and this post will cover the latest approach to sending emails through a Gmail account using Java.
We will follow the below steps to send an email –
Create an app password for your account
- Sign in to your Gmail account.
- Go to Security.
- Now, scroll to Signing in to Google area.
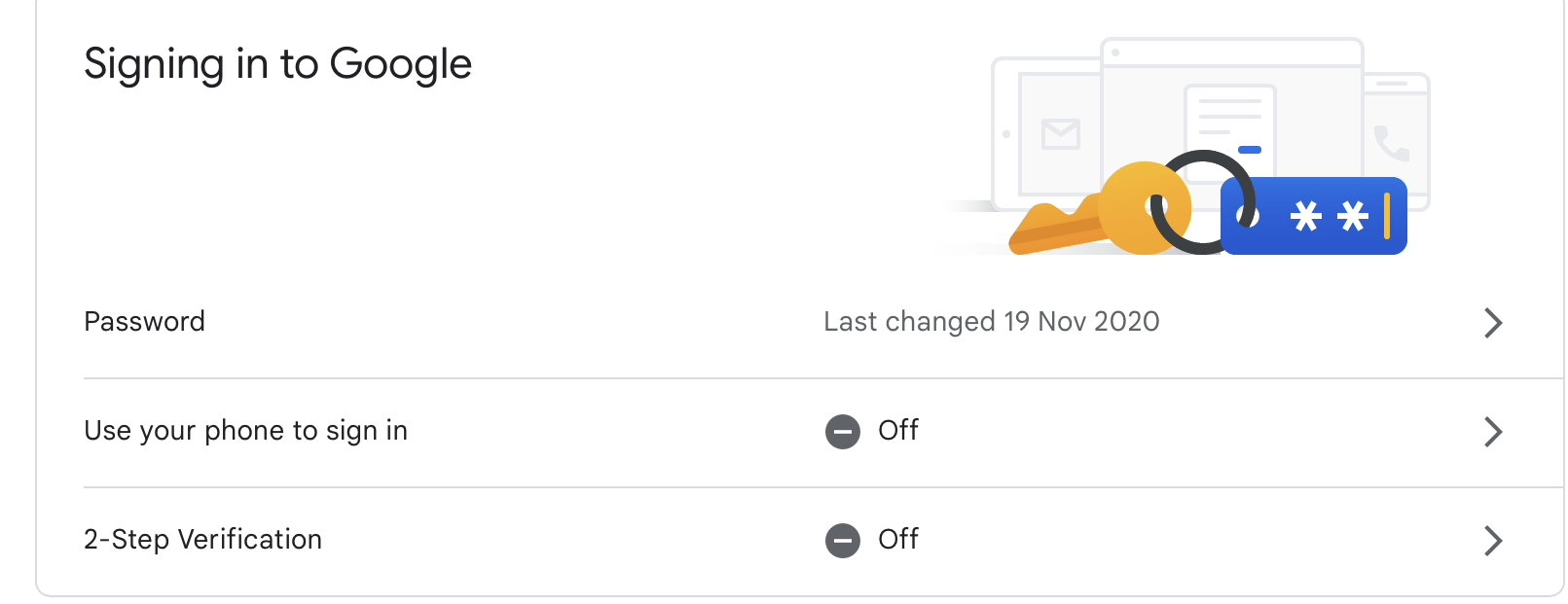
- Enable 2-Step Verification if it is disabled for your account.
- After enabling the 2-Step Verification, you will see an App passwords option for your account, as shown in the image below.
