Selenium is a popular open-source tool for automating web browsers. One of the key features of Selenium is its ability to interact with the browser’s developer console. This article will show you how to get console logs in Selenium using Java. These logs can be useful for debugging and troubleshooting issues with your Selenium scripts.
How to see the console logs of any webpage
The first step is to open the browser’s developer console.
You can do this in most browsers by right-clicking on the page and selecting “Inspect.” We will use our playground website – https://testkru.com/TestUrls/TestConsoleLogs.
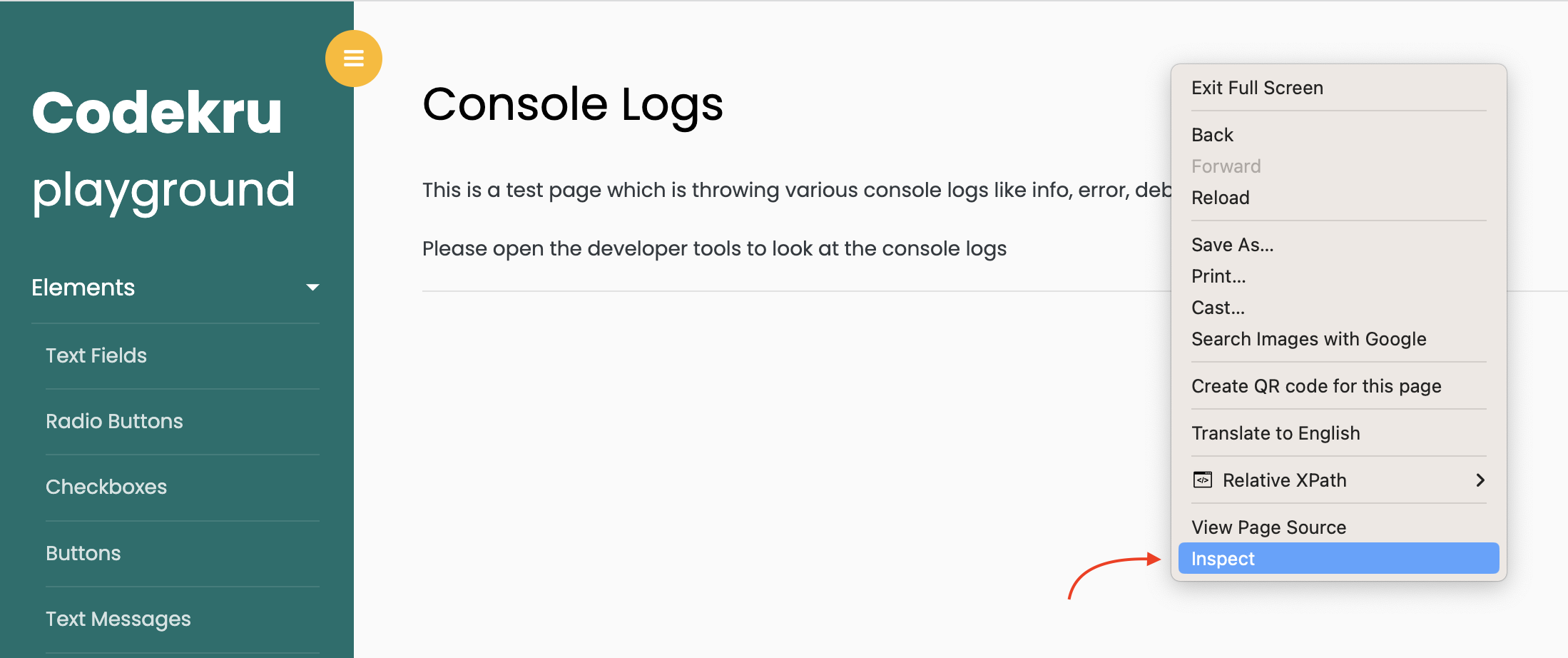
Once the console is open, you can access the logs by navigating to the “Console” tab.
