When automating web applications, clicking on an element is one of the most common actions that testers and developers perform. Playwright, a modern automation library for web browsers, provides a simple and efficient way to interact with web elements, including clicking on them. This post will cover ways to click an element in Playwright using Java.
- Using click() method
- And by using the evaluate() method
We will use the below-highlighted element as an example and click on it. You can find this element at https://testkru.com/Elements/Buttons.

Inspecting the element will reveal its DOM.
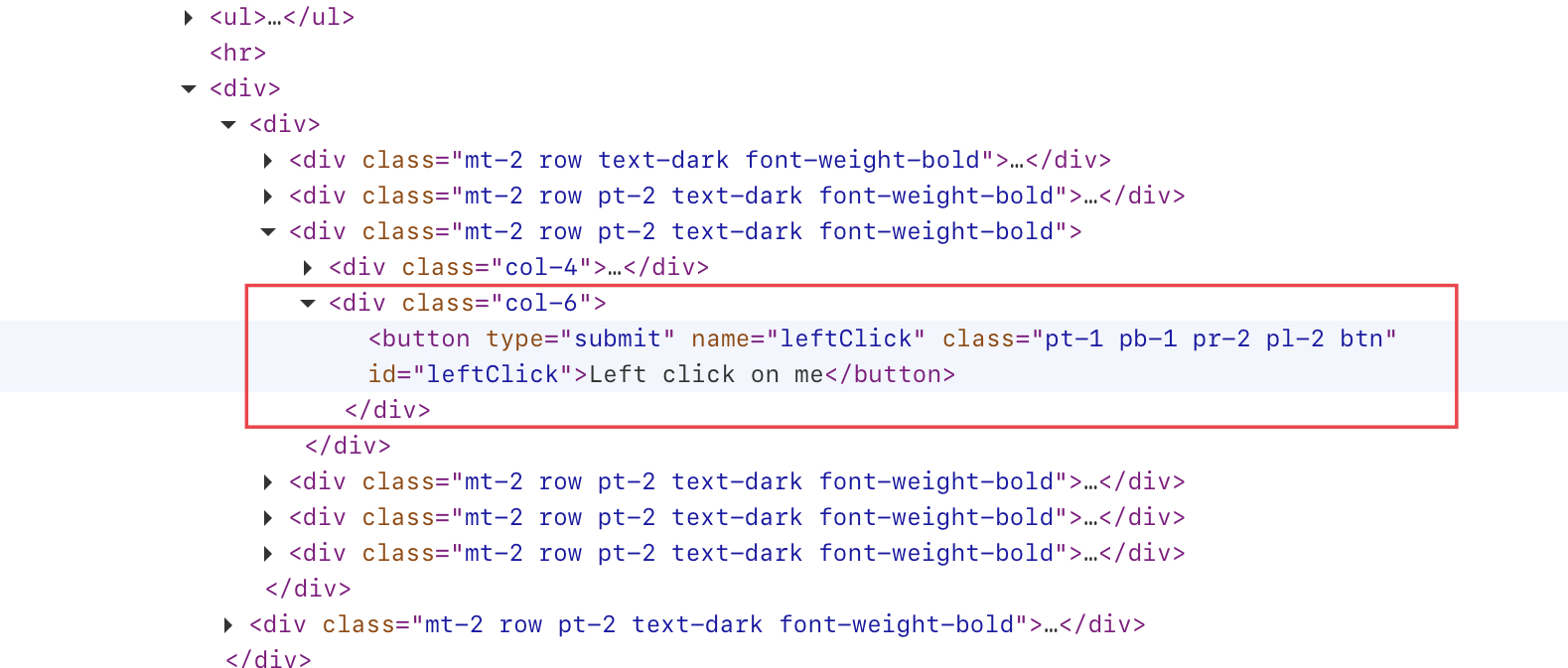
Now, let’s discuss the methods one by one.
Using click() method
Playwright has its click() method that helps click on an element. There are multiple implementations of the click() method, but it is recommended to use the Locator’s implementation of the click() method.
We can see that the id of the above-highlighted element is “leftClick“. We will locate the element with the help of the locator() method and then click on it using the click() method.
// locating the element
Locator locator = page.locator("#leftClick");
// Clicking on the element
locator.click();
Here is the whole code.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
// locating the element
Locator locator = page.locator("#leftClick");
// Clicking on the element
locator.click();
// closing the instances
browser.close();
playwright.close();
}
}
The above code will click on the button.
Other than using the click() method, we can also click on an element using the evaluate() method provided by the Playwright.
Using evaluate() method
evaluate() method in Playwright is used to execute the javascript code.
Javascript also provides its own click() method, which helps us to click on the specified element, and then we can execute that code using the evaluate() method in Playwright.
document.querySelector('#leftClick').click();
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
page.evaluate("document.querySelector('#leftClick').click();");
// closing the instances
browser.close();
playwright.close();
}
}
We hope that you have liked the article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.