Clicking on a button seems like a normal requirement for most website automation. We may have to click on a login button, a submit button, etc. This post will discuss how we can click on a button using Selenium Webdriver in Java.
We will use our selenium playground website to click on the highlighted button shown in the below image.

We can click on a button in selenium using various ways.
- Using the click() method
- Using Actions class
- Using JavascriptExecutor
Let’s look at each of the methods one by one.
Using click() method
WebElement interface provides a click() method that helps click on any clickable element. We can use the click() method to click on the highlighted button in the below image.
You can find this button at https://testkru.com/Elements/Buttons.



We will first have to find the element, and then we can click on it. So, let’s inspect the highlighted button.
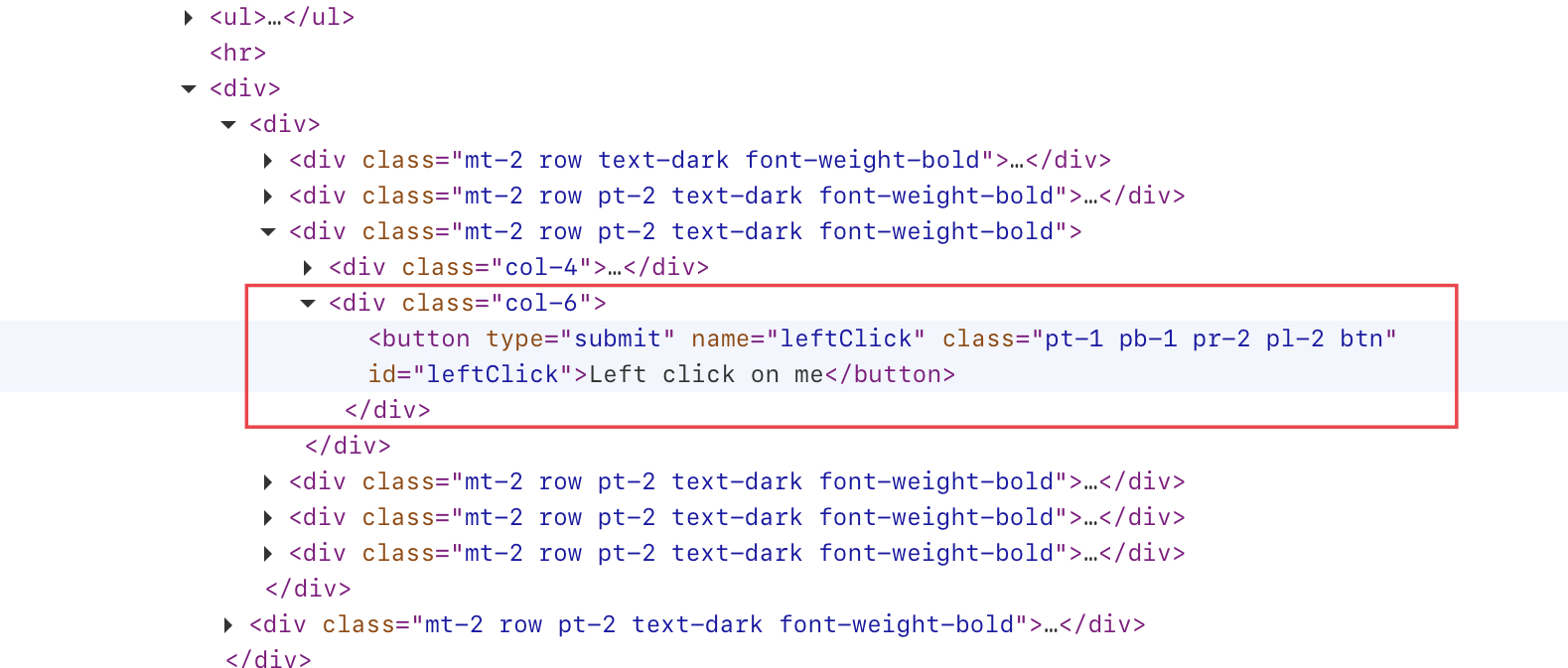
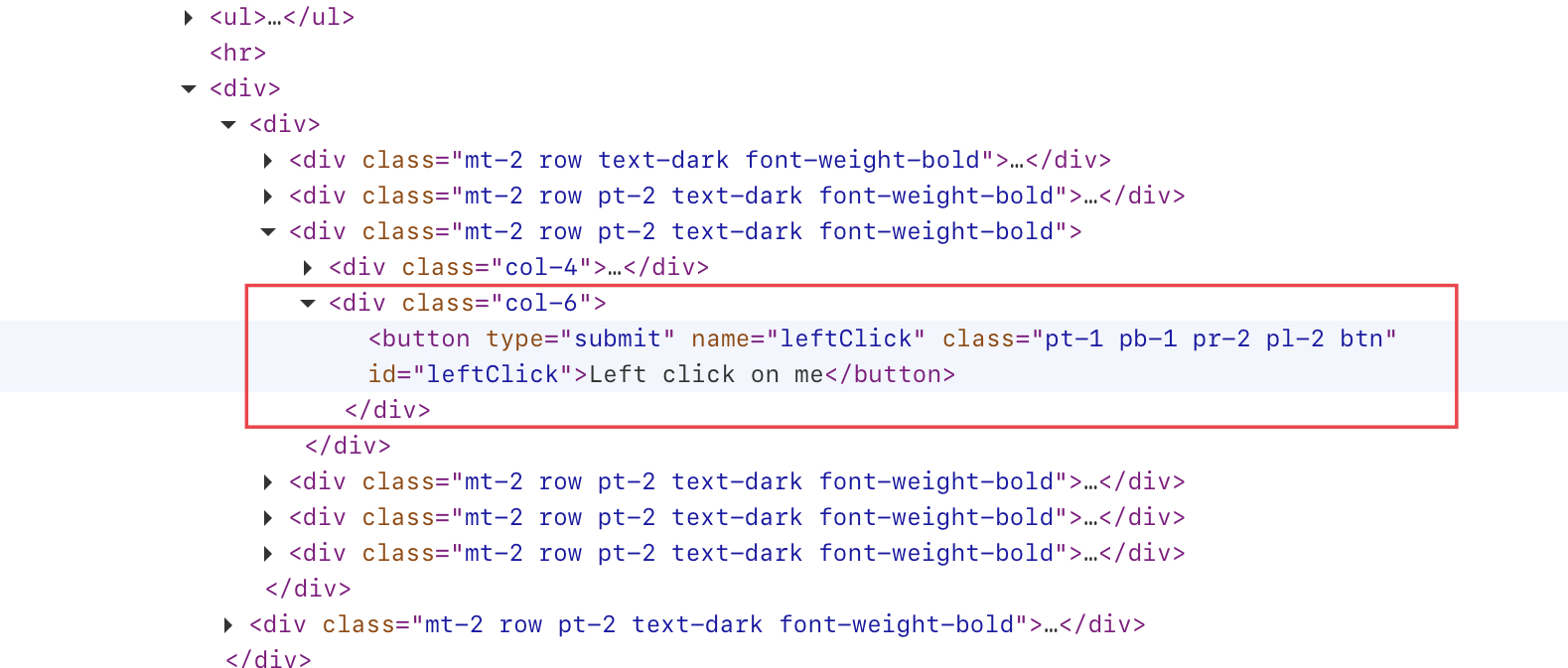
The element has a unique id (“leftClick”), so we can use it to find the element using the findElement() method. Below syntax can be used for it.
WebElement webElement = driver.findElement(By.id("leftClick"));
After we have the element, we can use the click() method, as shown below.
webElement.click();
Let’s write the whole program.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement webElement = driver.findElement(By.id("leftClick")); // finding the button
webElement.click(); // clicking on the button
}
}
Using Actions class
We can also use the Actions class to click on any element. Here also, we will find the element and then click on it, but instead of using the click() method provided by the WebElement interface, we will use the method provided by the Actions class.
Syntax of clicking the element using Actions class
Actions actions = new Actions(driver);
actions.moveToElement(webElement).click().build().perform();
Let’s write the program again by using the above syntax.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import org.testng.annotations.Test;
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement webElement = driver.findElement(By.id("leftClick")); // finding the button
Actions actions = new Actions(driver);
actions.moveToElement(webElement).click().build().perform();
}
}
Using JavascriptExecutor
We can also click an element using Javascript. Javascript also provides its own click() method, which helps us to click on the specified element, and we can execute that javascript code using the JavascriptExecutor in selenium.
JavascriptExecutor jse = (JavascriptExecutor)driver;
jse.executeScript("arguments[0].click();", webElement);
where webElement is the button element.
import org.openqa.selenium.By;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement webElement = driver.findElement(By.id("leftClick")); // finding the button
JavascriptExecutor jse = (JavascriptExecutor)driver;
jse.executeScript("arguments[0].click();", webElement);
}
}
This is it. We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.