Text boxes or fields are widely used throughout webpages, and this post will look at handling the text boxes using Selenium Webdriver in Java. There can be various text fields, and we will try to include most of them and perform various operations like clearing and entering text.
We will use our selenium playground website – testkru, to interact with various text boxes. You can also use the same website to perform any desired actions.
Below is the screenshot of the text boxes; we will use some of them to perform various operations on the text boxes.
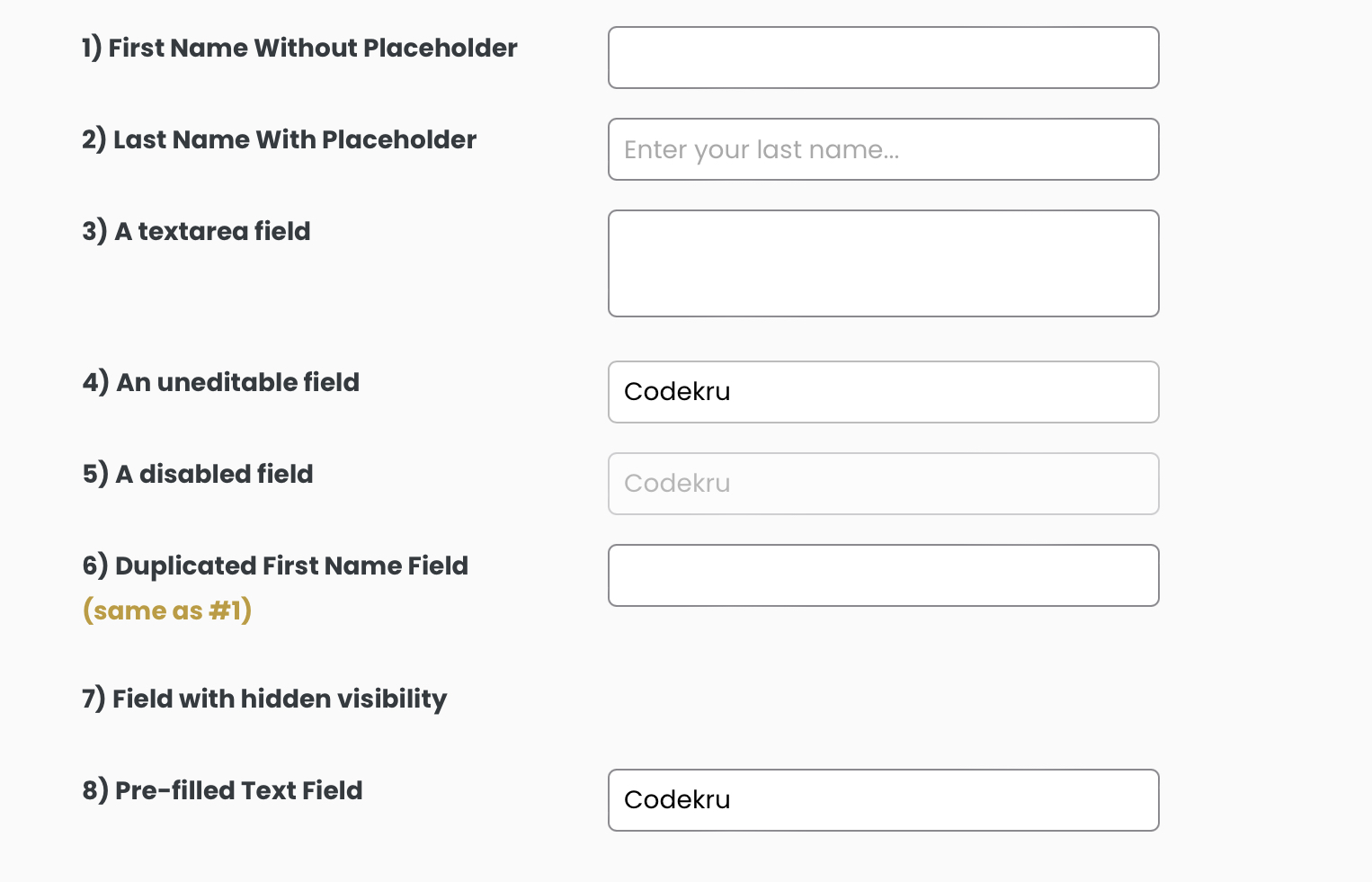
We will look at below points –
- What is a text box?
- How to find a text box element?
- How to enter a text in a text box?
- How to get a pre-filled text in a text box?
- How to get the placeholder text of an input text box?
- How to clear a pre-written text from a text box?
- How to check if a text field is editable or not?
- How to check if a text field is disabled or not?
What is a text box or a text field?
A text box or a text field is an area where the user can enter an input. Most of the text boxes will have the <input type = "text">
element. This HTML element denotes that a user is asked to enter some form of input in an area specified by the element.
