A String represents a sequence of characters. In Java, strings are objects just like any other object. One interesting point about String in java is that it is immutable. Immutable means a string cannot be changed or modified once created. We will look at the immutability later in the post, don’t let us forget about it 😛
A String literal is just a sequence of characters within double quotes.
String str = "codekru" ;
First, we want you to be comfortable with String and memory because it provides insight into how Strings are being stored and accessed in Java. It will further help you better understand the various concepts of the Strings.
Facts About String and memory
One of the key goals for any programming language is to make efficient memory use. String literals may occupy large amounts of a program’s memory as the application grows. So, to make Java more efficient, the JVM set aside a special area of memory called the String constant pool.
When the compiler encounters a String literal, it checks the String pool to see if an identical String already exists or not. If the string already exists, then the reference to the new literal is directed to that existing string, so no new String literal is created.
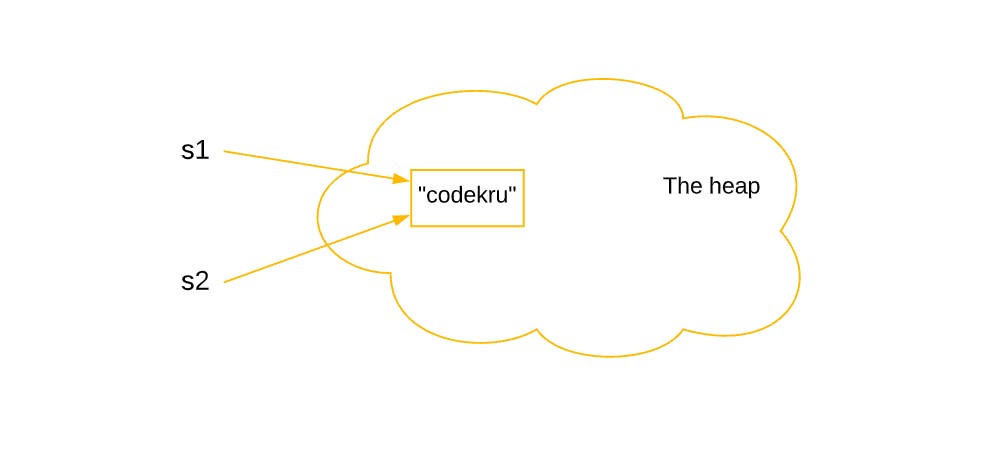
Those confused between heap and the String constant pool, don’t worry, remember, we talked about that JVM set aside a special area of memory. Well, that special area is within the heap itself. The below diagram may further clarify it.
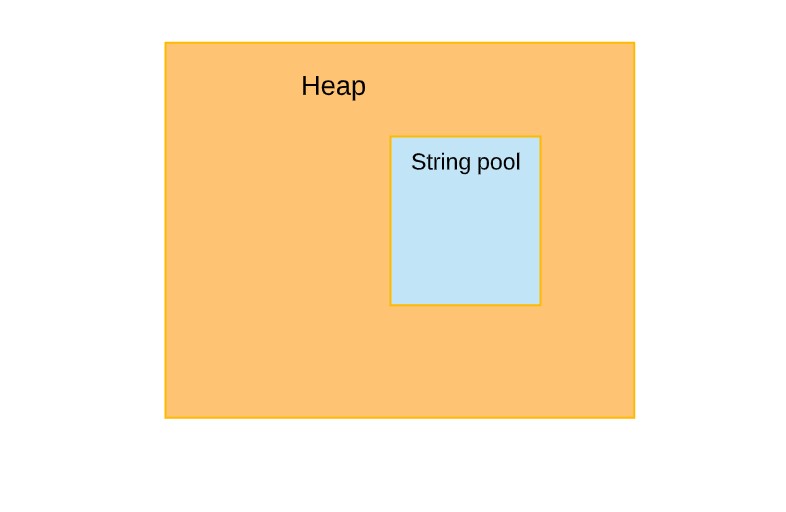
Now, you must be starting to see why immutability is vital in Strings?
If several variables are referencing the same String without even knowing it, it would be terrible if any of them could change the String’s value. Suppose there are two variables, s1, and s2, referencing the same String ( “codekru” ), then if s1 somehow changes the value of the String, it would affect s2 also.
You might say now, what if someone overrides the String class functionality? Wouldn’t that cause problems in the pool?
That is one of the main reasons the String class is marked as final. Nobody can override the functionality of the String methods. So, we can rest assured that the String objects will always be immutable.
How to create new Strings
There are many ways to create Strings, but we will discuss the most used ones.
Using String literals
We have to write the strings in the double quotes, and that’s it. Let’s assume that no String object exists in the pool yet.
String str = "codekru" ; // creates one String object and one reference variable
It will create “codekru” in the String pool, and the str variable will refer to it.
