In this post, we are going to look at the parseUnsignedInt() method in detail. The parseUnsignedInt() is a static method of the Integer wrapper class that helps in parsing the string as an unsigned integer.
Now, what are unsigned and signed integers? Signed integers contain both positive and negative numbers and their range is from [-2147483648 to 2147483647] whereas unsigned integers contain only non-negative numbers ranging from [0 to 4294967295].
parseUnsignedInt() method has three overloaded methods –
- public static int parseUnsignedInt(String s, int radix)
- public static int parseUnsignedInt(String s)
- public static int parseUnsignedInt(CharSequence s, int beginIndex, int endIndex, int radix)
Let’s look at all of the overloaded methods one by one.
public static int parseUnsignedInt(String s, int radix)
- What does it do? It will help in parsing the string as an unsigned integer using the radix passed in the arguments. The radix must be between 2 and 36 ( both inclusive) and the string passed in the argument should not have the negative sign (–) at the start of the string as unsigned integers cannot be negative but can have the positive sign (+).
Here, we can also pass non-decimal characters within the string provided that the radix passed along with the string also supports those characters. Like radix 16 supports the use of five characters ( A, B, C, D, and E )
String s1 = "1234" // this is correct
String s3 = "+1234" // this is correct too
String s2 = "-1234" // this is wrong
String s4 = "twenty" // this is also wrong
- What does it return? It will return an integer after parsing the string as an unsigned integer with the given radix
Code Example
public class Codekru {
public static void main(String[] args) {
String s1 = "1234";
String s2 = "+1234";
String s3 = "123";
// with radix 10
System.out.println("Integer representing string s1: " + Integer.parseUnsignedInt(s1, 10));
System.out.println("Integer representing string s2: " + Integer.parseUnsignedInt(s2, 10));
// with radix 16
System.out.println("Integer representing string s3: " + Integer.parseUnsignedInt(s3, 16));
}
}
Output –
Integer representing string s1: 1234
Integer representing string s2: 1234
Integer representing string s3: 291
The “123” string is converted using the radix 16 and the result was 291. The below representation will help in further clarification of the same.
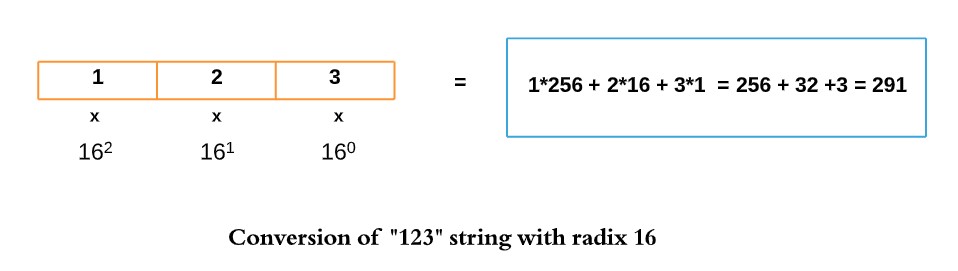
public static int parseUnsignedInt(String s)
- What does it do? It also parses the unsigned string into an integer by using the radix 10 as the default radix. It only accepts the decimal characters within the string along with the optional positive sign ( + ) at the start of the string.
- What does it return? It returns an int primitive after parsing the string with radix 10.
Code Example
public class Codekru {
public static void main(String[] args) {
String s1 = "1234";
String s2 = "+1234";
System.out.println("Integer representing string s1: " + Integer.parseUnsignedInt(s1));
System.out.println("Integer representing string s2: " + Integer.parseUnsignedInt(s2));
}
}
Output –
Integer representing string s1: 1234
Integer representing string s2: 1234
Internal Implementation of the parseUnsignedInt(String s) method
parseUnsignedInt(String s) internally uses the parseUnsignedInt(String s, int radix) with radix = 10. So, the time complexity of the parseUnsignedInt(String s) would be equal to that of the parseUnsignedInt(String s, int radix) method.
public static int parseUnsignedInt(String s) throws NumberFormatException {
return parseUnsignedInt(s, 10);
}
public static int parseUnsignedInt(CharSequence s, int beginIndex, int endIndex, int radix)
- What does it do? This one takes a CharSequence, a beginIndex (inclusive), and endIndex (exclusive), and finally a radix. So, the CharSequence will be parsed from beginIndex to endIndex-1 with the use of the given radix.
- What does it return? It will return an int primitive after parsing the CharSequence.
Now, what is CharSequence? CharSequence is an interface in Java that represents a sequence of characters. This interface provides uniform, read-only access to many different kinds of char sequences. Below is the list of classes that implement this interface –
- CharBuffer
- Segment
- String
- StringBuffer
- StringBuilder
So, we can pass other sequences of characters as well and not just the string.