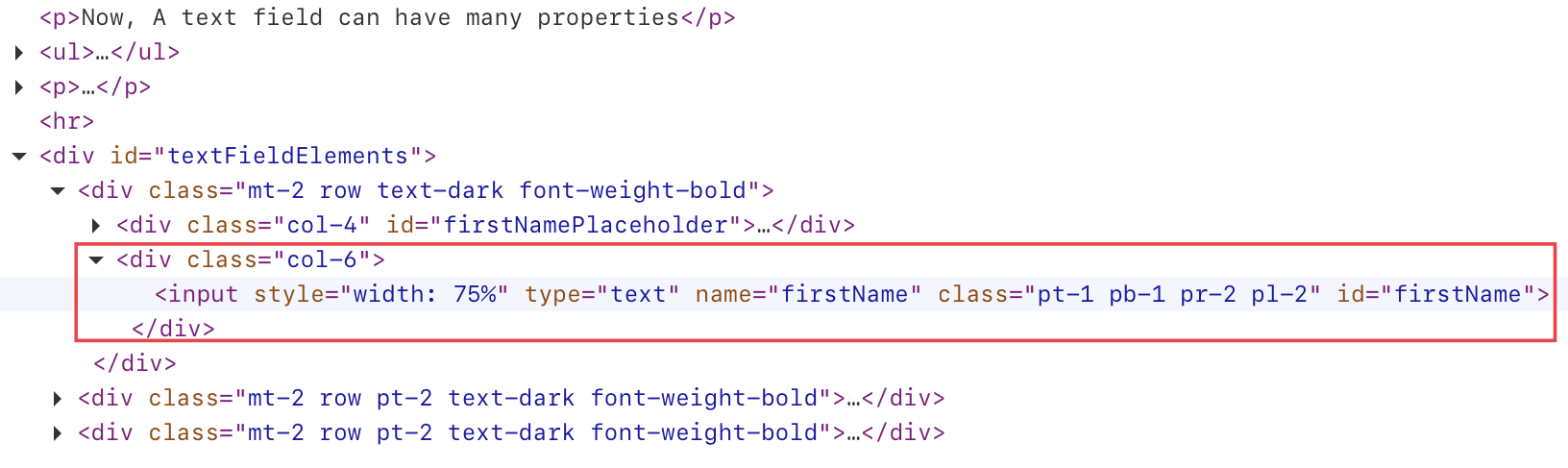
We can also use an alternate <textarea>
tag to take input from a user.
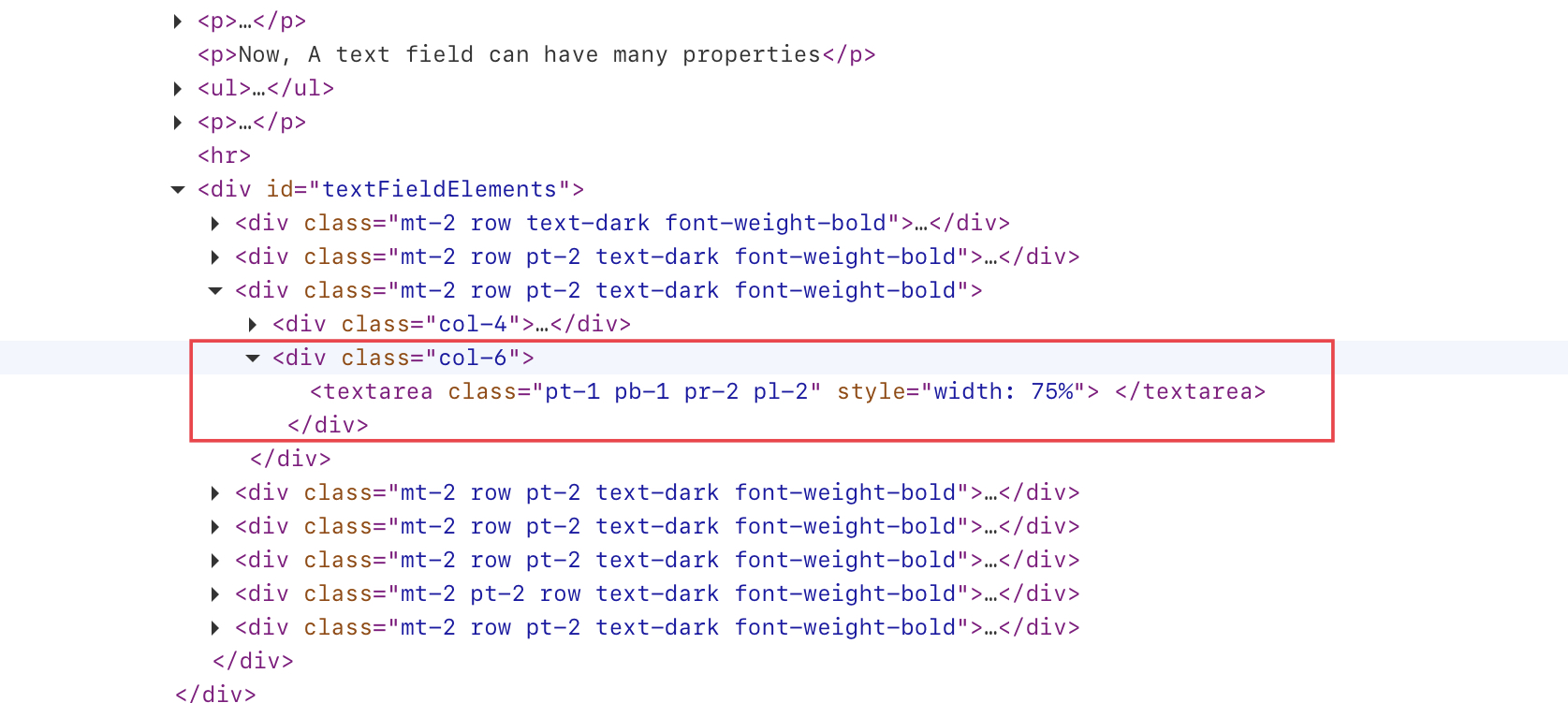
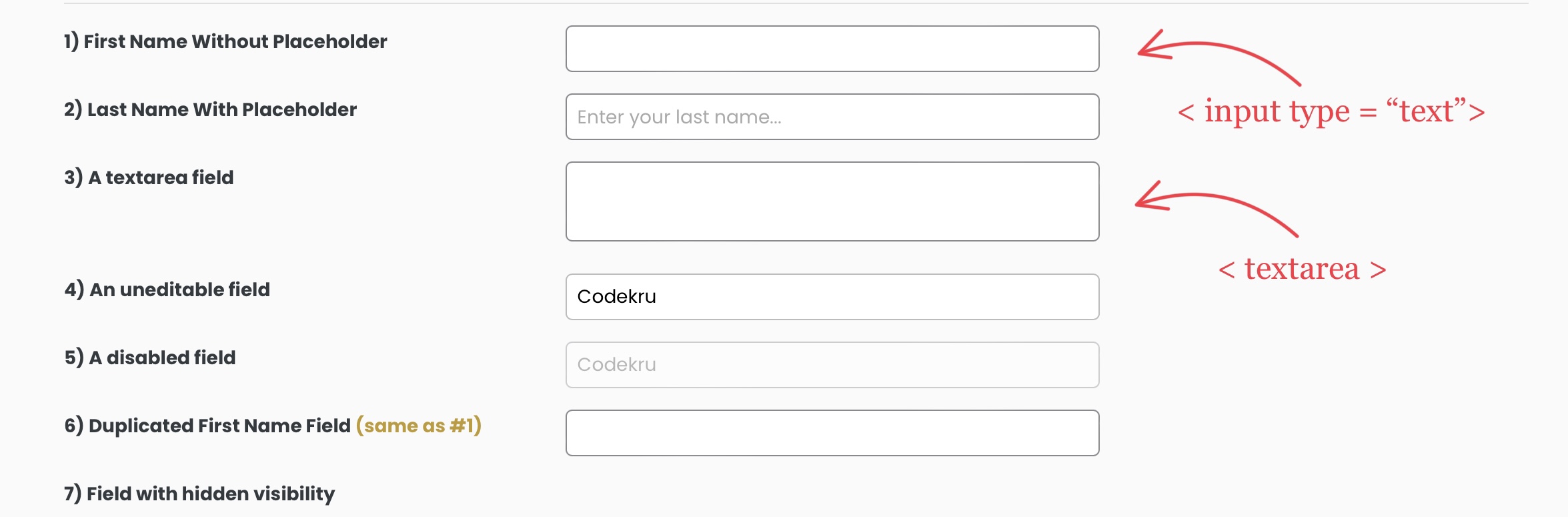
How to find a text box or a text field element?
Selenium provides us with many locators to locate any element on the webpage.
- Class name
- CSS selectors
- Id
- link text
- Name
- Partial link text
- Tag name
- XPath
We will only use the id attribute to find the text field element in our post.
How can we find a web element using the id?
There is a findElement() method provided by selenium webdriver, which we can use to find any element on the web page. As