In certain scenarios, retaining the responses obtained from a JMeter test run in a CSV (Comma-Separated Values) file may be necessary for future examination and analysis. This article delves into the details of how to save the responses to a CSV file using JMeter.
Let’s first execute a dummy API (“https://jsonplaceholder.typicode.com/posts/1“) and then we will save the result of this API in a CSV file.
Executing the API
Set Up a Test Plan
- Launch JMeter.
- Go to the “File” Menu and select “New”.
- This will create a new test plan to be used in our example.
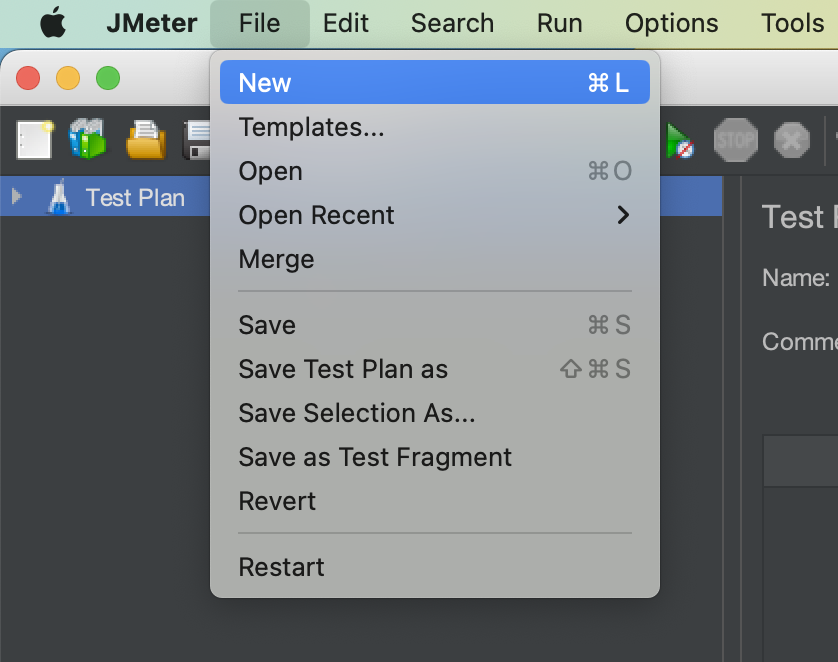
Create a Thread Group
- Right-click on the Test Plan.
- To create a Thread group, simply go to “Add > Threads (Users) > Thread Groups”.

The Thread Group is used to execute a specific number of tests. For simplicity, we will keep it set at 1. Please refer to the image below for configurations.
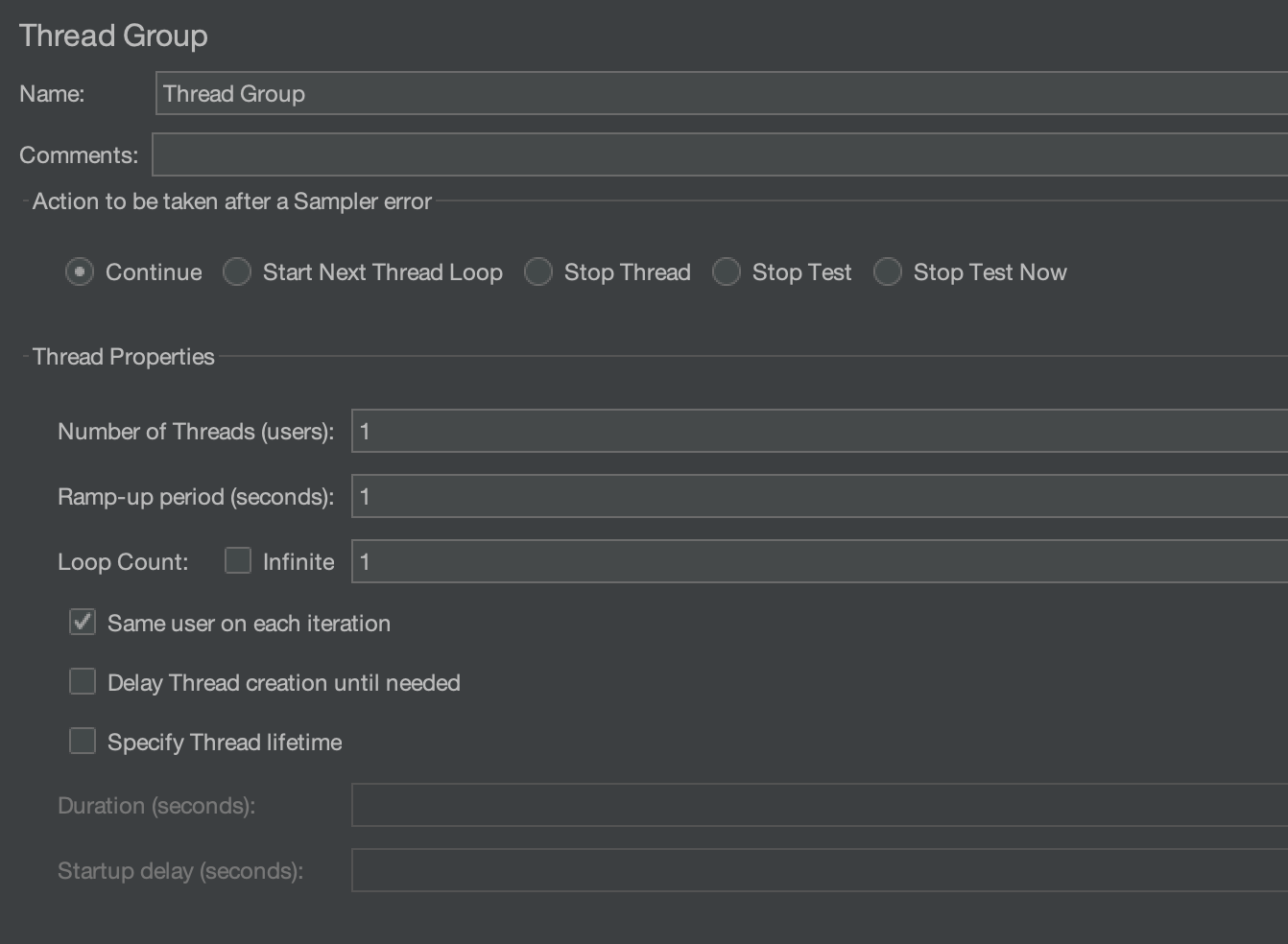
Add an HTTP sampler
We will use an HTTP sampler to execute a dummy API (https://jsonplaceholder.typicode.com/posts/1). We can create an HTTP sampler by right-clicking on the Thread Group and selecting “Add” > “Sampler” > “HTTP Request”.

We will hit the GET request on the “https://jsonplaceholder.typicode.com/posts/1” API. So, we will add it to the HTTP sampler by following the below steps.
- Please enter “https” in the input box that is labelled “Protocol[https]:“.
- Enter “jsonplaceholder.typicode.com” in the input box labelled “Server Name or IP”.
- By default, the HTTP request value is set to GET. Since we are only executing a GET request, we won’t be changing it.
- Set the Path to “/posts/1“.

Add “View Results Tree” listener
View Results Tree listener is one of the most used listeners in Jmeter. It provides detailed information about server responses during performance testing.
Add the “View Results Tree” listener by right-clicking on the HTTP request and selecting “Add” > “Listener” > “View Result Tree”.
