This post will discuss the parseLong() method of the Long wrapper class in detail. parseLong() is a static method, and the Long class has provided three overloaded implementations, and we can use any of those based on the requirements.
- public static long parseLong(String s)
- public static long parseLong(String s, int radix)
- public static long parseLong(CharSequence s, int beginIndex, int endIndex, int radix)
Let’s look at all of the requirements one by one.
public static long parseLong(String s)
- What does it do? It will take a string as an argument and convert it into a long primitive type. So, we can say that it will convert a string into a long value.
- What does it return? It will return a long primitive value representing the string value passed in the argument.
String s = "1234" // we can use it with parseLong(String s) method
String s = "+1234" // This will work as well
String s = "-1234" // This is correct too
String s = "twenty" // This will throw a "NumberFormatException"
Code Example
public class Codekru {
public static void main(String[] args) {
String s1 = "1234";
String s2 = "-1234";
String s3 = "+1234";
long val1 = Long.parseLong(s1);
long val2 = Long.parseLong(s2);
long val3 = Long.parseLong(s3);
System.out.println("long value representing s1: " + val1);
System.out.println("long value representing s2: " + val2);
System.out.println("long value representing s3: " + val3);
}
}
Output –
long value representing s1: 1234
long value representing s2: -1234
long value representing s3: 1234
When will parseLong(String s) throw a NumberFormatException?
We can only pass decimal characters with an optional positive or negative sign at the start of the string. If we pass any other character within the string, it will throw a NumberFormatException.
String s = "1234" // we can use it with parseLong(String s) method
String s = "+1234" // This will work as well
String s = "-1234" // This is correct too
String s = "twenty" // This will throw a "NumberFormatException"
public class Codekru {
public static void main(String[] args) {
String str = "1234A";
long val = Long.parseLong(str);
System.out.println("long value representing str: " + val);
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: For input string: "1234A"
at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:68)
at java.base/java.lang.Long.parseLong(Long.java:707)
at java.base/java.lang.Long.parseLong(Long.java:832)
Internal implementation of the Long parseLong(String s) method
public static long parseLong(String s) throws NumberFormatException {
return parseLong(s, 10);
}
We can see that parseLong(String s) internally uses the parseLong(String s, int radix) with radix as 10.
Time complexity of the Long parseLong(String s) method
The time complexity of parseLong(String s) is O(n), where n is the length of the string.
public static long parseLong(String s, int radix)
- What does it do? It parses the string to a long primitive value based on the radix passed as the second argument.
- What does it return? It returns a long primitive value represented by the string in the specified radix.
What role will radix play while parsing the string?
This is similar to how we convert a binary number to a decimal number using base 2.
Let’s show you one sample conversion of number 220 using radix 4.
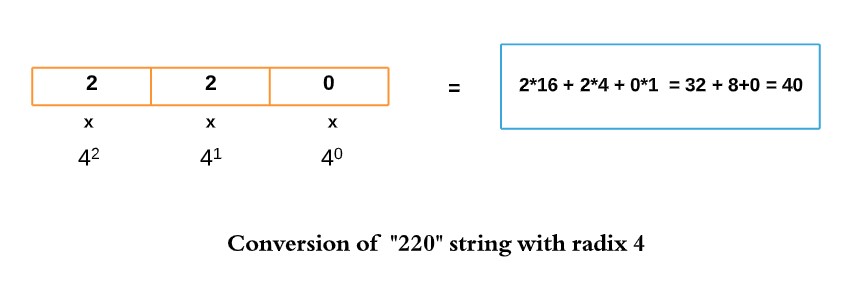
So, parseLong(“220”, 4) will also return 40.
Code Example
public class Codekru {
public static void main(String[] args) {
String s1 = "220";
String s2 = "-220";
String s3 = "+220";
long val1 = Long.parseLong(s1, 4);
long val2 = Long.parseLong(s2, 4);
long val3 = Long.parseLong(s3, 4);
System.out.println("long value representing s1: " + val1);
System.out.println("long value representing s2: " + val2);
System.out.println("long value representing s3: " + val3);
}
}
Output –
long value representing s1: 40
long value representing s2: -40
long value representing s3: 40
Some interesting points
- We can also use other than decimal characters, provided that the radix supports it. Eg. We can pass A, B, C, D, E and F with radix 16.
public class Codekru {
public static void main(String[] args) {
String s1 = "A20";
System.out.println("long value representing s1 with radix 16: " + Long.parseLong(s1,16));
}
}
Output –
long value representing s1 with radix 16: 2592
- The radix can only be between 2 and 36 ( inclusive ). If it is less than 2 or greater than 36, it will throw a NumberFormatException.
public class Codekru {
public static void main(String[] args) {
String s1 = "A20";
System.out.println("long value representing s1 with radix 17: " + Long.parseLong(s1,37));
}
}
Output –
Exception in thread "main" java.lang.NumberFormatException: radix 37 greater than Character.MAX_RADIX
at java.base/java.lang.Long.parseLong(Long.java:678)
public static long parseLong(CharSequence s, int beginIndex, int endIndex, int radix)
What does it do? It accepts a CharSequence instead of a string and converts it into a long primitive value based on beginIdex, endIndex and radix passed in the arguments.
We have talked about the radix. So, let’s talk about other arguments.
What is CharSequence?
CharSequence is an interface in Java that represents a sequence of characters. This interface provides uniform, read-only access to various char sequences. Below is the list of classes that implement this interface –
- CharBuffer
- Segment
- String
- StringBuffer
- StringBuilder
So, we can pass other sequences of characters and not just the string in the arguments of this parseLong() method.
What is the use of beginIndex and endIndex?
Till now, we were parsing the whole string, but now we can pass a beginIndex and an endIndex in the arguments. parseLong() method will parse the string starting from beginIndex till endIndex -1.
When can we use the public static int parseLong(CharSequence s, int beginIndex, int endIndex, int radix) method?
- This can be helpful in scenarios where we use StringBuffer or StringBuilder instead of String.
- We can also pass a beginIndex and an endIndex in the method’s argument to only parse a part of the string. This would save us from writing the code for creating the substring ourselves.
Code Example
public class Codekru {
public static void main(String[] args) {
String s1 = "200";
StringBuilder s2 = new StringBuilder("200");
StringBuffer s3 = new StringBuffer("200");
System.out.println("long value representing " + "s1 with radix 10: " + Long.parseLong(s1, 0, s1.length(), 10));
System.out.println("long value representing " + "s2 with radix 10: " + Long.parseLong(s2, 0, s2.length(), 10));
System.out.println("long value representing " + "s3 with radix 10: " + Long.parseLong(s3, 0, s3.length(), 10));
}
}
Output –
long value representing s1 with radix 10: 200
long value representing s2 with radix 10: 200
long value representing s3 with radix 10: 200
Please visit this link to learn more about the Long wrapper class of java and its other functions or methods.
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.