The click() method in Playwright Java is a powerful tool for automating user interactions with web applications. With just a few lines of code, you can simulate a user clicking on a web page’s button, link, or other element. This article will discuss the click() method provided by the Playwright in detail using Java.
Several implementations of the click() method are offered by the Page, Locator, and ElementHandle. However, it is advisable to use the Locator’s click() method, which we will focus on in this discussion.
Locator provides two overloaded click() methods.
Let’s discuss them one by one.
default void click()
As the name suggests, this method is used to click on an element.
The click() method will perform a click operation only if the element passes certain actionability checks. If the element does not pass these checks within a specific time ( By default, 30 seconds ), then the click() method will throw a TimeoutError exception.
What actionability checks need to be passed?
- Attached – Element is considered attached when connected to a Document or a ShadowRoot.
- Visible – Element should be visible on the webpage and not hidden by any CSS property.
- Stable – Element is considered stable when it has maintained the same bounding box for at least two consecutive animation frames.
- Receives events
- Enabled – Element is considered enabled unless it is a
<button>
,<select>
,<input>
or<textarea>
with adisabled
property.
We will now click on the below-highlighted element that satisfies all the actionability checks. You can find this element on our playground website – https://testkru.com/Elements/Buttons.

Inspecting the element will reveal its DOM.
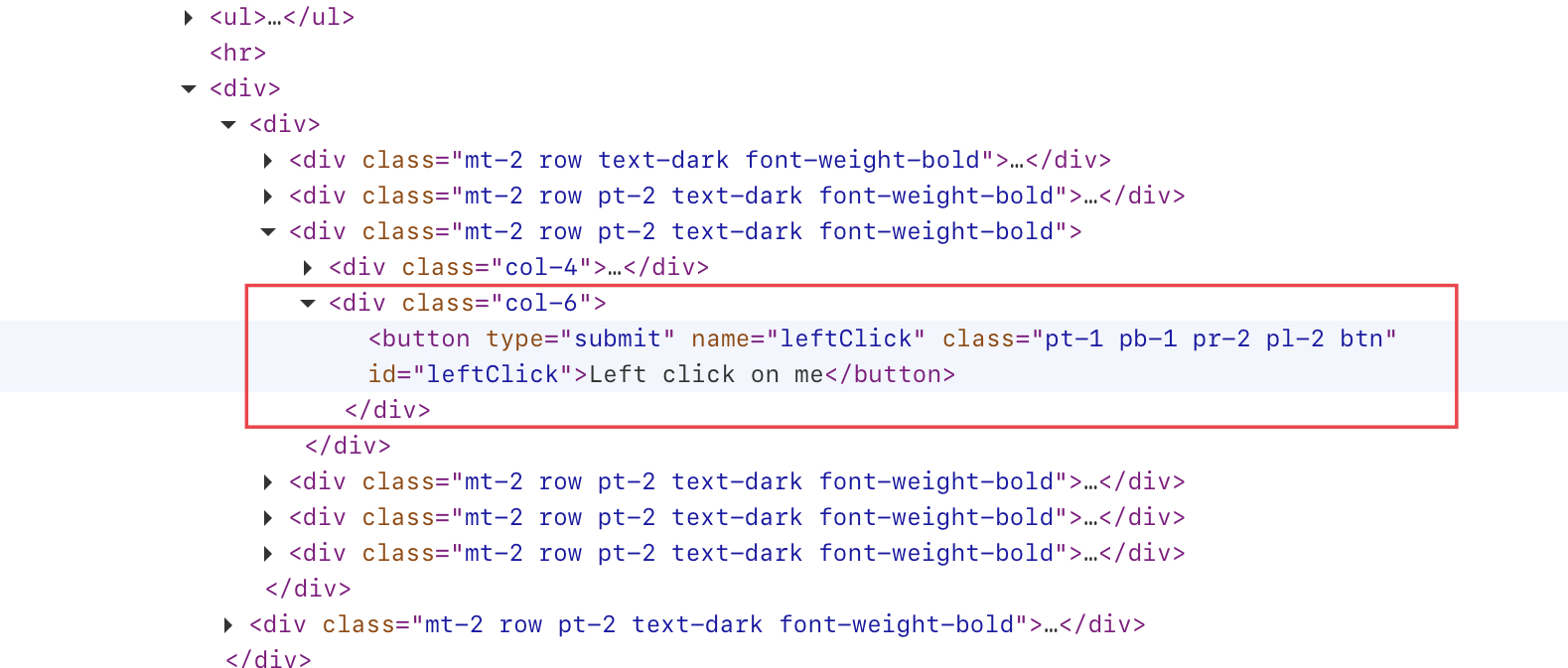
The “id” attribute value of our element is “leftClick“. We can use it to locate our element using the locator() method provided by the Playwright.