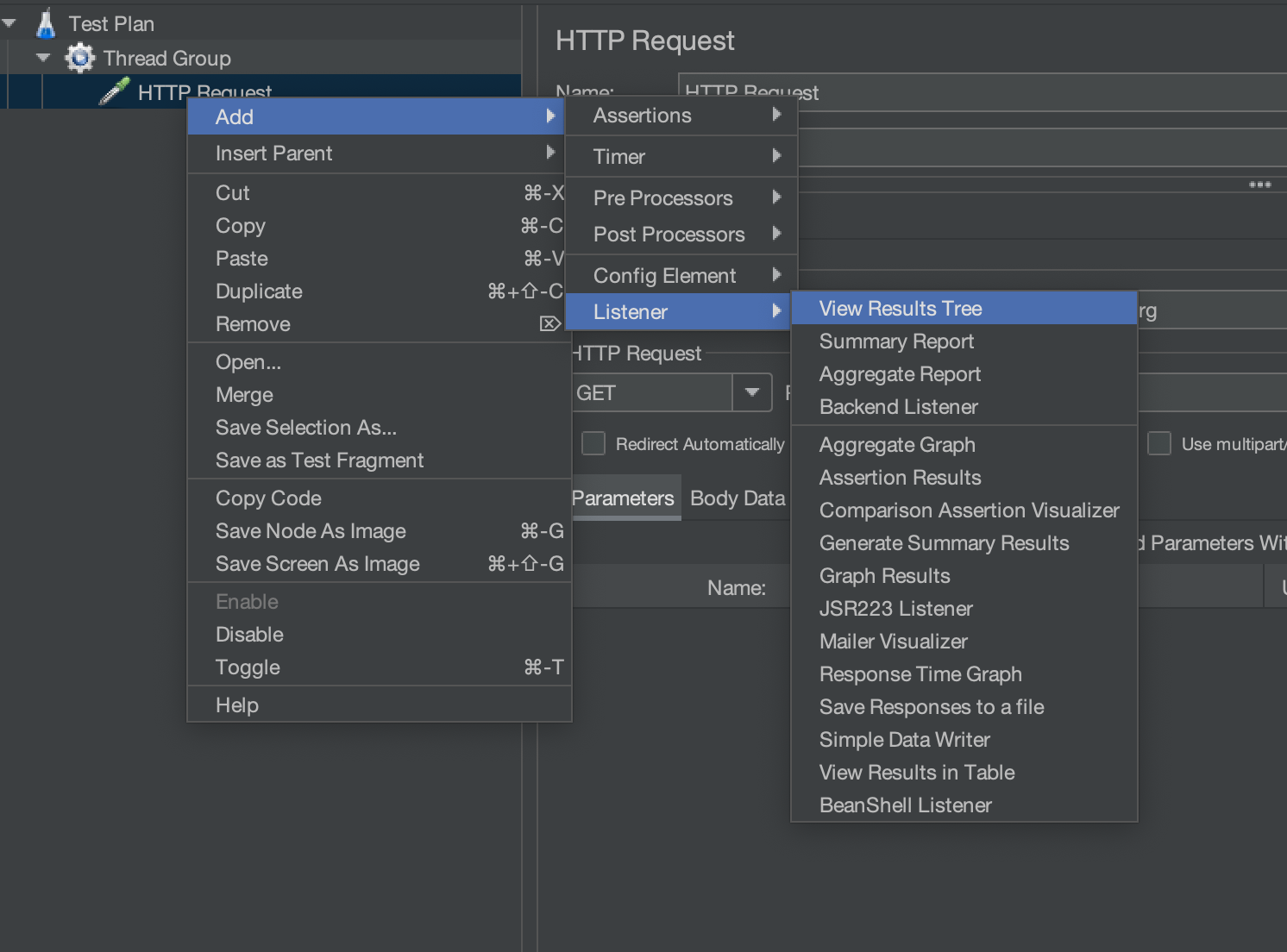
Run Test and monitor results
Run the test case by clicking on the green start button and analyze the results in the “view result tree” listener.
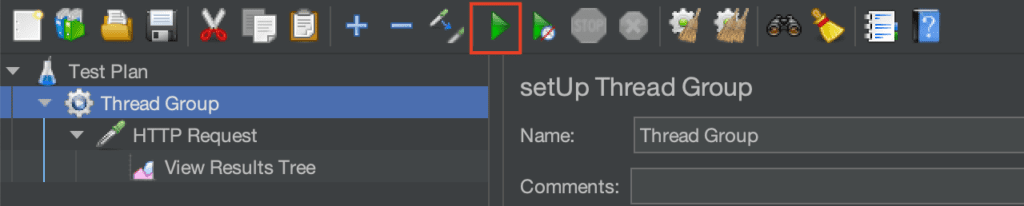
“View result tree” displays the request and response.
