Capturing screenshots is an essential task for various purposes, such as bug reporting, documentation, and website testing. Playwright provides a user-friendly approach to capture screenshots programmatically in Java. This article will explore how to take screenshots using Playwright in Java, enabling us to automate this process and enhance testing and debugging workflows.
We will delve into the following topics –
- Capture a screenshot of the whole webpage
- Capture a screenshot of an element
- Capturing screenshots into a buffer
Let’s discuss them one by one.
Capture a screenshot of the whole webpage
In Playwright, the Page interface offers a screenshot() method that enables us to capture a screenshot of our page and save it to any location on our device.
To take a screenshot, the screenshot() method requires a ScreenshotOptions class object as an argument. This class enables one to define various parameters that determine how the screenshot should be taken.
Capturing and storing a screenshot
Suppose we want to save the screenshot as a file named “screenshot.png”. The following code can assist us in achieving this task.
ScreenshotOptions screenshotOptions = new ScreenshotOptions();
screenshotOptions.setPath(Paths.get("screenshot.png"));
page.screenshot(screenshotOptions);
You may have observed that we have only specified the file name (“screenshot.png”) without indicating its storage location. In such instances, the file will be saved in the current working directory’s root.

Now, let’s take a screenshot of our playground website ( https://testkru.com/Elements/TextFields ).
import java.nio.file.Paths;
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserContext;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Page.ScreenshotOptions;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
// opening up the browser so that we can see the console message printed on the
// browser
Browser browser = playwright.chromium().launch();
// creating a BrowserContext
BrowserContext browserContext = browser.newContext();
// creating the page
Page page = browserContext.newPage();
// Navigating to the URL
page.navigate("https://testkru.com/Elements/TextFields");
ScreenshotOptions screenshotOptions = new ScreenshotOptions();
screenshotOptions.setPath(Paths.get("screenshot.png"));
// capturing screenshot in a file named "screenshot.png"
page.screenshot(screenshotOptions);
browser.close();
playwright.close();
}
}
Run the above code, and a screenshot with the file name “screenshot.png” will be saved in the current working directory’s root.
If you look at the screenshot, then you’ll see that only the visible portion of the webpage was captured.
If we need to capture the entire web page, including the content that is visible only after scrolling, then Playwright offers a way to accomplish this.
Taking a screenshot of an entire webpage, including the content that is visible only after scrolling
With the setFullPage() method in ScreenshotOptions, we can modify the value of the fullPage variable. The “fullPage” variable determines if the screenshot should capture the entire webpage or only the visible portion. By default, it’s set to false. However, when you set it to true, Playwright will capture a screenshot of the whole webpage, even the content that requires scrolling.
ScreenshotOptions screenshotOptions = new ScreenshotOptions();
screenshotOptions.setPath(Paths.get("screenshot.png"));
screenshotOptions.setFullPage(true);
page.screenshot(screenshotOptions);
Now, if we take a screenshot, then it will capture the entire webpage.
import java.nio.file.Paths;
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserContext;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Page.ScreenshotOptions;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
// opening up the browser so that we can see the console message printed on the
// browser
Browser browser = playwright.chromium().launch();
// creating a BrowserContext
BrowserContext browserContext = browser.newContext();
// creating the page
Page page = browserContext.newPage();
// Navigating to the URL
page.navigate("https://testkru.com/Elements/TextFields");
ScreenshotOptions screenshotOptions = new ScreenshotOptions();
screenshotOptions.setPath(Paths.get("screenshot.png"));
screenshotOptions.setFullPage(true);
// capturing screenshot in a file named "screenshot.png"
page.screenshot(screenshotOptions);
browser.close();
playwright.close();
}
}
Capture a screenshot of an element
In certain cases, we may only need to capture a specific element or locator instead of the entire webpage. In such situations, we can use the screenshot() method offered by the Locator to accomplish this task. This method functions in a similar way to the one provided by the Page interface.