Locator buttonLocator = page.locator("#leftClick");
After locating the element, we can execute the click() method to trigger a click on it.
buttonLocator.click();
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
Locator buttonLocator = page.locator("#leftClick");
// clicking on the element
buttonLocator.click();
// closing the instances
browser.close();
playwright.close();
}
}
Above code will click on the element that is highlighted in the image.
What if we try to click on a disabled button?
When an element is disabled, it fails to meet the criteria for actionability, as the standard requires the element to be enabled.
Let’s see what happens when we click on the highlighted element below. You can find the element at https://testkru.com/Elements/Buttons.
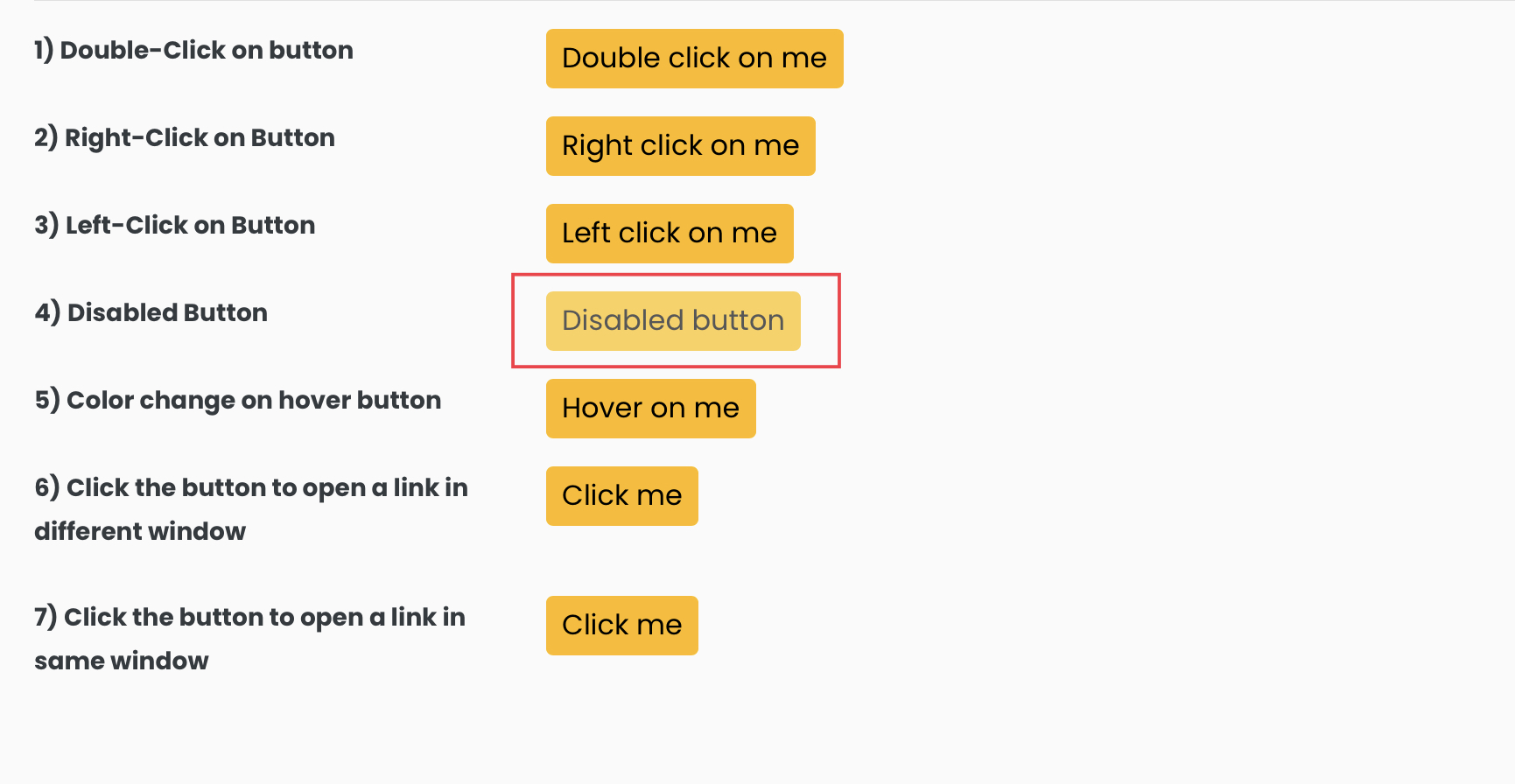
We can examine the element’s DOM to identify a means of locating it.
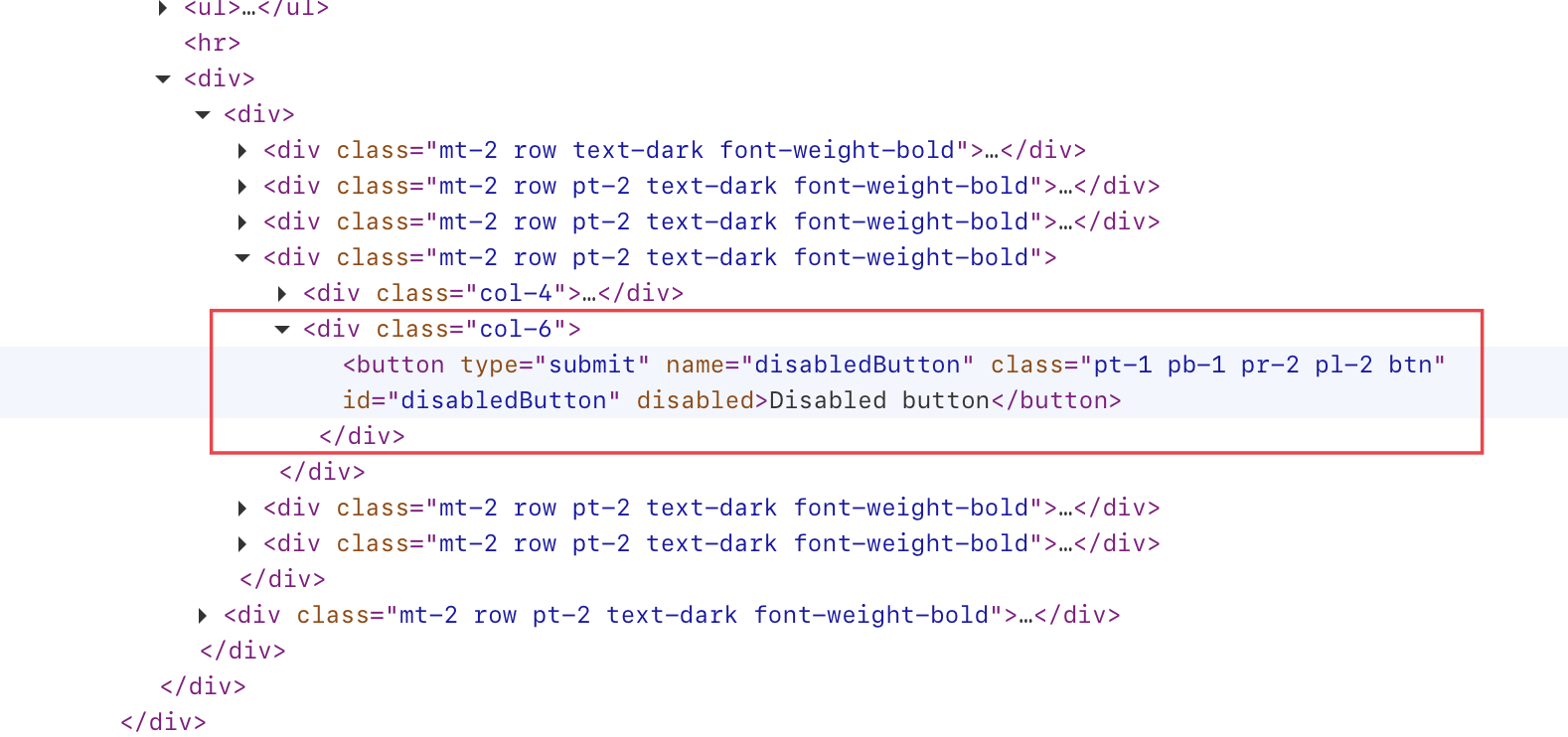