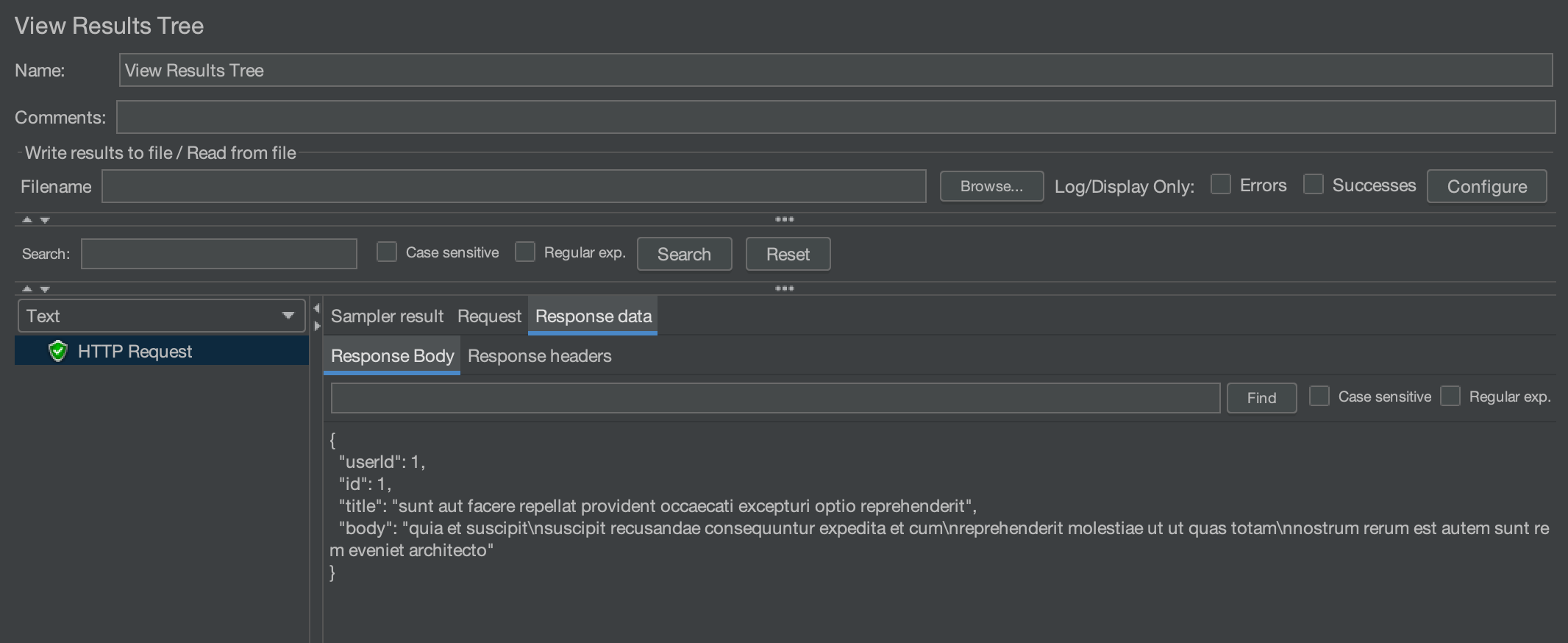
Saving the response data to a CSV file
We have received below response from the API and will attempt to save it to a CSV file.
{
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit",
"body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto"
}
We will use the JSR223 PostProcessor and write the code there to save the API’s response in a CSV file.
Adding the JSR223 PostProcessor
JSR223 PostProcessor in Jmeter is one of the most commonly used ways to process the response data. To add this PostProcessor, right-click on the HTTP sampler and select “Add” > “Post Processors” > “JSR223 PostProcessor”.
