This post will discuss the clear() method of the WebElement interface of Selenium in Java.
- Method declaration – void clear()
- What does it do? It will clear the value of the text entry element.
What is a text entry element? Text entry elements are INPUT and TEXTAREA elements. Any other element like a button, link, etc., is not considered a text entry element.
It means only elements having <input> or <textarea> tags will be able to use the clear() method. The clear() method will not have any impact on other elements.
How to use the clear() method on an element?
We can quickly call the clear() method on the reference of the WebElement interface.
ele.clear();
And this will clear the text of the text entry element if present.
Code Example
We will use our testkru website, where we will try to clear one of the text fields.

Now, we will have to find the web element for the Pre-filled text input box. As this element has an id, so will use the By.id() locator to find the element denoting the input box.
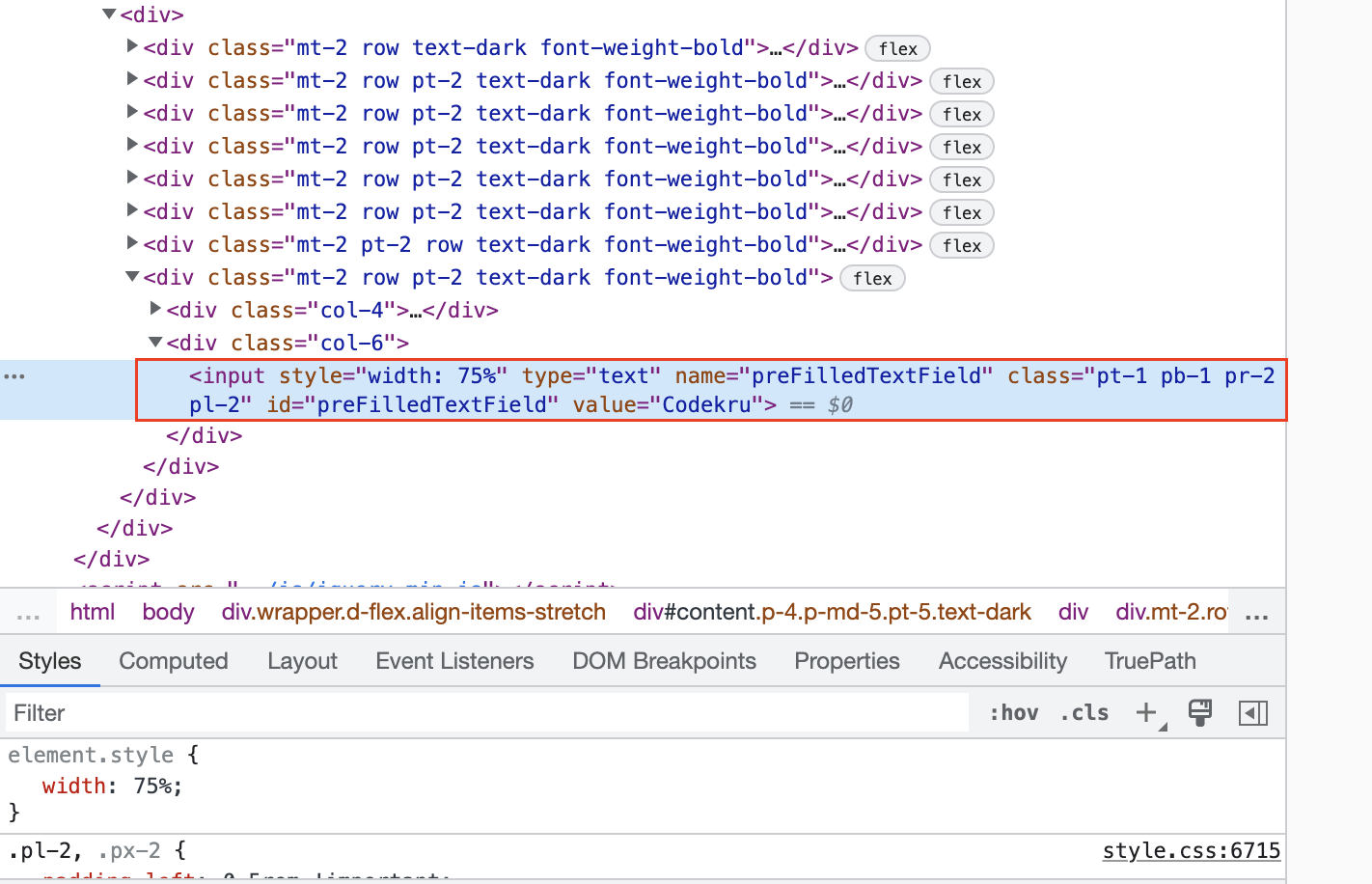
Now, we can use the below line to get the web element associated with the pre-filled input box.
