Maven has built-in support for unit tests, and testing is a part of the maven default lifecycle. This post will discuss how to execute the unit test cases of a maven project.
First, let’s create a maven project. We can do that by using any IDE or running the below command in the terminal.
mvn archetype:generate -DgroupId=org.website.codekru -DartifactId=DemoProject -DpackageName=org.website.codekru -Dversion=1.0-SNAPSHOT -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
The above command will create a maven project named DemoProject in our current directory. Below is the project structure of the created project

We will write our unit test cases in the src/test/java directory. Maven will run the cases inside this directory only.
Steps to set up for the execution of unit test cases with maven
- We will be using the JUnit to write or test cases. So, we will add the JUnit Jupiter API maven dependency to our project. This link has the list of maven dependencies for JUnit Jupiter API. We will be using the 5.8.2 version, but you can choose the version of your liking.
- Next, we will also add the surefire plugin to our project. It will facilitate the execution of unit test cases during the test phase of the maven build lifecycle. Remember that the test case would still be executed if we didn’t use the surefire plugin. Still, we prefer to keep it as it provides more functionality and flexibility while running the test cases.
- We will write our unit test case in AppTest class. If you have made a maven project using the above command, then there will be some pre-written code in AppTest class, but we will be erasing all of the pre-written code and writing our own.
Our pom.xml will finally be
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.website.codekru</groupId>
<artifactId>DemoProject</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>DemoProject</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.2</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
</plugin>
</plugins>
</build>
</project>
Let’s write our cases in the AppTest class now.
package org.website.codekru;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
/**
* Unit test for simple App.
*/
public class AppTest {
@Test
public void test1() {
System.out.println("Executing the first test case");
Assertions.assertTrue(true);
}
@Test
public void test2() {
System.out.println("Executing the second test case");
Assertions.assertTrue(true);
}
}
Now, we will use the below command to execute unit test cases in our maven project.
mvn test
After executing the above command, all cases under the src/test/java directory will run. In our scenario, only two test cases were present in AppTest.java, so those two cases will be executed after running the command.

We can see that both unit cases are executed.
Note: Even if you run the “mvn install” command, maven will still execute the test cases as the test phase comes before the install phase, and the “mvn install” command will run every phase up to the install phase. You can read this article to learn about the phases in maven.
This would be enough to run every test case in the src/test/java directory. But what if we want to run only a single class, a bunch of classes, or only some selected class methods? How can we do that?
Now, to answer these questions. Let’s add some more test classes and methods to our project.
We have been working with only one Test class, i.e., AppTest class. So, let’s take two more test classes ( App2Test and App3Test ).
App2Test.java
package org.website.codekru;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
/**
* Unit test for simple App.
*/
public class App2Test {
@Test
public void test3() {
System.out.println("Executing the third test case");
Assertions.assertTrue(true);
}
@Test
public void test4() {
System.out.println("Executing the fourth test case");
Assertions.assertTrue(true);
}
}
App3Test.java
package org.website.codekru;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
/**
* Unit test for simple App.
*/
public class App3Test {
@Test
public void test5() {
System.out.println("Executing the fifth test case");
Assertions.assertTrue(true);
}
@Test
public void test6() {
System.out.println("Executing the sixth test case");
Assertions.assertTrue(true);
}
}
Now, our project structure would look like this –

