isEnabled() method in Playwright tells whether an element is enabled, and this post will discuss the isEnabled() method in detail.
According to the documentation, the element is considered enabled unless it’s a <button>
, <select>
, <input>
or <textarea>
with disabled
property.
There are multiple implementations of the isEnabled() method in Playwright.
Out of the above implementations, it is recommended to use the Locator implementation as per the playwright documentation. The Playwright altogether discourages the use of ElementHandle.
According to their documentation
The use of ElementHandle is discouraged, use Locator objects and web-first assertions instead.
– from Playwright
Let’s look at them one by one.
Page isEnabled() method
The Page in Playwright further has two overloaded implementations of the isEnabled() method.
- default boolean isEnabled(String selector)
- boolean isEnabled(String selector, IsEnabledOptions options)
default boolean isEnabled(String selector)
- Method declaration – default boolean isEnabled(String selector)
- What does it do? – It checks whether the element satisfying the selector ( passed in the argument ) is enabled.
- What does it return? – It returns true if the element is enabled; otherwise false.
Note: The first element will be used if multiple elements satisfy the selector.
Let’s choose one of our selenium playground website’s enabled buttons – https://testkru.com/Elements/Buttons.

Let’s inspect the highlighted element.
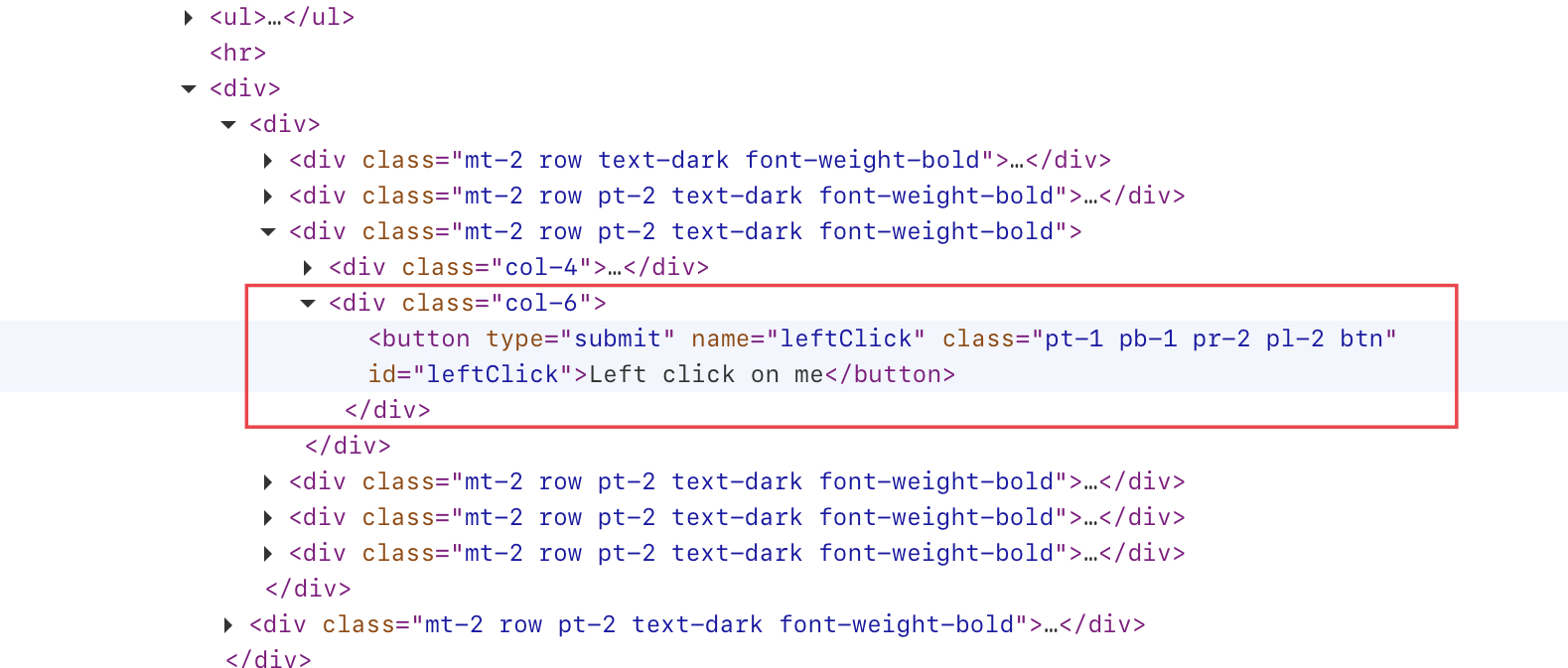
We can see that the id attribute’s value is “leftClick“, and we can pass it as a selector string and check whether the element is enabled or not.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
System.out.println("Is element enabled: " + page.isEnabled("#leftClick")); // isEnabled() method
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Is element enabled: true
What if the selector satisfies multiple elements?

