There might be scenarios where we might have to verify whether a certain is disabled or not or is becoming disabled after performing a certain operation. This post will discuss how we can check whether an element is disabled.
First, we should also know what makes a field disabled.
The “disabled“ attribute, when used on an element, will make it disabled.
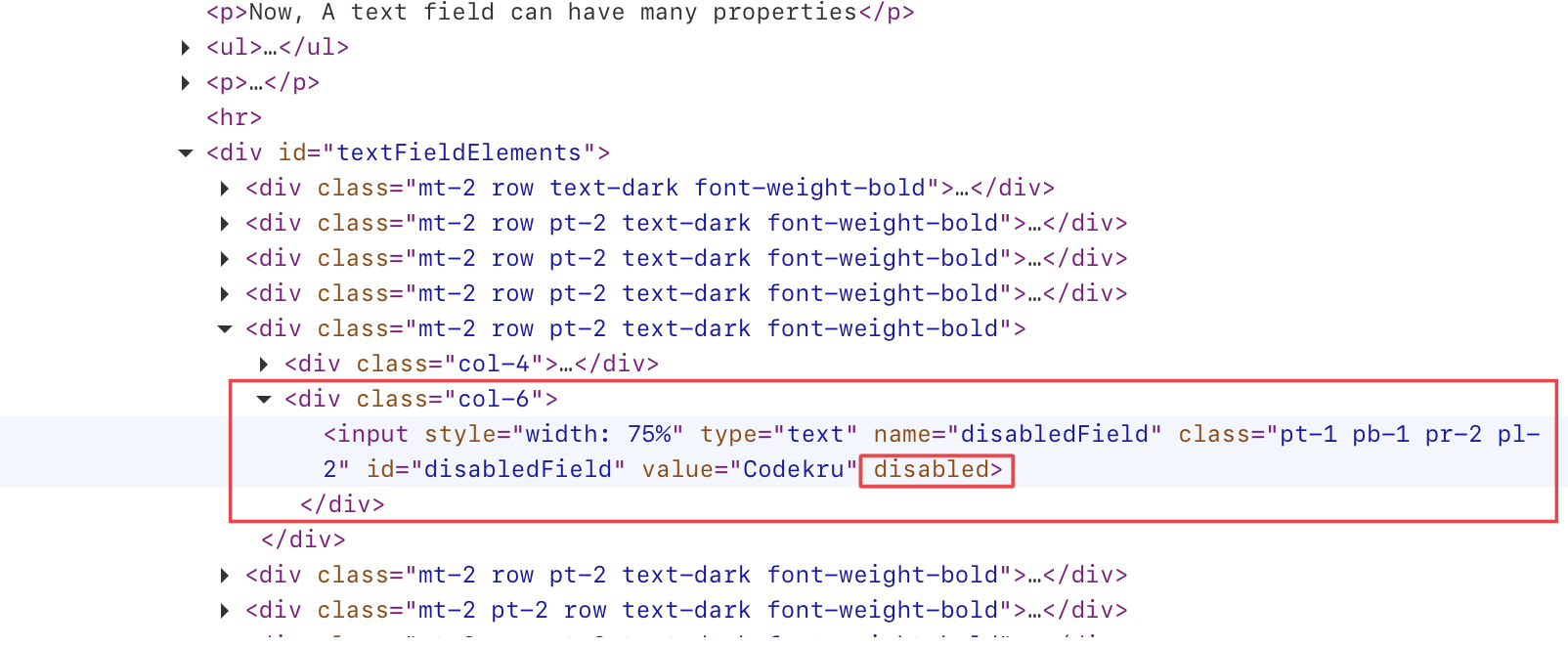
Below are ways that can be used to check whether an element is disabled or not –
- Using isEnabled() method
- Using getAttribute() method
- And lastly, using the JavascriptExecutor
Let’s look at each of them one by one.
Using isEnabled() method
isEnabled() method of the WebElement interface returns true if the element is enabled and false if it’s disabled. We will use this on one disabled text field on our selenium playground website – https://testkru.com/Elements/TextFields.