we are using the id attribute, we have to pass the id in the argument of the findElement() method.
Below is the syntax for finding a web element using the id attribute.
driver.findElement(By.id(id_attribute_value));
Let’s now find the element of one of the text boxes on our selenium playground website.

If we inspect the highlighted element, it will point us to its DOM, where we can see the ID attribute of the element. The value of the id attribute of our text box is firstName.
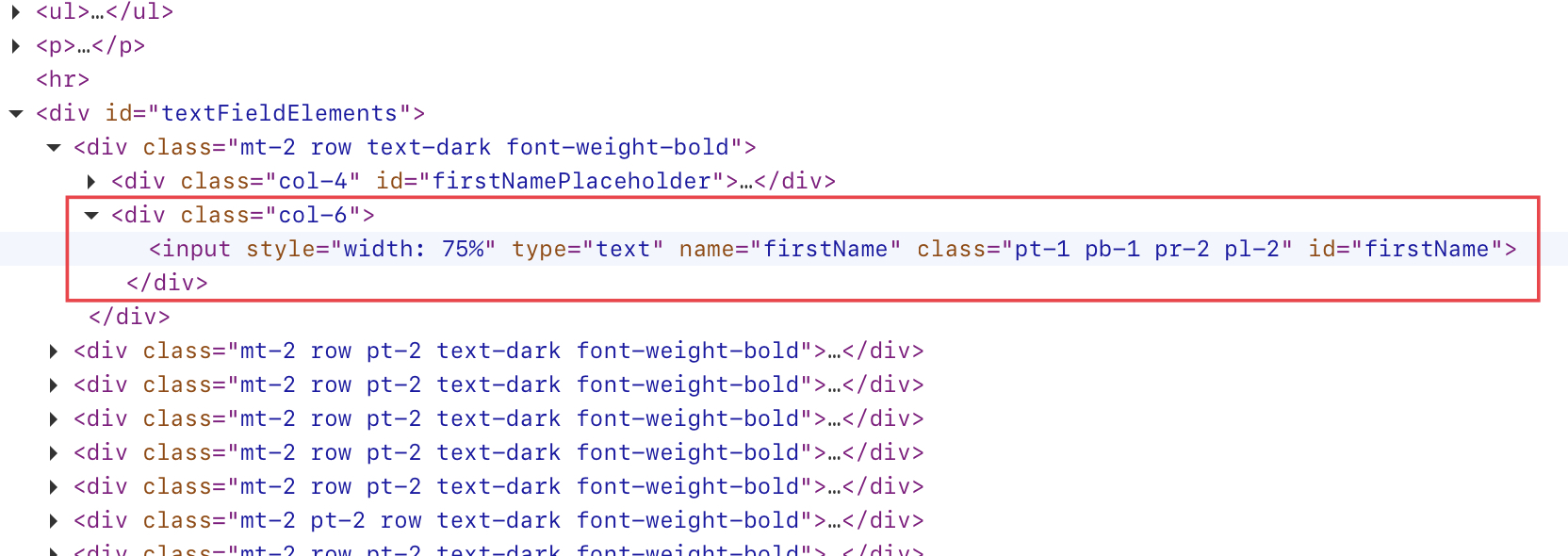
So, driver.findElement(By.id("firstName"))
will return the intended web element on which we can perform various operations.
