The isEditable() method in Playwright Java is used to determine whether an element is editable or not on a web page. This method can be very useful in test automation, as it can ensure that only the expected fields can be modified by users. This article will discuss on how to use the isEditable() method in Playwright Java and how it can help write reliable and robust automated tests for web applications.
According to the documentation, an element is considered editable when it is enabled and does not have readonly
property set.
Multiple interfaces have declared the isEditable() method in Playwright – Page, Locator, and ElementHandle.
Out of all the implementations, it is recommended to use the Locator implementation of the isEditable() method. So, we will discuss it only and leave the other Implementations.
Two overloaded implementations of the isEditable() method are provided by the Locator in Playwright.
Let’s look at each of them one by one.
default boolean isEditable()
- What does it do? It tells whether the element is editable or not.
- What does it return? It returns “true” if an element is editable; otherwise, it returns “false”.
We will use the below-highlighted element on our playground website – https://testkru.com/Elements/TextFields.
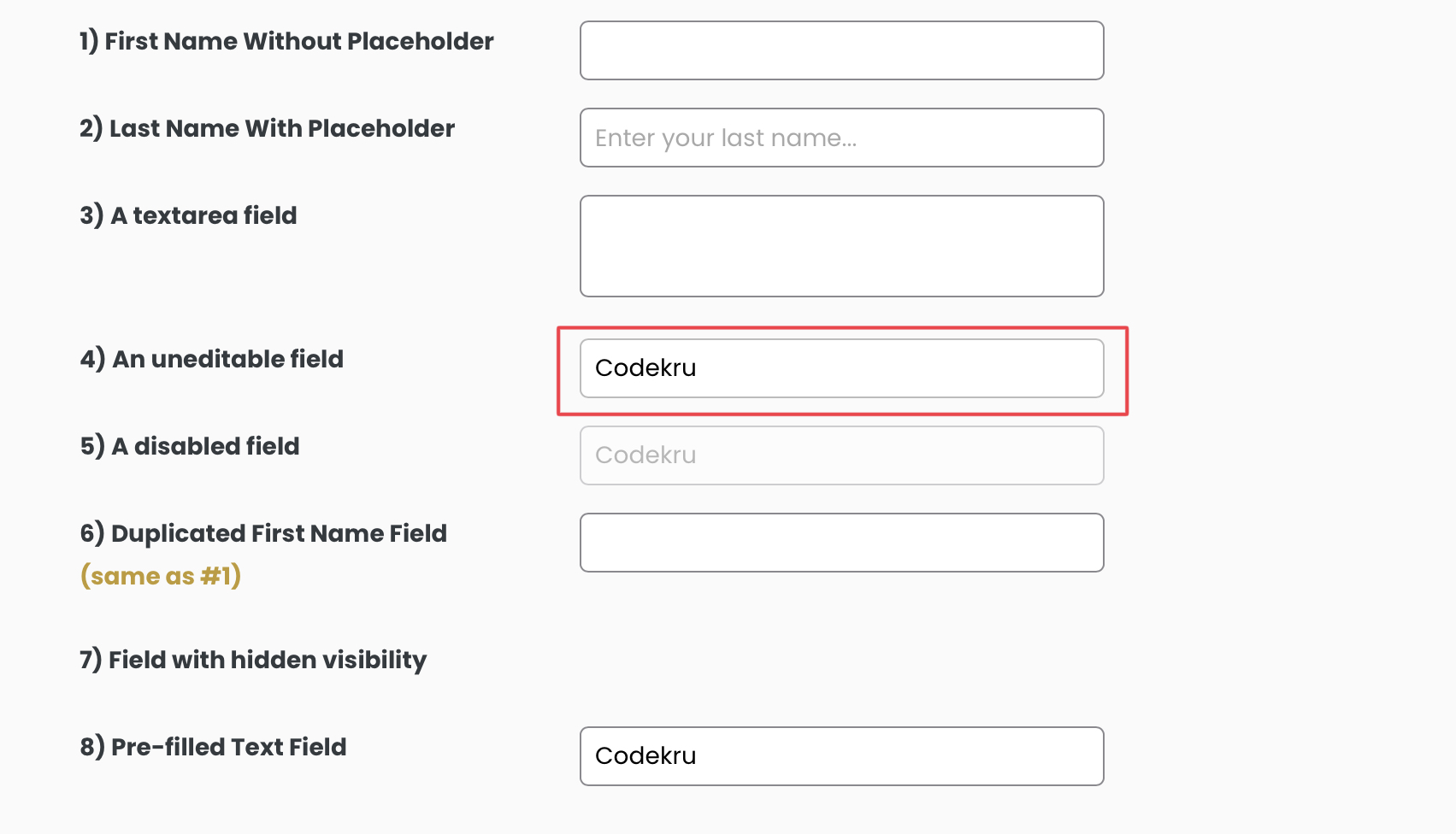
The element has the “readonly” attribute, so the field is not editable. So, the isEditable() method should return “false”.

Now, let’s see the things in action with code.