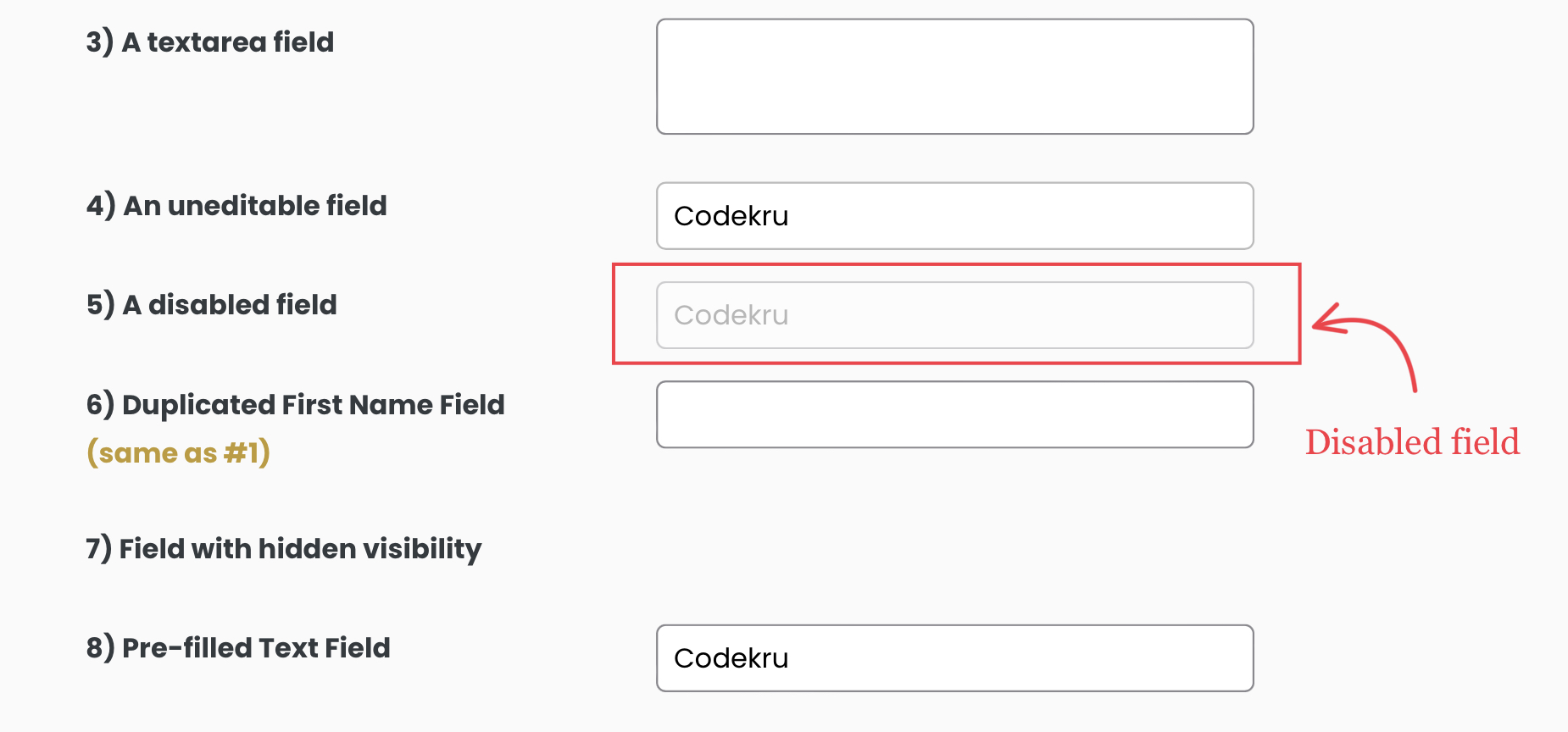
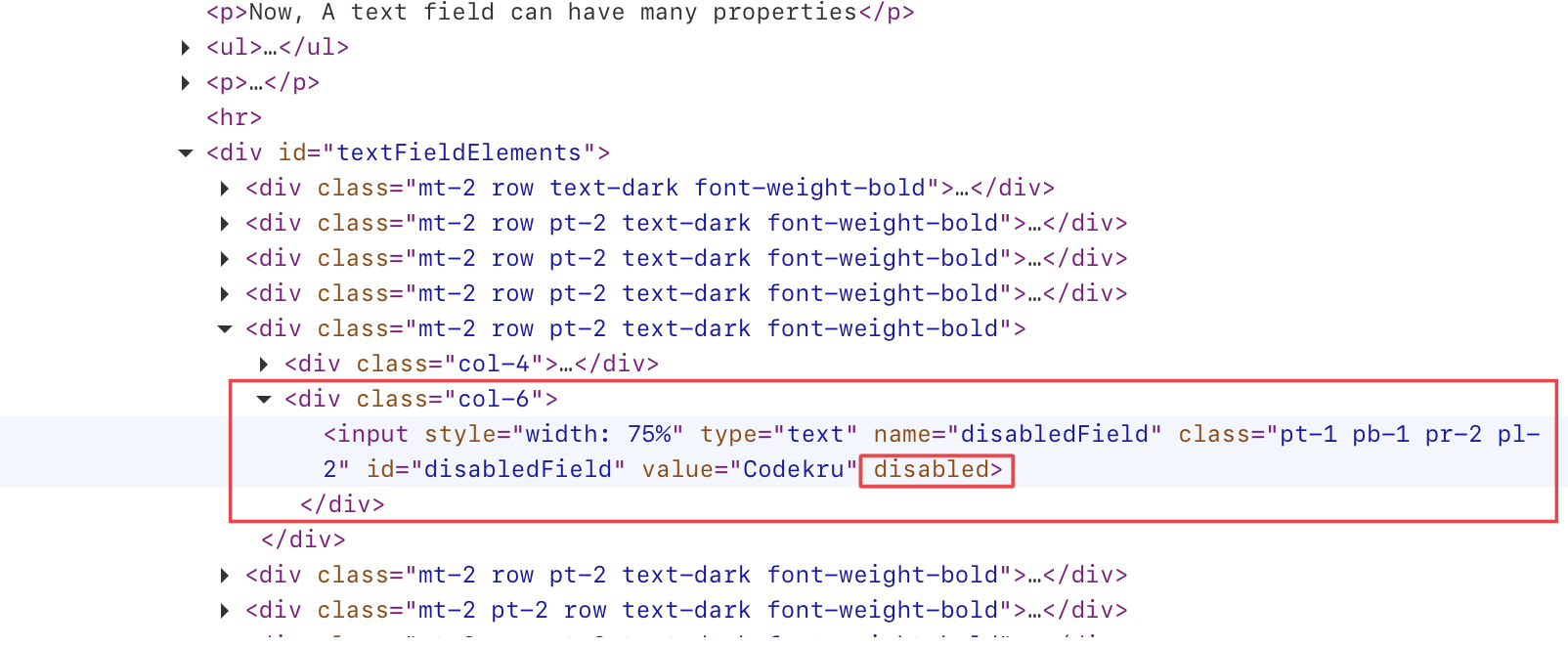
The highlighted element is a disabled text field with the id as “disabledFiled“. We will use the id attribute to find the element using the findElements() method, and then we will use the isEnabled() method on the element to check whether the field is disabled.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("disabledField"));
System.out.println("Is Element enabled : " + element.isEnabled());
}
}
Output –
Is Element enabled : false
We can see that isEnabled() returned false. This is because the element was disabled.
Let’s use the getAttribute() method to see if an element is disabled.
Using getAttribute() method
getAttribute() method is used to get the attribute’s value of an element. Here, we will use it to get the value of the “disabled” attribute. It will return true if the attribute is present on the element; otherwise, it will return false.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("disabledField"));
System.out.println("Is Element disabled : " + element.getAttribute("disabled"));
}
}
Output –
Is Element disabled : true
We can also get the attribute value using the JavascriptExecutor in Selenium.
Using JavascriptExecutor
JavascriptExecutor in selenium helps in executing the Javascript code. So, we will write a Javascript code to get the “disabled” attribute value and execute that code using the JavascriptExecutor.
Below is the script we will execute via JavascriptExecutor.
return arguments[0].disabled
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
JavascriptExecutor jse = (JavascriptExecutor) driver;
WebElement element = driver.findElement(By.id("disabledField"));
System.out.println("Is Element disabled : " + jse.executeScript("return arguments[0].disabled", element));
}
}
Output –
Is Element disabled : true
We hope that you have liked this article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.