isDisplayed() method is used to determine whether an element is visible. This post will discuss the isDisplayed() method of the WebElement interface in detail.
- Method declaration – boolean isDisplayed()
- What does it do? This method is used to tell whether an element is displayed or not. This method saves us the time of parsing the style attribute to deduce whether the element is hidden.
- What will it return? isDisplayed() method will return true if the element is visible on the webpage. Otherwise, it will return false.
Code Example
Let’s take an example of a visible text first.
Below highlighted element is a visible element. This element is present on our selenium playground website. We will use the isDisplayed() method on this element and see what happens.
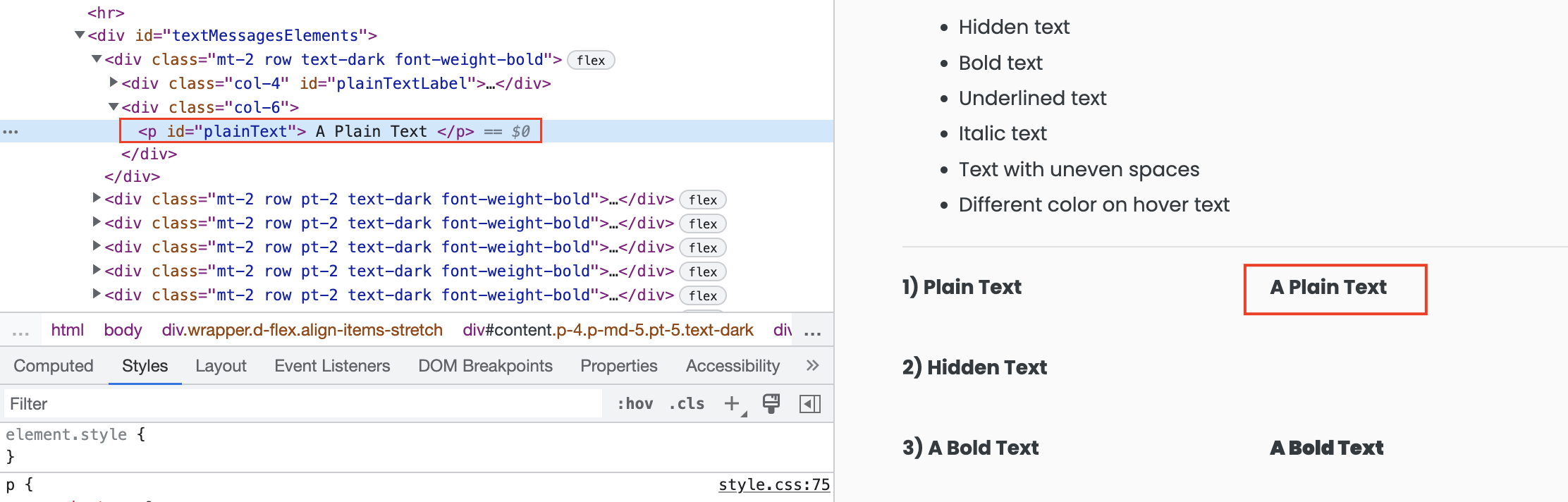
- We will use the findElement() method to find the element.
- And after finding the element, we can use the isDisplayed() method.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextMessages");
WebElement element = driver.findElement(By.id("plainText"));
System.out.println("Is element visible on webpage: " + element.isDisplayed());
}
}
Output –
Is element visible on webpage: true
Now, we will look at a hidden element
We can find the hidden element again on the same webpage ( our beloved playground website 😉). We have highlighted the hidden element in the below image.

Let’s use the isDisplayed() method on the hidden element now.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextMessages");
WebElement element = driver.findElement(By.id("hiddenText"));
System.out.println("Is element visible on webpage: " + element.isDisplayed());
}
}
Output –
Is element visible on webpage: false
What if we use the isDisplayed() method on a null element?
We will get a NullPointerException, as illustrated by the below example.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "C:\\Users\\MEHUL\\OneDrive\\Desktop\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextMessages");
WebElement element = null;
System.out.println("Is element visible on webpage: " + element.isDisplayed());
}
}
Output –
java.lang.NullPointerException: Cannot invoke "org.openqa.selenium.WebElement.isDisplayed()" because "element" is null
This is it. Please visit this link to learn more about WebElement and its methods.
We hope that you have liked the article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.