WebElement ele = driver.findElement(By.id("preFilledTextField"));
Here, preFilledTextField is the id of the Pre-filled input text box.
We can use ele.clear() to clear the above element’s value.
The whole program will be like as shown below.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
// getting WebElement associated with the id
WebElement ele = driver.findElement(By.id("preFilledTextField"));
// clear the value present at the element
ele.clear();
}
}
The above program will successfully clear the value present in the input box.
The What If scenarios
Q – What if we use the clear() method on a non-editable text field?
We will get an InvalidElementStateException as we are trying to clear a non-editable field.
There is one input box on the Testkru website which we can use to test this.
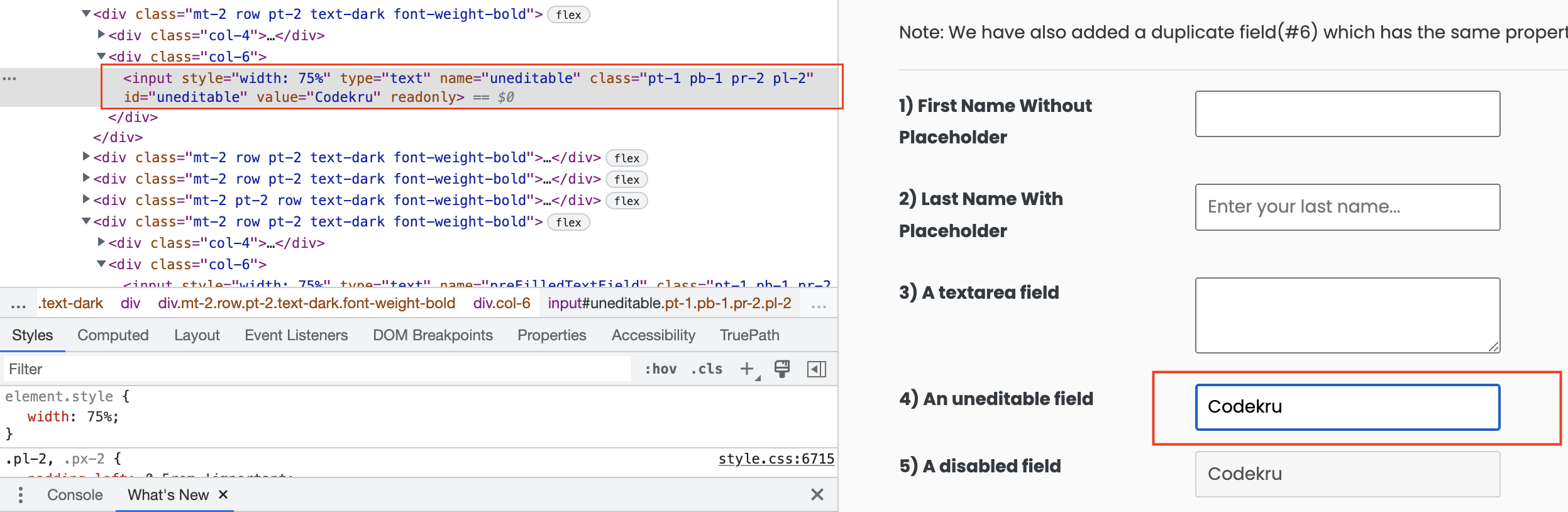
Let’s execute the clear() method on the non-editable field.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "/usr/local/bin/chromedriver");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
// getting WebElement associated with the id
WebElement ele = driver.findElement(By.id("uneditable"));
// clear the value present at the element
ele.clear();
}
}
Executing the above test case will throw the below error.