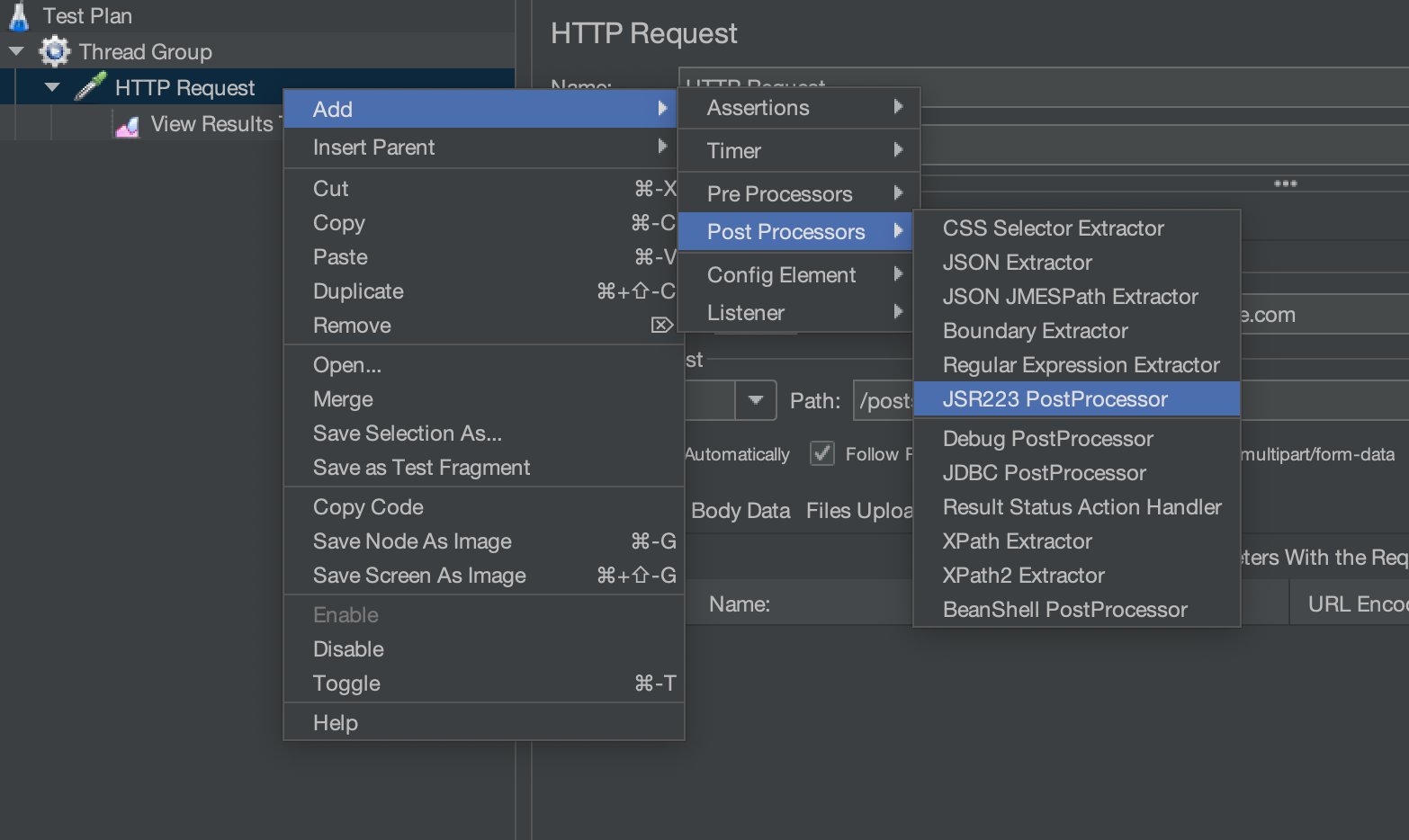

Writing code
Getting the API response
prev.getResponseData()
will get us the response that we received from the API. It returns a byte array( byte[] ), which we can convert to a String using new String(prev.getResponseData())
.