We will take a screenshot of the below-highlighted element present on the page – https://testkru.com/Elements/TextFields.
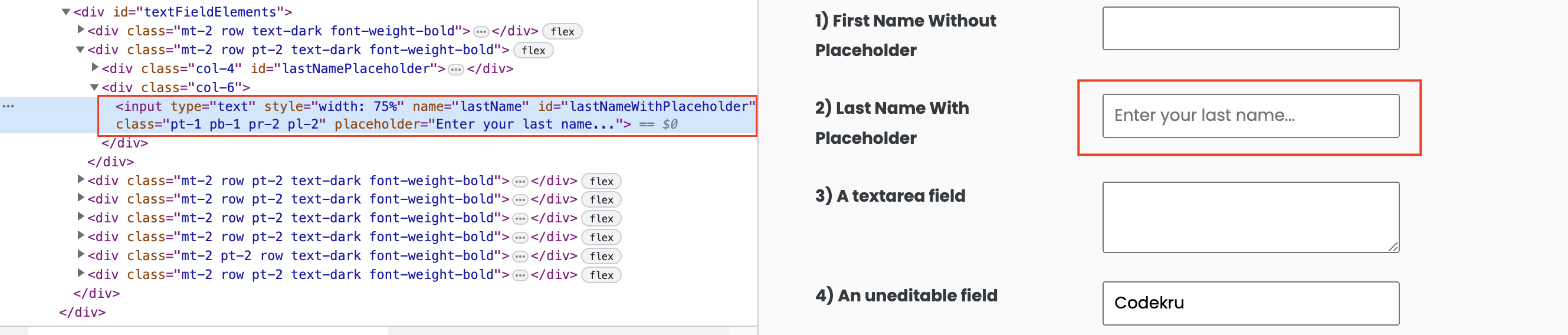
The id of the highlighted element is “lastNameWithPlaceholder“. We can use it to locate the element using the locator() method provided by the Page interface.
Locator locator = page.locator("#lastNameWithPlaceholder");
Now, we can use the screenshot() method to capture the specific element.
Locator locator = page.locator("#lastNameWithPlaceholder");
ScreenshotOptions screenshotOptions = new ScreenshotOptions();
screenshotOptions.setPath(Paths.get("screenshot.png"));
locator.screenshot(screenshotOptions);
Note – Locator’s screenshot() method doesn’t have the setFullPage() method.
The below code will take a screenshot of a specific element.
import java.nio.file.Paths;
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserContext;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Locator.ScreenshotOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
// opening up the browser so that we can see the console message printed on the
// browser
Browser browser = playwright.chromium().launch();
// creating a BrowserContext
BrowserContext browserContext = browser.newContext();
// creating the page
Page page = browserContext.newPage();
// Navigating to the URL
page.navigate("https://testkru.com/Elements/TextFields");
// locating the element
Locator locator = page.locator("#lastNameWithPlaceholder");
ScreenshotOptions screenshotOptions = new ScreenshotOptions();
screenshotOptions.setPath(Paths.get("screenshot1.png"));
locator.screenshot(screenshotOptions);
browser.close();
playwright.close();
}
}
Capturing screenshots into a buffer
We can also store the screenshot in a buffer instead of files. Capturing screenshots into a buffer has many advantages –
- Faster Processing
- Efficient Image Manipulation
- Reduced Disk Space Usage
The below line of code takes a screenshot of the webpage and stores it in a buffer.
byte[] buffer = page.screenshot();
Here is the whole code
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserContext;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
// Launching the browser
Browser browser = playwright.chromium().launch();
// creating a BrowserContext
BrowserContext browserContext = browser.newContext();
// creating the page
Page page = browserContext.newPage();
// Navigating to the URL
page.navigate("https://testkru.com/Elements/TextFields");
// capturing screenshot into a buffer
byte[] buffer = page.screenshot();
// closing the instances
browser.close();
playwright.close();
}
}
We hope that you have liked this article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.