You might have faced situations where you want to capture console logs from a website or application. Console logs can provide valuable insights into the performance, behaviour, and errors of your website or application.
This article will discuss capturing console logs using Playwright Java, a powerful tool for automating web browser interactions.
Playwright provides “page.onConsoleMessage()
” method to intercept and handle console messages. This method takes a callback function whenever a console message is logged on the page.
Below line of code will print every message shown on the browser console.
page.onConsoleMessage(msg -> System.out.println(msg.text()));
We’ll use one of our Playwright playground URLs ( https://testkru.com/TestUrls/TestConsoleLogs ), which displays multiple console messages.
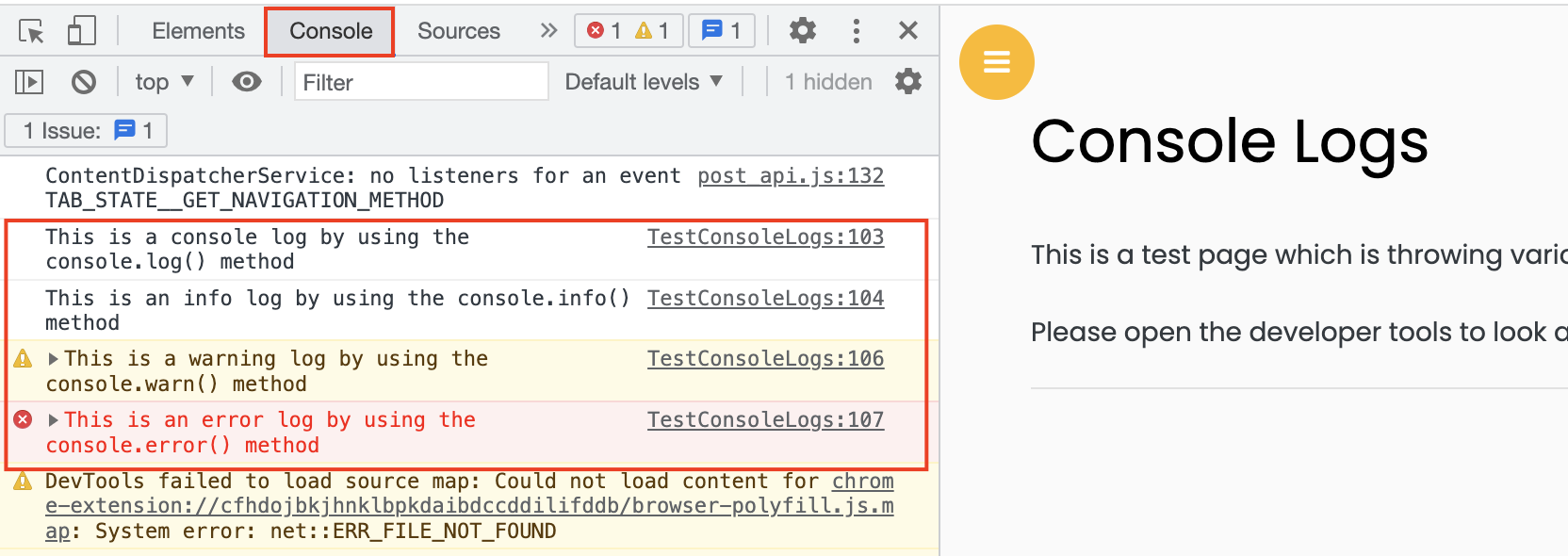
One additional debug message is present that is not visible in the screenshot above.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.onConsoleMessage(msg -> System.out.println(msg.text()));
page.navigate("https://testkru.com/TestUrls/TestConsoleLogs");
// closing the instances
browser.close();
playwright.close();
}
}
Output –
This is a console log by using the console.log() method
This is an info log by using the console.info() method
This is a debug log by using the console.debug() method
This is a warning log by using the console.warn() method
This is an error log by using the console.error() method
We can also print the type of the console message using msg.type()
. The type could any of the following –
"log", "debug", "info", "error", "warning", "dir", "dirxml", "table", "trace", "clear", "startGroup", "startGroupCollapsed", "endGroup", "assert", "profile", "profileEnd", "count", "timeEnd".
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.onConsoleMessage(msg -> {
System.out.println("Type: " + msg.type());
System.out.println("Message: " + msg.text());
});
page.navigate("https://testkru.com/TestUrls/TestConsoleLogs");
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Type: log
Message: This is a console log by using the console.log() method
Type: info
Message: This is an info log by using the console.info() method
Type: debug
Message: This is a debug log by using the console.debug() method
Type: warning
Message: This is a warning log by using the console.warn() method
Type: error
Message: This is an error log by using the console.error() method
This is it. We hope that you have liked the article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.