When filling out a form, you may notice a small text hint in the input field or textarea. That is called the placeholder attribute, and it provides a brief description of the information that is expected to be entered. For example, the input field for last name may display the placeholder text “Enter your last name…” which will disappear once a value is entered.
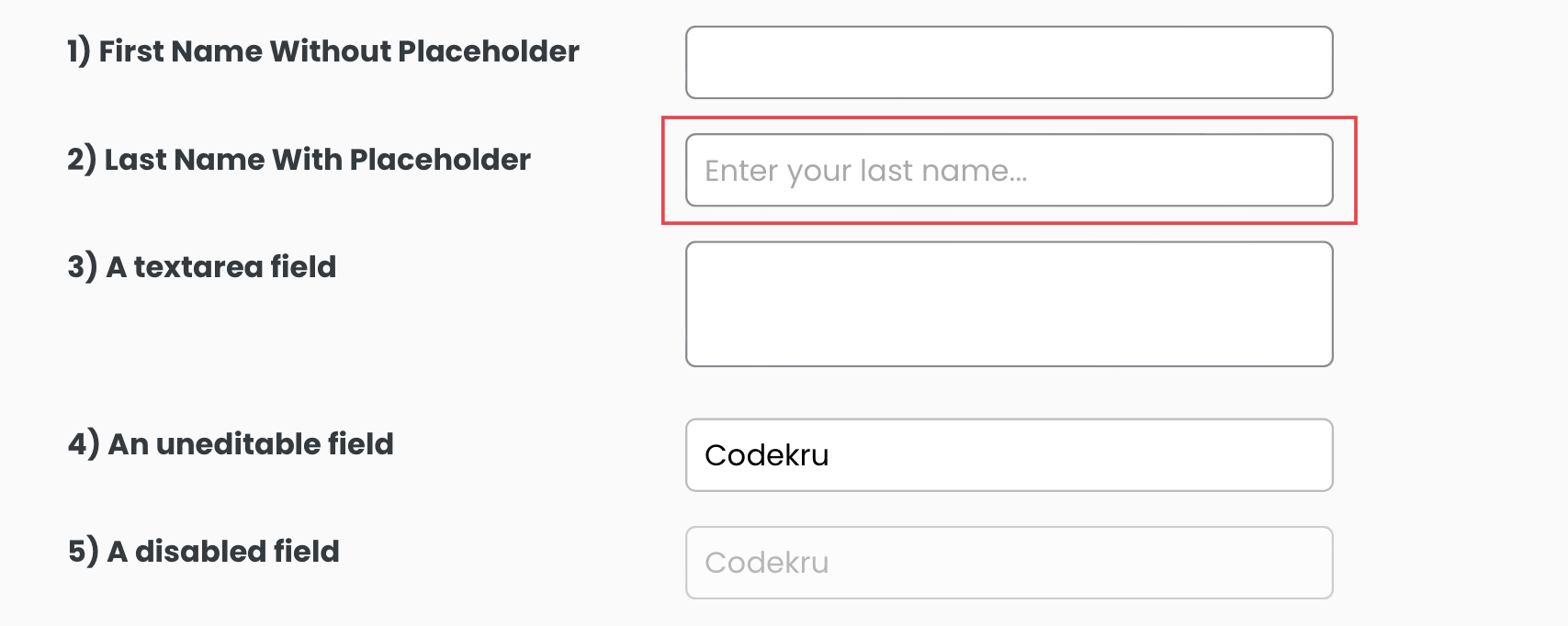
Today’s discussion will focus on acquiring placeholder text for an element through Playwright Java. We can use the following techniques to accomplish this task.
Let’s discuss them one by one.
Using getAttribute() method
We have created a comprehensive article about the getAttribute method. Reading it will give you more knowledge and understanding about this method.
But in short, the getAttribute() method retrieves an attribute’s value of an element. So, to get the placeholder text of an element, we can simply get its “placeholder” attribute value.
Let’s try to get the placeholder text of the below-highlighted image.