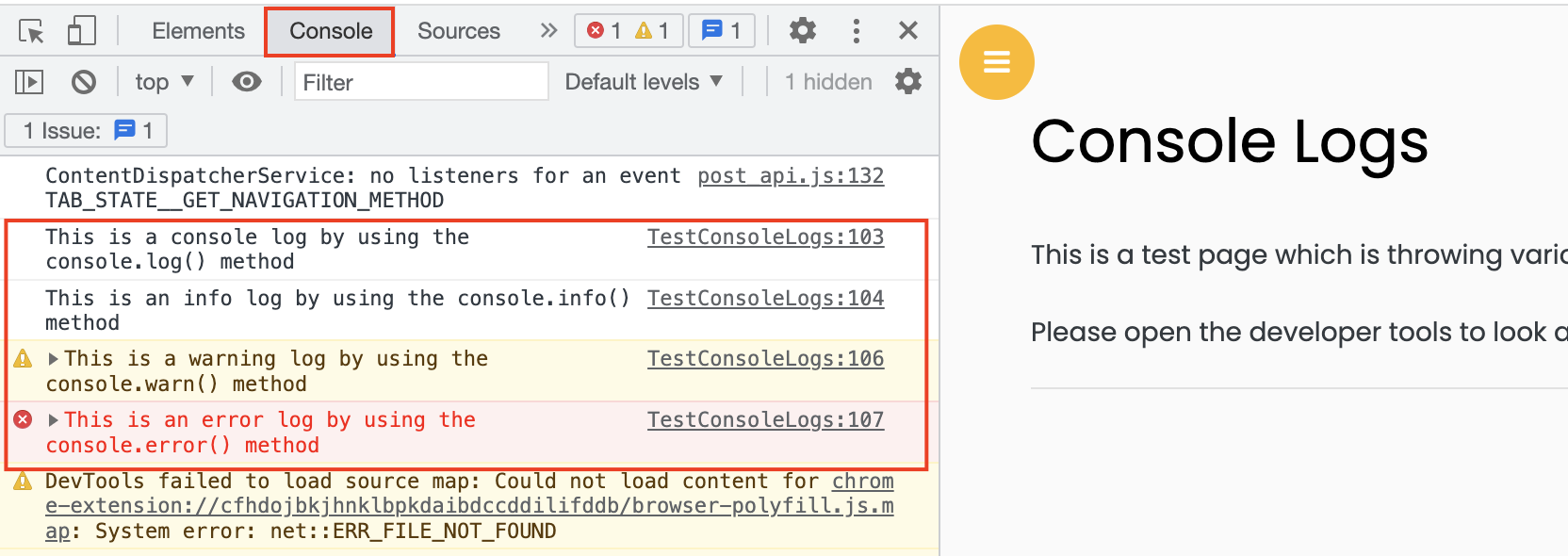
Let’s see how we can get these console logs using Selenium.
How to capture console logs in Selenium
We will discuss how we can capture the logs in Selenium 3 and Selenium 4.
Let’s look at them one by one.
Capture the console logs in Selenium 4
Note: We are using 4.4.0 version.
We can retrieve the logs in Selenium using driver.manage().logs().get(LogType.BROWSER)
code, which will return a LogEntries object with all console logs.
Enable the logging capability
Before capturing the logs, we will add the logging capability in the driver instance.
ChromeOptions options = new ChromeOptions();
LoggingPreferences logPrefs = new LoggingPreferences();
logPrefs.enable(LogType.BROWSER, Level.ALL);
options.setCapability(options.LOGGING_PREFS, logPrefs);
WebDriver driver = new ChromeDriver(options);
Then we can easily get the console logs using the getLog() method.
LogEntries entry = driver.manage().logs().get(LogType.BROWSER);
This will return a list of log entries, which we can then iterate through and print to the console.
// Retrieving all logs
List<LogEntry> logs = entry.getAll();
// Printing details separately
for (LogEntry e : logs) {
System.out.println("Message: " + e.getMessage());
System.out.println("Level: " + e.getLevel());
}
- getMessage() prints the messages that are being shown on the console.
- and getLevel() prints the level of the message. It can be INFO, WARNING, SEVERE, etc.
- We can also use the getTimestamp() to get the timestamp at which the message was printed on the browser console.
Full Code
import java.util.List;
import java.util.logging.Level;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.logging.LogEntries;
import org.openqa.selenium.logging.LogEntry;
import org.openqa.selenium.logging.LogType;
import org.openqa.selenium.logging.LoggingPreferences;
import org.testng.annotations.Test;
public class CodekruTest {
@Test
public void getLogs() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
ChromeOptions options = new ChromeOptions();
LoggingPreferences logPrefs = new LoggingPreferences();
logPrefs.enable(LogType.BROWSER, Level.ALL);
options.setCapability(options.LOGGING_PREFS, logPrefs);
WebDriver driver = new ChromeDriver(options);
driver.get("https://testkru.com/TestUrls/TestConsoleLogs");
LogEntries entry = driver.manage().logs().get(LogType.BROWSER);
// Retrieving all logs
List<LogEntry> logs = entry.getAll();
// Printing details separately
for (LogEntry e : logs) {
System.out.println("Message: " + e.getMessage());
System.out.println("Level: " + e.getLevel());
System.out.println("Timestamp: "+ e.getTimestamp());
}
}
}
Output –
Message: https://testkru.com/TestUrls/TestConsoleLogs 102:30 "This is a console log by using the console.log() method"
Level: INFO
Timestamp: 1673764474531
Message: https://testkru.com/TestUrls/TestConsoleLogs 103:30 "This is an info log by using the console.info() method"
Level: INFO
Timestamp: 1673764474531
Message: https://testkru.com/TestUrls/TestConsoleLogs 104:30 "This is a debug log by using the console.debug() method"
Level: FINE
Timestamp: 1673764474531
Message: https://testkru.com/TestUrls/TestConsoleLogs 105:29 "This is a warning log by using the console.warn() method"
Level: WARNING
Timestamp: 1673764474531
Message: https://testkru.com/TestUrls/TestConsoleLogs 106:29 "This is an error log by using the console.error() method"
Level: SEVERE
Timestamp: 1673764474531
What if we haven’t enabled the logging capability?