How to enter a text in a text box?
As we have found the web element of the text field, let’s now enter some value in that input box.
We can use the sendKeys() method of the WebElement interface to enter a text into a text field.
Syntax of using sendKeys()
element.sendKeys(value_to_write_in_text_box)
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("firstName"));
// this will type "codekru" in the text field.
element.sendKeys("codekru");
}
}
The above program will type “codekru” in the text field.
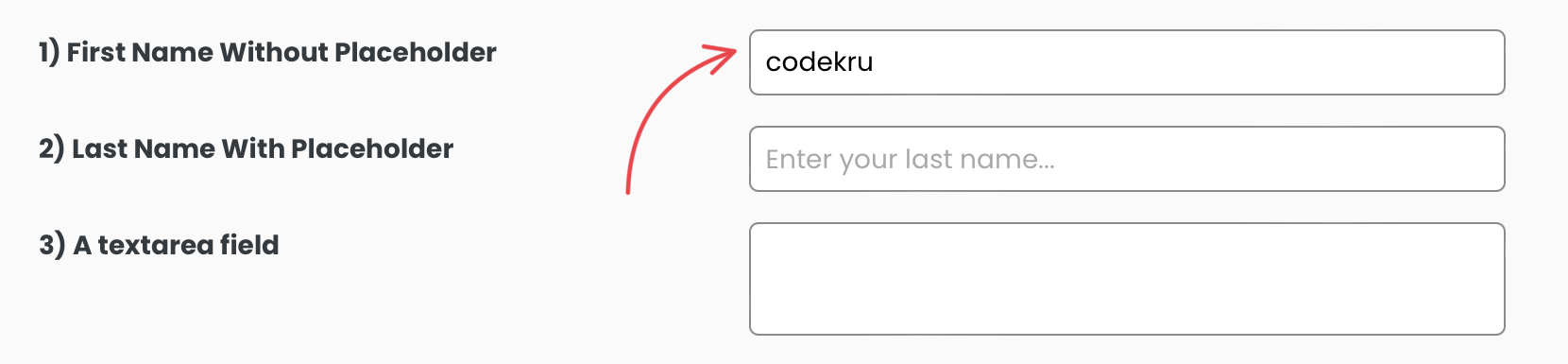
How to get a pre-filled text in a text box?
There are times when there is a pre-filled text in a text field, and we may have to validate that text.
We have one such element on our selenium playground website and will use that element in our example.

And below is the DOM structure of the highlighted element.
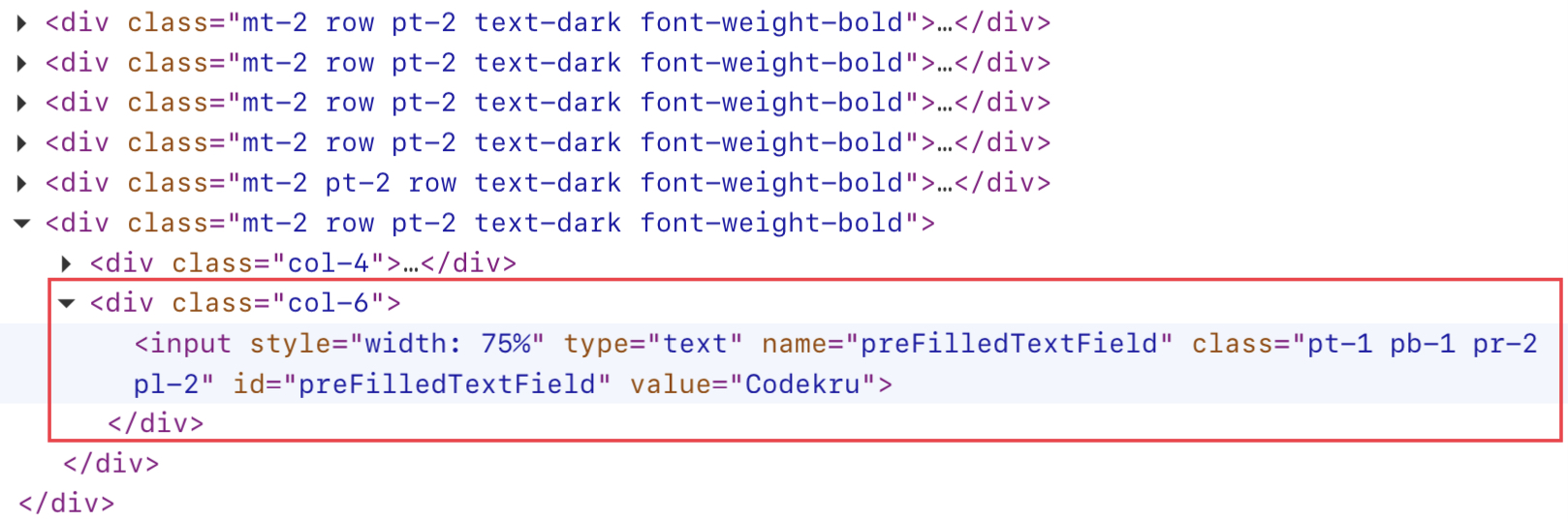
The id of the highlighted element is “preFilledTextField“.