When do we need to use the parseUnsignedInt(CharSequence s, int beginIndex, int endIndex, int radix) method over other overloaded methods?
- When we have to pass StringBuffer, StringBuilder, or some other characters sequences, then we can use the parseUnsignedInt(CharSequence s, int beginIndex, int endIndex, int radix) method. It can accept CharSequence in its arguments which make it more useful in places where we are dealing with the various sequence of characters and not just the string.
- It also takes a beginIndex and an endIndex, which makes it easier to use in cases where we have to parse only a part of the string.
Code Example
public class Codekru {
public static void main(String[] args) {
String s1 = "1234";
StringBuilder s2 = new StringBuilder("+1234"); // Making a StringBuilder object
System.out.println("s1 with beginIndex = 0 and endIndex = 4: " + Integer.parseUnsignedInt(s1, 0, 4, 10));
System.out.println("s1 with beginIndex = 0 and endIndex = 3: " + Integer.parseUnsignedInt(s1, 0, 3, 10));
System.out.println("s2 with beginIndex = 0 and endIndex = 5: " + Integer.parseUnsignedInt(s2, 0, 5, 10));
}
}
Output –
s1 with beginIndex = 0 and endIndex = 4: 1234
s1 with beginIndex = 0 and endIndex = 3: 123
s2 with beginIndex = 0 and endIndex = 5: 1234
parseInt() vs parseUnsignedInt()
- parseInt() takes a normal string into its argument that can optionally have the positive or negative sign at the start of the string ( “+1234” or “-12345”) while parseUnsignedInt() can only have the unsigned string passed into its arguments. So, the string can only have the positive sign (“+”) at the front of the string and not the negative one (“-“)
- parseInt() can take the decimal strings ranging from [-2147483648 to 2147483647] into its arguments whereas the parseUnsignedInt() can have the decimal string ranging from [0 to 4294967295]
The WhatIf scenarios
Q- What if passed a negative decimal string within the function’s argument?
A negative decimal string is not an unsigned string. So, the method will throw a NumberFormatException.
public class Codekru {
public static void main(String[] args) {
String s1 = "-1234";
System.out.println(Integer.parseUnsignedInt(s1));
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: Illegal leading minus sign on unsigned string -1234.
at java.base/java.lang.Integer.parseUnsignedInt(Integer.java:827)
at java.base/java.lang.Integer.parseUnsignedInt(Integer.java:928)
Q- What if we passed a string greater than “4294967295” in the function’s argument?
As we know that the range of the unsigned integers is from [0 to 4294967295]. So, let’s see what will happen if we are passing “4294967296” as an unsigned string in the function’s argument.
public class Codekru {
public static void main(String[] args) {
String s1 = "4294967296";
System.out.println(Integer.parseUnsignedInt(s1));
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: String value 4294967296 exceeds range of unsigned int.
at java.base/java.lang.Integer.parseUnsignedInt(Integer.java:839)
at java.base/java.lang.Integer.parseUnsignedInt(Integer.java:928)
Here, we again got a NumberFormatException with a message explaining that “4294967296” exceeds the range of unsigned int.
Q- What if we pass “0” as a string in the arguments?
Here, everything will work fine as illustrated by the below program.
public class Codekru {
public static void main(String[] args) {
String s1 = "0";
System.out.println(Integer.parseUnsignedInt(s1));
}
}
Output –
0
Q- What if we pass a null string in the arguments?
public class Codekru {
public static void main(String[] args) {
String s1 = null;
System.out.println(Integer.parseUnsignedInt(s1));
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: null
at java.base/java.lang.Integer.parseUnsignedInt(Integer.java:819)
at java.base/java.lang.Integer.parseUnsignedInt(Integer.java:928)
Q- What if we pass radix > 36 in the arguments?
We will again get a NumberFormatException telling that the radix is greater than Character.MAX_RADIX ( 36 ).
public class Codekru {
public static void main(String[] args) {
String s1 = "1234";
// passing 37 as the radix
System.out.println(Integer.parseUnsignedInt(s1, 37));
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: radix 37 greater than Character.MAX_RADIX
at java.base/java.lang.Integer.parseInt(Integer.java:623)
at java.base/java.lang.Integer.parseUnsignedInt(Integer.java:832)
Similarly, we will also get the exception when radix<2
Exception in thread "main" java.lang.NumberFormatException: radix 1 less than Character.MIN_RADIX
at java.base/java.lang.Integer.parseInt(Integer.java:618)
at java.base/java.lang.Integer.parseUnsignedInt(Integer.java:832)
Please visit this link if you want to know more about the Integer wrapper class of java and its other functions or methods.
Hope you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.