- First, we will locate the element using Playwright’s locator() method. We can use the id attribute value to locate the element.
page.locator("#uneditable")
- And, then we can use the isEditable() method on the element.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
Locator textFieldLocator = page.locator("#uneditable");
// Using "isEditable()" method
System.out.println("is field editable: " + textFieldLocator.isEditable());
// closing the instances
browser.close();
playwright.close();
}
}
Output –
is field editable: false
We can see that the method returned “false” because the field wasn’t editable.
boolean isEditable(IsEditableOptions options)
We can also pass an IsEditableOptions class’s object as an argument for customizing the behaviour of the isEditable() method.
IsEditableOptions class has only one variable and a method.
class IsEditableOptions {
public Double timeout;
public IsEditableOptions setTimeout(double timeout) {
this.timeout = timeout;
return this;
}
}
So, we can set a timeout while using the isEditable() method.
The default timeout is set to 30 seconds. We can override it using the options, and if the result doesn’t come in that specified time, then isEditable() method will throw a TimeoutError exception.
Note: Timeout is passed in milliseconds
We will set the timeout to 10ms in our example.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Locator.IsEditableOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
IsEditableOptions isEditableOptions = new IsEditableOptions();
isEditableOptions.setTimeout(10); // setting the timeout to 10ms
Locator textFieldLocator = page.locator("#uneditable");
// Using "isEditable()" method
System.out.println("is field editable: " + textFieldLocator.isEditable(isEditableOptions));
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Exception in thread "main" com.microsoft.playwright.TimeoutError: Error {
message='Timeout 10ms exceeded.
=========================== logs ===========================
waiting for locator("#uneditable")
============================================================
name='TimeoutError
stack='TimeoutError: Timeout 10ms exceeded.
=========================== logs ===========================
waiting for locator("#uneditable")
Few QnA
What if we use the isEditable() method on a null element?
It will throw a NullPointerException, as illustrated in the below example.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserType;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch( new BrowserType.LaunchOptions().setHeadless(false)); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
Locator textFieldLocator = null;
// Using "isEditable()" method
System.out.println("is field editable: " + textFieldLocator.isEditable());
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "com.microsoft.playwright.Locator.isEditable()" because "textFieldLocator" is null
Can we use isEditable() method on a non <input> type element?
We can use isEditable() method on any element. It is not restricted to <input> type element.
What if we use the isEditable() method on a disabled field?
A disabled field is different from a non-editable field. The disabled field has a “disabled” attribute, and the non-editable field has a “readonly” attribute. Please read this article to learn the difference between “disabled” and “readonly” attributes.
So, let’s use the isEditable() method on a disabled element. Lucky for us, our playground website has one such element on https://testkru.com/Elements/TextFields.
The isEditable() method should return “false” because we cannot edit a disabled field.
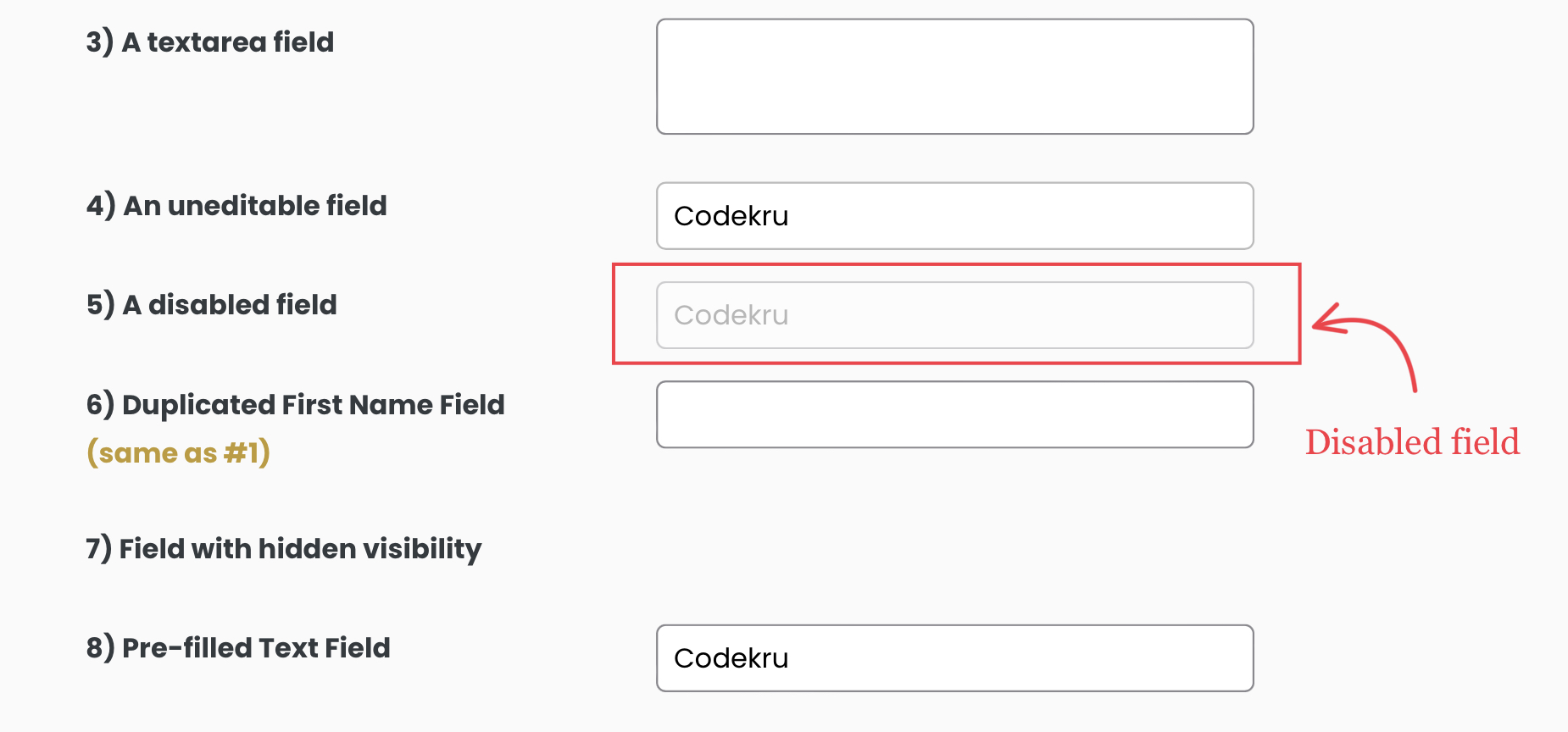
Inspecting on the element would reveal its DOM.