We can see a “value” attribute that reflects the pre-filled text of the input box. So, if we can find a way to get the value of the “value” attribute, we will also have the pre-filled text.
Selenium provides a getAttribute() method, which can help us find the value of any attribute.
So, if we use getAttribute(“value”), it would give us the pre-filled text of an input box.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("preFilledTextField"));
// this will get the pre-filled text in an input box
System.out.println("Pre-filled text: " + element.getAttribute("value"));
}
}
Output –
Pre-filled text: Codekru
How to get the placeholder text of an input text box?
A placeholder is normally a sample value or a short description of the value to be entered in an input field.
We also have one such element on https://testkru.com/Elements/TextFields.
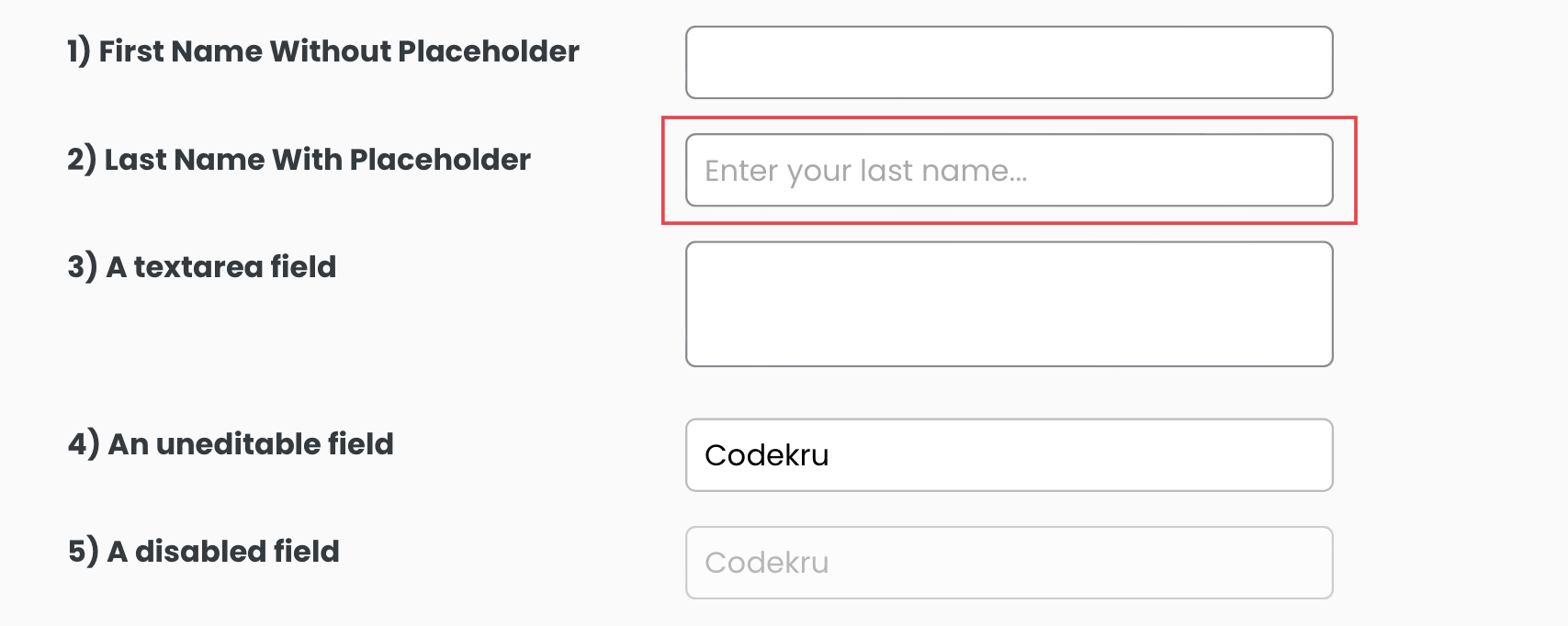

We can see that the id of the highlighted element is “lastNameWithPlaceholder“. Now, we will find the placeholder text written inside the input field.
