This post will check the ways we can check whether a field is editable or not in selenium webdriver using Java.
How do we know if we can edit a field or not? The “readonly” attribute governs the editability of a field. If the “readonly” attribute is present on an element, then that element or field cannot be edited or manipulated.

So, if we find a way to know whether “readonly” is present for an element or not, then we can also determine the editability of a field.
We can find using –
- getAttribute() method
- and JavascriptExecutor
Let’s look at both ways one by one.
getAttribute() method
getAttribute() method of the WebElement interface is used to get an attribute’s value of an element.
We will get the value of the “readOnly” attribute using the getAttribute() method. If the method returned true, this means that the field is non-editable. Otherwise, the field is editable.
We will use a non-editable element present on https://testkru.com/Elements/TextFields.
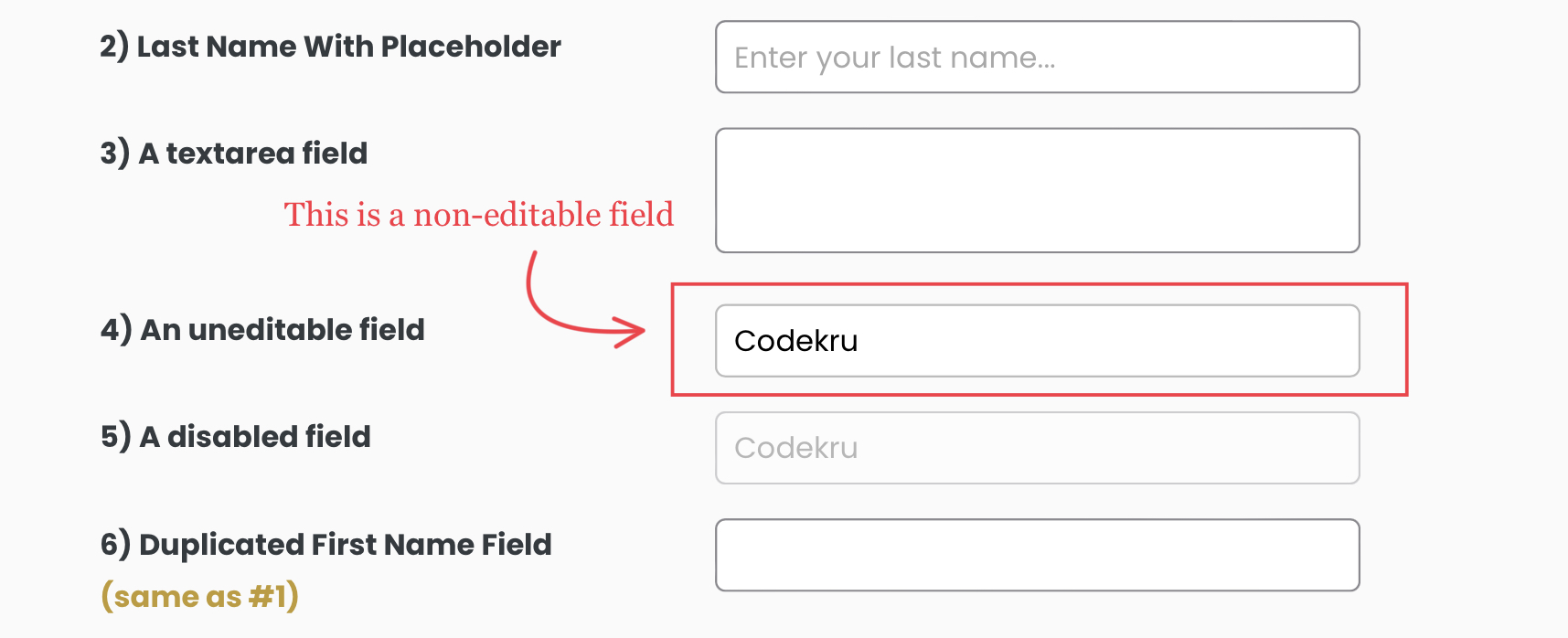

The id of the highlighted element is “uneditable“. We will use this id to find the element and then get to know its editability feature.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("uneditable"));
// this will tell whether the field is editable or not
System.out.println("Is text field non-editable: " + element.getAttribute("readonly"));
}
}
Output –
Is text field non-editable: true
As the readonly attribute was present for our element, we have gotten true in the result.
Using JavascriptExecutor
JavascriptExecutor is used to execute the javascript code in selenium.
We can also fetch the value of the readonly attribute via javascript code. The code below will give us true or false depending on whether the field has a readOnly attribute or not.
return arguments[0].readOnly
where arguments[0] is the element whose editability we want to check.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("uneditable"));
// this will tell whether the field is editable or not
JavascriptExecutor jse = (JavascriptExecutor) driver;
System.out.println("Is text field non-editable: " + jse.executeScript("return arguments[0].readOnly", element));
}
}
Output –
Is text field non-editable: true
This is it. We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.