Buttons are mostly elements with the <button> attribute and are used to submit or display information. They are used everywhere on the web, so we might need to perform some operations to validate the buttons or the information they display.
This post will look at how we can automate various actions performed on the buttons in Selenium Webdriver using Java. We will perform various operations on different types of buttons.
We have our selenium playground website, where we have a lot of different kinds of buttons, and we will be using those in our post.

We will look at below topics in our post –
- How to find the button element?
- How to click on a button?
- How to right-click a button?
- How to double-click on a button?
- How to get the text written on a button?
- How to hover over a button?
- How to know if a button is disabled?
How to find the button element?
Selenium provides many locator strategies to help us locate any element on the webpage. Below is a list of the locator strategies.
- Class name
- CSS selectors
- Id
- link text
- Name
- Partial link text
- Tag name
- XPath
For the post’s simplicity, we will use the id locator to find the button elements and perform operations on them.
How can we find a web element using the id?
There is a findElement() method provided by selenium webdriver, which we can use to find any element on the web page. As we are using the id attribute, we have to pass the id in the argument of the findElement() method.
Below is the syntax for finding a web element using the id attribute.
driver.findElement(By.id(id_attribute_value));
Let’s now find one of the button elements on https://testkru.com/Elements/Buttons

If we inspect the highlighted element, we will get the below DOM, and there we will be able to see the id of the button element.
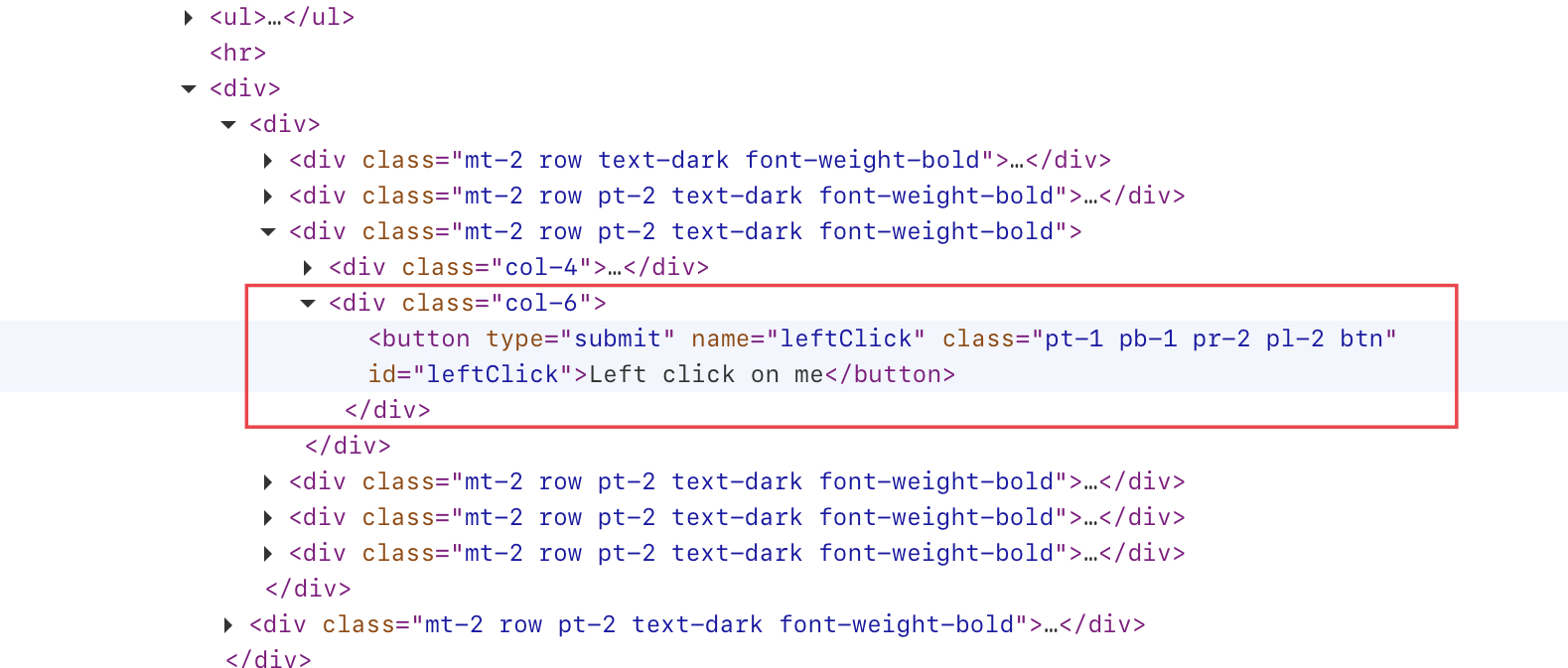
The id of the element is “leftClick“. We will use the below piece of code to get the desired element associated with the id.
driver.findElement(By.id("leftClick"));
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement element = driver.findElement(By.id("leftClick"));
}
}
element variable is now referring to the highlighted element in the image above.