Let’s look at the below code, where we haven’t added logging preferences.
import java.util.List;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.logging.LogEntries;
import org.openqa.selenium.logging.LogEntry;
import org.openqa.selenium.logging.LogType;
import org.testng.annotations.Test;
public class CodekruTest {
@Test
public void getLogs() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("https://testkru.com/TestUrls/TestConsoleLogs");
LogEntries entry = driver.manage().logs().get(LogType.BROWSER);
// Retrieving all logs
List<LogEntry> logs = entry.getAll();
// Printing details separately
for (LogEntry e : logs) {
System.out.println("Message: " + e.getMessage());
System.out.println("Level: " + e.getLevel());
System.out.println("Timestamp: " + e.getTimestamp());
}
}
}
Output –
Message: https://testkru.com/TestUrls/TestConsoleLogs 105:29 "This is a warning log by using the console.warn() method"
Level: WARNING
Timestamp: 1673764653771
Message: https://testkru.com/TestUrls/TestConsoleLogs 106:29 "This is an error log by using the console.error() method"
Level: SEVERE
Timestamp: 1673764653771
We can see that it only printed the warning and severe logs. So, to get all logs, including info and debug, we should enable the logging preferences.
Capture the console logs in Selenium 3
We only have to change a bit of code on how we enable logging preferences, and then the code we wrote above will work for Selenium 3 too.
ChromeOptions options = new ChromeOptions();
LoggingPreferences logPrefs = new LoggingPreferences();
logPrefs.enable(LogType.BROWSER, Level.ALL);
options.setCapability("goog:loggingPrefs", logPrefs);
WebDriver driver = new ChromeDriver(options);
Full Code with Selenium 3
import java.util.List;
import java.util.logging.Level;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.logging.LogEntries;
import org.openqa.selenium.logging.LogEntry;
import org.openqa.selenium.logging.LogType;
import org.openqa.selenium.logging.LoggingPreferences;
import org.testng.annotations.Test;
public class CodekruTest {
@Test
public void getLogs() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
ChromeOptions options = new ChromeOptions();
LoggingPreferences logPrefs = new LoggingPreferences();
logPrefs.enable(LogType.BROWSER, Level.ALL);
options.setCapability("goog:loggingPrefs", logPrefs);
WebDriver driver = new ChromeDriver(options);
driver.get("https://testkru.com/TestUrls/TestConsoleLogs");
LogEntries entry = driver.manage().logs().get(LogType.BROWSER);
// Retrieving all logs
List<LogEntry> logs = entry.getAll();
// Printing details separately
for (LogEntry e : logs) {
System.out.println("Message: " + e.getMessage());
System.out.println("Level: " + e.getLevel());
System.out.println("Timestamp: " + e.getTimestamp());
}
}
}
Output –
Message: https://testkru.com/TestUrls/TestConsoleLogs 102:30 "This is a console log by using the console.log() method"
Level: INFO
Timestamp: 1673764474531
Message: https://testkru.com/TestUrls/TestConsoleLogs 103:30 "This is an info log by using the console.info() method"
Level: INFO
Timestamp: 1673764474531
Message: https://testkru.com/TestUrls/TestConsoleLogs 104:30 "This is a debug log by using the console.debug() method"
Level: FINE
Timestamp: 1673764474531
Message: https://testkru.com/TestUrls/TestConsoleLogs 105:29 "This is a warning log by using the console.warn() method"
Level: WARNING
Timestamp: 1673764474531
Message: https://testkru.com/TestUrls/TestConsoleLogs 106:29 "This is an error log by using the console.error() method"
Level: SEVERE
Timestamp: 1673764474531
This is it. We hope that you have liked the article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.