// Getting the response body
String responseBody = new String(prev.getResponseData());
Using FileWriter to write into a CSV file
Let’s assume that the CSV file path is “D:/Demo.csv”
String fileName = "D:/Demo.csv";
Below code writes the response in the CSV file.
try {
FileWriter fileWriter = new FileWriter(new File(fileName), true);
responseBody ="\"" + responseBody.replaceAll("\"", "\"\"") + "\"";
fileWriter.write(responseBody);
fileWriter.write("\n");
fileWriter.flush();
fileWriter.close();
}
catch (IOException e) {
}
responseBody ="\"" + responseBody.replaceAll("\"", "\"\"") + "\"";
line is necessary to tackle all the newlines or other escape characters that we might encounter while saving the response.
Below is the whole Code –
// Getting the response body
String responseBody = new String(prev.getResponseData());
String fileName = "D:/Demo.csv";
try {
FileWriter fileWriter = new FileWriter(new File(fileName), true);
responseBody ="\"" + responseBody.replaceAll("\"", "\"\"") + "\"";
fileWriter.write(responseBody);
fileWriter.write("\n");
fileWriter.flush();
fileWriter.close();
}
catch (IOException e) {
}

Now, again click on the “Run” button to execute the test plan.

You will see that the API’s response is now saved to the CSV file.
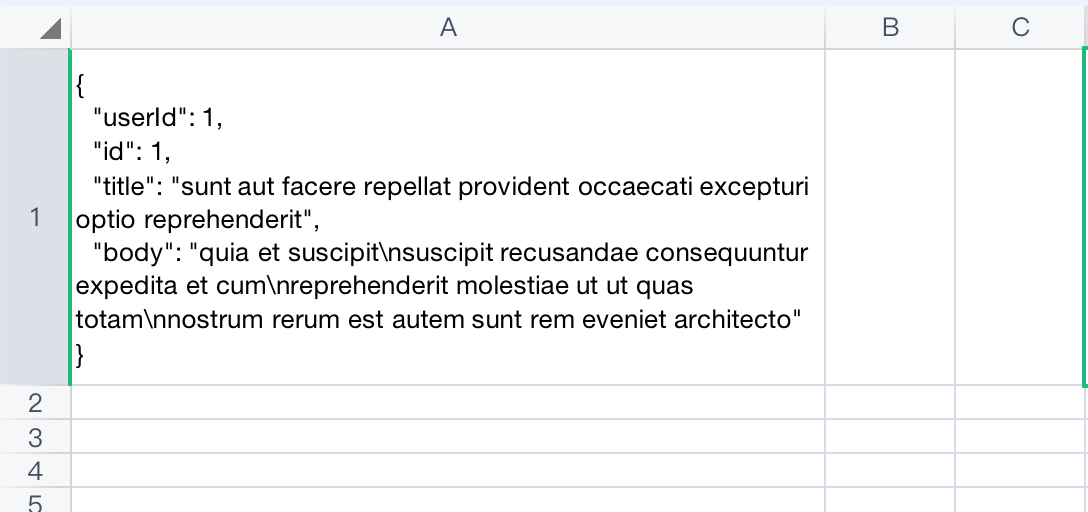
Now, this should be enough if you are looking to save a response in a CSV file, but we will explore more to save multiple responses in the CSV file.
Saving multiple responses from each HTTP sampler in the CSV file
So far, we have set all the Thread Group configurations to 1, which resulted in the HTTP request being executed only once, and thus, only one response was saved in the CSV file. However, in a real-world scenario, there will be hundreds or thousands of requests whose data we need to save in a CSV file. Let’s attempt to do that now.
- We will execute the “https://jsonplaceholder.typicode.com/posts/${postID}” request where postID is the post number, such as 1, 2, 3, and so on.
- We will send a request, then increase the postID value by 1 and send the request again.
- We will keep repeating Step 2 until all the requests are completed.