We can locate our element using the value “disabledButton” of the “id” attribute.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
Locator buttonLocator = page.locator("#disabledButton");
// clicking on the element
buttonLocator.click();
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Exception in thread "main" com.microsoft.playwright.TimeoutError: Error {
message='Timeout 30000ms exceeded.
=========================== logs ===========================
waiting for locator("#disabledButton")
============================================================
name='TimeoutError
stack='TimeoutError: Timeout 30000ms exceeded.
=========================== logs ===========================
waiting for locator("#disabledButton")
So, the click() method threw a TimeoutError exception because the element remained disabled even after waiting for 30 seconds.
Internal implementation of the click() method
default void click() {
click(null);
}
click() method internally uses the click(ClickOptions options) method. So, let’s start discussing it.
void click(ClickOptions options)
It accepts the ClickOptions class object in the argument and clicks on the element.
The ClickOptions class allows us to modify how the click() method behaves. E.g. By default, the click() method will wait 30 seconds before throwing a TimeoutError exception. However, we can adjust or turn off this timeout period using ClickOptions.
What can be modified using ClickOptions while performing the click operation?
Left-click operation
As you may have observed, the click() method currently performs a left-click operation. However, it can be modified to perform a right-click or middle-click operation instead.
Below code helps us perform a right-click operation on an element.
// performing right click
ClickOptions clickOptions = new ClickOptions();
clickOptions.setButton(MouseButton.RIGHT);
// clicking on the element
buttonLocator.click(clickOptions);
Let’s try this on the below element.

