Iframes, also known as inline frames, embed another document within the current HTML document. Iframes are mostly used to embed third-party content into the HTML, and we might come across a scenario where we have to automate the content contained within an iframe. An iframe is represented by a <iframe> tag.
While we automate iframes, one cannot directly locate elements within an iframe. We have to first switch to an iframe, or we can say, go inside an iframe, and only then will we be able to locate and automate the elements.
There are various methods available to switch to an iframe.
- Switch to an iframe using index
- Switch to an iframe using an id or frame name
- And switch to an iframe using a web element
Let’s discuss all of them one by one. We will use our selenium playground website to switch to iframes – https://testkru.com/Interactions/Frames.
We will also look at how to switch to nested frames as well.
Switch to an iframe using index
We can switch to an iframe using the index, and the indexing starts from zero ( 0 ). We can use the below code to switch to an iframe using an index.
driver.switchTo().frame(index_number);
Indexing numbering starts from the top to bottom, where top most iframe has an index of 0.

Let’s see this with an example. We will use the indexing to switch to the first frame on this page.
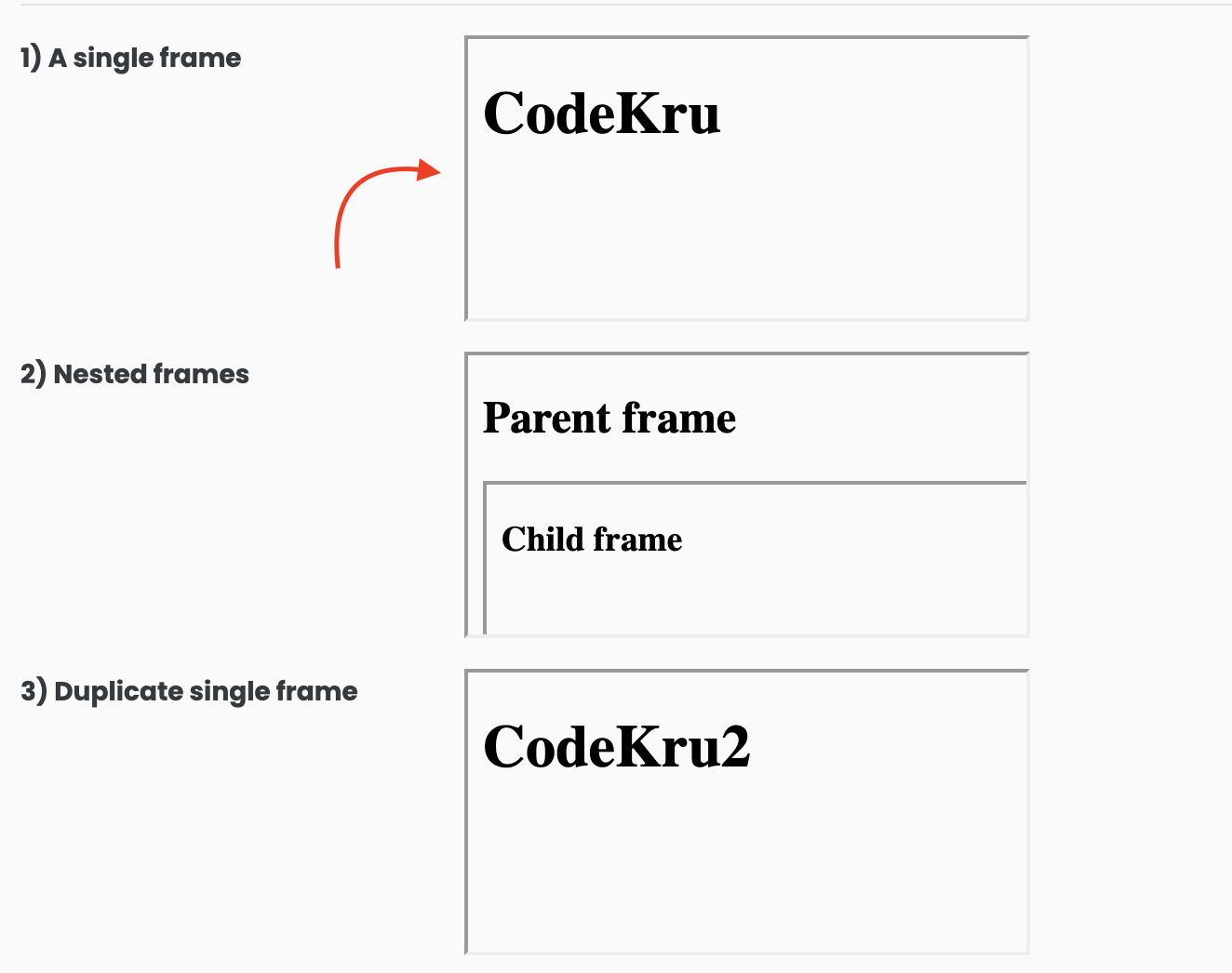