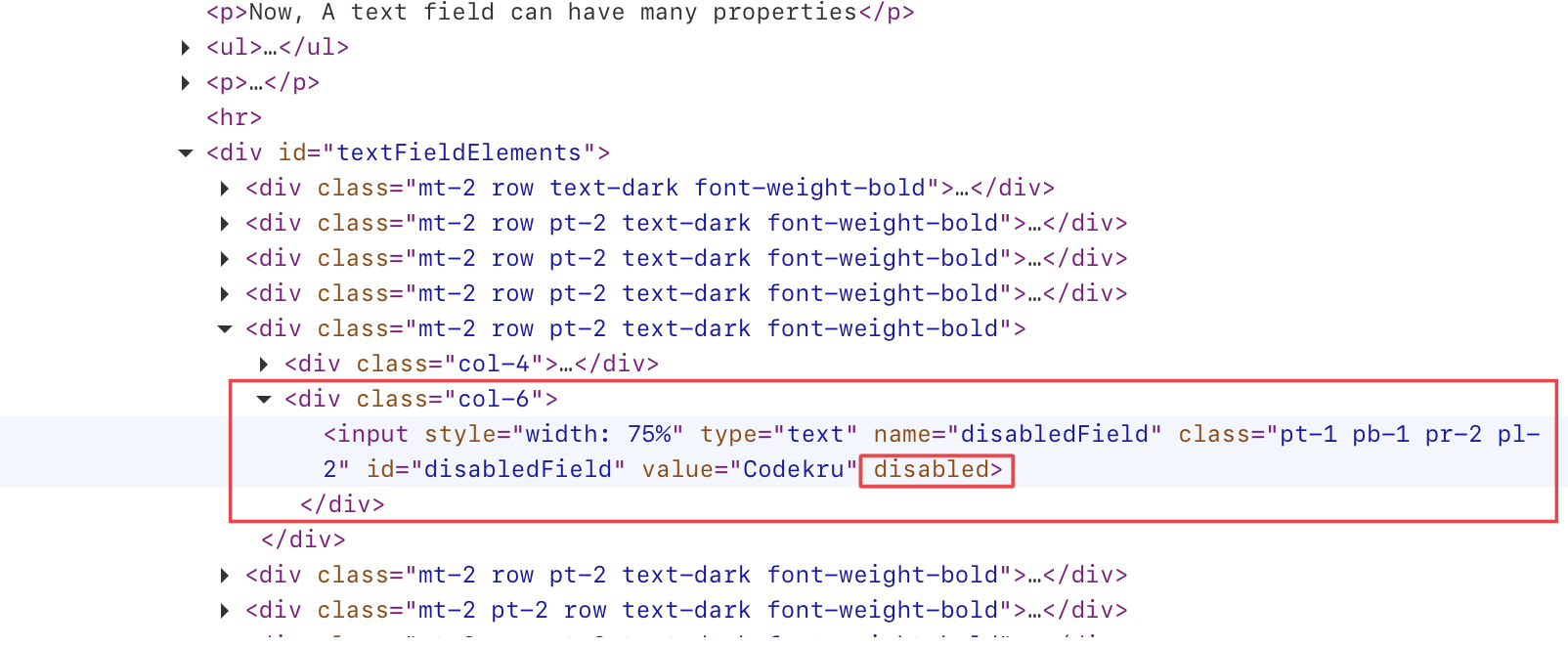
We can use the id attribute value “disabledField” to locate the element and then use the isEditable() method on it.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
Locator textFieldLocator = page.locator("#disabledField");
// Using "isEditable()" method
System.out.println("is field editable: " + textFieldLocator.isEditable());
// closing the instances
browser.close();
playwright.close();
}
}
Output –
is field editable: false
We hope that you have liked the article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.