This article will discuss the hover() method in Playwright using Java to simulate hovering over an element in web automation. This article covers everything you need to know about the hover() method, including how to use it, what it does, and how it can help you automate web interactions efficiently.
Many interfaces in Java have declared the hover() method – Page, Locator and ElementHandle.
Playwright recommends using the Locator hover() method, so we will discuss only the Locator implementation of the hover() method in this article.
The Locator provides two overloaded implementations of the hover method.
Let’s look at them one by one.
default void hover()
- What does it do? It hovers over the specified element.
- What does it return? It doesn’t return anything, as the method’s return type is void.
Let’s pick the below-highlighted button on our selenium playground website – https://testkru.com/Elements/Buttons.
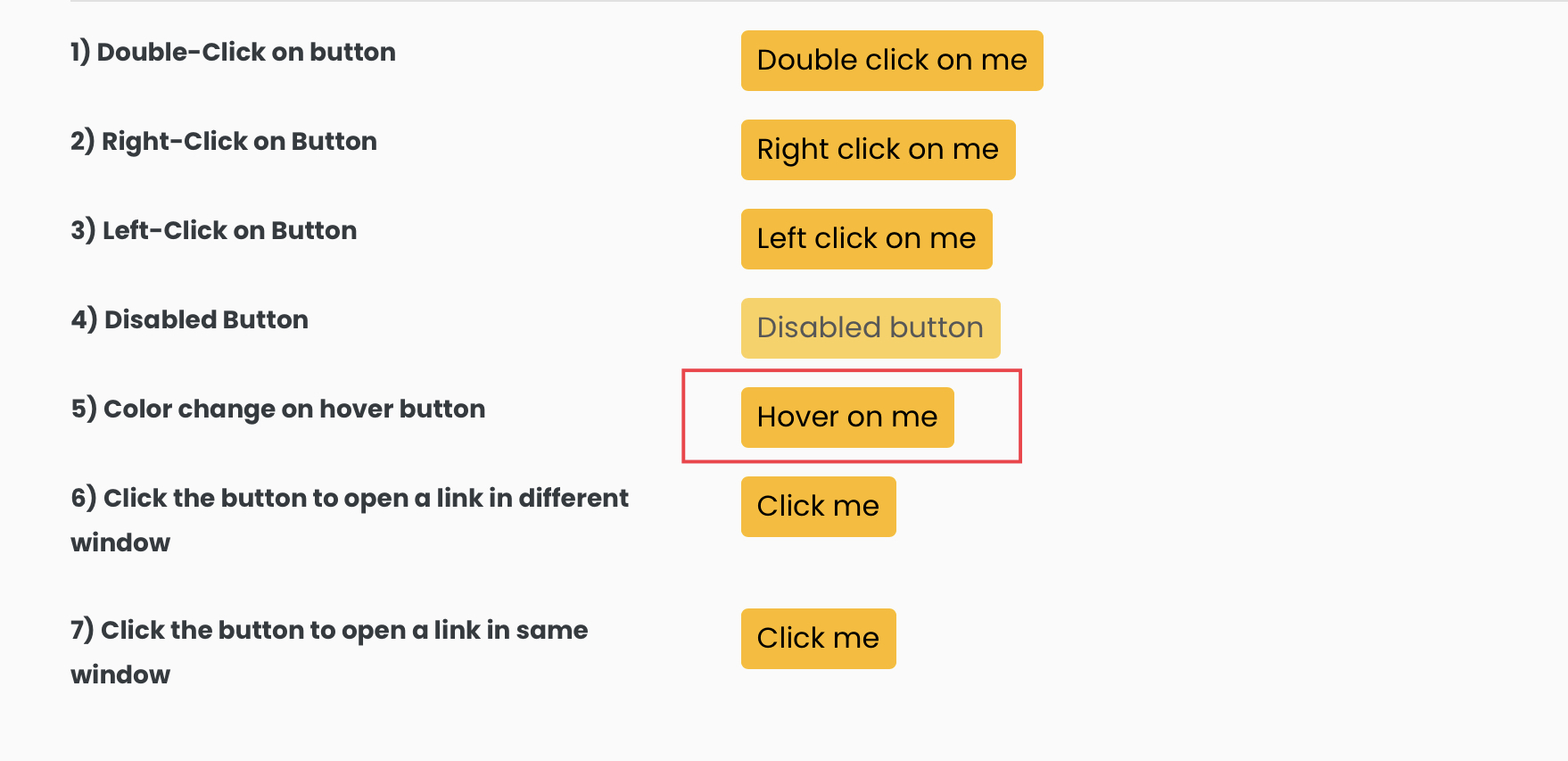
Let’s inspect the highlighted element to find any identifier which can be used to locate the element.