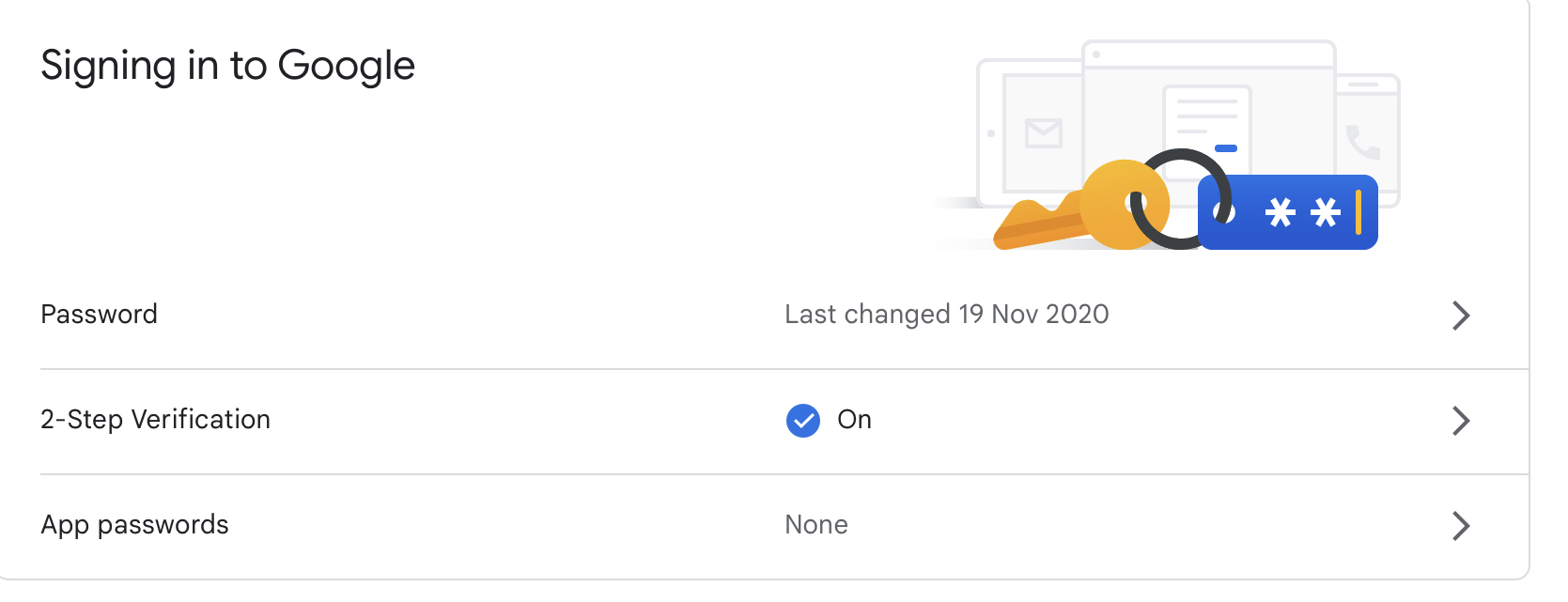
- Now, click on the App passwords and follow the steps to create an App password.

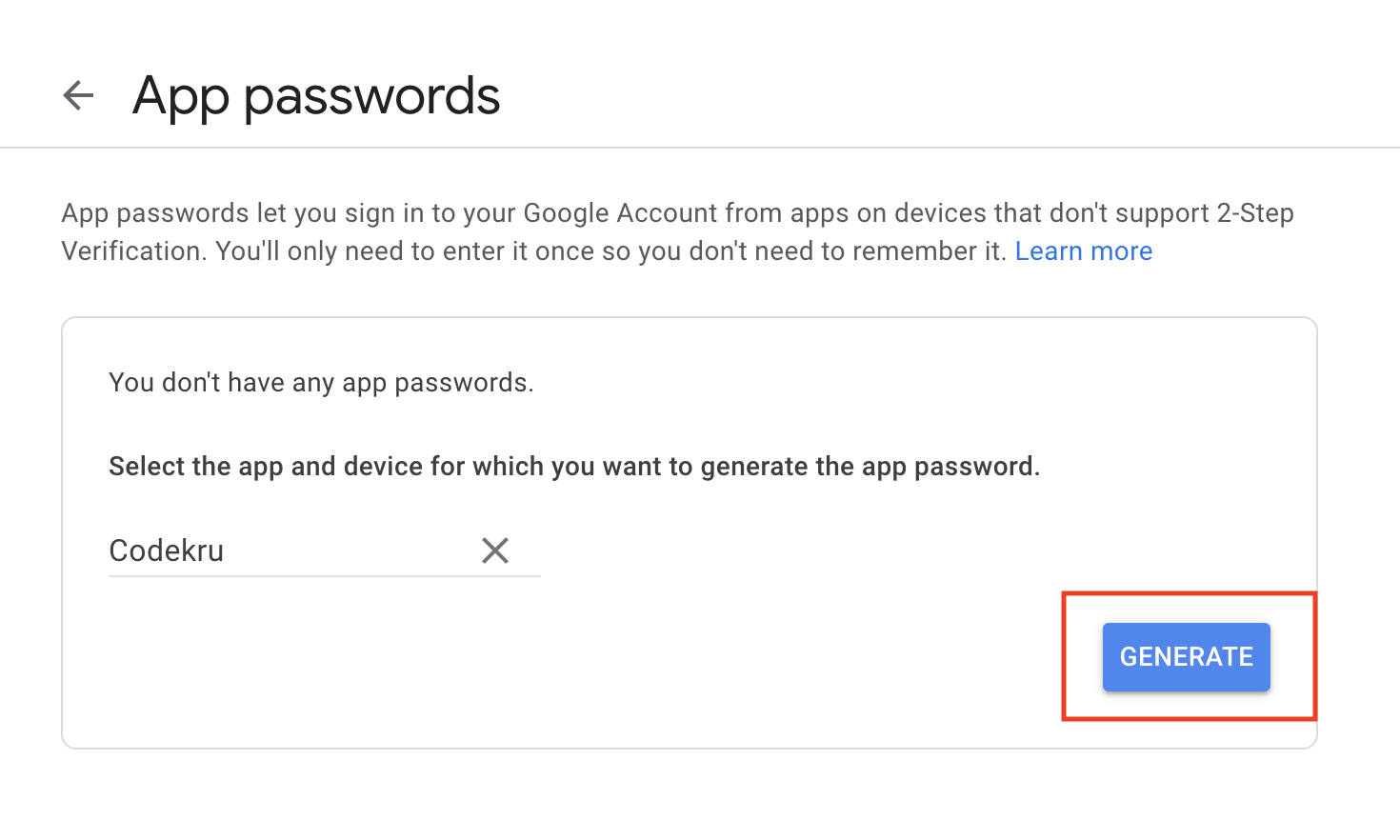
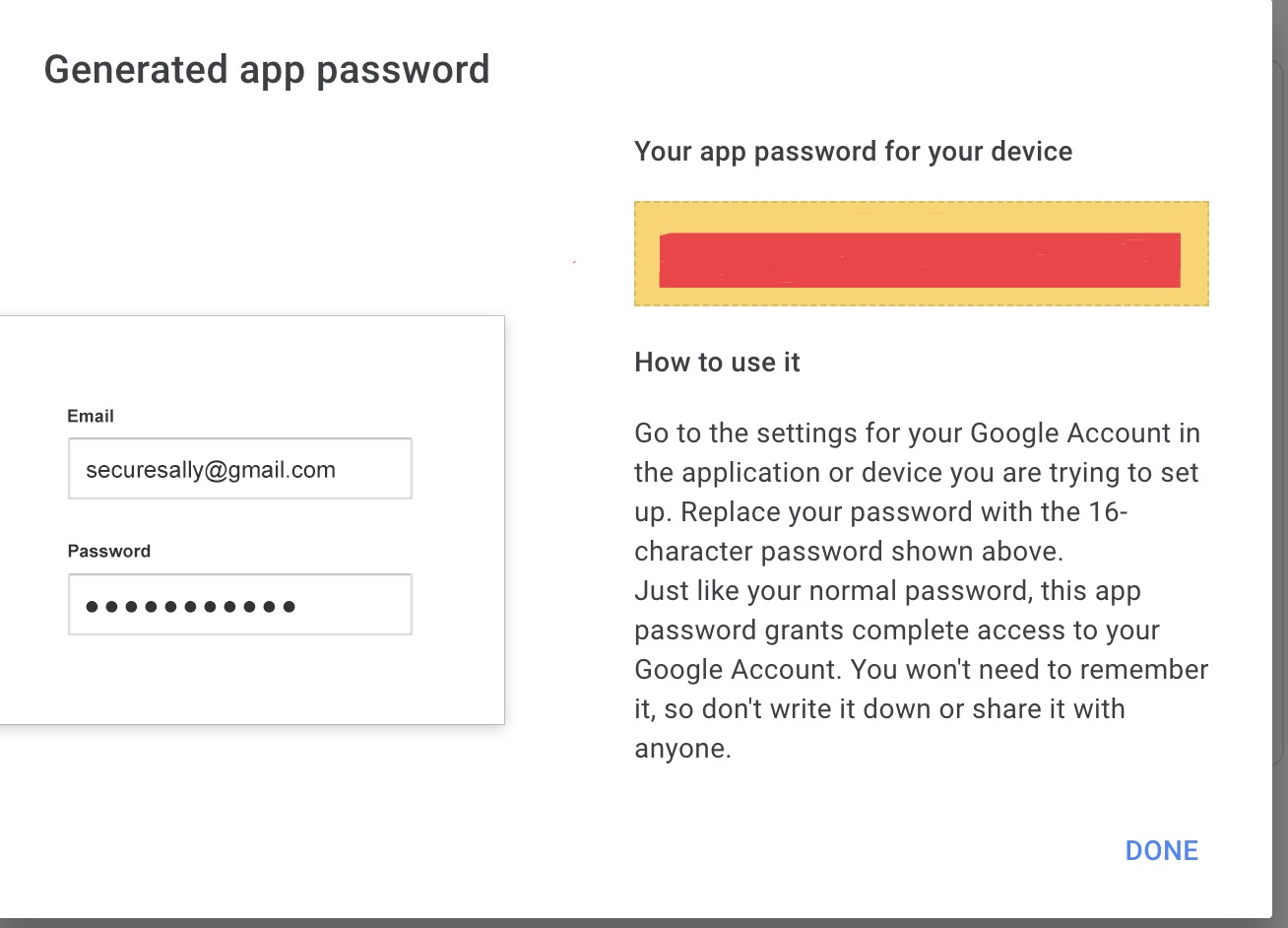
- Please copy the app password that has been generated. We will use it later in our post.
Send email using SMTP
Now, we will write the code to send emails using SMTP in java
Add dependencies
We will add the dependencies necessary to run our code. If you are not using Maven, add the jar for that dependency and use it in your code.
Add the below dependency in your pom.xml file
<dependency>
<groupId>com.sun.mail</groupId>
<artifactId>javax.mail</artifactId>
<version>1.6.2</version>
</dependency>
or you can download the jars and add them to your project.
Write the Java Code
- Setting the properties
Properties prop = new Properties();
prop.put("mail.smtp.auth", true);
prop.put("mail.smtp.host", "smtp.gmail.com");
prop.put("mail.smtp.starttls.enable", "true");
prop.put("mail.smtp.port", "587");
prop.put("mail.smtp.ssl.protocols", "TLSv1.2");