In the above image, seven elements satisfy the selector, but only the first one will be picked.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
System.out.println("Is element enabled: " + page.isEnabled("//button[@type='submit']")); // isEnabled() method
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Is element enabled: true
What if we use the isEnabled() method on a disabled element?
Let’s pick one disabled element and use the isEnabled() method.
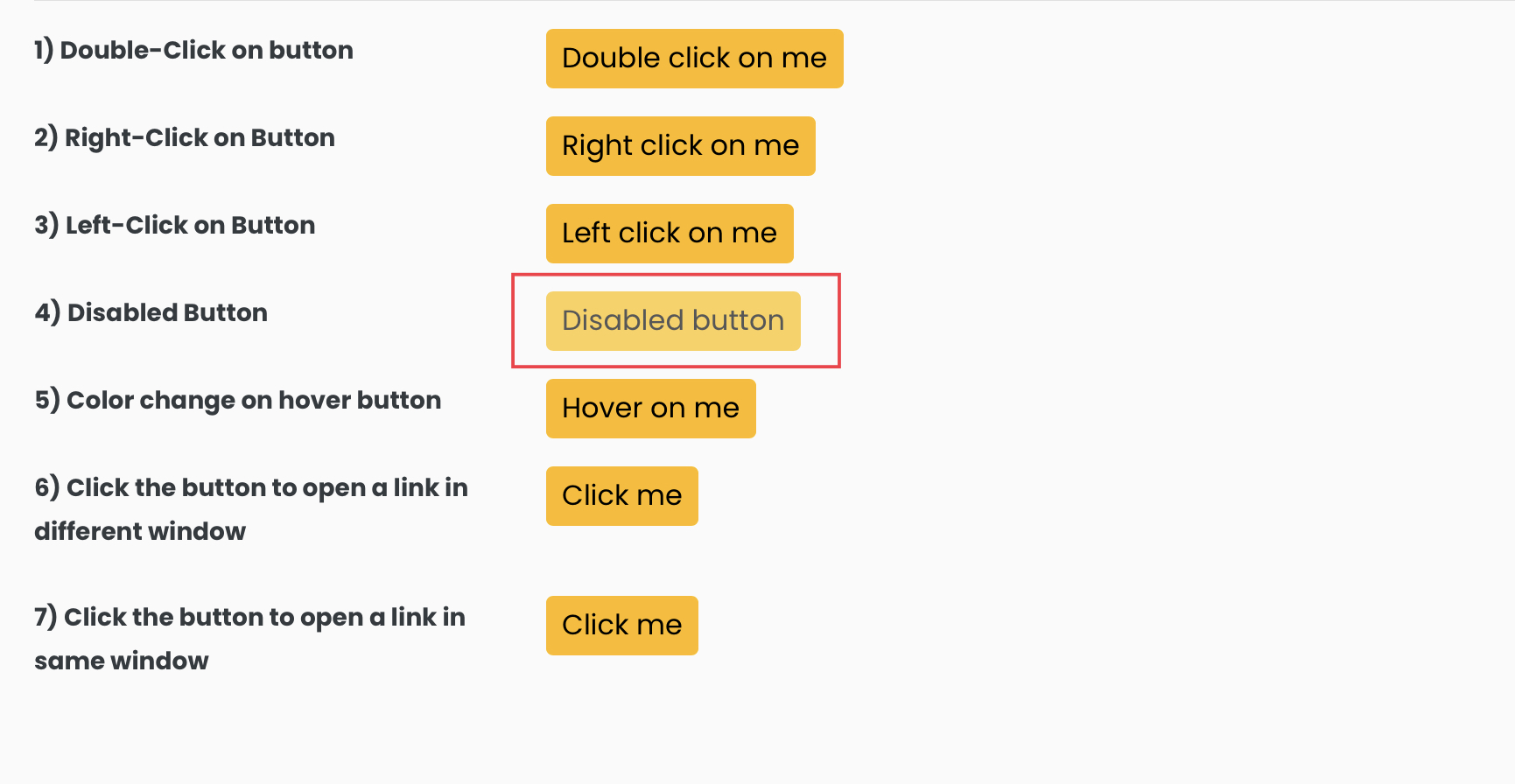
Let’s inspect the element and find a unique selector string for the element.
