Earlier, we talked about XPath and its basics, and this post will discuss the use of the contains() method while writing the XPath in Selenium.
contains() method help locate element(s) using partial text and thus plays a key role in writing dynamic XPath for locating the element.
contains() method takes two arguments and determines whether the first argument contains the second one. If it does, then it returns true; otherwise false.
Note: Before moving ahead, please remember that contains() method is case-sensitive. So, “codekru” and “Codekru” aren’t the same for contains() method.
contains() method can be used in many ways
- XPath contains() method with attributes (@class, @id attributes, etc.)
- contains() method with text()
- contains() method with dot (.)
Let’s look at them one by one.
XPath contains() method with attributes
Syntax for contains() method with attributes
//tag_name[contains(@attribute_name,'value_to_be_checked')]
or, if we don’t want to use tag_name, below syntax could also be used.
//*[contains(@attribute_name,'value_to_be_checked')]
* (star) is a wildcard that searches in the whole DOM irrespective of the tags.
We can see that contains() method takes two arguments, one is the attribute name, and the second is the value it contains.
Let’s take the below HTML code.
<html>
<head>
</head>
<body>
<div>
<p>Codekru Tutorials</p>
</div>
<div>
<a class = "codekru" href = "https://www.codekru.com" id = "websiteLink" >Codekru Website link</a>
<p>Guide to XPath in Selenium</p>
</div>
<div>
<a href = "https://www.codekru.com/selenium">Selenium Link</a>
</div>
</body>
</html>
We want to find the element that contains “selenium” in its “href” attribute value.
Keeping the syntax in mind, below XPath can help us locate the element –
//*[contains(@href,'selenium')]
or, if we want to limit our search to the “<a>” tag only, then we could also use the tag name.
//a[contains(@href,'selenium')]
The above XPath will select the element containing the “selenium” keyword in the “href” attribute’s value.
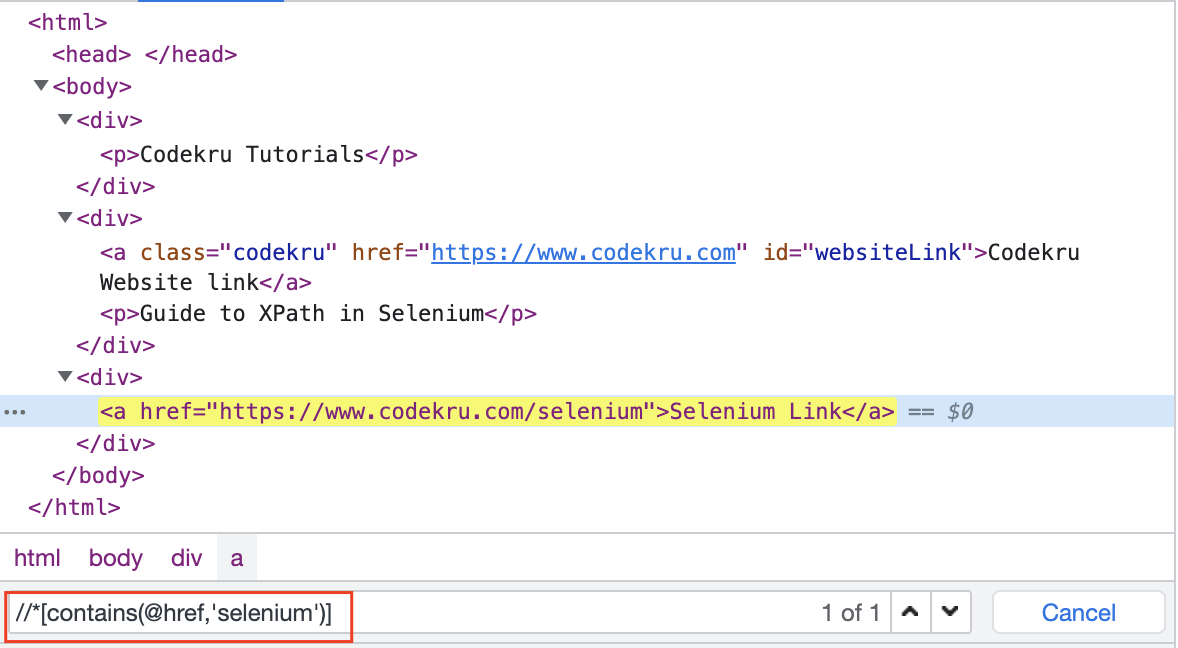
Now, let’s look at some of the most commonly used attributes with contains() method
XPath contains() method with the “id” attribute
We will take the same HTML code again and find the element whose “id” attribute value contains the “website” keyword.
