Playwright is a powerful Java library for automating web browser interactions and performing various actions on web pages. One common action is double-clicking on a button.
We can double-click on a button using a couple of ways –
- Using dbClick method
- and by using the evaluate method
We will use our selenium playground website to double-click on the below-highlighted button. The button is present on the page – https://testkru.com/Elements/Buttons.
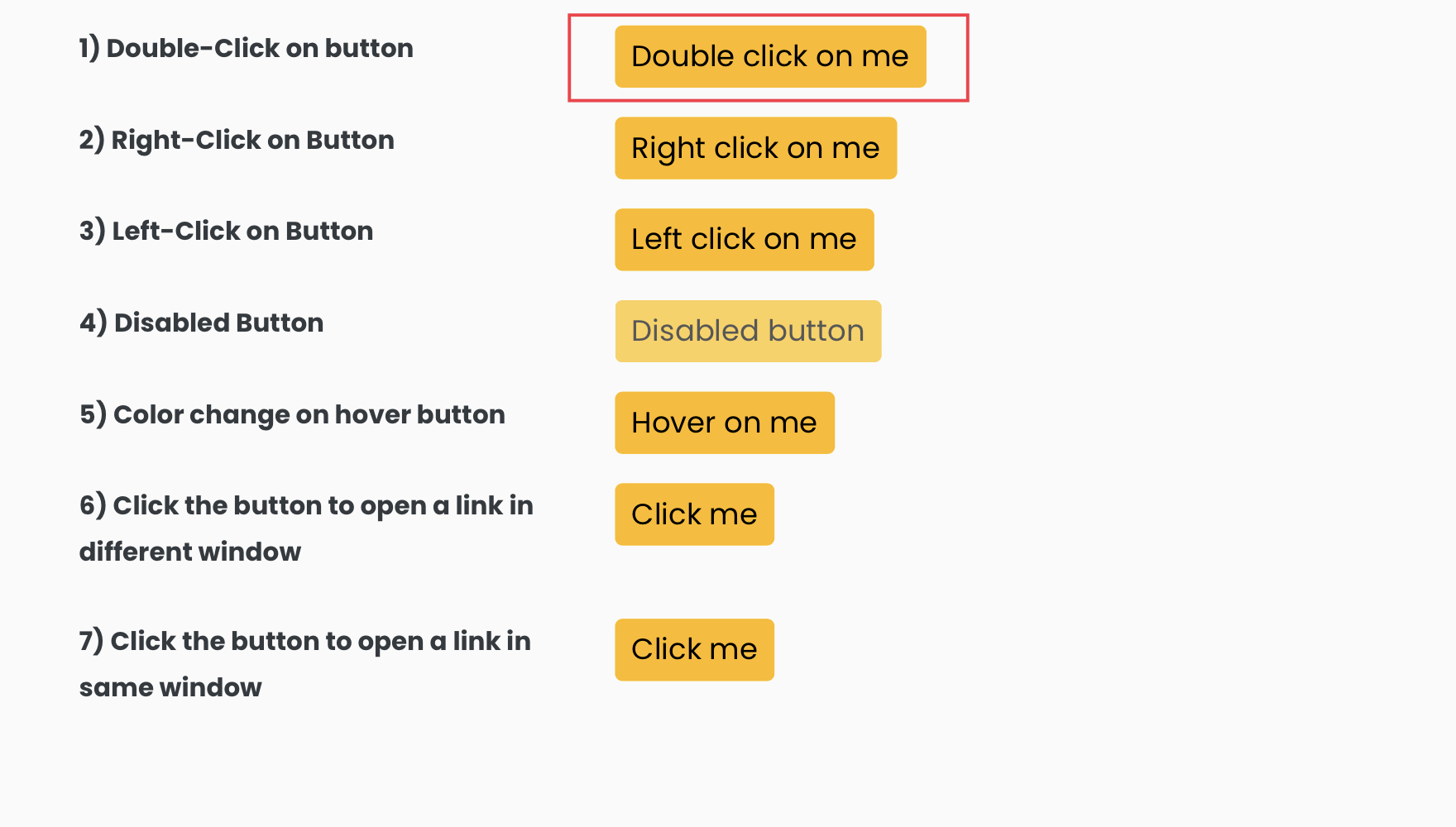
Using dbClick method
Playwright provides a dbClick() method to double-click on a specific element.
We will locate the highlighted element and double-click it using the dbClick() method.
So, let’s inspect the highlighted element to find the locator we can use.