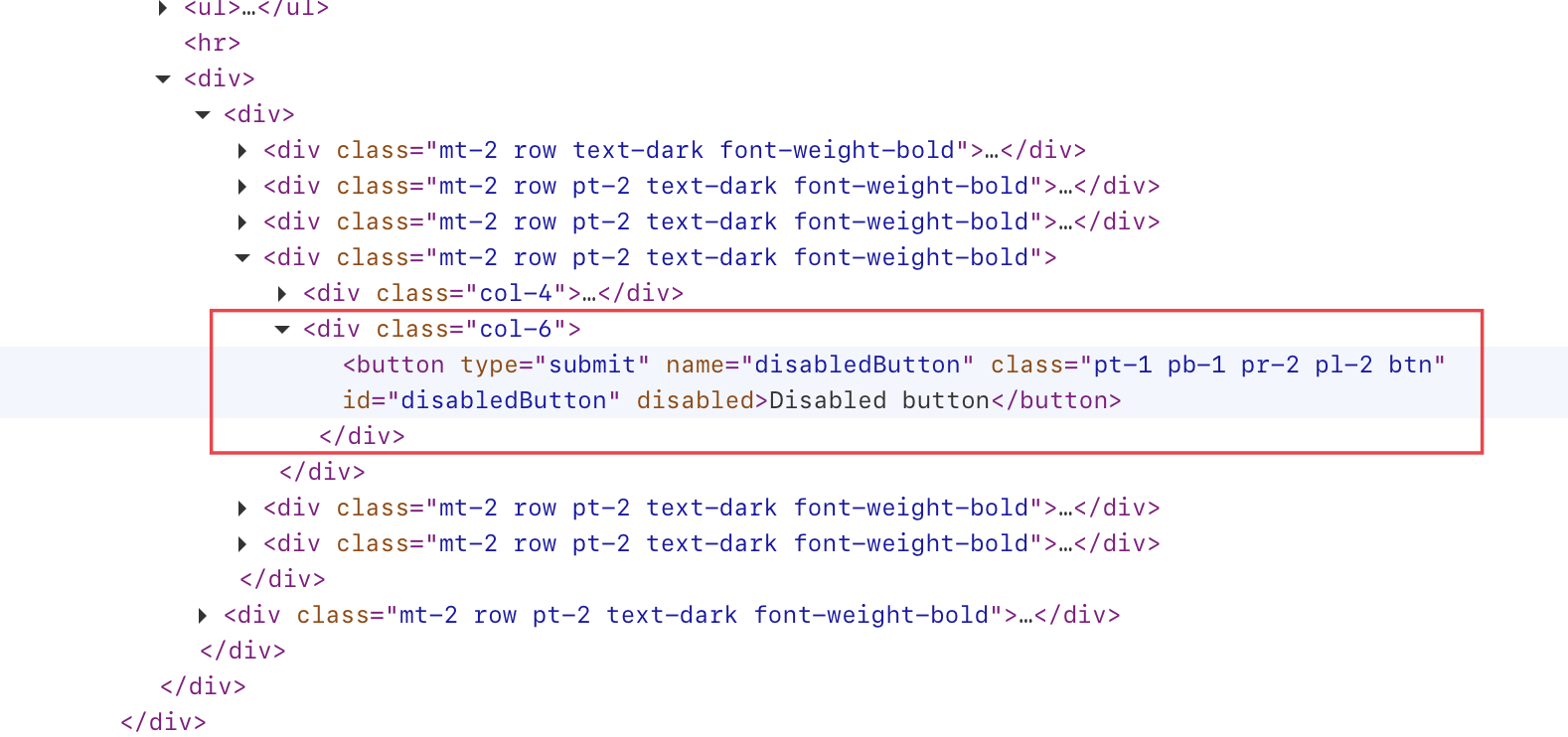
The id attribute’s value is “disabledButton“. We can use it as a selector with the isEnabled() method.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
System.out.println("Is element enabled: " + page.isEnabled("#disabledButton")); // isEnabled() method
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Is element enabled: false
boolean isEnabled(String selector, IsEnabledOptions options)
- Method declaration – boolean isEnabled(String selector, IsEnabledOptions options)
- What does it do? – It takes two arguments – one is the selector string, and another is the options.