If we look closely, we can see that the value of the “placeholder” attribute in the DOM element represents the value shown as a placeholder text on the web page. So, if we can get the value of the “placeholder” attribute, it will get us the placeholder text.
We can use the getAttribute() method to get the “placeholder” attribute value.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("lastNameWithPlaceholder"));
// this will get the placeholder text
System.out.println("Placeholder text: " + element.getAttribute("placeholder"));
}
}
Output –
Placeholder text: Enter your last name...
How to clear a pre-written text from a text box?
There may be situations where we have to clear some pre-written text. We will look at how we can achieve this using selenium webdriver.
Selenium has a clear() method which clears the value of an input or a textarea element. We will only have to call the clear() method on a particular element, which will clear the pre-filled value from the field.
element.clear() // this will clear the pre-filled text
We will use the pre-filled element on https://testkru.com/Elements/TextFields.

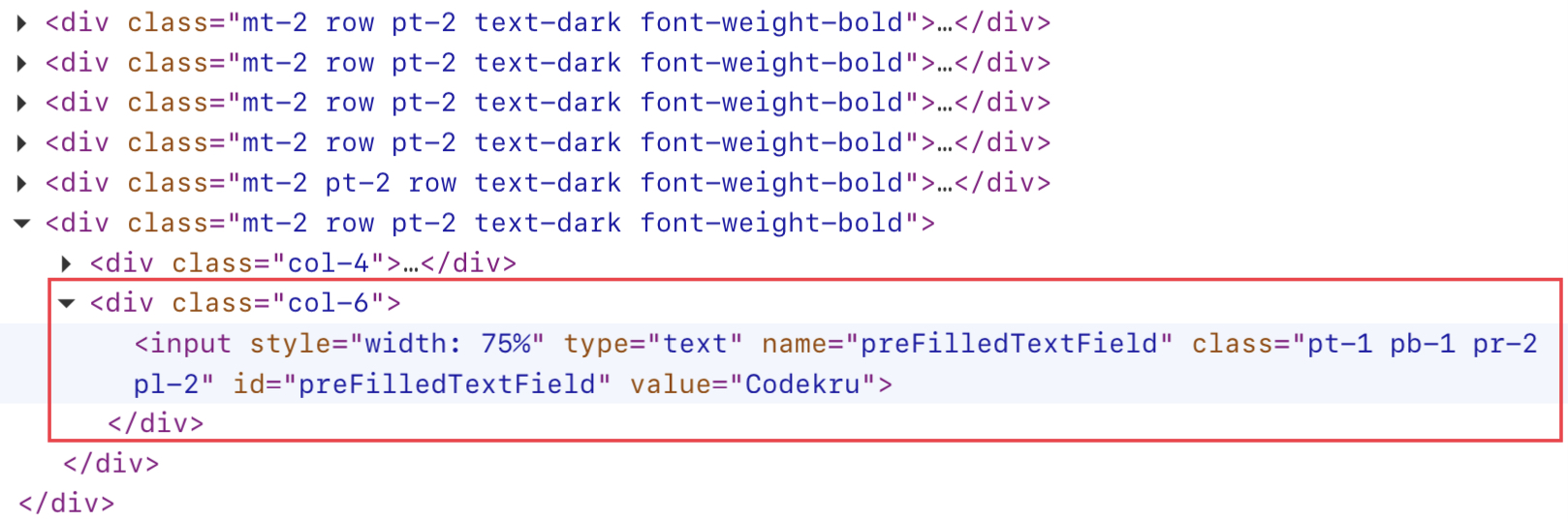
The id of the highlighted element is “preFilledTextField“. We will find the highlighted using the id attribute and then perform the clear operation on it.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("preFilledTextField"));
// this will clear the pre-filled text
element.clear();
}
}
After executing the above code, any pre-filled text will be removed.
How to check if a text field is editable or not?
What is a non-editable field? As indicated by the name itself, a non-editable field is a field that cannot be edited or manipulated by any operation performed by the user.
How do we know whether a field is editable or not? There is a “readonly” attribute that indicates whether a field can be edited or not. The field is not editable if this attribute is present on any element.
We have one such element on https://testkru.com/Elements/TextFields.