How to click a button in Selenium?
We now have our element, and the next step would be to click on that element or button.
How can we click on a button? Selenium Webdriver has a click() method which helps click on a specified element.
click() method does a left-click or a right-click? click() method does a left click on the specified element.
Syntax of using the click() method –
element.click()
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement element = driver.findElement(By.id("leftClick"));
element.click();
}
}
The above program will click on the element with the id “leftClick“.
How to right-click a button in Selenium?
Earlier, we performed a left-click on the button and let’s now try to perform a right-click on a button.
We will use one of the buttons on this page: https://testkru.com/Elements/Buttons.

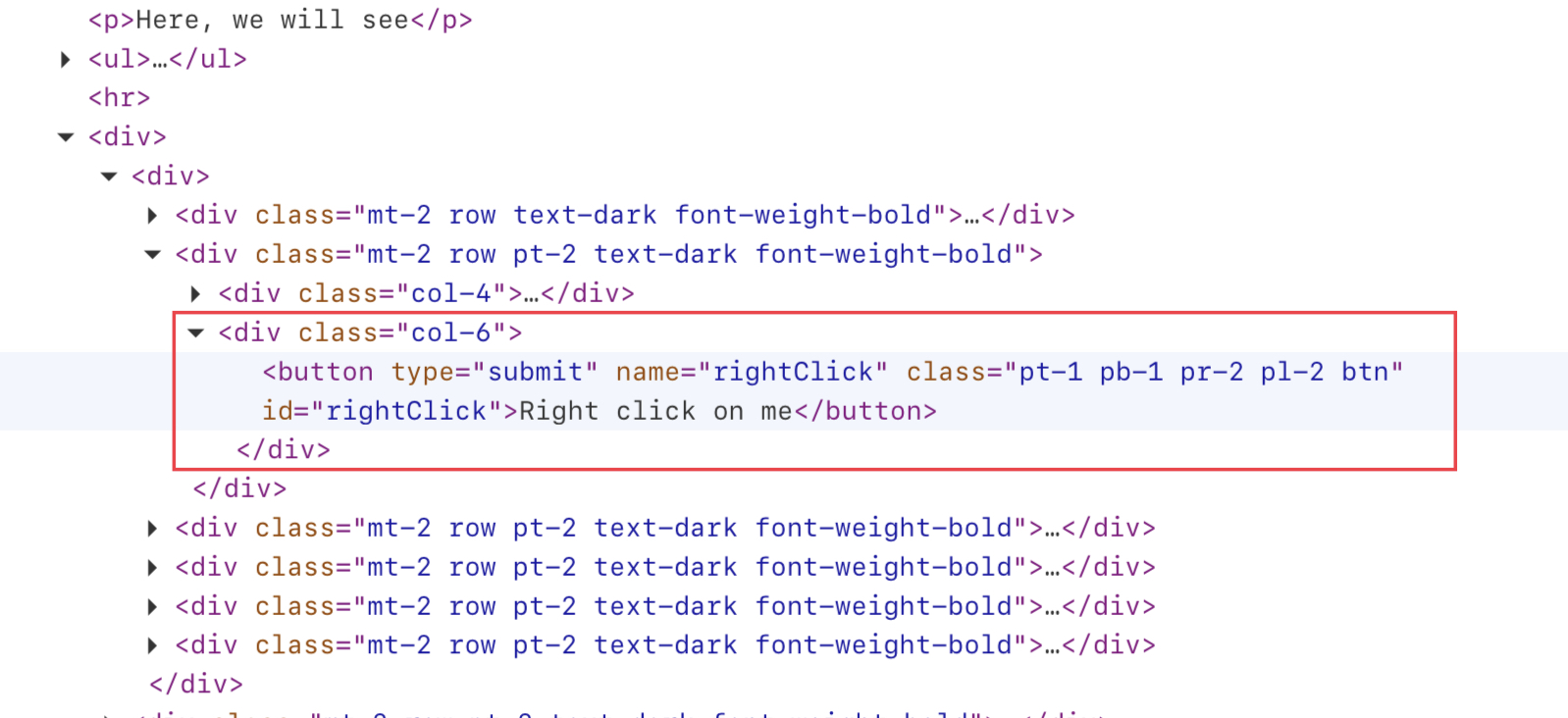
We will use the Actions class to perform a right-click on the button. Again we will use the id attribute to find the element using the findElement() method.
Actions class has a contextClick() method, which will right-click on the specified button. The below syntax is used to right-click on an element.
// Right click on a button
// using Actions class
Actions action = new Actions(driver);
action.contextClick(element).perform();
Now, let’s write the whole code.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement element = driver.findElement(By.id("rightClick"));
// Right click on a button
// using Actions class
Actions action = new Actions(driver);
action.contextClick(element).perform();
}
}
The above program will open https://testkru.com/Elements/Buttons and right-click on the specified element.