- What does it return? – It returns true if the element is enabled; otherwise false.
IsEnabledOptions class provides two methods in Playwright which we can use with the isEnabled() method as per our requirements.
- public IsEnabledOptions setStrict(boolean strict)
- public IsEnabledOptions setTimeout(double timeout)
setStrict() comes into the picture when multiple elements satisfy a selector string. Earlier, we saw that isEnabled() will use the first matching element and discard the rest if multiple elements satisfy a selector string.
But what if you didn’t want it? You want an error to be thrown whenever this happens. Here we can use the setStrict() method and pass “true” as a boolean argument, and then it will throw an error whenever multiple elements match the selector string.
page.isEnabled("//button[@type='submit']",new IsEnabledOptions().setStrict(true))
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Page.IsEnabledOptions;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
// isEnabled() method with
// IsEnabledOptions()
System.out.println("Is element enabled: "
+ page.isEnabled("//button[@type='submit']", new IsEnabledOptions().setStrict(true)));
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Exception in thread "main" com.microsoft.playwright.PlaywrightException: Error {
message='Error: strict mode violation: locator("xpath=//button[@type='submit']") resolved to 7 elements:
We can see that it threw a PlaywrightException.
Locator isEnabled() method
The Locator also has two overloaded implementations of the isEnabled() method.
- default boolean isEnabled()
- boolean isEnabled(IsEnabledOptions options)
They work similarly to the Page implementation of the isEnabled() method. We don’t have to pass the selector string with the locator because we already have our specific element.
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
Locator buttonLocator = page.locator("#leftClick"); // locator
System.out.println("Is element enabled: " + buttonLocator.isEnabled());
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Is element enabled: true
But there is a difference when using IsEnabledOptions with the Locator class isEnabled() method.
com.microsoft.playwright.Locator.IsEnabledOptions
only has one method, unlike com.microsoft.playwright.Page.IsEnabledOptions
, which had two methods
So, IsEnabledOptions class only has the setTimeout() method and not the setStrict() method. And it also makes sense because the setStrict() method is used when multiple elements satisfy the selector string, but in the case of locators, there is no need for it. We use the isEnabled() method on a specific element.
ElementHandle isEnabled() method
ElementHandle only has one implementation of the isEnabled() method.
- Method declaration – boolean isEnabled()
- What does it do? – It checks whether the element is enabled or not.
- What does it return? – It returns true if the element is enabled; otherwise false.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.ElementHandle;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(); // Creating a "Browser" instance
Page page = browser.newContext().newPage(); // Creating a new page
page.navigate("https://testkru.com/Elements/Buttons");
ElementHandle elementHandle = page.querySelector("#leftClick");
System.out.println("Is element enabled: " + elementHandle.isEnabled());
// closing the instances
browser.close();
playwright.close();
}
}
Output –
Is element enabled: true
We hope that you have liked the article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.