We have discussed how we can execute the unit test cases in maven, but those were JUnit test cases and not TestNG. Those cases were executed as maven entered the test phase using the “mvn test” command.
This post will look at how we can integrate Maven with TestNG and execute the TestNG tests when maven enters the test phase.
Maven, by default, execute the unit test cases in the src/test/java directory, and we will follow this norm only and make out cases in the src/test/java directory.
We will integrate Maven with TestNG in a series of steps –
- Create a maven project
- Add maven dependencies and plugins
- Add TestNG tests
- Create a testng.xml file
- And finally, execute the TestNG tests
Create a maven project
We can easily create a project by running the below command on the command line tool.
mvn archetype:generate -DgroupId=org.website.codekru -DartifactId=DemoProject -DpackageName=org.website.codekru -Dversion=1.0-SNAPSHOT -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Please read this post to learn more about how we can create a maven project.
Moving ahead, a maven project will be created in the directory where we run the command, and it will have a default project structure created by maven.

Add maven dependencies and plugins
We will add the TestNG maven dependency and the maven surefire plugin in the pom.xml file.
TestNG maven dependency
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.6.1</version>
</dependency>
Maven surefire plugin dependency
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
</plugin>
</plugins>
</build>
The whole pom.xml will now be like this –
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.website.codekru</groupId>
<artifactId>DemoProject</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>DemoProject</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.testng/testng -->
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.6.1</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
</plugin>
</plugins>
</build>
</project>
Add TestNG tests
We will now add the TestNG tests in the AppTest class. A test case is a method marked with the @Test annotation of TestNG.
package org.website.codekru;
import org.testng.annotations.Test;
public class AppTest {
@Test
public void test1() {
System.out.println("Executing test1");
}
@Test
public void test2() {
System.out.println("Executing test2");
}
}
We have added two TestNG tests in the AppTest class. Now we would have to run these cases.
Create testng.xml file
Create a testng.xml file at the root of the project. testng.xml file helps in executing the test cases in TestNG.
Below is the content inside the testng.xml file.
<!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd" >
<suite name="codekru">
<test name="codekruTest">
<classes>
<class name="org.website.codekru.AppTest" />
</classes>
</test>
</suite>
The above XML file will execute all cases inside of AppTest class. Please read this article to learn how to create a testng.xml file.
Here is our updated project structure.

Execute the test cases
These test cases should be executed when maven executes its test phase.
For this to happen, we must make some XML file changes. If you remember, we added the maven surefire plugin earlier, and now we have to add some configurations to the plugin.
Earlier
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
</plugin>
</plugins>
</build>
Now
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
<configuration>
<suiteXmlFiles>
<suiteXmlFile>testng.xml</suiteXmlFile>
</suiteXmlFiles>
</configuration>
</plugin>
</plugins>
</build>
The newly added configuration will run the mentioned testng.xml file at the time when maven executes the test phase.
So, now our updated pom.xml will be
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.website.codekru</groupId>
<artifactId>DemoProject</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>DemoProject</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.testng/testng -->
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.6.1</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
<configuration>
<suiteXmlFiles>
<suiteXmlFile>testng.xml</suiteXmlFile>
</suiteXmlFiles>
</configuration>
</plugin>
</plugins>
</build>
</project>
Now, run the “mvn test” command from the command line, and now TestNG tests will also be executed.

But if you are getting the below error.

Please add the below properties in the pom.xml file.
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
So, now the updated pom.xml file would be –
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.website.codekru</groupId>
<artifactId>DemoProject</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>DemoProject</name>
<url>http://maven.apache.org</url>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.testng/testng -->
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.6.1</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M7</version>
<configuration>
<suiteXmlFiles>
<suiteXmlFile>testng.xml</suiteXmlFile>
</suiteXmlFiles>
</configuration>
</plugin>
</plugins>
</build>
</project>
Run the “mvn test” command again from the command line, and it will execute the TestNG tests.
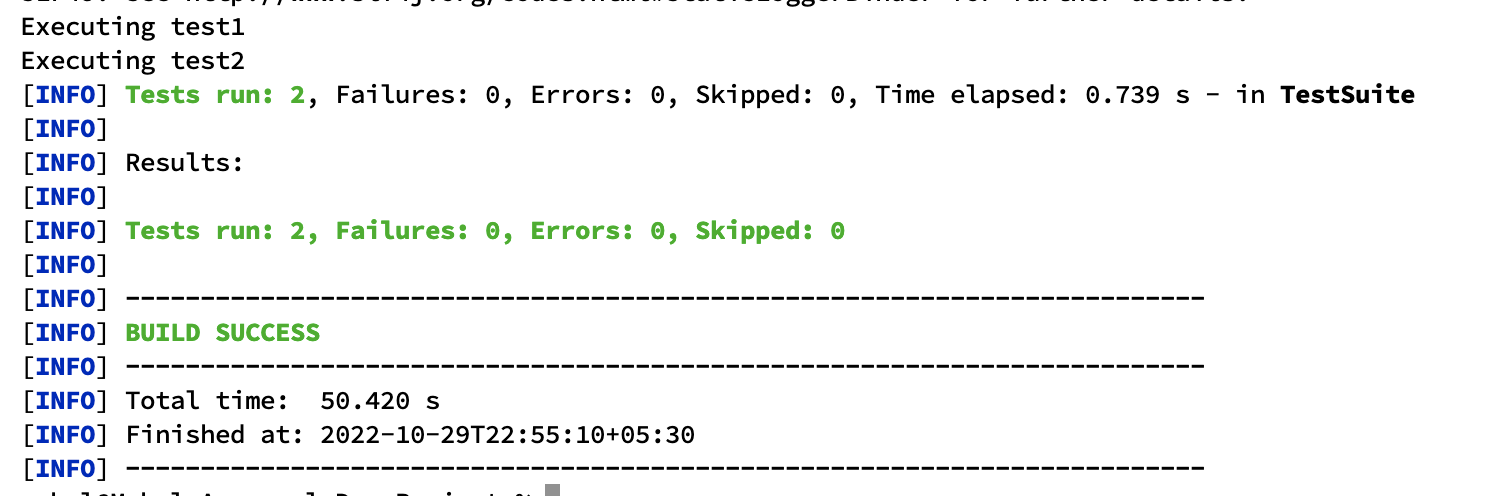
Finally, our TestNG tests were executed; this is how we can integrate maven with TestNG by using the maven surefire plugin.
We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.