How to double-click on a button in Selenium?
Actions class also provides a method to perform a double-click operation on the specified element.
We will again take one of the buttons on this page – https://testkru.com/Elements/Buttons. We have highlighted it in the below image.
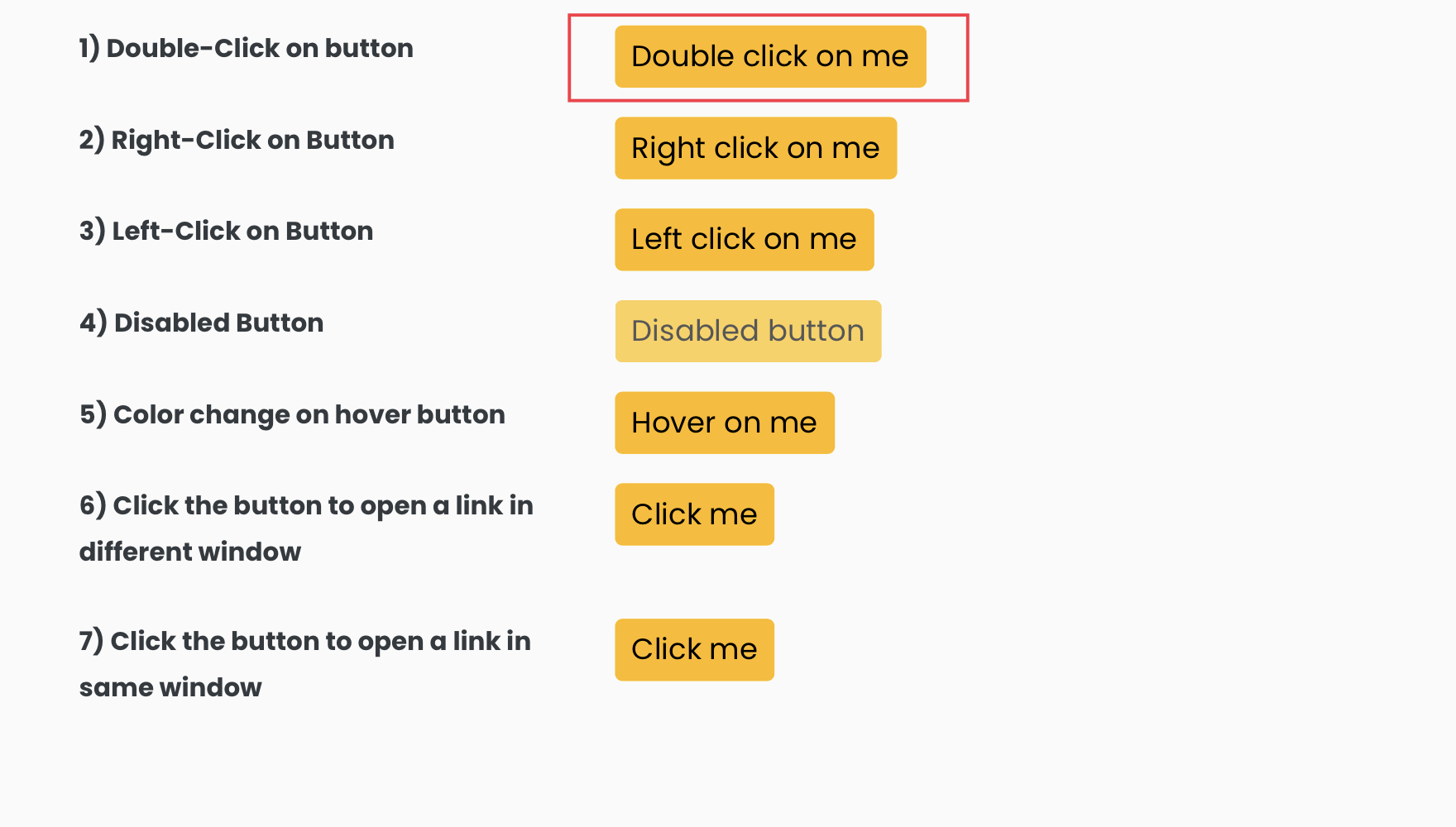
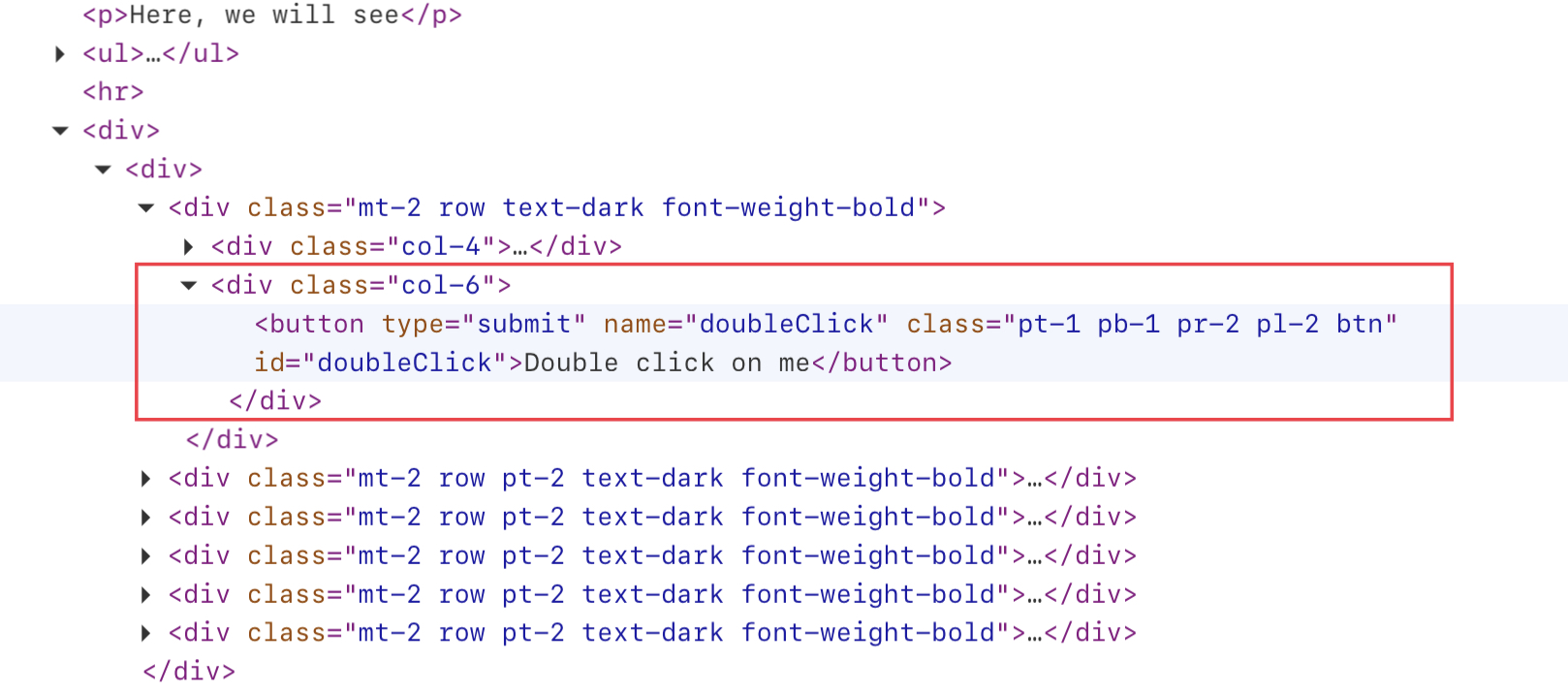
The id of the highlighted element is “doubleClick“, and we will perform a double-click action on this element.
Actions class has a doubleClick() method, which can perform a double-click event on any element. The below syntax is used to perform a double-click operation.
// Double click on a button
// using Actions class
Actions action = new Actions(driver);
action.doubleClick(element).perform();
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement element = driver.findElement(By.id("doubleClick"));
Actions action = new Actions(driver);
action.doubleClick(element).perform();
}
}
This will double-click on the highlighted button.
How to get the text written on a button in Selenium?
Sometimes some information is shown on the button, and we may have to validate it to check whether it is correct. Like if we submit a form using a button and the button text changes to “submitted”. How can we get the text displayed on the button?
We can use the getText() method to get the text written on a button. Let’s use a simple button to test this out.