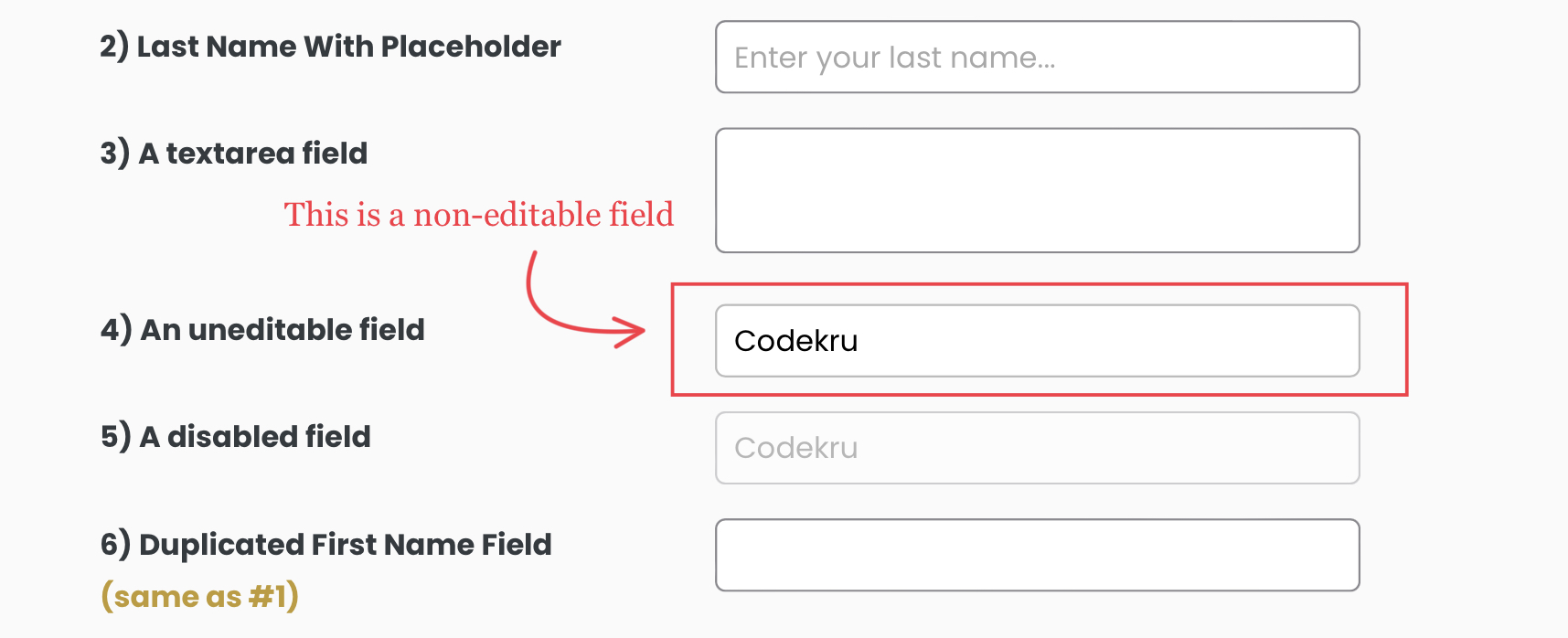

We have highlighted the readonly attribute, which makes a field non-editable.
How do we know whether the “readonly” attribute is present for the element or not? Here also, we can get help from the getAttribute() method.
If getAttribute(“readonly”) returns true, this means the “readonly” attribute is present on the element, otherwise, not.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("uneditable"));
// this will tell the field is editable or not
System.out.println("Is text field non-editable: " + element.getAttribute("readonly"));
}
}
Output –
Is text field non-editable: true
How to check if a text field is disabled or not?
Earlier, we discussed the non-editable field, and now we will focus on the disabled field. There are differences between an editable and a disabled field; we will not go much deeper into these differences. We only need to know that the “readonly” attribute governs editability and that making a field disabled is governed by the “disabled” attribute.
