Earlier, we talked about XPath and its basics, and this post will discuss the use of the starts-with() method while writing the XPath in Selenium.
starts-with() method locates the element(s) whose string value starts from a specified prefix.
Note: The starts-with() method is case-sensitive. So, “codekru” and “Codekru” aren’t the same for the starts-with() method.
starts-with() method can be used in various ways –
Let’s talk about it one by one.
Xpath starts-with() method with attributes
starts-with() method can locate the elements where attribute values start from a specific text.
Syntax for writing the XPath using the starts-with() method
//*[starts-with(@attribute_name,'prefix_string')]
or we can use the below syntax to limit the search to a specific tag.
//tag_name[starts-with(@attribute_name,'prefix_string')]
Code Example
We will locate the element whose “href” attribute value starts with “https” in the below code block.
<html>
<head>
</head>
<body>
<div>
<p>Codekru Tutorials</p>
</div>
<div>
<a class = "codekru" href = "https://www.codekru.com" id = "websiteLink">Codekru Website link</a>
<p>Guide to XPath in Selenium</p>
<em>This is a test node</em>
</div>
<div>
<a class = "col-6" href = "https://www.codekru.com/selenium">Selenium Link</a>
</div>
</body>
</html>
Keeping the syntax in mind, below XPath can find the element(s) whose “href” attribute starts from “https”.
//*[starts-with(@href,"http")]
It selected two elements, as shown in the below image.

If we want to limit our search to the “<a>” tag, then we can use the below XPath –
//a[starts-with(@href,"http")]
Let’s see a few examples with some of the most used attributes
starts-with() method with class attribute
Let’s take the same HTML code, and we will write the XPath to select element(s) whose class name starts with the “code” keyword.
<html>
<head>
</head>
<body>
<div>
<p>Codekru Tutorials</p>
</div>
<div>
<a class = "codekru" href = "https://www.codekru.com" id = "websiteLink">Codekru Website link</a>
<p>Guide to XPath in Selenium</p>
<em>This is a test node</em>
</div>
<div>
<a class = "col-6" href = "https://www.codekru.com/selenium">Selenium Link</a>
</div>
</body>
</html>
The class name for an element is defined by the “class” attribute. So, the below XPath would select such element(s) whose “class” attribute’s value start from the “code” keyword.
//*[starts-with(@class,"code")]
starts-with() method with id attribute
Below XPath will select element(s) whose id attribute value starts with the “website” keyword.
//*[starts-with(@id,"website")]
starts-with() method with text()
text() method helps select the elements based on the text shown on the webpage. If we combine the text() method with the starts-with() method, we can locate the elements whose text starts from a specific string.
Syntax
//*[starts-with(text(),prefix_text)]
or we can also limit the search to a specific tag by using –
//tag_name[starts-with(text(),prefix_text)]
Example
We will take the below HTML code and will find the element which starts with the “Guide” text.
<html>
<head>
</head>
<body>
<div>
<p>Codekru Tutorials</p>
</div>
<div>
<a class = "codekru" href = "https://www.codekru.com" id = "websiteLink">Codekru Website link</a>
<p>Guide to XPath in Selenium</p>
<em>This is a test node</em>
</div>
<div>
<a class = "col-6" href = "https://www.codekru.com/selenium">Selenium Link</a>
</div>
</body>
</html>
//*[starts-with(text(),"Guide")]
Above Xpath will select the required elements, as shown in the below image.
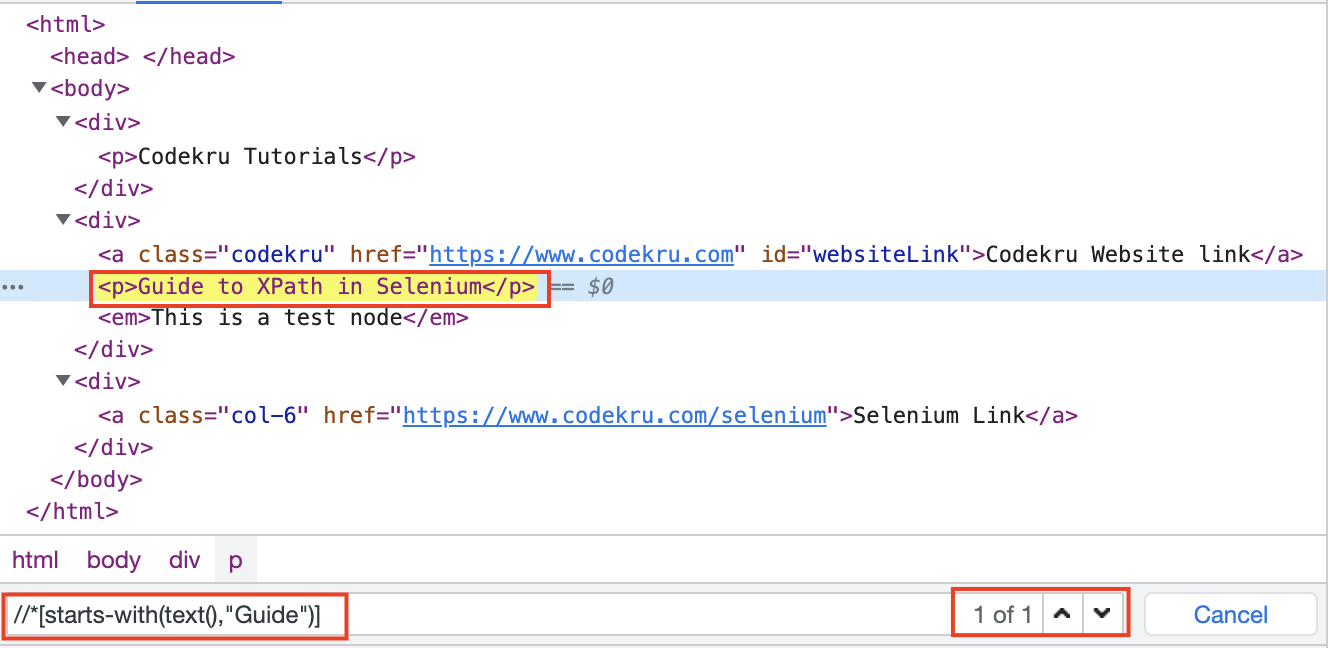
This is it. We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.