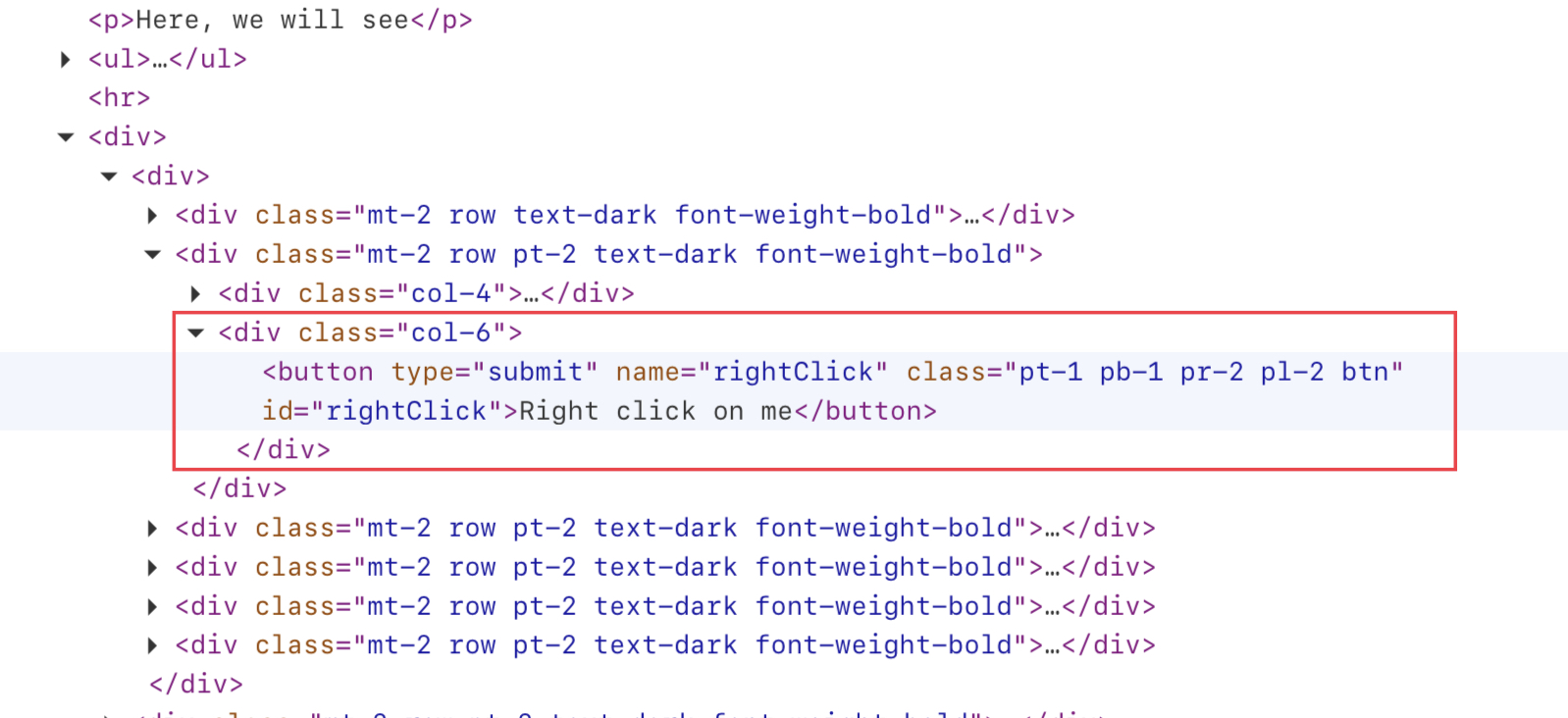
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Locator.ClickOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
import com.microsoft.playwright.options.MouseButton;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
Locator buttonLocator = page.locator("#rightClick");
// performing right click
ClickOptions clickOptions = new ClickOptions();
clickOptions.setButton(MouseButton.RIGHT);
// clicking on the element
buttonLocator.click(clickOptions);
// closing the instances
browser.close();
playwright.close();
}
}
The above code will perform a right-click operation on our element. Likewise, we can also perform a middle-click operation.
Click count
Playwright’s click() method will only click on a button once but can be modified to click multiple times as needed.
The ClickOptions class includes a method called setClickCount(), which allows us to specify the number of clicks for a button. For instance, if we need to double-click on a particular element, we can simply set the click count to 2.
// performing double click
ClickOptions clickOptions = new ClickOptions();
clickOptions.setClickCount(2);
// clicking on the element
buttonLocator.click(clickOptions);
We will double-click on the below element.