<html>
<head>
</head>
<body>
<div>
<p>Codekru Tutorials</p>
</div>
<div>
<a class = "codekru" href = "https://www.codekru.com" id = "websiteLink">Codekru Website link</a>
<p>Guide to XPath in Selenium</p>
</div>
<div>
<a href = "https://www.codekru.com/selenium">Selenium Link</a>
</div>
</body>
</html>
Below XPath will search the whole DOM and select the element(s) containing the “website” keyword in the “id” attribute’s value/
//*[contains(@id,"website")]
XPath contains() method with the “class” attribute
We could also do the same with the “class” attribute. The below XPath selects the element(s) that contains the “code” keyword in the “class” attribute’s value.
//*[contains(@class,'code')]
contains() method with text()
We can use the XPath functions in place of the attribute_name with contains() method. Let’s take the example of one of the most used functions – text().
With the help of contains() and the text() functions, we can write such an XPath that selects the element(s) based on partial text only. We won’t require the whole text to write the XPath anymore.
Syntax
//*[contains(text(),'partial_text_to_be_searched')]
Let’s take the below HTML example.
<html>
<head>
</head>
<body>
<div>
<p>Codekru Tutorials</p>
</div>
<div>
<a class = "codekru" href = "https://www.codekru.com" id = "websiteLink">Codekru Website link</a>
<p>Guide to XPath in Selenium</p>
</div>
<div>
<a href = "https://www.codekru.com/selenium">Selenium Link</a>
</div>
</body>
</html>
We want to locate the element(s) that contains the “Codekru” text.
Below XPath would get us those element(s).
//*[contains(text(),"Codekru")]
We can see that it selected two elements containing the “Codekru” keyword.
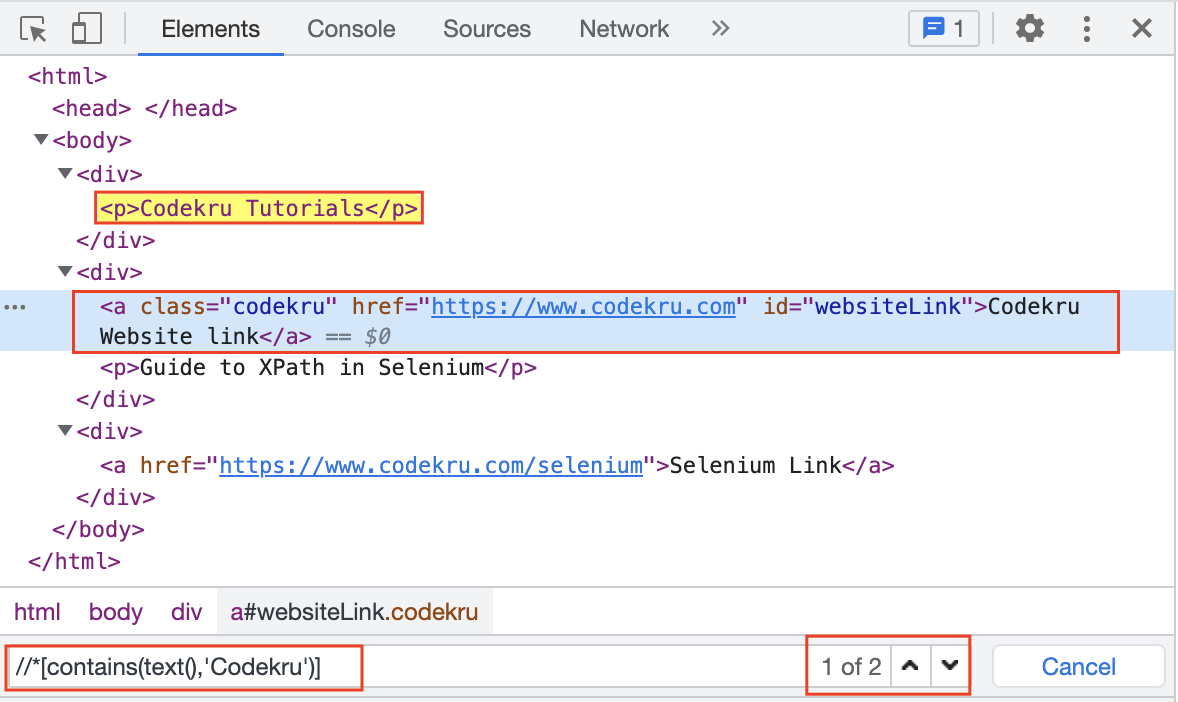
We can also use the tags to limit the search to a specific tag element. Like if we only wanted the <p> tags that contain the “Codekru” text, then we can modify our XPath as shown below –
//p[contains(text(),"Codekru")]

We can see that it only returned the element(s) with <p> tag.
