The press() method offers an effective means of simulating key presses, allowing us to automate and test different scenarios within web applications. This article will delve into the press() method’s specifics.
press() method has two overloaded implementations –
Let’s look at both of them one by one.
default void press(String key)
- It will take a key as an argument and press it. While the type() method can handle multiple keys simultaneously, the press() method is limited to one key at a time. ( except when we are using modifier keys ).
- press() method also accepts the modifier keys like Meta, Alt, Shift etc.
- If we hold down the Shift key while typing, the letter corresponding to the key will appear in uppercase. For example,
press("Shift + a")
will result in the letter “A
“.
Syntax of using the Keyboard’s press() method
page.keyboard().press("key");
Code Example
Let’s try to press the “Enter” key. We can test it on our playground website to see which key is being pressed.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserContext;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
// Launching the browser
Browser browser = playwright.chromium().launch();
// creating a BrowserContext
BrowserContext browserContext = browser.newContext();
// creating the page
Page page = browserContext.newPage();
page.navigate("https://testkru.com/Interactions/KeyboardActions");
page.keyboard().press("Enter");
browser.close();
playwright.close();
}
}
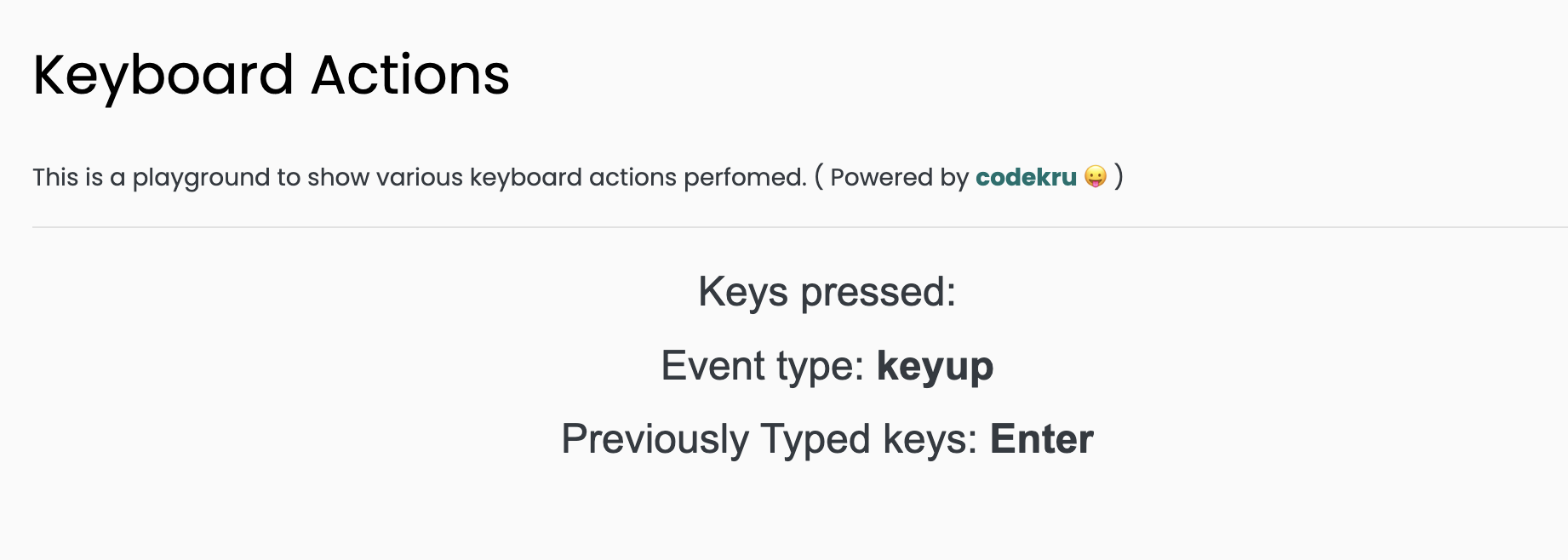
We can see from the above image that “Enter” was pressed.
What if we pass an unknown key to press() method?
We will get a PlaywrightException, as illustrated by the below example.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserContext;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
// Launching the browser
Browser browser = playwright.chromium().launch();
// creating a BrowserContext
BrowserContext browserContext = browser.newContext();
// creating the page
Page page = browserContext.newPage();
page.navigate("https://testkru.com/Interactions/KeyboardActions");
page.keyboard().press("ABC");
browser.close();
playwright.close();
}
}
Output –
Exception in thread "main" com.microsoft.playwright.PlaywrightException: Error {
message='Unknown key: "ABC"
name='Error
stack='Error: Unknown key: "ABC"
void press(String key, PressOptions options)
press() method also accepts PressOptions as another argument along with the key.
PressOptions help in enacting more realistic user behaviour by putting a delay between when a key is pressed and when it’s released. The time delay is passed in milliseconds.
PressOptions pressOptions = new PressOptions();
pressOptions.setDelay(2000); // setting a 2 sec delay
page.keyboard().press("Enter",pressOptions);
Now, let’s see the whole code.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserContext;
import com.microsoft.playwright.Keyboard.PressOptions;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
// Launching the browser
Browser browser = playwright.chromium().launch();
// creating a BrowserContext
BrowserContext browserContext = browser.newContext();
// creating the page
Page page = browserContext.newPage();
page.navigate("https://testkru.com/Interactions/KeyboardActions");
PressOptions pressOptions = new PressOptions();
pressOptions.setDelay(2000); // setting a 2 sec delay
page.keyboard().press("Enter", pressOptions);
browser.close();
playwright.close();
}
}
Above code will press Enter with a 2-second delay. This way, we can control the time it takes to press a key.
We hope that you have liked the article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.