Q – How to run only a single test class using maven?
Let’s suppose that we have to run the AppTest2 class only. Then we can do that by running the below command on the command line.
mvn -Dtest=App2Test test
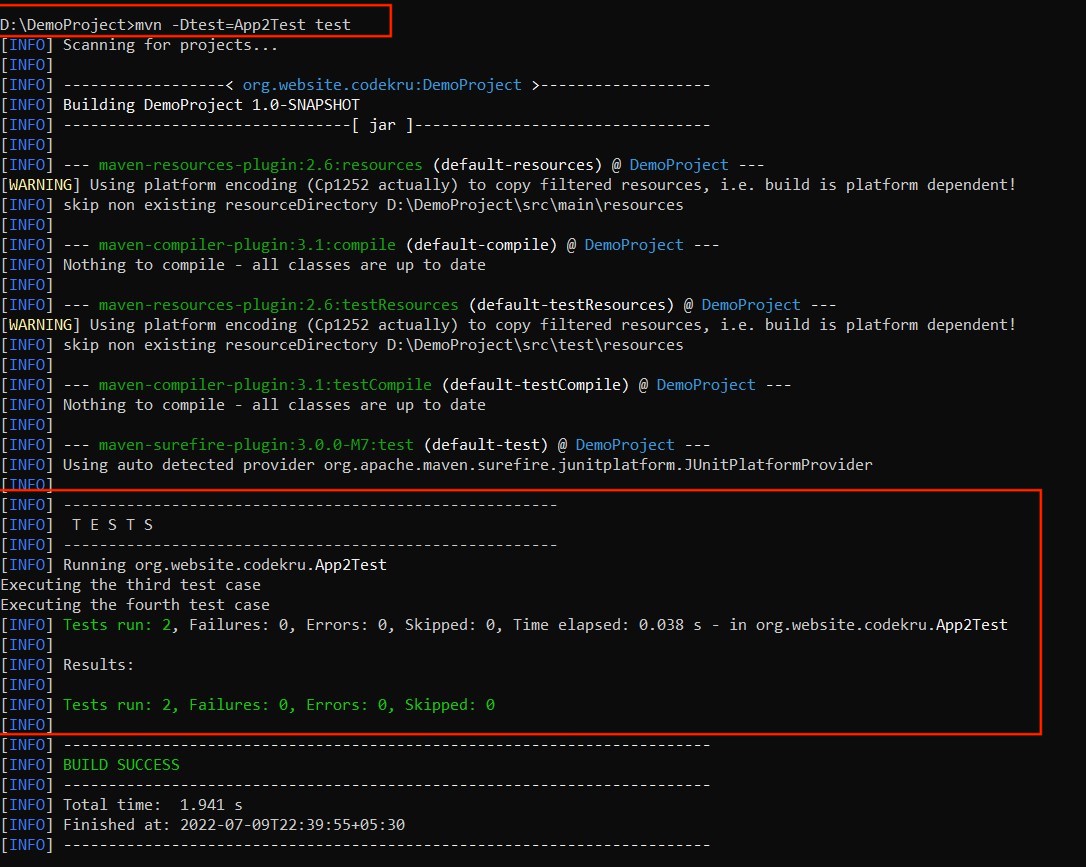
We can see in the above image that only App2Test class methods have been executed. There is one more way to achieve the same functionality, and that is by modifying the pom.xml file.
We will have to use the include tag to include only specific classes.
<project xmlns="http://maven.apache.org/POM/4.0.0"
[...]
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
<configuration>
<includes>
<include>App2Test.java</include>
</includes>
</configuration>
</plugin>
</plugins>
</build>
[...]
</project>
Now, we would only have to run the “mvn test” command, and it will only execute the App2Test class.
Q- How to run multiple test classes using maven?
We can also run multiple classes as well. The below command would help us achieve this functionality.
mvn -Dtest=AppTest,App2Test test
The above command will execute the unit test cases of the AppTest and App2Test classes.
Same can also be achieved by using the pom.xml file.
<project xmlns="http://maven.apache.org/POM/4.0.0"
[...]
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
<configuration>
<includes>
<include>App2Test.java</include>
<include>AppTest.java</include>
</includes>
</configuration>
</plugin>
</plugins>
</build>
[...]
</project>
Now, run the mvn test command, and maven will execute test cases of App2Test and AppTest classes.
Q- How to run a single test method of a class using maven?
We can also run a single method of a test class by using the below syntax –
mvn -Dtest=className#methodName test
Let’s say we want to execute the test1() method of the AppTest class.
mvn -Dtest=AppTest#test1 test
Q – How to run multiple methods of a class using maven?
Syntax of running the multiple test cases of a class in maven –
mvn -Dtest=className#method1+method2 test
We can use the below command to execute the test1() and test2() methods of AppTest class.
mvn -Dtest=AppTest#test1+test2 test
We have created a video demonstrating how to execute unit tests while building a Maven project.
Related Articles –
- How to ignore test failures while building projects in maven?
- How to skip unit test cases in a maven project?
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.