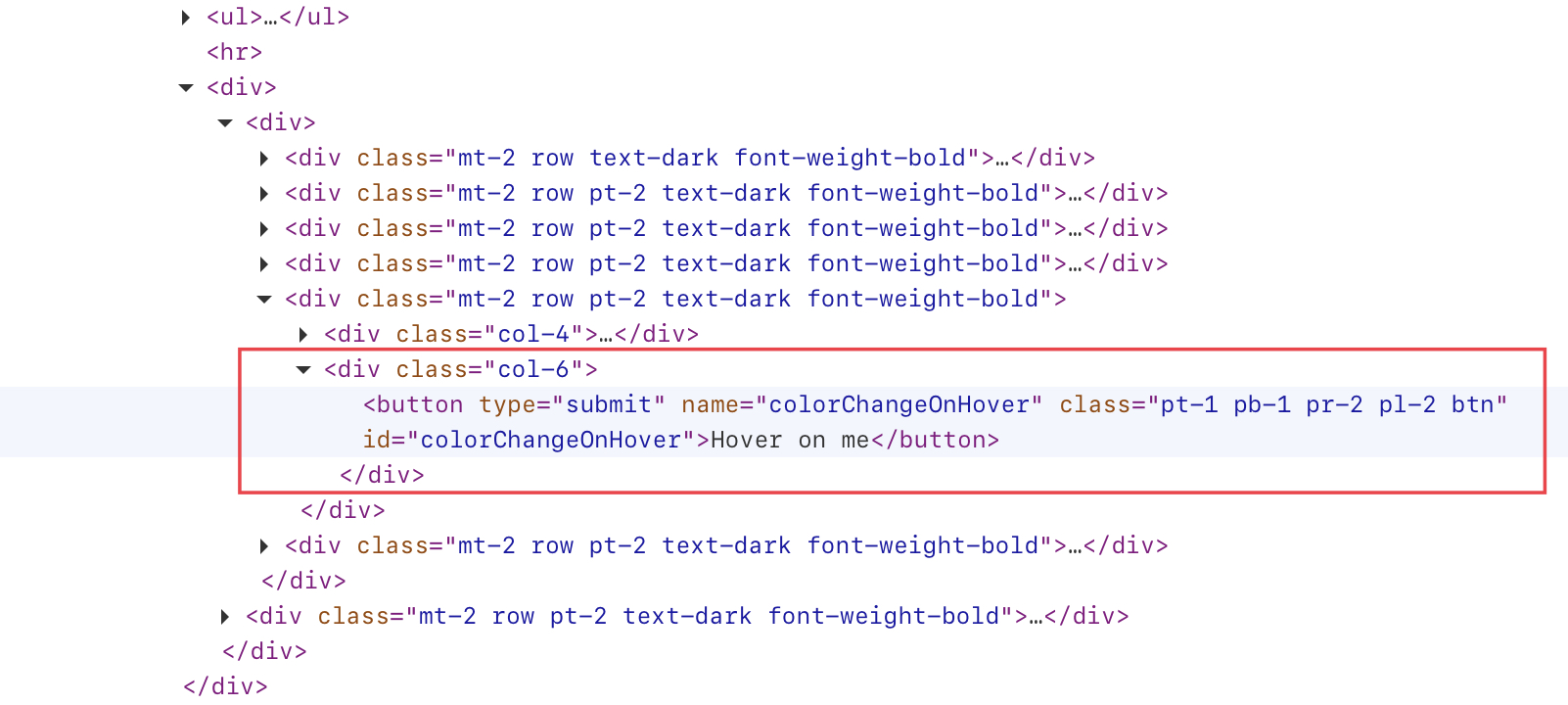
We can see that the id attribute value is “colorChangeOnHover“. We can use it to locate the element using the locator() method provided by the Page.