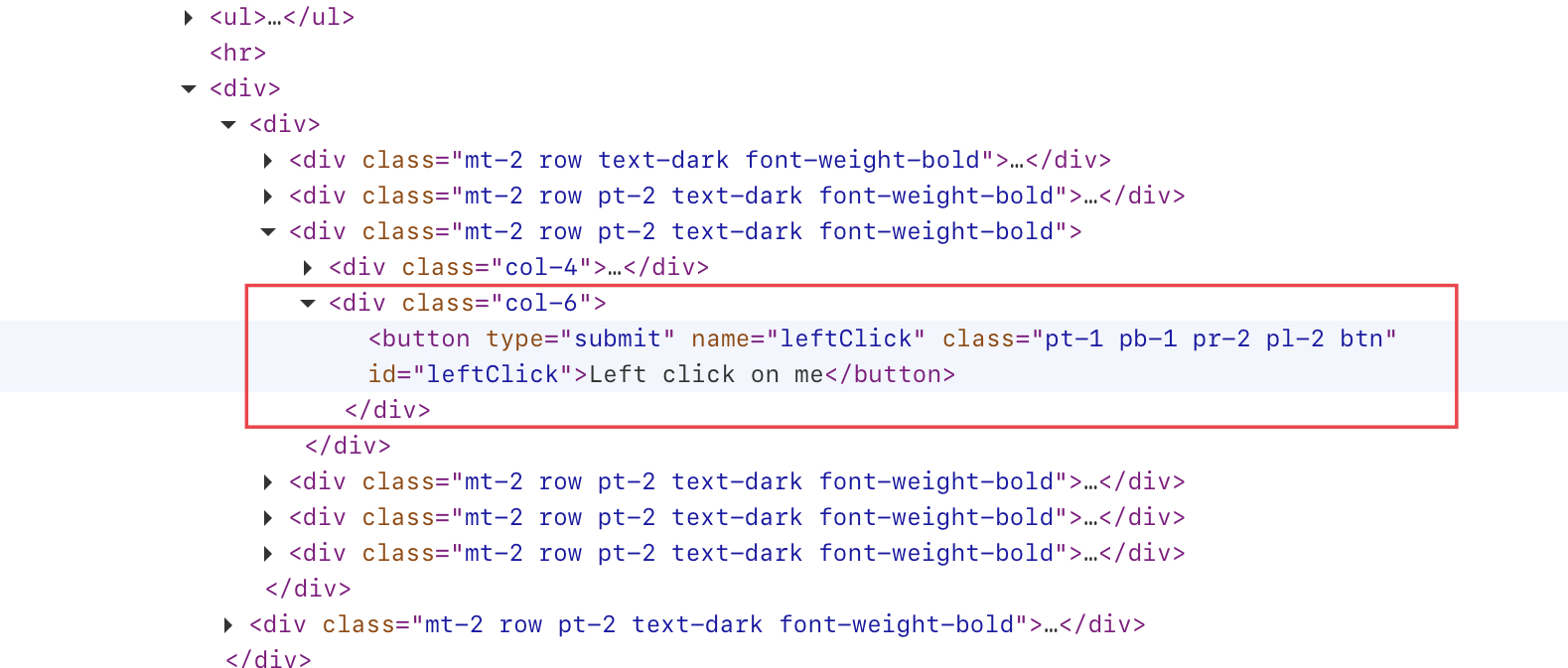
We can see that the text displayed on the button is “Left click on me“. We will try to get this text using the selenium getText() method.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement element = driver.findElement(By.id("leftClick"));
System.out.println("Text written on the button: " + element.getText());
}
}
Output –
Text written on the button: Left click on me
How to hover over a button in Selenium?
Here also, the Actions class method will come to the rescue. Actions class has a moveToElement() method, which is used to hover over an element.
moveToElement() method moves the mouse to the middle of an element and thus imitates a hover impact.