What if we created another variable, s2, with the same String literal “codekru”? Now, it will not create another string literal. Instead, it will reference the already existing one, which can be proved by using the == operator.
public class Codekru {
public static void main(String[] args) {
String str = "codekru";
String s2 = "codekru";
if (str == s2) {
System.out.println("referring to the same instance"); // will print this
} else {
System.out.println("Not referring to the same instance");
}
}
}
Output –
referring the same instance
Using String() constructor
We can also create a String object using the new keyword.
String str = new String("Codekru"); // creates two objects and one reference variable
As we have used the new keyword, Java will create a new String object in normal (non-pool) memory, and str will refer to it. In addition to this, the literal “codekru” will also be placed in the pool.
Let’s try making a new object here with the same string literal ( “codekru” )
public class Codekru {
public static void main(String[] args) {
String str = new String("codekru");
String s2 = new String("codekru");
if (str == s2) {
System.out.println("referring to the same instance");
} else {
System.out.println("Not referring to the same instance");
}
}
}
Output –
Not referring to the same instance
So, we can see that a new String object will be created every time after using the new keyword.
Now, let’s get back to the immutability concept of the String. Look at the below program.
public class Codekru {
public static void main(String[] args) {
String str = "hello";
str = str.concat(" codekru");
System.out.println(str);
}
}
Output –
hello codekru
Now, it seems like the string has changed. It was “hello” earlier, and now it became “hello codekru”. So what was all the talk about that String can never be changed or modified?
What happened here is that the String itself has not changed. It just created a new String, “hello codekru” and our variable is just pointing to that now. So, now, we have two literals in the heap. One is “hello”, and the other is “hello codekru”.
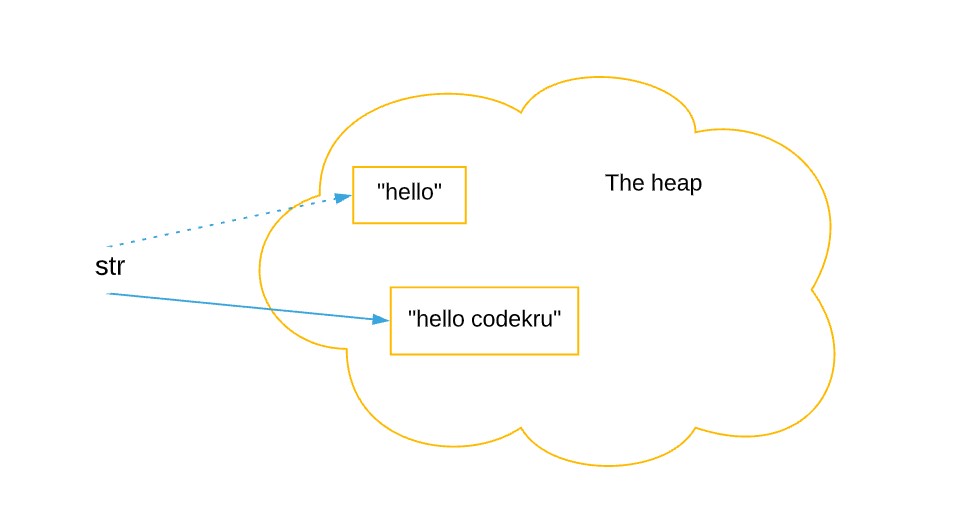
Java String Methods
Java provides us with many methods to access and perform various operations on the String. Some of them are listed below –
Method name | What it does |
---|---|
public char charAt(int index)
  |
Returns the char value present at the specified index. |
public int codePointAt(int index)
  |
Returns the character (Unicode code point) at the specified index. |
public int compareTo(String anotherString)
  |
Compares two strings lexicographically. |
public String concat(String str)
  |
Concatenates the specified string to the end of this string. |
public boolean contains(CharSequence s)
  |
Returns true if and only if this string contains the specified sequence of char values. |
public boolean endsWith(String suffix)
  |
Checks whether a string ends with a specified string or not. |
public boolean equalsIgnoreCase(String anotherString)
  |
Compares two strings while ignoring cases. |
public int length()
  |
Returns the length of the string. |
public int indexOf(int ch)
  |
Returns the index of the first occurrence of the specified char. |
public int indexOf(String str)
  |
Returns the index of the first occurrence of the specified string. |
public boolean isEmpty()
  |
Returns true if length() is 0. |
public String strip()
  |
Returns the string with all leading and trailing whitespaces removed |
public String[] split(String regex)
  |
Splits this string around matches of the given regular expression |
public boolean startsWith(String prefix)
  |
It checks whether the string starts with the specified string or not. |
public String substring(int beginIndex)
  |
Returns a string that is a substring of the string starting from the specified index. |
public char[] toCharArray()
  |
Converts the string to a new character array. |
public String toLowerCase()
  |
It converts all of the characters in the string to lowercase. |
public String toUpperCase()
  |
Converts all of the characters in the string to uppercase. |
public static String valueOf()
  |
It returns the string representation of the argument |
public String trim()
  |
Returns the string with all leading and trailing spaces removed |
We hope that you liked the article. If you have any doubts or concerns, please feel free to reach us in the comments or mail us at admin@codekru.com.