The getAttribute() method is a useful tool for retrieving an element’s attribute value, such as a link’s “href” or an image’s “src”. With this method, you can easily check attribute values and take actions based on them. Whether you’re dealing with dynamic web pages or testing user interfaces, having a solid understanding of the getAttribute method is crucial for efficient automation. In this post, we’ll dive into the details of Playwright’s getAttribute() method using Java.
Attributes are typically presented in the form of key-value pairs. For instance, the <input> tag possesses the type and id attributes.
<input type="text" id = "firstName">
type and id are the attribute keys, whereas “text” and “firstName” are their values.
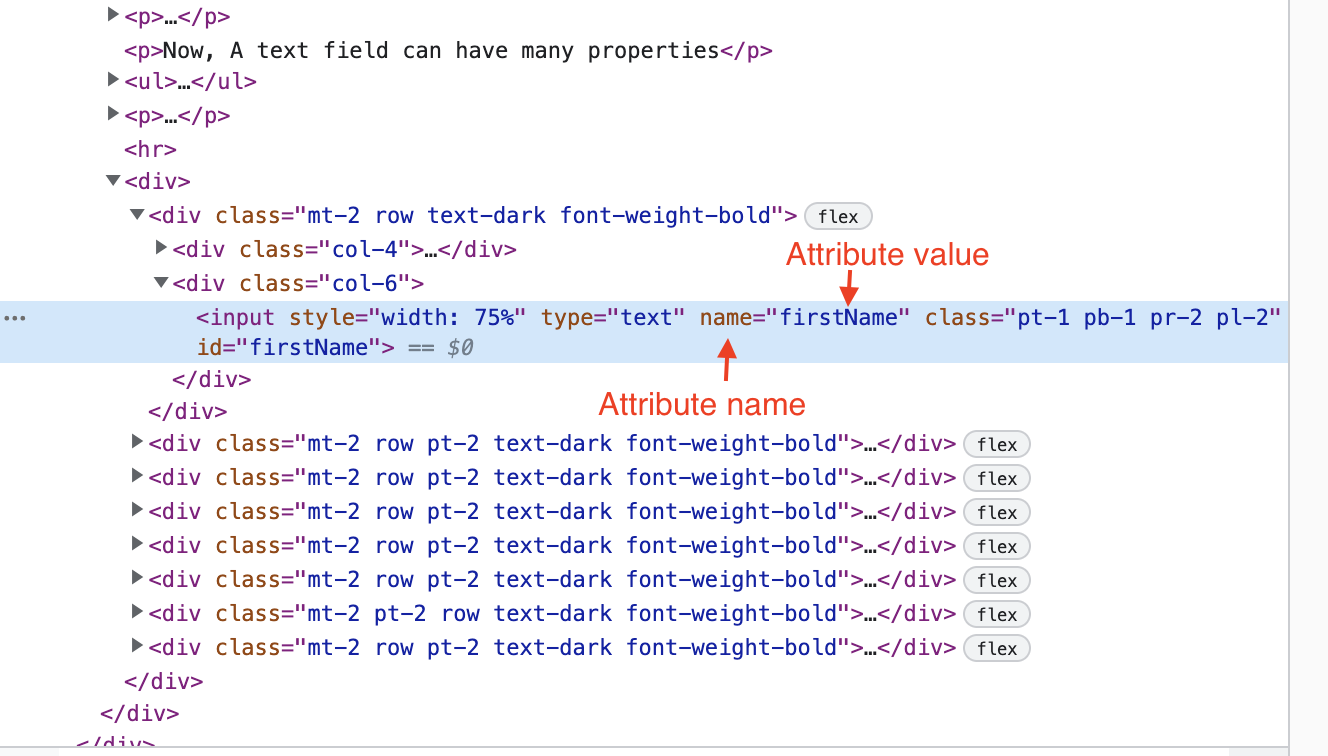
Although multiple implementations are provided by the Page, Locator and ElementHandle in Playwright, it is recommended to use the Locator’s implementation of the getAttribute() method.
Two overloaded implementations of the getAttribute() method are provided by the Locator’s implementation.
- default String getAttribute(String name)
- String getAttribute(String name, GetAttributeOptions options)
Let’s discuss both of them one by one.
default String getAttribute(String name)
It accepts the attribute key as an argument and returns that attribute’s value.
Let’s fetch the “name” attribute value of the below-highlighted element. This element is present on our playground website – https://testkru.com/Elements/TextFields.

Inspecting the element would reveal its DOM, where we can see multiple attributes associated with it.
