The isEnabled() method is used to tell whether an element is enabled or not. This post will discuss the isEnabled() method of the WebElement interface in detail.
- Method declaration – boolean isEnabled()
- What does it do? It will tell whether the element is enabled or not. So, if you want to find out whether an element is disabled or not, then the isEnabled() method can help here.
- What does it return? It will return true if an element is enabled. Otherwise, it will return false. This will generally return true for everything except disabled input elements.
Code Example
Let’s take the example of a disabled button, and we will use the isEnabled() method. The disabled button can be found on this page.
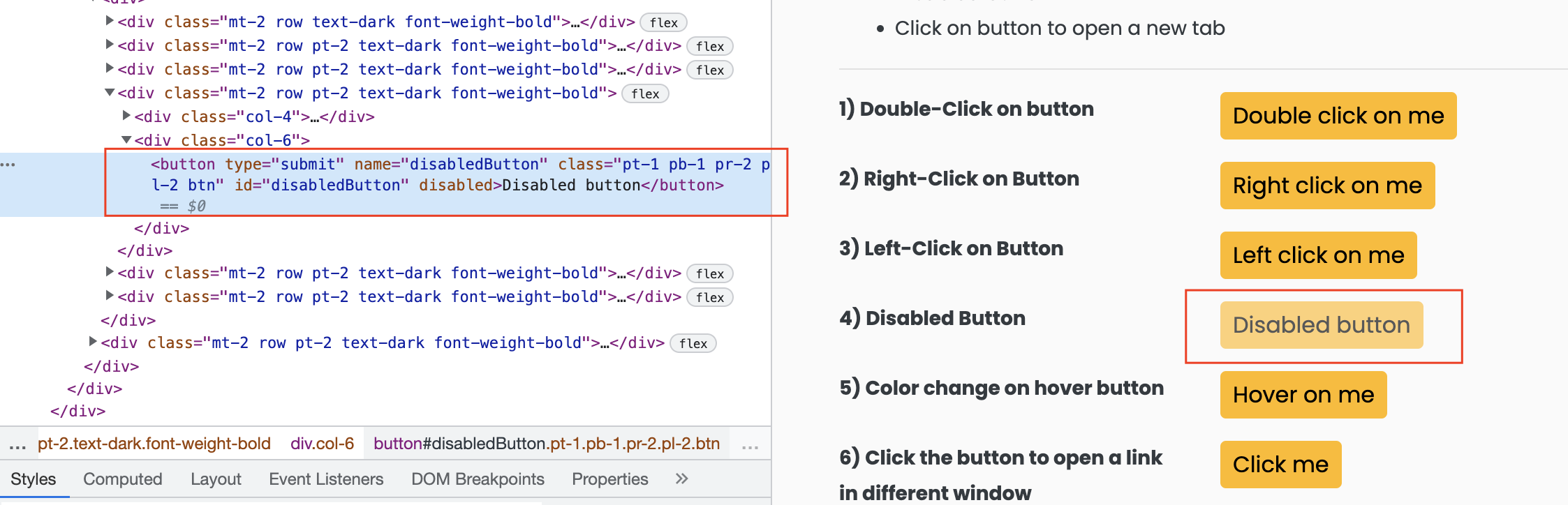
- The disabled button element id is “disabledButton“.
- We can use the above id to find the element using the findElement() method.
- And then, we can use the isEnabled() method to check whether the element is enabled or not.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement element = driver.findElement(By.id("disabledButton"));
System.out.println("Is element enabled on webpage: " + element.isEnabled());
}
}
Output –
Is element enabled on webpage: false
We can see here the method returned false because the button was disabled.
We will now try the same on an enabled button. We can pick any of the buttons on the same page. So, let’s pick the first button on the page.