Syntax of using moveToElement() method
// hover over the button element
Actions action = new Actions(driver);
action.moveToElement(element).perform();
There is one button on https://testkru.com/Elements/Buttons, which changes its color after hovering over it. We will be using that button in our example.
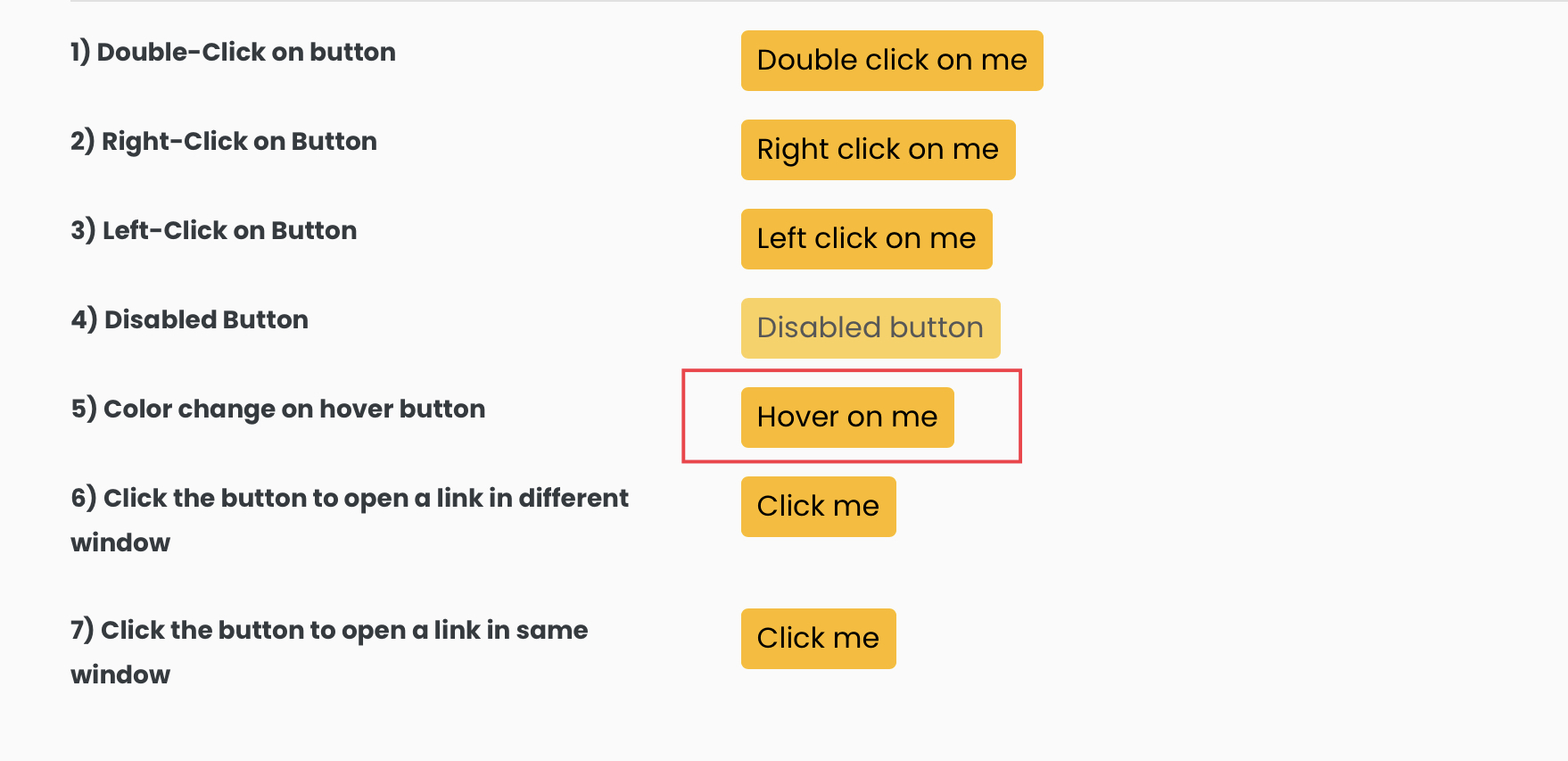
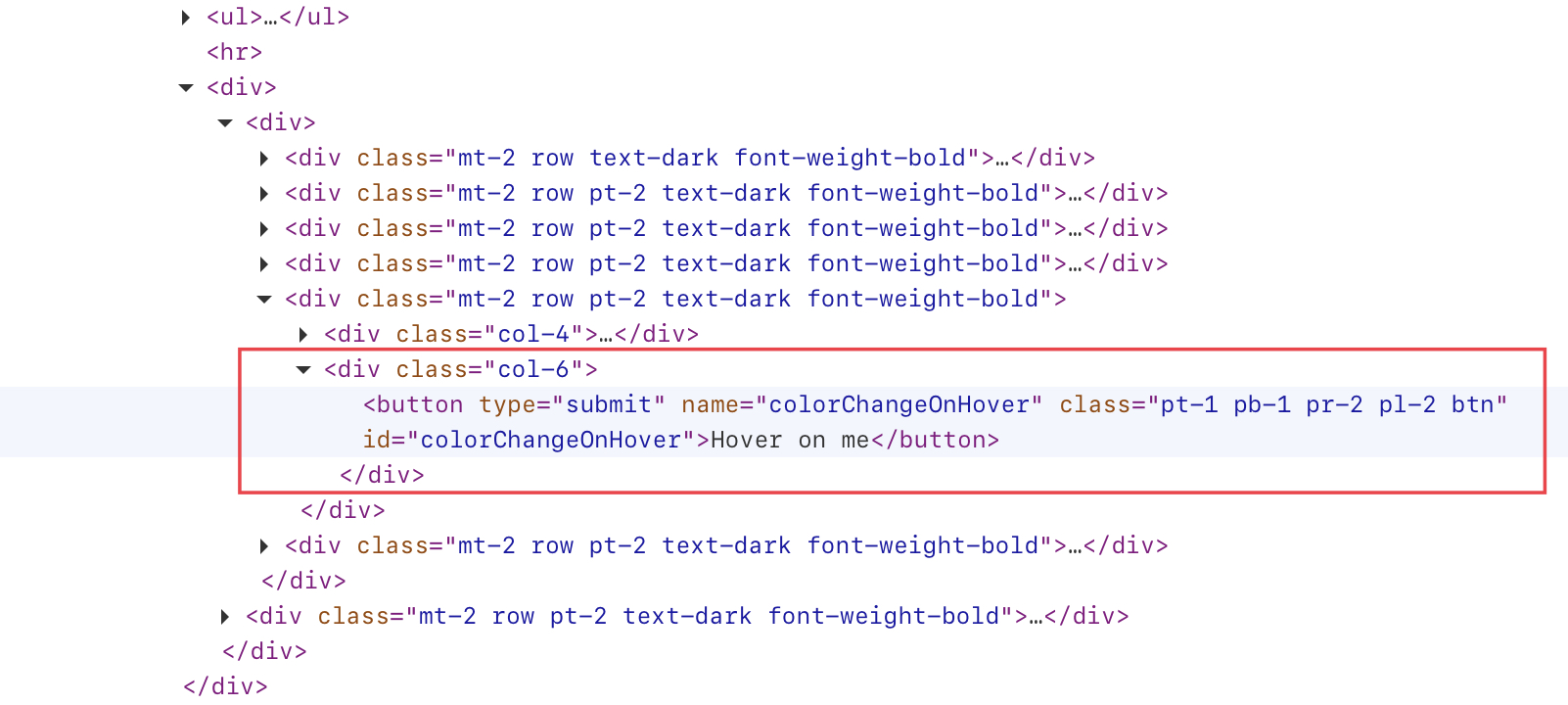
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement element = driver.findElement(By.id("colorChangeOnHover"));
Actions action = new Actions(driver);
action.moveToElement(element).perform();
}
}
You will be able to see a color change on the button after you’ll execute the above program.