Add postID variable in Test Plan
First, make a “postID” variable and initialize its value to 0. To do this, navigate to the “Test Plan” screen, click the “Add” button, and enter the variable’s name and its value.

Increment the postID value
And now, we would need to increment the postID value by 1 with every request. This can be done with the help of a PreProcessor called “User Parameters” in Jmeter.
To add the “User Parameters”, right-click on the “Thread Group” and “Add” > “Pre Processors” > “User Parameters”.

Click on the “Add variable” button and add the “postID” under the “Name:” section and “${__intSum(${postID},1,)}” under the “User_1” section as shown below. ${__intSum(${postID},1,)} is used to increment the value by 1.
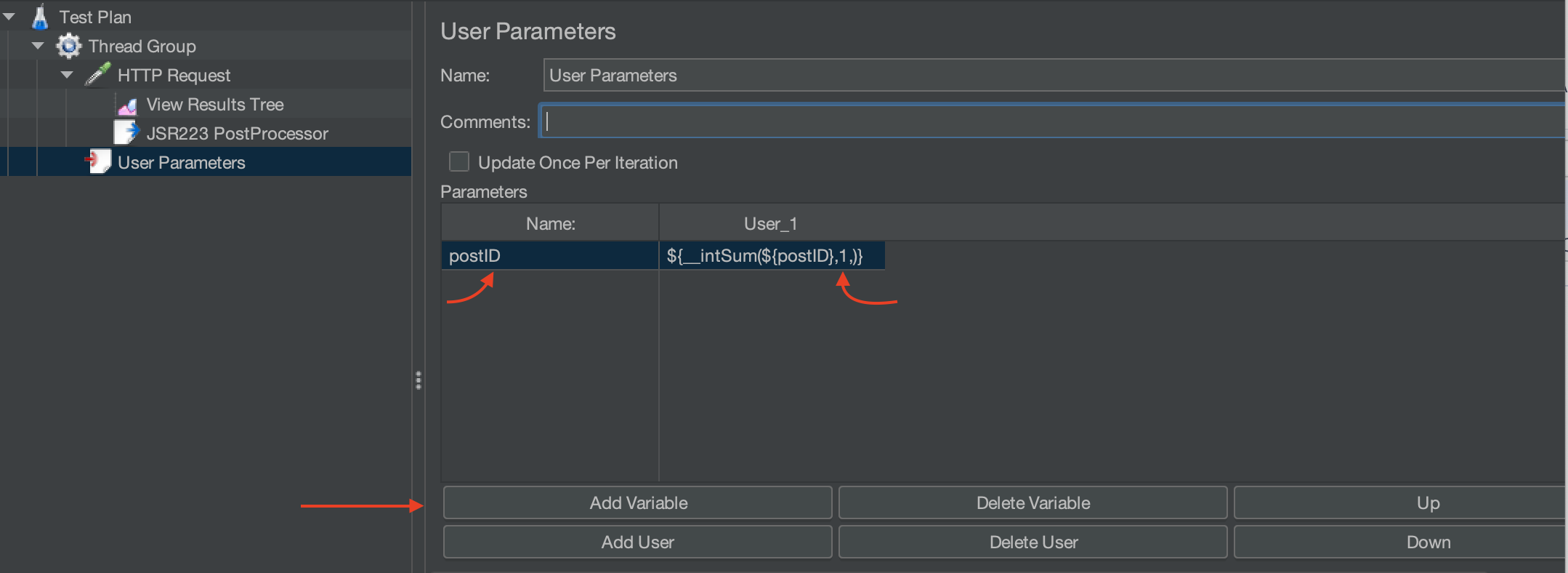
Parameterize the postID value in the HTTP sampler

Update the Loop count in Thread Group
Please update the Loop count in the Thread Group to 5. This will ensure that 5 requests are executed with postID values ranging from 1 to 5. After this, the code present in JSR223 PostProcessor will save the responses from all 5 requests in the CSV file.