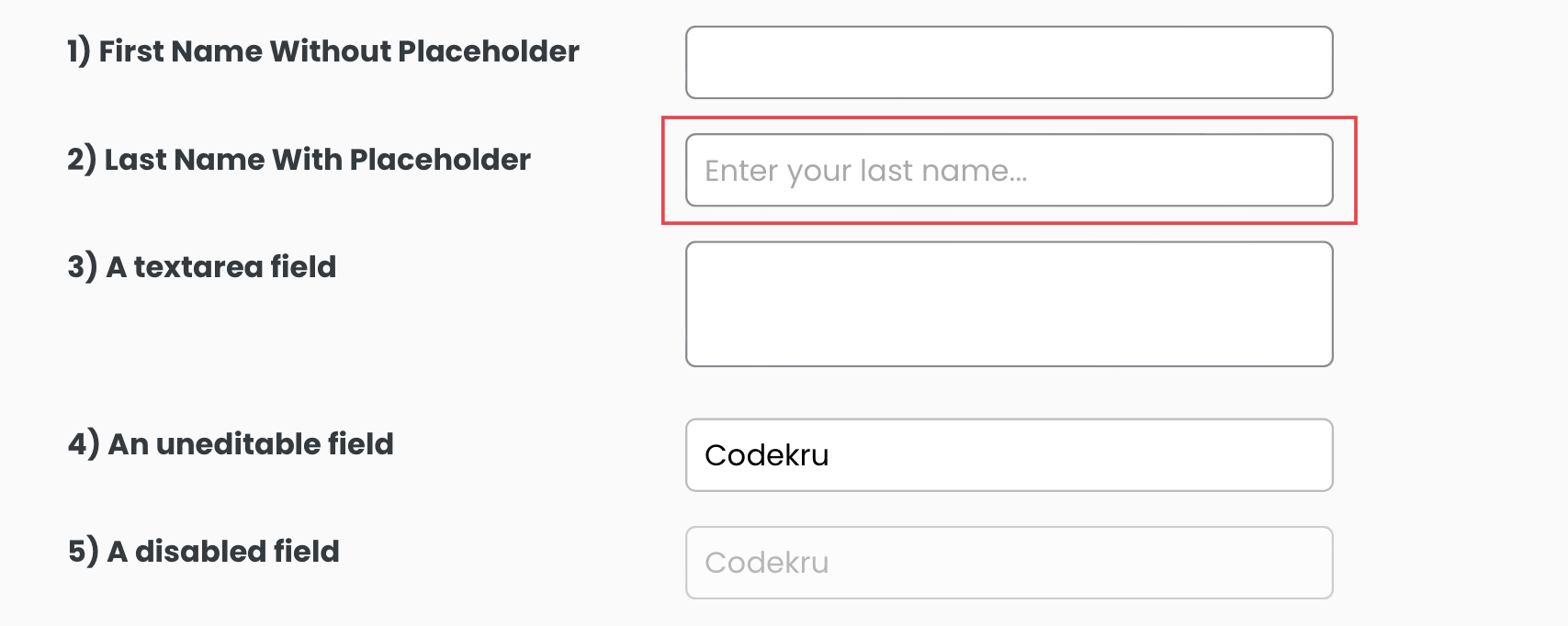
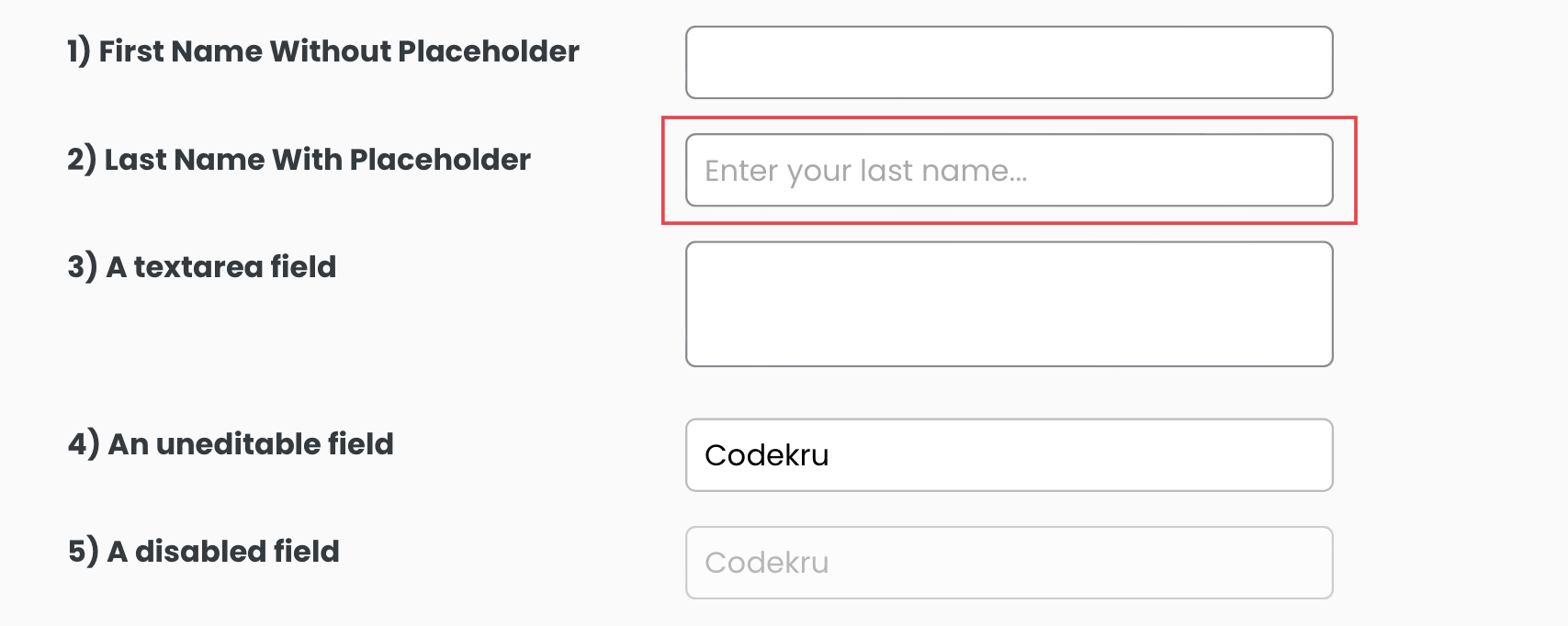
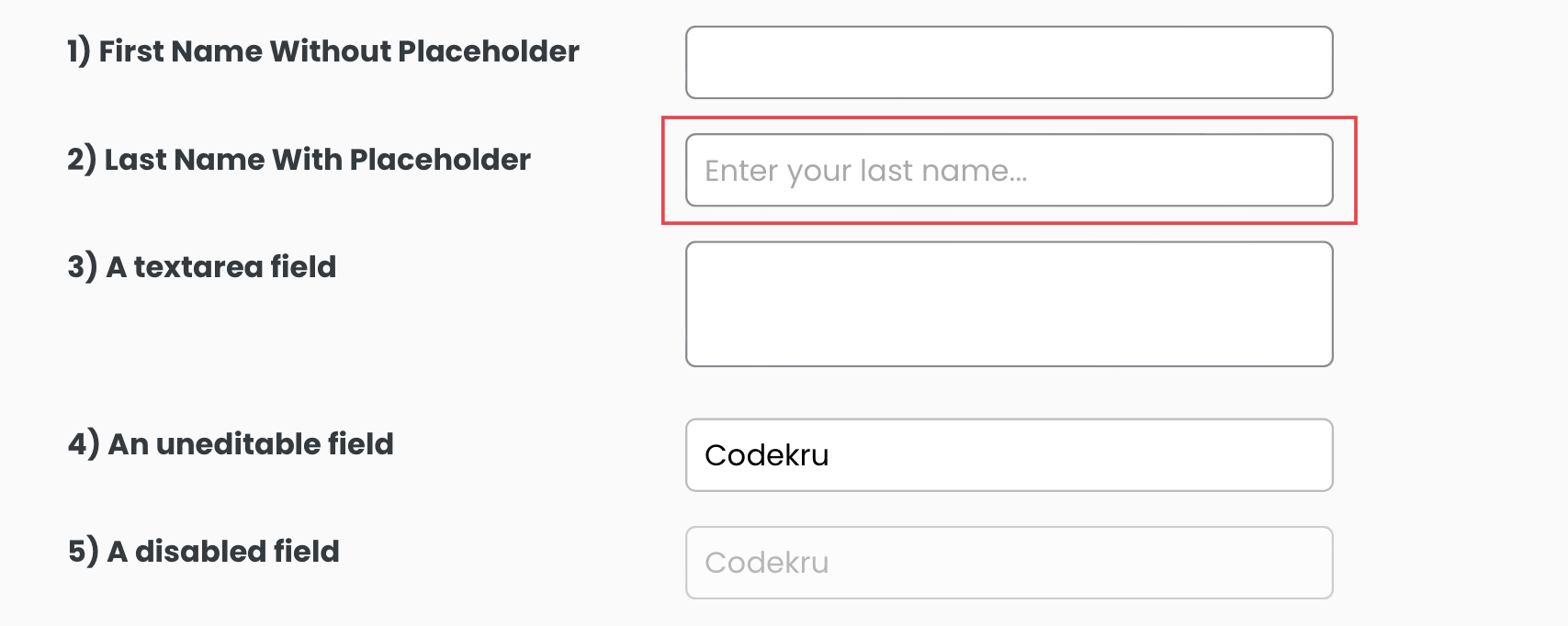
By inspecting the highlighted element, we can access its DOM and utilize the “id” attribute to locate it using the locator() method provided by Playwright.


We can locate the element using the below code.
page.locator("#lastNameWithPlaceholder")
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
Locator textLocator = page.locator("#lastNameWithPlaceholder");
// using getAttribute() method to get the placeholder text
System.out.println("Placeholder text: " + textLocator.getAttribute("placeholder"));
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Placeholder text: Enter your last name...
So, we got our placeholder text, “Enter your last name…”. Let’s attempt to obtain the same result with the help of evaluate() function.
Using evaluate() method
The evaluate() method is utilized to execute JavaScript code in Playwright. With this in mind, we can use JavaScript to obtain the placeholder text of an element.
Here is the code that assists in retrieving the placeholder text.
textLocator.evaluate("node => node.placeholder")
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
Locator textLocator = page.locator("#lastNameWithPlaceholder");
// using evaluate() method to get the placeholder text
System.out.println("Placeholder text: " + textLocator.evaluate("node => node.placeholder"));
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Placeholder text: Enter your last name...
We hope that you have liked the article. If you have any doubts or concerns, please write us in the comments or mail us at admin@codekru.com.