import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class CodekruTest {
public static void main(String[] args) throws InterruptedException {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("https://testkru.com/Interactions/Frames");
// switching to first frame of the webpage
driver.switchTo().frame(0);
WebElement webElement = driver.findElement(By.tagName("h1"));
System.out.println(webElement.getText());
}
}
Output –
CodeKru
We can see that it printed “CodeKru“, a text inside the first frame of the webpage. Switching to a frame using an index is an easy way of switching, but it may happen that a new frame has been introduced in the webpage, due to which the index of frames is changed, and the cases are failing.
We can switch to a frame using an id or the frame name to avoid this. Let’s have a look at that.
Switch to an iframe using id or name
Syntax of switching to a frame using id or name
driver.switchTo().frame(name_or_id)
We will again switch to the first frame using the id and name.

We can see that the id of the frame is “frame1“, and the name is “singleFrame“.
Switching to a frame using id
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class CodekruTest {
public static void main(String[] args) throws InterruptedException {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("https://testkru.com/Interactions/Frames");
// switching to frame
driver.switchTo().frame("frame1");
WebElement webElement = driver.findElement(By.tagName("h1"));
System.out.println(webElement.getText());
}
}
Output –
CodeKru
Switching to a frame using name
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class CodekruTest {
public static void main(String[] args) throws InterruptedException {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("https://testkru.com/Interactions/Frames");
// switching to frame
driver.switchTo().frame("singleFrame");
WebElement webElement = driver.findElement(By.tagName("h1"));
System.out.println(webElement.getText());
}
}
Output –
CodeKru
What if a frame does have the id or the name attribute associated with it? How will we switch to a frame?
Don’t worry, then we can use the web element to switch to an iframe.
Switch to an iframe using a web element
We can first locate the frame element and then switch to it.
driver.switchTo().frame(frame_webelement)
We can locate an element using the findElement() method.
WebElement frameElement = driver.findElement(By.xpath("//iframe[@class='frame']"));
And then, we switch to it using the below code.
driver.switchTo().frame(frameElement);
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class CodekruTest {
public static void main(String[] args) throws InterruptedException {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("https://testkru.com/Interactions/Frames");
WebElement frameElement = driver.findElement(By.xpath("//iframe[@class='frame']"));
// switching to frame using web element
driver.switchTo().frame(frameElement);
WebElement webElement = driver.findElement(By.tagName("h1"));
System.out.println(webElement.getText());
}
}
Output –
CodeKru
Switching In Nested Frames
We can also switch to nested frames using the methods we discussed earlier.
Let’s take a nested frame on our selenium playground website and will try to switch to a child frame.


- We will first switch to the parent frame using the id (“frame2”)
- And then, as there is only a single frame inside the parent frame, we can switch to it using the frame index.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class CodekruTest {
public static void main(String[] args) throws InterruptedException {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("https://testkru.com/Interactions/Frames");
// switching to parent frame
driver.switchTo().frame("frame2");
WebElement parentElement = driver.findElement(By.tagName("h2"));
System.out.println(parentElement.getText());
// switching to child frame
driver.switchTo().frame(0);
WebElement childElement = driver.findElement(By.tagName("h3"));
System.out.println(childElement.getText());
}
}
Output –
Parent frame
Child frame
We hope that you have liked the article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.