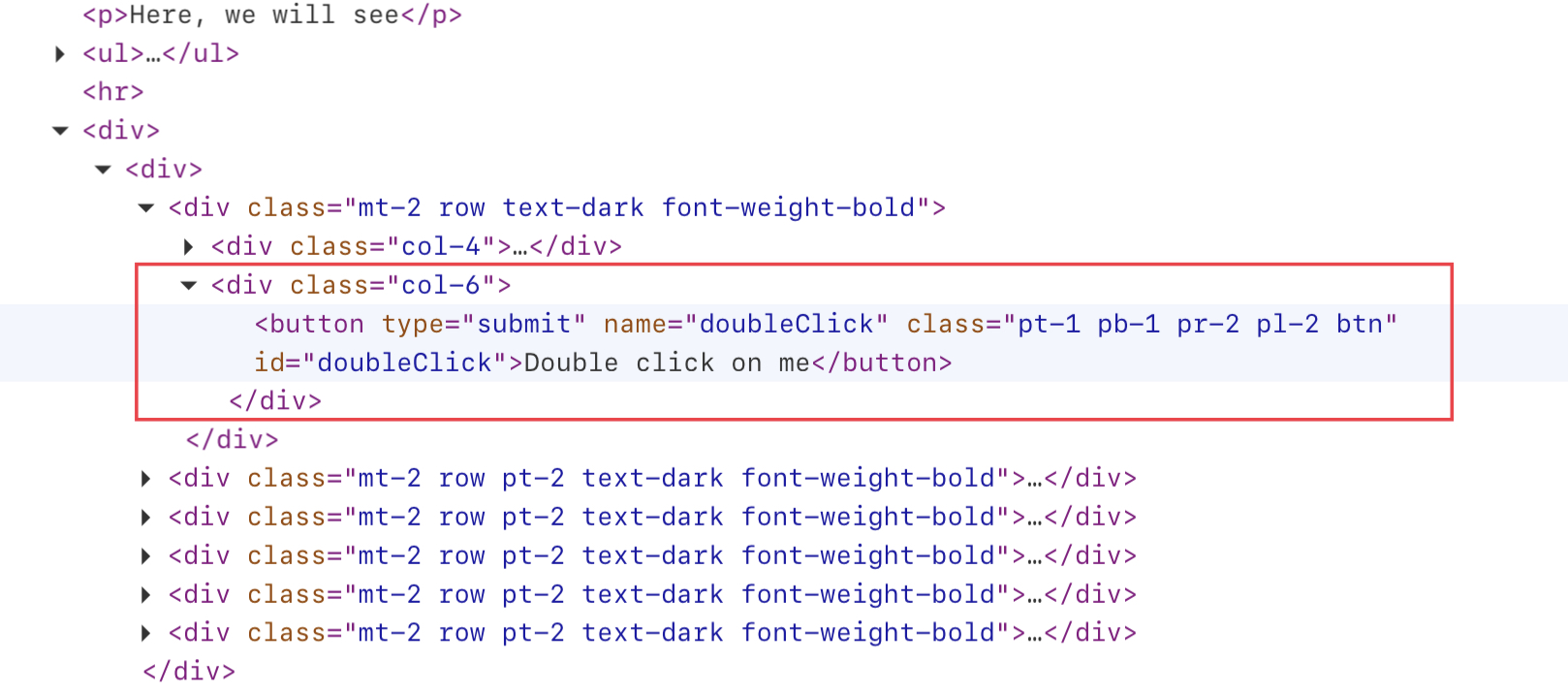
We can see that the id attribute’s value is doubleClick. We can write an XPath using it and locate the element.
//button[@id='doubleClick']
locator() helps us locate the element in the playwright framework. We will use it to locate our element.
Locator buttonLocator = page.locator("//button[@id='doubleClick']");
Now, use the dbClick() method to double-click on the element.
buttonLocator.dblclick(); // double clicking on the element
Let’s see the whole program and the things in action now.
import com.microsoft.playwright.Browser;
import com.microsoft.playwright.BrowserType;
import com.microsoft.playwright.Locator;
import com.microsoft.playwright.Page;
import com.microsoft.playwright.Playwright;
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(new BrowserType.LaunchOptions().setHeadless(false));
Page page = browser.newContext().newPage();
page.navigate("https://testkru.com/Elements/Buttons");
Locator buttonLocator = page.locator("//button[@id='doubleClick']");
System.out.println("Text before double click: " + buttonLocator.textContent());
buttonLocator.dblclick(); // double clicking on the element
System.out.println("Text after double click: " + buttonLocator.textContent());
browser.close();
playwright.close();
}
}
Output –
Text before double click: Double click on me
Text after double click: I was double-clicked!

evaluate() method
We can also double-click the button using javascript and then execute it using the evaluate() method provided by the playwright.
Below Javascript code can help us double-click on the highlighted element –
var button = document.getElementById("doubleClick");
var clickEvent = document.createEvent ('MouseEvents');
clickEvent.initEvent ('dblclick', true, true);
button.dispatchEvent (clickEvent);
The above script gets the element using the id attribute’s value and then double-clicks on it. Now, we will execute it using the evaluate() method.
String javascript = "var button = document.getElementById(\"doubleClick\");\r\n"
+ "var clickEvent = document.createEvent ('MouseEvents');\r\n"
+ "clickEvent.initEvent ('dblclick', true, true);\r\n"
+ "button.dispatchEvent (clickEvent);";
page.evaluate(javascript); // double clicking on the element
Let’s see the whole code in action.
public class CodekruTest {
public static void main(String[] args) {
Playwright playwright = Playwright.create();
Browser browser = playwright.chromium().launch(new BrowserType.LaunchOptions().setHeadless(false));
Page page = browser.newContext().newPage();
page.navigate("https://testkru.com/Elements/Buttons");
String javascript = "var button = document.getElementById(\"doubleClick\");\r\n"
+ "var clickEvent = document.createEvent ('MouseEvents');\r\n"
+ "clickEvent.initEvent ('dblclick', true, true);\r\n"
+ "button.dispatchEvent (clickEvent);";
Locator buttonLocator = page.locator("//button[@id='doubleClick']");
System.out.println("Text before double click: " + buttonLocator.textContent());
page.evaluate(javascript); // double clicking on the element
System.out.println("Text after double click: " + buttonLocator.textContent());
browser.close();
playwright.close();
}
}
Output –
Text before double click: Double click on me
Text after double click: I was double-clicked!
This is it. We hope that you have liked the article. If you have any doubts or concerns, please write us in the comments or mail us at admin@codekru.com.