This post will discuss the parseInt() function of the Integer Wrapper class in detail. The parseInt() is a static method of the Integer class and can be called directly using the class name( Integer.parseInt() ) and has three overloaded methods which can be used as per the requirements.
- public static int parseInt(String s)
- public static int parseInt(String s, int radix)
- public static int parseInt(CharSequence s, int beginIndex, int endIndex, int radix)
Let’s look at all of the overloaded methods one by one.
public static int parseInt(String s)
- What does it do? The parseInt() method will parse the string passed in the arguments as a signed decimal integer. Here the string passed should only have the decimal characters, except that the first character of the string can be a minus sign (-) to represent a negative value or a positive sign (+) to indicate a positive value.
String s1 = "20"; // this is correct, as string only contains decimal values
String s2 = "-20"; // this is also correct, as minus sign
//can be put as the first character of the string
String s3 = "+20"; // this is also correct
String s3 = "twenty20" // this is wrong as string contains non-decimal characters
- What does parseInt(String s) return? After parsing the string passed in the arguments, it will return an integer value.
Code Example
public class Codekru {
public static void main(String[] args) {
String s1 = "20";
String s2 = "-20";
String s3 = "+20";
int i1 = Integer.parseInt(s1);
int i2 = Integer.parseInt(s2);
int i3 = Integer.parseInt(s3);
System.out.println("int value representing s1: " + i1);
System.out.println("int value representing s2: " + i2);
System.out.println("int value representing s3: " + i3);
}
}
Output –
int value representing s1: 20
int value representing s2: -20
int value representing s3: 20
There are some scenarios where parseInt() would throw an exception, which we will discuss later in this post.
Time Complexity of parseInt(String s)
The time complexity of parseInt(String s) is O(n) as it runs a while loop on the string to convert it into an integer.
public static int parseInt(String s, int radix)
- What does it do? This is like converting binary numbers into decimal numbers ( base 10 ) or any other base. It will also parse the string as a signed integer using the radix passed in the arguments.
Remember that the radix passed in the argument must be between 2 and 36 ( inclusive ). So, if we enter radix argument as 37, then parseInt() will throw a NumberFormatException.
parseInt("20",4) -> 8
parseInt("20",8) -> 16
parseInt("220",4) -> 40
Below is the sample representation of converting a number with any radix. We have used radix as 4 in our example.
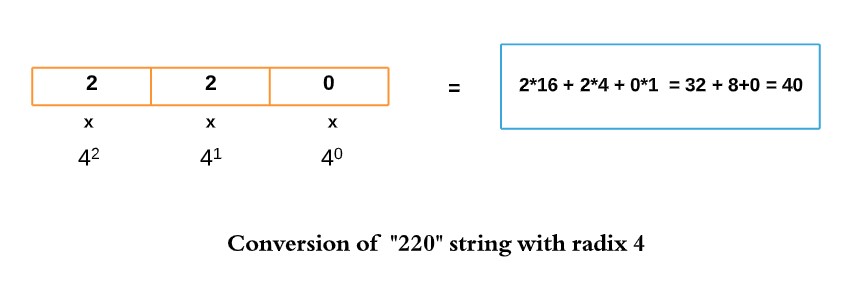
- What does parseInt(String s, int radix) return? It will also return an integer.
Code Example
public class Codekru {
public static void main(String[] args) {
String s1 = "20";
String s2 = "20";
String s3 = "220";
String s4 = "-20";
int i1 = Integer.parseInt(s1, 4);
int i2 = Integer.parseInt(s2, 8);
int i3 = Integer.parseInt(s3, 4);
int i4 = Integer.parseInt(s4, 8);
System.out.println("int value representing s1 with radix 4: " + i1);
System.out.println("int value representing s2 with radix 8: " + i2);
System.out.println("int value representing s3 with radix 4: " + i3);
System.out.println("int value representing s4 with radix 8: " + i4);
}
}
Output –
int value representing s1 with radix 4: 8
int value representing s2 with radix 8: 16
int value representing s3 with radix 4: 40
int value representing s4 with radix 8: -16
Time Complexity of parseInt(String s, int radix)
The time complexity of parseInt(String s, int radix) is also O(n).
Some Interesting points
- parseInt(String s) internally uses the parseInt(String s, int radix) with radix as 10. Below is the internal implementation of the parseInt() method –
public static int parseInt(String s) throws NumberFormatException {
return parseInt(s,10);
}
- We mentioned earlier that the parseInt(String s) method only allows the decimal characters and a positive or a minus sign at the front. This is because parseInt() internally uses the radix 10, which doesn’t allow using other characters. Still, with parseInt(String s, int radix), we can use different characters, provided that the radix passed in the argument supports it.