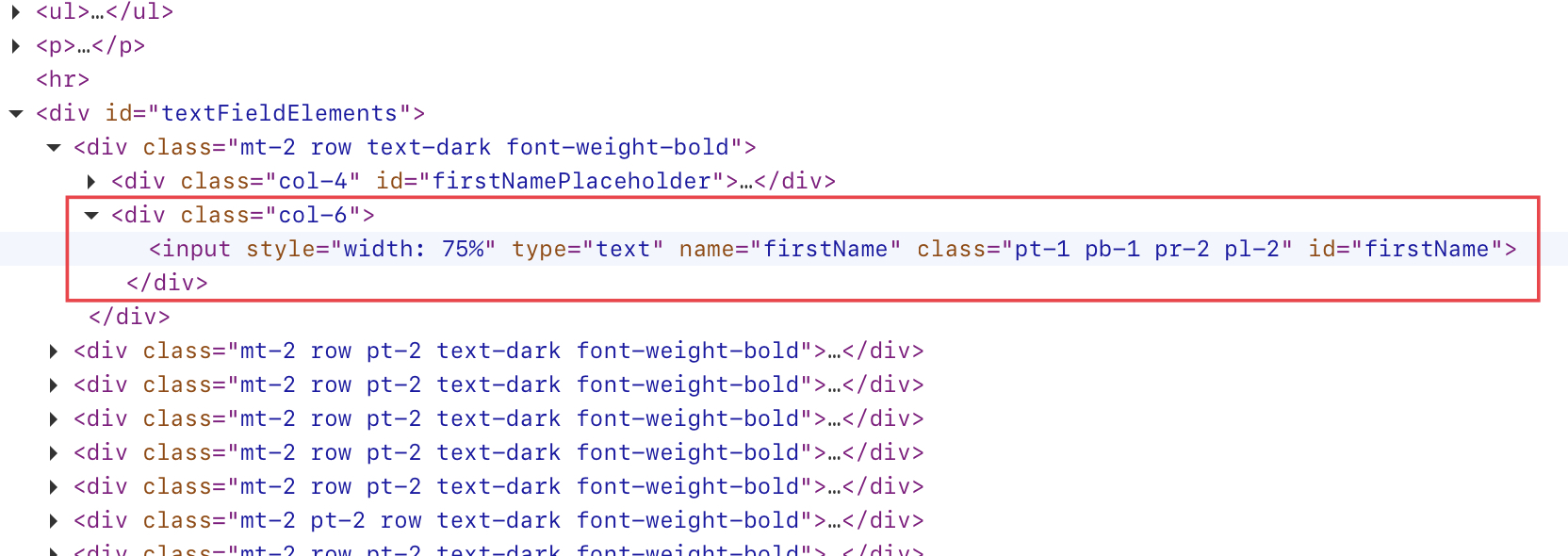
Here, we can see that the “name” attribute value is “firstName” and try to get the same using the getAttribute() method provided by the Playwright.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
Locator textLocator = page.locator("#firstName").first();
// using getAttribute() method
System.out.println("\"name\" attribute value: " + textLocator.getAttribute("name"));
// closing the instances
browser.close();
playwright.close();
}
}
Output –
"name" attribute value: firstName
In a similar way, we can get the value of any attribute associated with the element.
Attributes normally come in key-value pairs, but this isn’t always true. E.g. “disabled” attribute doesn’t have any value associated with it, as shown in the below image.
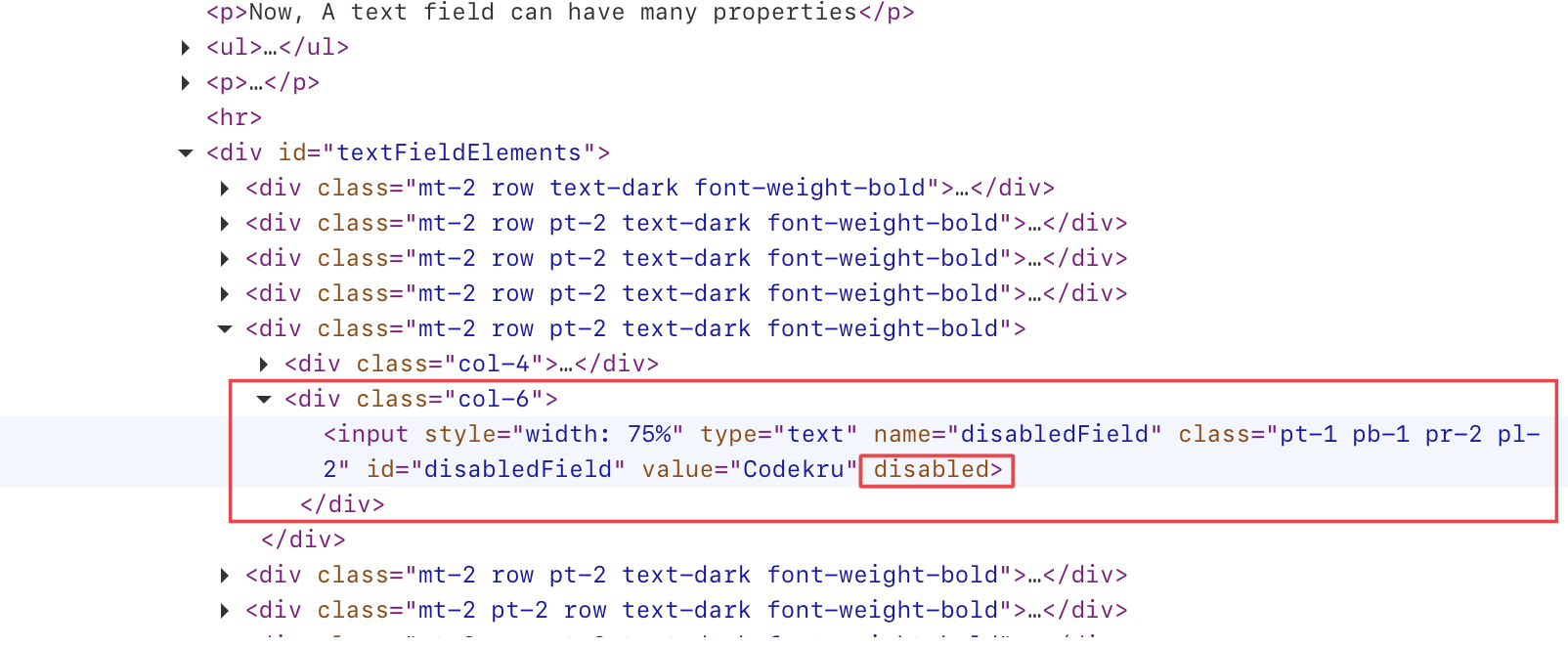
In these cases, the getAttribute() method would return an empty string if attributes don’t have any value attached to them.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
Locator textLocator = page.locator("#disabledField").first();
// using getAttribute() method
System.out.println("Attribute value: " + textLocator.getAttribute("disabled"));
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Attribute value:
Internal implementation of the getAttribute() method
default String getAttribute(String name) {
return getAttribute(name, null);
}
getAttribute(String name) internally uses the getAttribute(String name, GetAttributeOptions options). So, let’s discuss it next.
String getAttribute(String name, GetAttributeOptions options)
It also fetches the attribute value associated with an element while considering the options ( passed as an argument ).
What are these options?
option is an object of the GetAttributeOptions class, which only has one variable and a method.
class GetAttributeOptions {
public Double timeout;
public GetAttributeOptions setTimeout(double timeout) {
this.timeout = timeout;
return this;
}
}
So, the option will help us set the timeout while fetching an attribute’s value.
But where is the timeout used while fetching the attribute’s value?
timeout is the maximum time the getAttribute() method will wait to get the attribute value. If the value is not retrieved in the specified time, it throws a TimeoutError exception.
By default, the timeout is set to 30 seconds.
We can change the timeout using the GetAttributeOptions class and pass it in the getAttribute() method.
GetAttributeOptions getAttributeOptions = new GetAttributeOptions();
getAttributeOptions.setTimeout(10); // time in milliseconds