How to know if a button is disabled in Selenium?
isEnabled() method of the WebElement interface in Selenium can be used to tell whether a button is disabled or not.
isEnabled() method will return true if the button is enabled. Otherwise, it will return false.
We will use a disabled button for our example.
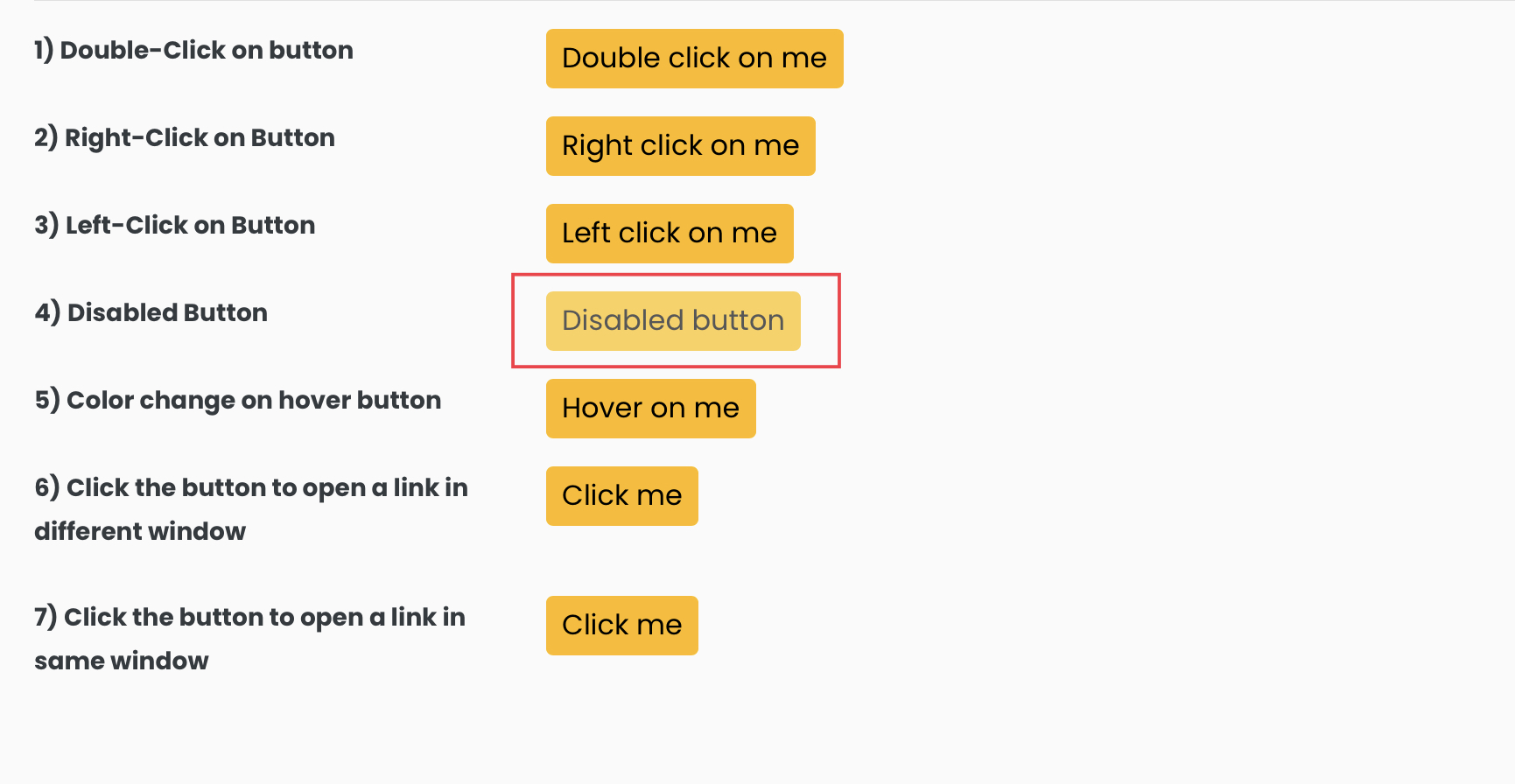
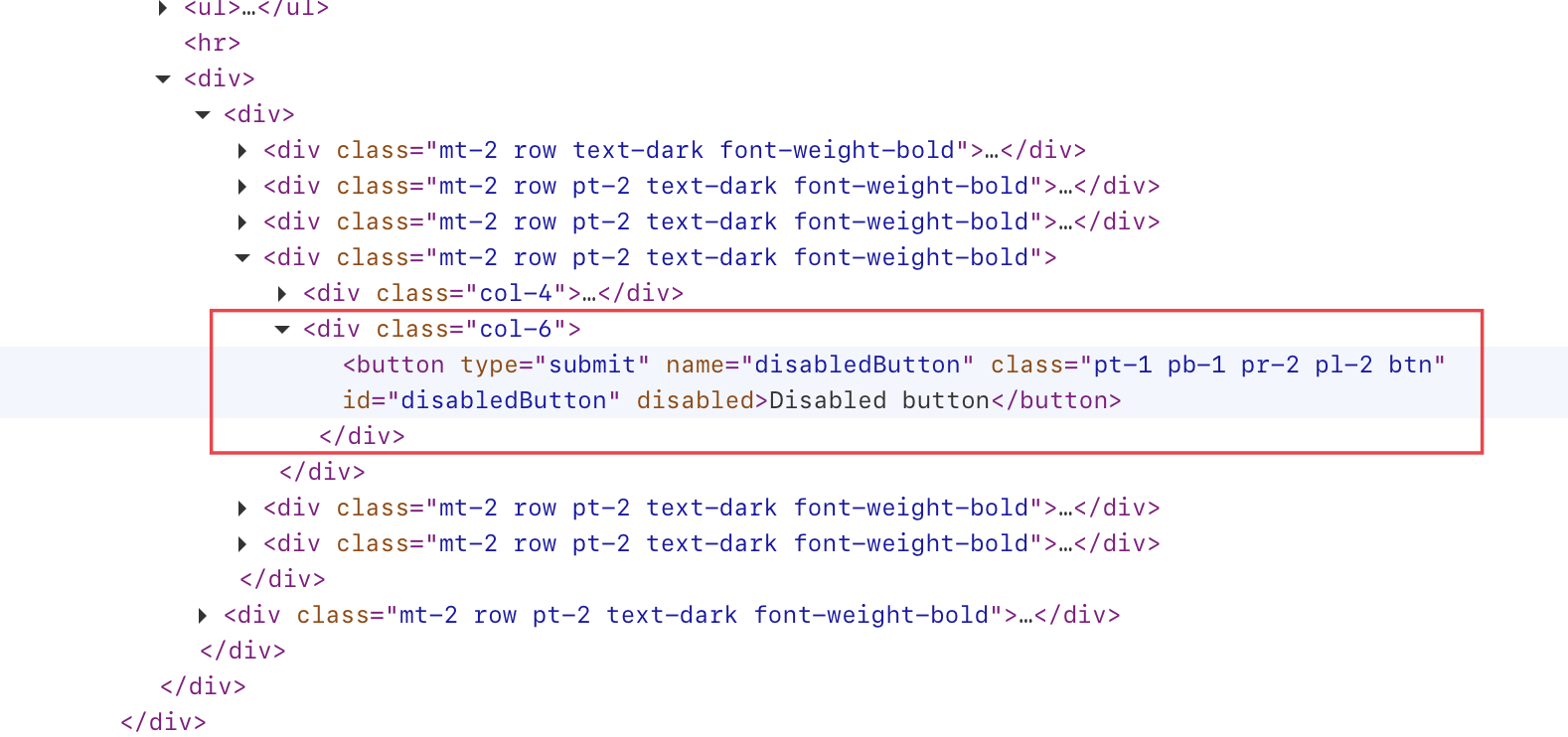
The id of the highlighted element is “disabledButton“. We will use this id first to find the element and then use the isEnabled() method on it.
public class CodekruTest {
@Test
public void test() {
// pass the path of the chromedriver location in the second argument
System.setProperty("webdriver.chrome.driver", "E:\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
// opening the url
driver.get("https://testkru.com/Elements/Buttons");
WebElement element = driver.findElement(By.id("disabledButton"));
System.out.println("Is Element enabled: "+ element.isEnabled());
}
}
Output –
Is Element enabled: false
We can see that the method returned false because the button was disabled. It would have returned true for an enabled button.
We hope that you have liked this article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.