Like, radix 16 supports using five more characters ( A, B, C, D, and E) other than the decimal ones. So, the below program will work fine.
public class Codekru {
public static void main(String[] args) {
String s1 = "A20";
System.out.println("int value representing s1 with radix 16: " + Integer.parseInt(s1,16));
}
}
int value representing s1 with radix 16: 2592
public static int parseInt(CharSequence s, int beginIndex, int endIndex, int radix)
This method was introduced in Java 9.
- What does it do? While the other parseInt() method took a string argument, this one will take a CharSequence reference, a beginIndex ( inclusive ), and an endIndex ( exclusive ) along with the radix to parse that CharSequence reference as a signed Integer. The CharSequence is parsed from beginIndex to endIndex -1
- What does it return? It also returns an integer.
Now, what is CharSequence? CharSequence is an interface in Java that represents a sequence of characters. This interface provides uniform, read-only access to various char sequences. Below is the list of classes that implement this interface –
- CharBuffer
- Segment
- String
- StringBuffer
- StringBuilder
So, we can pass other sequences of characters and not just the string in the arguments of this parseInt() method.
When can we use public static int parseInt(CharSequence s, int beginIndex, int endIndex, int radix) method?
- This parseInt() method can be helpful in scenarios where we use StringBuffer or StringBuilder instead of String.
- We can also pass a beginIndex and an endIndex in the method’s argument to only parse a part of the string. This would save us in writing the code for creating the substring ourselves.
Code Example
public class Codekru {
public static void main(String[] args) {
String s1 = "200";
StringBuilder s2 = new StringBuilder("200");
StringBuffer s3 = new StringBuffer("200");
System.out.println("int value representing "
+ "s1 with radix 10: " + Integer.parseInt(s1, 0, s1.length(), 10));
System.out.println("int value representing "
+ "s2 with radix 10: " + Integer.parseInt(s2, 0, s2.length(), 10));
System.out.println("int value representing "
+ "s3 with radix 10: " + Integer.parseInt(s3, 0, s3.length(), 10));
}
}
Output –
int value representing s1 with radix 10: 200
int value representing s2 with radix 10: 200
int value representing s3 with radix 10: 200
Time Complexity of parseInt(CharSequence s, int beginIndex, int endIndex, int radix)
The time complexity of parseInt(CharSequence s, int beginIndex, int endIndex, int radix) is also O(k) where k = endIndex-beginIndex.
The What If scenarios
Q – What if we pass other than decimal characters in parseInt(String s)?
Then parseInt() will throw NumberFormatException as illustrated by the below program.
public class Codekru {
public static void main(String[] args) {
String s1 = "20abc";
System.out.println("int value representing s1 :" + Integer.parseInt(s1));
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: For input string: "20abc"
at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:68)
at java.base/java.lang.Integer.parseInt(Integer.java:652)
at java.base/java.lang.Integer.parseInt(Integer.java:770)
Q – What if we pass a null string in parseInt(String s)?
public class Codekru {
public static void main(String[] args) {
String s1 = null;
System.out.println("int value representing s1 :" + Integer.parseInt(s1));
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: null
at java.base/java.lang.Integer.parseInt(Integer.java:614)
at java.base/java.lang.Integer.parseInt(Integer.java:770)
Q – What if we pass an empty string in parseInt(String s)?
public class Codekru {
public static void main(String[] args) {
String s1 = "";
System.out.println("int value representing s1 :" + Integer.parseInt(s1));
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: For input string: ""
at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:68)
at java.base/java.lang.Integer.parseInt(Integer.java:662)
at java.base/java.lang.Integer.parseInt(Integer.java:770)
Q – What if we pass radix > 36 in parseInt(String s, int radix)?
parseInt() will again throw a NumberFormatException.
public class Codekru {
public static void main(String[] args) {
String s1 = "1234";
System.out.println("int value representing s1 with radix 37: " + Integer.parseInt(s1, 37));
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: radix 37 greater than Character.MAX_RADIX
at java.base/java.lang.Integer.parseInt(Integer.java:623)
and similarly, we will get the NumberFormatException for the radix value of less than 2.
Exception in thread "main" java.lang.NumberFormatException: radix 1 less than Character.MIN_RADIX
at java.base/java.lang.Integer.parseInt(Integer.java:618)
Please visit this link to learn more about the Integer wrapper class of java and its other functions or methods.
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.