locator.getAttribute("attribute_name",getAttributeOptions);
The timeout is passed in milliseconds.
Let’s use the timeout in our example now.
We will set the timeout to 10 milliseconds. It will throw the TimeoutError exception, as it takes more than ten milliseconds to fetch the attribute’s value for our element.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Locator.GetAttributeOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
Locator textLocator = page.locator("#firstName").first();
GetAttributeOptions getAttributeOptions = new GetAttributeOptions();
getAttributeOptions.setTimeout(10); // time in milliseconds
// using getAttribute() method
System.out.println("Attribute value: " + textLocator.getAttribute("name", getAttributeOptions));
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Exception in thread "main" com.microsoft.playwright.TimeoutError: Error {
message='Timeout 10ms exceeded.
=========================== logs ===========================
waiting for locator("#firstName").first()
============================================================
name='TimeoutError
stack='TimeoutError: Timeout 10ms exceeded.
=========================== logs ===========================
waiting for locator("#firstName").first()
The What If scenarios
Q – What if we try to get an attribute’s value that doesn’t exist?
Let’s see this with an example as well. We will try to the value of the “qwerty” attribute.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
Locator textLocator = page.locator("#firstName").first();
// using getAttribute() method
System.out.println("Attribute value: " + textLocator.getAttribute("qwerty"));
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Attribute value: null
We can see that the getAttribute() method in Playwright returns null when an invalid attribute is passed as an argument.
Q – What if we pass the “null” argument in the getAttribute() method?
It will throw a PlaywrightException, as illustrated in the below program.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
Locator textLocator = page.locator("#firstName").first();
// using getAttribute() method
System.out.println("Attribute value: " + textLocator.getAttribute(null));
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Exception in thread "main" com.microsoft.playwright.PlaywrightException: Error {
message='name: expected string, got undefined
name='Error
stack='Error: name: expected string, got undefined
We hope that you have liked the article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.