Sometimes we have to verify the placeholder text shown inside a text field or an input box. This post will discuss how we can get the placeholder text in selenium webdriver using Java.
Here is one of the elements on https://testkru.com/Elements/TextFields, which has a placeholder text.
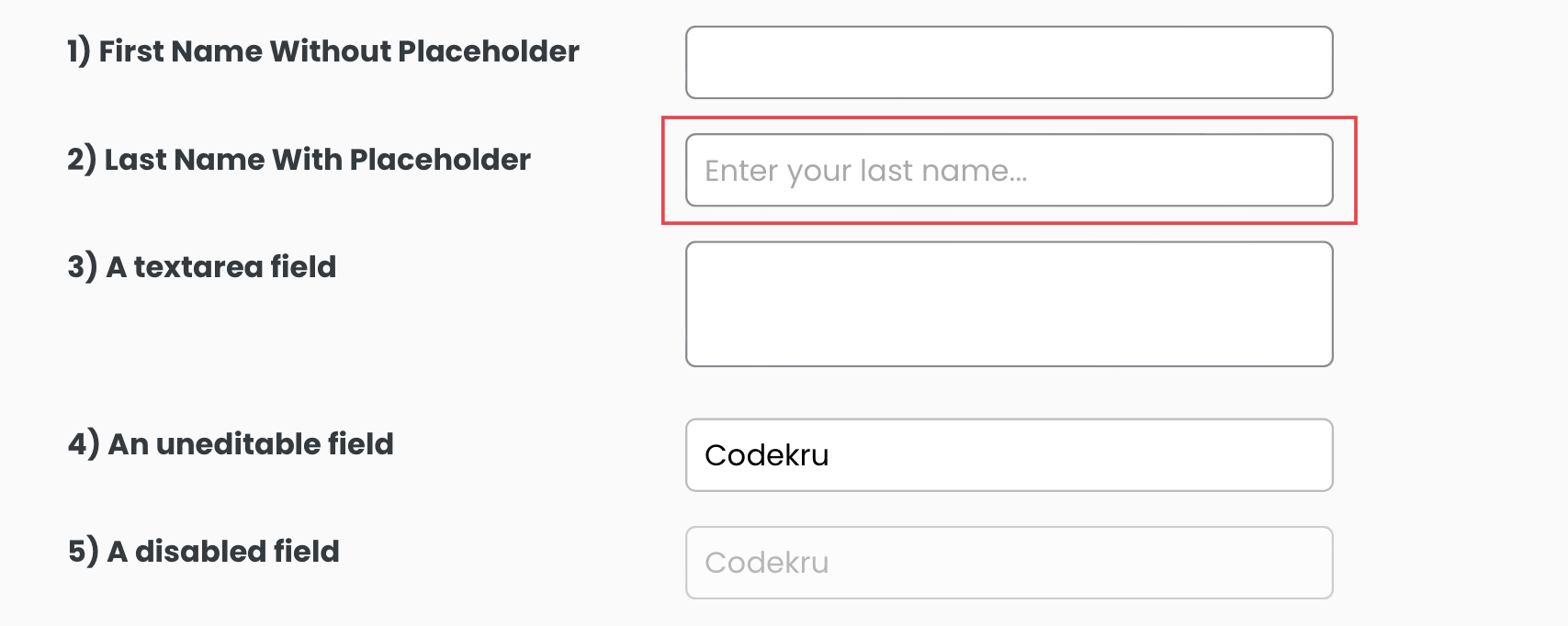
The placeholder text shown in the above image is “Enter your last name…” and is the value of the “placeholder” attribute of the element.

So, if we get the value of the placeholder attribute, then we will have our placeholder text shown on the webpage too.
We can use a couple of ways to get the placeholder attribute value
- getAttribute() method
- Using JavascriptExecutor
getAttribute() method
getAttribute() method of the WebElement interface is used to get the attribute value of an element. Here we want the value of the placeholder attribute, so we can use the below syntax to do that.
element.getAttribute("placeholder")
where the element is the WebElement whose placeholder text we want to find.
We will use the id attribute to find the element using the findElement() method.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("lastNameWithPlaceholder"));
System.out.println("Placeholder text: " + element.getAttribute("placeholder"));
}
}
Output –
Placeholder text: Enter your last name...
Using JavascriptExecutor
JavascriptExecutor in selenium is used to execute the javascript code. We can execute the script below to get an element’s placeholder text.
return arguments[0].placeholder
where arguments[0] is the WebElement whose placeholder text we want to find.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("lastNameWithPlaceholder"));
JavascriptExecutor jse = (JavascriptExecutor) driver;
System.out.println("Placeholder text: " + jse.executeScript("return arguments[0].placeholder", element));
}
}
Output –
Placeholder text: Enter your last name...
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.