valueOf() is a static method of the Long wrapper class in Java. This post will discuss the valueOf() method in detail.
Long class has provided three overloaded implementations of the valueOf() method –
- public static Long valueOf(long l)
- public static Long valueOf(String s)
- public static Long valueOf(String s, int radix)
Let’s look at each of them one by one.
public static Long valueOf(long l)
- What does it do? It will take a long primitive value as an argument and create an instance of the Long class representing that value.
- What does it return? It will return a Long instance representing the value passed in the argument.
Note: valueOf() is the preferred way of creating the objects of the Long class because it provides better space and time performance over the normal constructors.
Code Example
public class Codekru {
public static void main(String[] args) {
long longValue = 134242342;
Long longInstance = Long.valueOf(longValue);
System.out.println("Long instance value: " + longInstance);
}
}
Output –
Long instance value: 134242342
Time complexity of Long valueOf(long l) method
The time complexity of the valueOf(long l) method is O(1).
public static Long valueOf(String s)
It will take a string as an argument and convert it into a Long instance.
Note: We can only pass a string containing decimal characters with an optional positive or negative character at the beginning. If we pass any other character, it will throw a NumberFormatException.
Code Example
public class Codekru {
public static void main(String[] args) {
String str1 = "1234";
String str2 = "+1234";
String str3 = "-1234";
Long longInstance1 = Long.valueOf(str1);
Long longInstance2 = Long.valueOf(str2);
Long longInstance3 = Long.valueOf(str3);
System.out.println("Long instance representing str1: " + longInstance1);
System.out.println("Long instance representing str2: " + longInstance2);
System.out.println("Long instance representing str3: " + longInstance3);
}
}
Output –
Long instance representing str1: 1234
Long instance representing str2: 1234
Long instance representing str3: -1234
What if we pass other characters and not just decimal characters?
valueOf() method will throw a NumberFormatException as illustrated below.
public class Codekru {
public static void main(String[] args) {
String str = "twenty";
Long longInstance = Long.valueOf(str);
System.out.println("Long instance representing str1: " + longInstance);
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: For input string: "twenty"
at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:68)
at java.base/java.lang.Long.parseLong(Long.java:707)
at java.base/java.lang.Long.valueOf(Long.java:1159)
What if we pass null as an argument?
It will also throw a NumberFormatException
public class Codekru {
public static void main(String[] args) {
Long longInstance = Long.valueOf(null);
System.out.println("Long instance value: " + longInstance);
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: null
at java.base/java.lang.Long.parseLong(Long.java:670)
at java.base/java.lang.Long.valueOf(Long.java:1159)
Internal implementation of the valueOf(String s) method
public static Long valueOf(String s) throws NumberFormatException{
return Long.valueOf(parseLong(s, 10));
}
valueOf(String s) internally uses –
- parseLong() method to first parse the string.
- and then uses the valueOf(long l) method to create the Long instance.
Time complexity of the valueOf(String s) method
parseLong() method has a time complexity of O(n) and valueOf(long l) has O(1) time complexity. So, the time complexity of valueOf(String s) is O(n), where n is the length of the string.
public static Long valueOf(String s, int radix)
It will also convert a string into a Long instance but according to the radix passed as the second argument.
What role will radix play while parsing the string?
This is similar to how we convert a binary number to a decimal number using base 2.
Let’s show you one sample conversion of number 220 using radix 4.
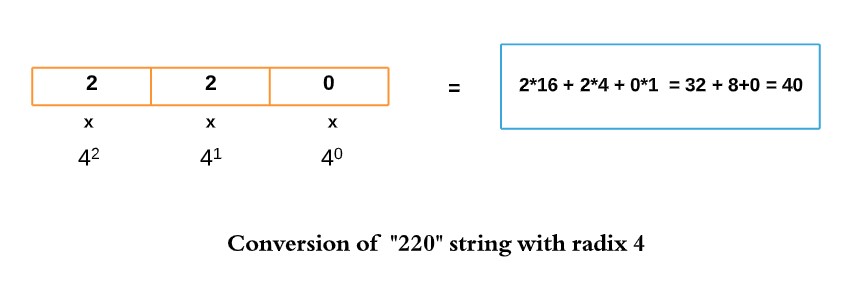
So, Long.valueOf(“220”,4) will return a Long instance with value 40.
public class Codekru {
public static void main(String[] args) {
Long longInstance = Long.valueOf("220", 4);
System.out.println("Long instance value: " + longInstance);
}
}
Output –
Long instance representing str1: 40
Some important points
- We can also use other than decimal characters, provided that the radix supports it. Eg. We can pass A, B, C, D, E and F with radix 16.
public class Codekru {
public static void main(String[] args) {
Long longInstance = Long.valueOf("220F", 16);
System.out.println("Long instance value: " + longInstance);
}
}
Output –
Long instance value: 8719
- The radix can only be between 2 and 36 ( inclusive ). If it is less than 2 or greater than 36, it will throw a NumberFormatException.
public class Codekru {
public static void main(String[] args) {
Long longInstance = Long.valueOf("220F", 37);
System.out.println("Long instance value: " + longInstance);
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: radix 37 greater than Character.MAX_RADIX
at java.base/java.lang.Long.parseLong(Long.java:678)
at java.base/java.lang.Long.valueOf(Long.java:1132)
Please visit this link to learn more about the Long wrapper class of java and its other functions or methods.
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.