- Make Session and message objects
Session session = Session.getDefaultInstance(prop);
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress(fromEmailAddress));
message.addRecipients(Message.RecipientType.TO, InternetAddress.parse(toEmailAddress)); // setting "TO" email address
message.setSubject(subject); // setting subject
message.setText(bodyText); // setting body
- Final Code
We will use the app password we created in the previous step.
import java.util.Properties;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
public class Codekru {
public void sendEmail(String toEmailAddress, String fromEmailAddress, String appPassword, String subject,
String bodyText) {
Properties prop = new Properties();
prop.put("mail.smtp.auth", true);
prop.put("mail.smtp.host", "smtp.gmail.com");
prop.put("mail.smtp.starttls.enable", "true");
prop.put("mail.smtp.port", "587");
prop.put("mail.smtp.ssl.protocols", "TLSv1.2");
Session session = Session.getDefaultInstance(prop);
Message message = new MimeMessage(session);
try {
message.setFrom(new InternetAddress(fromEmailAddress));
message.addRecipients(Message.RecipientType.TO, InternetAddress.parse(toEmailAddress)); // setting "TO" email address
message.setSubject(subject); // setting subject
message.setText(bodyText); // setting body
System.out.println("Sending Email...");
Transport t = session.getTransport("smtp");
t.connect(fromEmailAddress, appPassword);
t.sendMessage(message, message.getAllRecipients());
t.close();
} catch (MessagingException e) {
e.printStackTrace();
}
System.out.println("Email sent successfully...");
}
public static void main(String[] args) {
Codekru codekru = new Codekru();
String toEmailAddress = "admin@codekru.com";
String fromEmailAddress = "testemailforcodekru@gmail.com";
String appPassword = "****"; // Enter your app password here
String subject = "Test Subject";
String body = "Test Body";
codekru.sendEmail(toEmailAddress, fromEmailAddress, appPassword, subject, body);
}
}
Output –
Sending Email...
Email sent successfully...
The above code will send the email using SMTP.
This is it. We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.