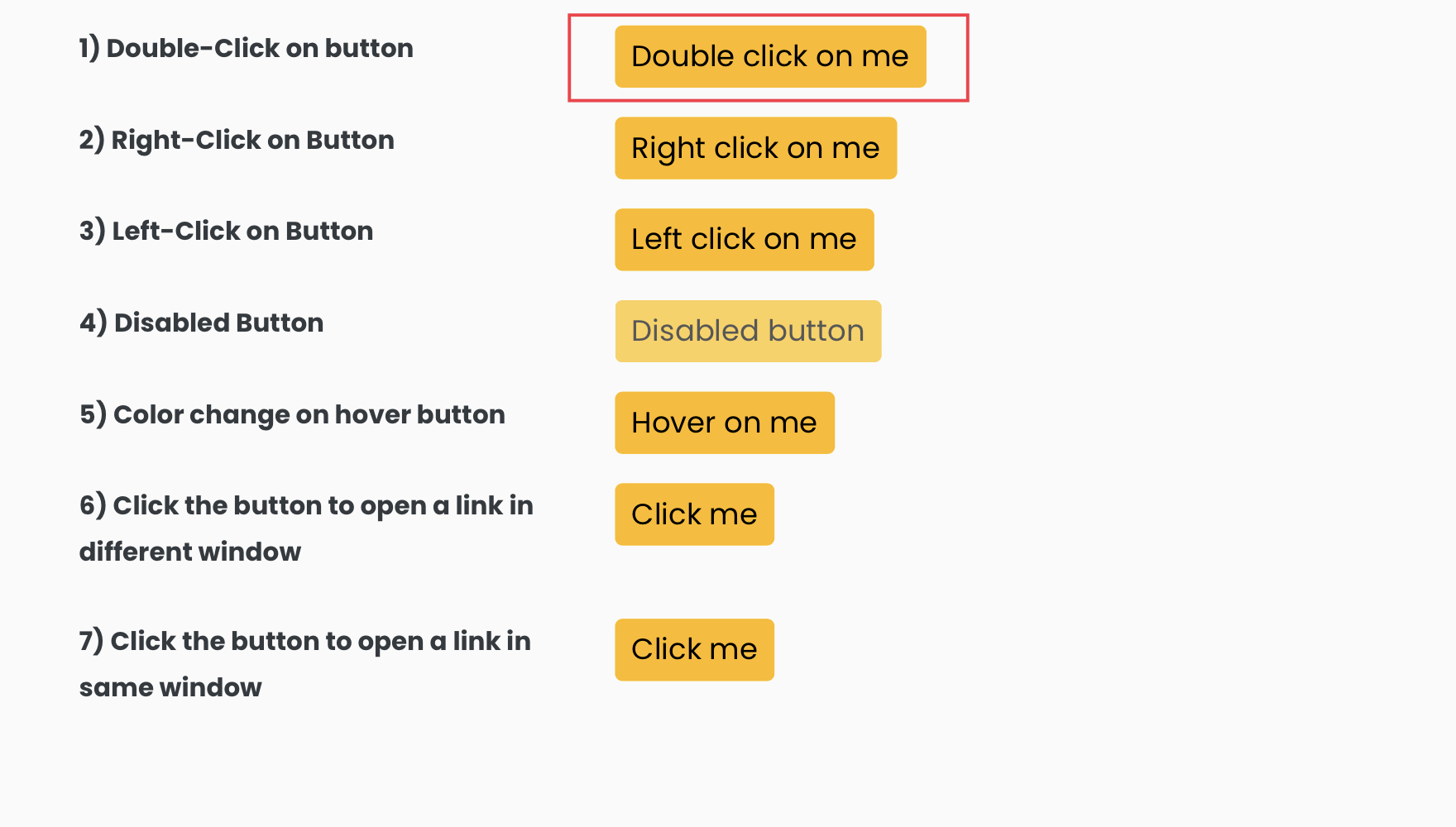
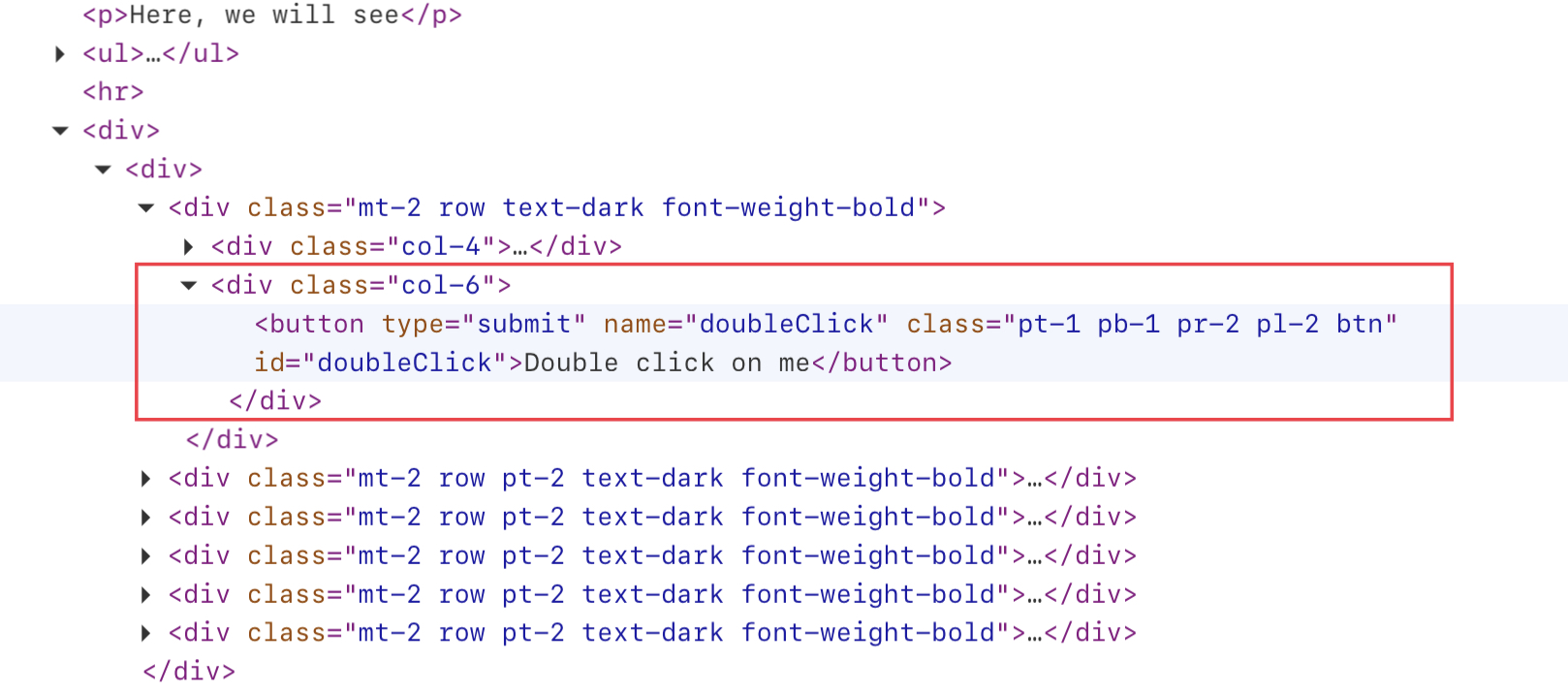
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Locator.ClickOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
Locator buttonLocator = page.locator("#doubleClick");
// performing double click
ClickOptions clickOptions = new ClickOptions();
clickOptions.setClickCount(2);
// clicking on the element
buttonLocator.click(clickOptions);
// closing the instances
browser.close();
playwright.close();
}
}
Above code will double-click on our element.
Skipping the actionability checks
As previously mentioned, the click() method in Playwright requires certain actionability checks to be met before executing the click action. However, we can bypass this requirement by utilizing the ClickOptions class.