FAILED: test
org.openqa.selenium.InvalidElementStateException: invalid element state
(Session info: chrome=103.0.5060.134)
Build info: version: '3.141.59', revision: 'e82be7d358', time: '2018-11-14T08:17:03'
System info: host: 'LAPTOP-9A8M7TQJ', ip: '192.168.1.11', os.name: 'Windows 10', os.arch: 'amd64', os.version: '10.0', java.version: '15.0.1'
Driver info: org.openqa.selenium.chrome.ChromeDriver
Capabilities {acceptInsecureCerts: false, browserName: chrome, browserVersion: 103.0.5060.134, chrome: {chromedriverVersion: 103.0.5060.134 (8ec6fce403b..., userDataDir: C:\Users\MEHUL\AppData\Loca...}, goog:chromeOptions: {debuggerAddress: localhost:57618}, javascriptEnabled: true, networkConnectionEnabled: false, pageLoadStrategy: normal, platform: WINDOWS, platformName: WINDOWS, proxy: Proxy(), setWindowRect: true, strictFileInteractability: false, timeouts: {implicit: 0, pageLoad: 300000, script: 30000}, unhandledPromptBehavior: dismiss and notify, webauthn:extension:credBlob: true, webauthn:extension:largeBlob: true, webauthn:virtualAuthenticators: true}
Session ID: fb39973cfcbafedf4458b3ee3a273892
at java.base/jdk.internal.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
at java.base/jdk.internal.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:64)
at java.base/jdk.internal.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:45)
at java.base/java.lang.reflect.Constructor.newInstanceWithCaller(Constructor.java:500)
at java.base/java.lang.reflect.Constructor.newInstance(Constructor.java:481)
at org.openqa.selenium.remote.http.W3CHttpResponseCodec.createException(W3CHttpResponseCodec.java:187)
at org.openqa.selenium.remote.http.W3CHttpResponseCodec.decode(W3CHttpResponseCodec.java:122)
at org.openqa.selenium.remote.http.W3CHttpResponseCodec.decode(W3CHttpResponseCodec.java:49)
at org.openqa.selenium.remote.HttpCommandExecutor.execute(HttpCommandExecutor.java:158)
at org.openqa.selenium.remote.service.DriverCommandExecutor.execute(DriverCommandExecutor.java:83)
at org.openqa.selenium.remote.RemoteWebDriver.execute(RemoteWebDriver.java:552)
at org.openqa.selenium.remote.RemoteWebElement.execute(RemoteWebElement.java:285)
at org.openqa.selenium.remote.RemoteWebElement.clear(RemoteWebElement.java:124)
at Demo.CodekruTestFirst.test(CodekruTestFirst.java:29)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:64)
at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.base/java.lang.reflect.Method.invoke(Method.java:564)
at org.testng.internal.MethodInvocationHelper.invokeMethod(MethodInvocationHelper.java:133)
at org.testng.internal.TestInvoker.invokeMethod(TestInvoker.java:598)
at org.testng.internal.TestInvoker.invokeTestMethod(TestInvoker.java:173)
at org.testng.internal.MethodRunner.runInSequence(MethodRunner.java:46)
at org.testng.internal.TestInvoker$MethodInvocationAgent.invoke(TestInvoker.java:824)
at org.testng.internal.TestInvoker.invokeTestMethods(TestInvoker.java:146)
at org.testng.internal.TestMethodWorker.invokeTestMethods(TestMethodWorker.java:146)
at org.testng.internal.TestMethodWorker.run(TestMethodWorker.java:128)
at java.base/java.util.ArrayList.forEach(ArrayList.java:1511)
at org.testng.TestRunner.privateRun(TestRunner.java:794)
at org.testng.TestRunner.run(TestRunner.java:596)
at org.testng.SuiteRunner.runTest(SuiteRunner.java:377)
at org.testng.SuiteRunner.runSequentially(SuiteRunner.java:371)
at org.testng.SuiteRunner.privateRun(SuiteRunner.java:332)
at org.testng.SuiteRunner.run(SuiteRunner.java:276)
at org.testng.SuiteRunnerWorker.runSuite(SuiteRunnerWorker.java:53)
at org.testng.SuiteRunnerWorker.run(SuiteRunnerWorker.java:96)
at org.testng.TestNG.runSuitesSequentially(TestNG.java:1212)
at org.testng.TestNG.runSuitesLocally(TestNG.java:1134)
at org.testng.TestNG.runSuites(TestNG.java:1063)
at org.testng.TestNG.run(TestNG.java:1031)
at org.testng.remote.AbstractRemoteTestNG.run(AbstractRemoteTestNG.java:115)
at org.testng.remote.RemoteTestNG.initAndRun(RemoteTestNG.java:251)
at org.testng.remote.RemoteTestNG.main(RemoteTestNG.java:77)
===============================================
Default test
Tests run: 1, Failures: 1, Skips: 0
===============================================
Q – What if we use the clear() method on a radio button?
We will again get the InvalidElementStateException. This you can try by yourself with the radio button elements on this page.
Q – What if we use the clear() method on a null WebElement?
There might be a scenario when we might be executing the clear() method on a null WebElement. Then what would happen?
We will get a NullPointerException as illustrated by the below program.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "C:\\Users\\MEHUL\\OneDrive\\Desktop\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = null;
element.clear();
}
}
Output –
java.lang.NullPointerException: Cannot invoke "org.openqa.selenium.WebElement.clear()" because "element" is null
Please visit this link to learn more about WebElement and its methods.
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.