Earlier, we discussed executing unit test cases in maven, and this post will discuss how to skip them.
By default, Maven will execute the tests under the src/test/java directory. Below is our project structure, where we have one test class ( AppTest.java ) in the src/test/java directory.

AppTest.java
package org.website.codekru;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
/**
* Unit test for simple App.
*/
public class AppTest {
@Test
public void test1() {
System.out.println("Executing the first test case");
Assertions.assertTrue(true);
}
@Test
public void test2() {
System.out.println("Executing the second test case");
Assertions.assertTrue(true);
}
}
Our pom.xml file –
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.website.codekru</groupId>
<artifactId>DemoProject</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>DemoProject</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.2</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
</plugin>
</plugins>
</build>
</project>
If we run the “mvn install” command, the test cases under src/test/java will be executed.
Let’s see how we can skip all the test cases while building the Maven project
There are multiple ways to skip unit test cases, and we are going to discuss some of them –
- Using maven.test.skip
- Using skipTests configuration of maven surefire plugin
- And by using exclude configuration of Maven surefire plugin
Skipping tests using maven.test.skip
maven.test.skip can be used in the below ways –
– maven.test.skip as a command-line argument
mvn install -Dmaven.test.skip=true
This will skip all test cases under the src/test/java directory without making any other changes.
– maven.test.skip in pom.xml file
We can also skip the test cases by modifying the pom.xml file. It would require putting the below properties in the pom.xml file.
<properties>
<maven.test.skip>true</maven.test.skip>
</properties>
The whole pom.xml will look like this –
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.website.codekru</groupId>
<artifactId>DemoProject</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>DemoProject</name>
<url>http://maven.apache.org</url>
<properties>
<maven.test.skip>true</maven.test.skip>
</properties>
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.2</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
</plugin>
</plugins>
</build>
</project>
Now, if we run the “mvn install” command after modifying the pom.xml file, it will skip the test cases.
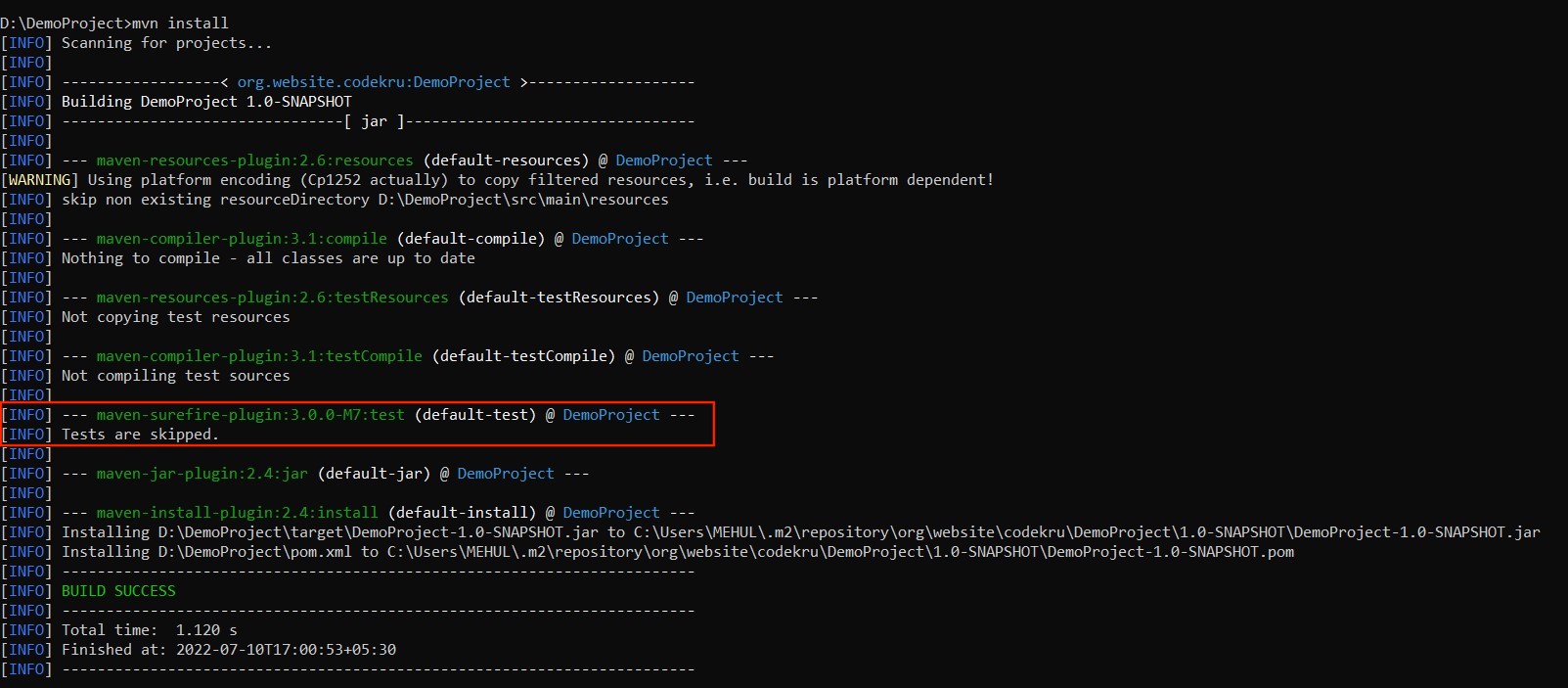
Using skipTests configuration of maven surefire plugin
– skipTests as a command-line argument
mvn install -DskipTests
This will also skip the unit test cases.
– skipTests by modifying the pom.xml file
We have to add the below configuration in the pom.xml file
<configuration>
<skipTests>true</skipTests>
</configuration>
The whole pom.xml will look like this –
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.website.codekru</groupId>
<artifactId>DemoProject</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>DemoProject</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.2</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
<configuration>
<skipTests>true</skipTests>
</configuration>
</plugin>
</plugins>
</build>
</project>
Now, the test cases will be skipped.
Using exclude configuration of maven surefire plugin
We can skip all unit test cases using the <excludes> configuration of the Maven surefire plugin.
<configuration>
<excludes>
<exclude>**</exclude>
</excludes>
</configuration>
Below is our pom.xml file
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.website.codekru</groupId>
<artifactId>DemoProject</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>DemoProject</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.2</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
<configuration>
<excludes>
<exclude>**</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
This will skip all unit test cases under the src/test/java directory. You can verify it by running the “mvn install” command.
We have created a video demonstrating how to skip test cases while building a Maven project.
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.