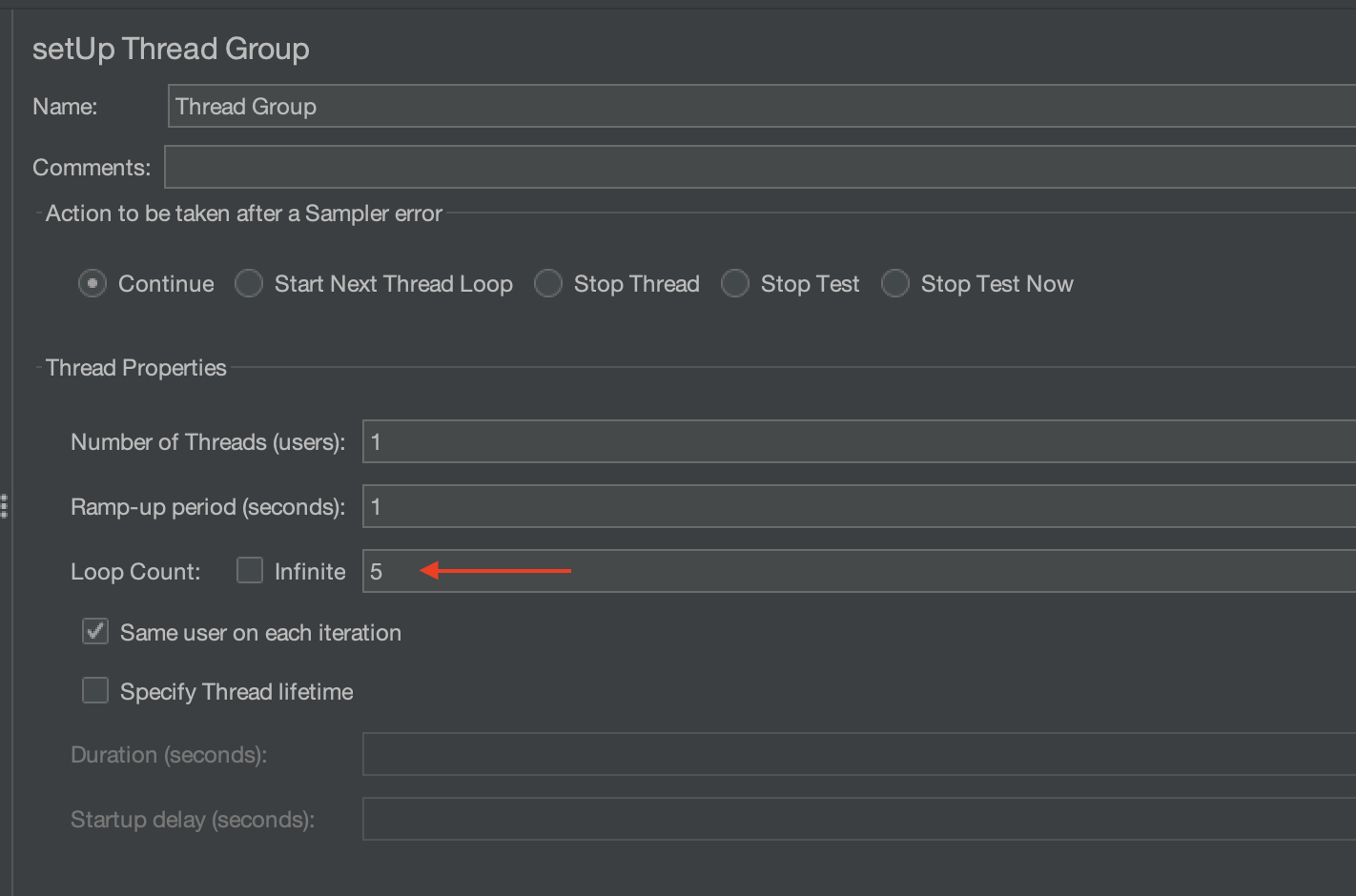
Finally, click on the run button to execute the Test Plan. You will see that the responses from all the executed requests is saved in the CSV file.
This is it. We hope that you liked the article. If you have any doubts or concerns, please write to us in the comments or mail us at admin@codekru.com.