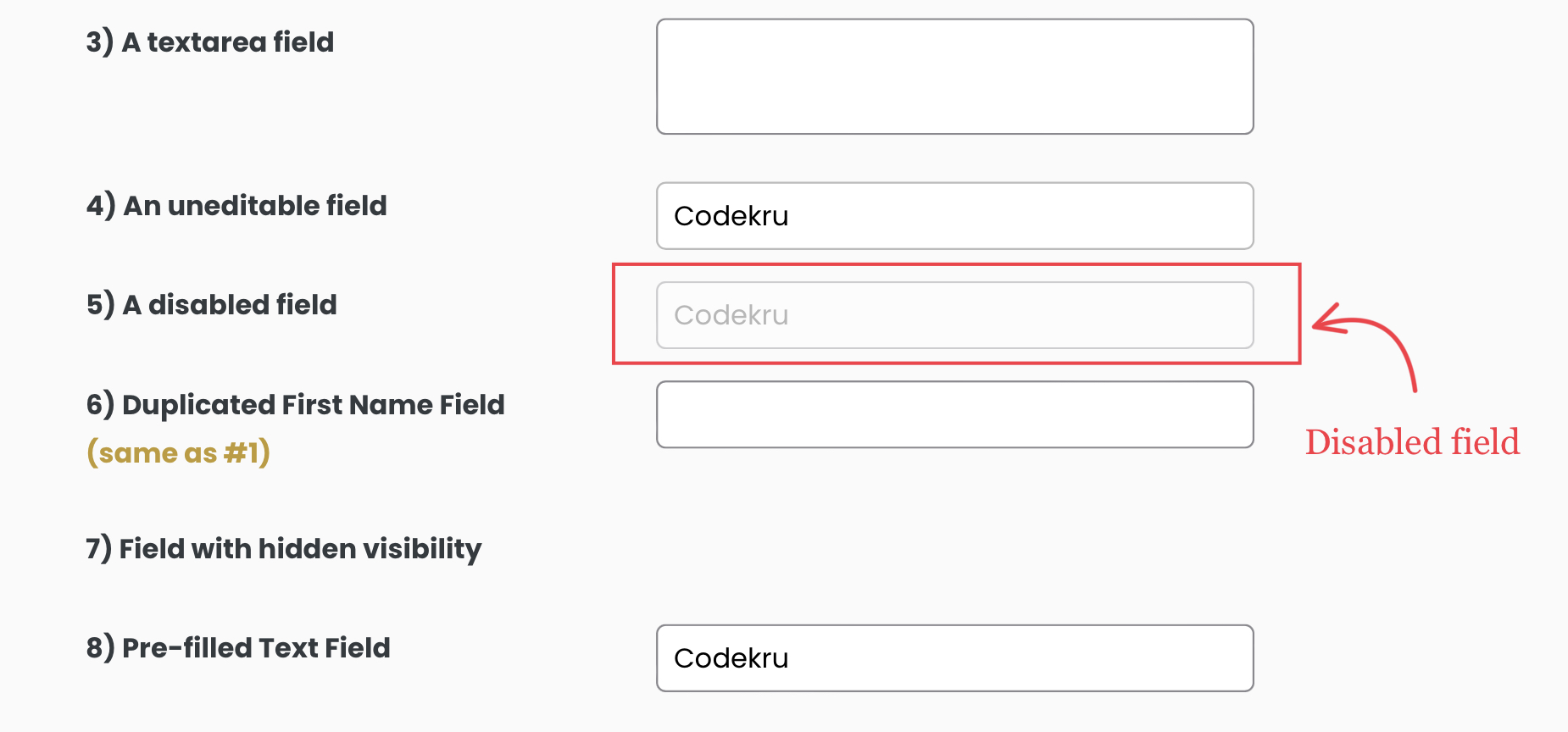
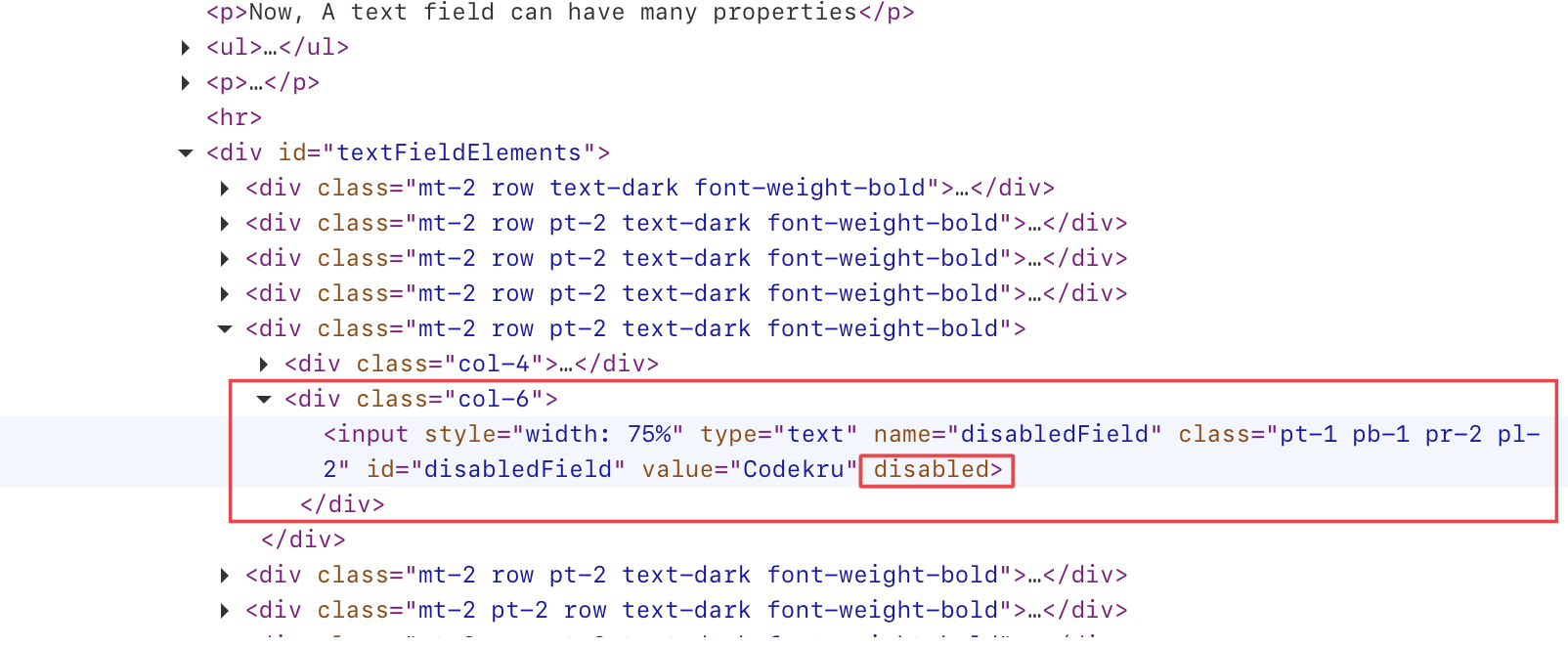
We can use the isEnabled method of the WebElement interface provided by selenium webdriver to check whether an element is enabled or not.
isEnabled() method will return true if a field is enabled and false if a field is disabled.
Let’s try this on our highlighted element. You can also find this element at https://testkru.com/Elements/TextFields.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/TextFields");
WebElement element = driver.findElement(By.id("disabledField"));
// this will tell the field is editable or not
System.out.println("Is text field enabled: " + element.isEnabled());
}
}
Output –
Is text field enabled: false
We have demonstrated our examples using an input type element, but we can also use the textarea element and perform the same operations.
We hope that you have liked this article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.