The ClickOptions class includes a variable called “force,” whose value can be set using the setForce() method. The default value for this variable is false, but it can be changed to true in order to bypass actionability checks.
// skipping actionability checks
ClickOptions clickOptions = new ClickOptions();
clickOptions.setForce(true);
// clicking on the element
buttonLocator.click(clickOptions);
Let’s use the same disabled button that we used above. Previously, we got a TimeoutError exception, as the click() method waited for actionability checks to pass. However, by enabling the force value to true, the click() method will bypass the actionability checks and successfully click on the disabled element without throwing any exceptions.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Locator.ClickOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
Locator buttonLocator = page.locator("#disabledButton");
// skipping actionability checks
ClickOptions clickOptions = new ClickOptions();
clickOptions.setForce(true);
System.out.println("Before clicking");
// clicking on the element
buttonLocator.click(clickOptions);
System.out.println("After clicking");
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Before clicking
After clicking
Using keyboard modifier keys
According to Wikipedia, a modifier key is a special key (or combination) on a computer keyboard that temporarily modifies the normal action of another key when pressed together.
There are multiple modifier keys, but the click() method only allows below four keys –
- ALT
- CONTROL
- META
- SHIFT
Sometimes we need to perform operations like CONTROL + click that require pressing modifier keys along with clicking. To achieve this, we can use the setModifiers() method provided by the ClickOptions class.
setModifiers() method accepts a list of the keyboard modifiers.
// adding "CONTROL" key in the list
List<KeyboardModifier> keyboardModifiers = new ArrayList<KeyboardModifier>();
keyboardModifiers.add(KeyboardModifier.CONTROL);
// setting the modifiers
ClickOptions clickOptions = new ClickOptions();
clickOptions.setModifiers(keyboardModifiers);
// clicking on the element
buttonLocator.click(clickOptions);
We know that the “Control + click” operation will launch a new tab upon clicking an element that contains a hyperlink. So, let’s perform this operation on the below-highlighted element.

import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Locator.ClickOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
import com.microsoft.playwright.options.KeyboardModifier;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
Locator buttonLocator = page.locator("#disabledButton");
// adding "CONTROL" key in the list
List<KeyboardModifier> keyboardModifiers = new ArrayList<KeyboardModifier>();
keyboardModifiers.add(KeyboardModifier.CONTROL);
// setting the modifiers
ClickOptions clickOptions = new ClickOptions();
clickOptions.setModifiers(keyboardModifiers);
// clicking on the element
buttonLocator.click(clickOptions);
// closing the instances
browser.close();
playwright.close();
}
}
Set timeout
As mentioned earlier, the click() method has a default wait time of 30 seconds for actionability checks to pass. However, we change this timeout using the setTimeout() method.
setTiemout() accepts the time in milliseconds, and we can pass 0 to disable the timeout altogether.
The below code sets the timeout to 15 seconds.
// setting the modifiers
ClickOptions clickOptions = new ClickOptions();
clickOptions.setTimeout(15000);
// clicking on the element
buttonLocator.click(clickOptions);
Let’s again use our disabled element and see what happens when we set the timeout to 15 seconds.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Locator.ClickOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
Locator buttonLocator = page.locator("#disabledButton");
// setting the timeout to 15 seconds
ClickOptions clickOptions = new ClickOptions();
clickOptions.setTimeout(15000);
// clicking on the element
buttonLocator.click(clickOptions);
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Exception in thread "main" com.microsoft.playwright.TimeoutError: Error {
message='Timeout 15000ms exceeded.
=========================== logs ===========================
waiting for locator("#disabledButton")
locator resolved to <button disabled type="submit" id="disabledButton" name=…>Disabled button</button>
attempting click action
waiting for element to be visible, enabled and stable
element is not enabled - waiting...
============================================================
name='TimeoutError
stack='TimeoutError: Timeout 15000ms exceeded.
So, we again got the exception, but this time after waiting only for 15 seconds.
We hope that you have liked this article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.