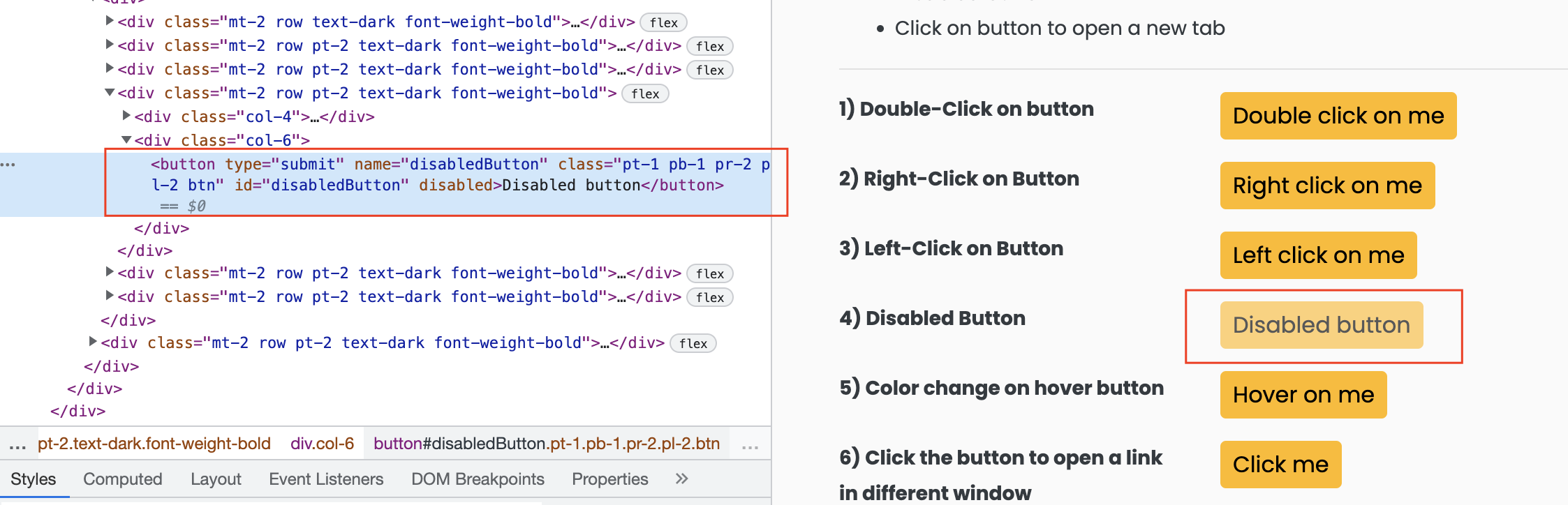
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement element = driver.findElement(By.id("doubleClick"));
System.out.println("Is element enabled on webpage: " + element.isEnabled());
}
}
Output –
Is element enabled on webpage: true
Here, the function returned true as the button was enabled.
Using the isEnabled() method after an element changes its state
Let’s pick an element that was enabled initially but became disabled after performing some operations. We will use the isEnabled() method before and after performing the operations to see if it represents the element’s current state.
We can use the 3rd button on this page. This button will become disabled after we perform the click operation on the button.

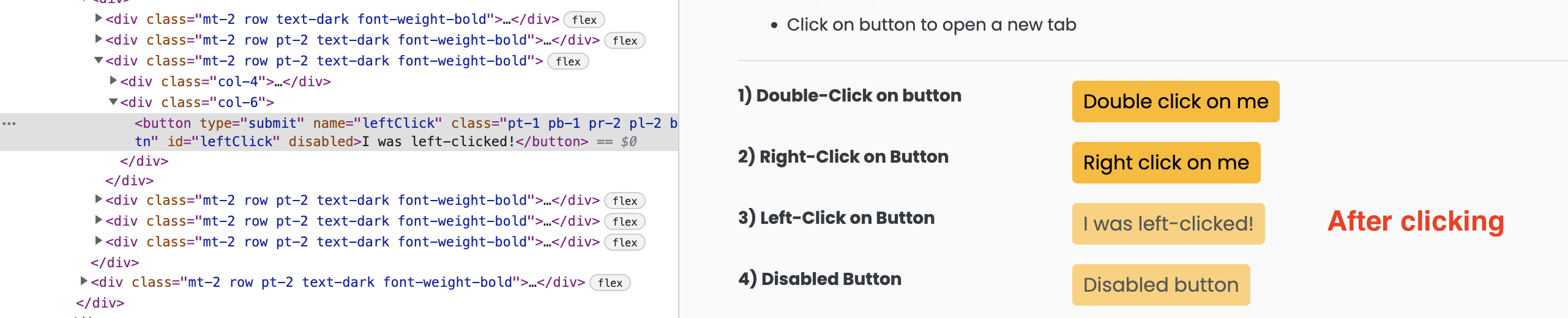
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement element = driver.findElement(By.id("leftClick"));
System.out.println("Is element enabled before clicking: " + element.isEnabled());
// click on the web element
element.click();
System.out.println("Is element enabled after clicking: " + element.isEnabled());
}
}
Output –
Is element enabled before clicking: true
Is element enabled after clicking: false
So, the isEnabled() method also changes its value if the element changes its state.
What if we use the isEnabled() method on a null element?
It will throw a NullPointerException as illustrated by the below example.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement element = null;
System.out.println("Is element enabled after clicking: " + element.isEnabled());
}
}
Output –
java.lang.NullPointerException: Cannot invoke "org.openqa.selenium.WebElement.isEnabled()" because "element" is null
This is it. Please visit this link to learn more about WebElement and its methods.
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.