page.locator("#colorChangeOnHover")
And then, we cause the hover() method for hovering over the element.
buttonLocator.hover()
Here is the whole code in action.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
Locator buttonLocator = page.locator("#colorChangeOnHover");
buttonLocator.hover(); // hovering over the element
// closing the instances
browser.close();
playwright.close();
}
}
While hovering over the element, the button colour will change.

Internal implementation of the hover() method
default void hover() {
hover(null);
}
We can see that hover() uses the hover(HoverOptions options) internally. So, let’s discuss the hover(HoverOptions options) in detail.
void hover(HoverOptions options)
- What does it do? It hovers over the specified element and accepts options as the parameter.
- What does it return? It doesn’t return anything, as the method’s return type is void.
Now, what are the HoverOptions?
There are six options which we can use.
Let’s discuss a few of them.
force
If its value is set to true, then it can be used to bypass the actionability checks.
By default, its value is false; thus, all actionability checks should be passed, and only then will the hover() function hover over the element.
Now, what are these actionability checks?
- Attached
- Visible
- Stable
- Receives Events
hover() method will wait for the above actionability checks to pass before moving ahead with the execution. So, if we want to bypass these checks, we can use the force attribute and set it to true, as shown below.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Locator.HoverOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
Locator buttonLocator = page.locator("#colorChangeOnHover");
// setting "force" value as "true"
HoverOptions hoverOptions = new HoverOptions();
hoverOptions.setForce(true);
buttonLocator.hover(hoverOptions); // hovering over the element
// closing the instances
browser.close();
playwright.close();
}
}
timeout
hover() function waits for 30 seconds for actionability checks to pass. We can change that timeout using the “timeout” attribute.
Let’s pick an invisible field present on the page – https://testkru.com/Elements/TextFields.

As the element is not visible, the hover() function will wait 30 seconds before throwing an exception.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
Locator buttonLocator = page.locator("#hiddenText");
buttonLocator.hover(); // hovering over the element
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Exception in thread "main" com.microsoft.playwright.TimeoutError: Error {
message='Timeout 30000ms exceeded.
We can change this timeout and set it to wait for an amount as per our requirement. We can set its value as zero ( 0 ) to disable the timeout.
The timeout is in milliseconds.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Locator.HoverOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/TextFields");
// setting a timeout of 3 sec
HoverOptions hoverOptions = new HoverOptions();
hoverOptions.setTimeout(3000);
Locator buttonLocator = page.locator("#hiddenText");
buttonLocator.hover(hoverOptions); // hovering over the element
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Exception in thread "main" com.microsoft.playwright.TimeoutError: Error {
message='Timeout 3000ms exceeded.
It will only wait 3 seconds before throwing the TimeoutError exception.
position
It is used to hover over the specific position of an element.
We can set the x and y position relative to the top left corner of the element padding box, and the hover() method will move the mouse over that element’s position.
Let’s say we want to over the element at (1,1) position; then we can pass as such using the setPosition() method.

import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Locator.HoverOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
HoverOptions hoverOptions = new HoverOptions();
hoverOptions.setPosition(1, 1);
Locator buttonLocator = page.locator("#colorChangeOnHover");
buttonLocator.hover(hoverOptions); // hovering over the element
// closing the instances
browser.close();
playwright.close();
}
}
It will hover over the element at the (1,1) position.
Can we use the negative coordinates?
No, we cannot. We tried doing it, and hover() was not hovering over the element because (0,0) is treated as the top-left position of the element. So, we should only pass the positive coordinates.
We hope that you have liked the article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.