XPath contains() method with dot
We can also use a dot (.) instead of the text() function,
Syntax of using the dot
//*[contains(.,'text_to_be_searched')]
But there is a difference between the text() and the dot.
text() function will only select the element(s) that have the specified text, it won’t select any of the ancestor nodes of the element, but the dot will select all ancestor elements along with the element containing the text.
Let’s take a look at this using an example.
<html>
<head>
</head>
<body>
<div>
<p>Codekru Tutorials</p>
</div>
<div>
<a class = "codekru" href = "https://www.codekru.com" id = "websiteLink">Codekru Website link</a>
<p>Guide to XPath in Selenium</p>
<em>This is a test node</em>
</div>
<div>
<a href = "https://www.codekru.com/selenium">Selenium Link</a>
</div>
</body>
</html>
Now, we will find the element(s) that contains the “test” keyword using both the text() method and dot.
Using text() method
Using text() with the contains() method selects only the element(s) containing the text. No ancestor nodes will be selected using it.
//*[contains(text(),'test')]
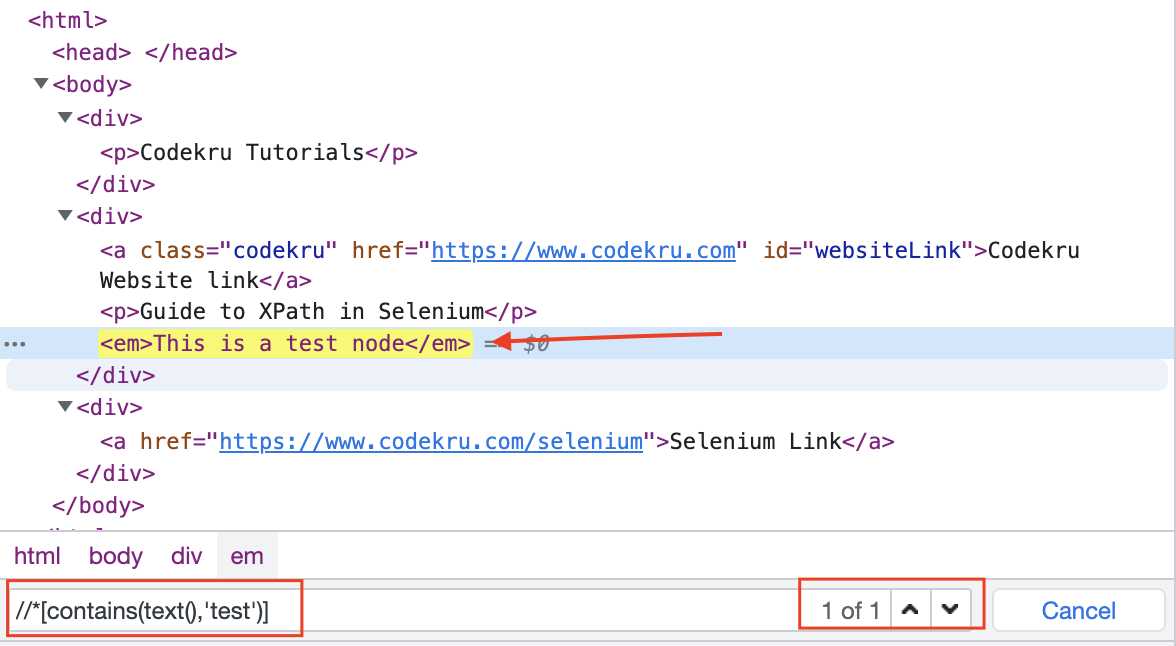
Using dot
If we use the dot expression with the contains() method, it will select ancestor elements along with the node containing the text.
Below XPath uses the dot expression and searches for the “test” keyword using contains() method.
//*[contains(.,'test')]
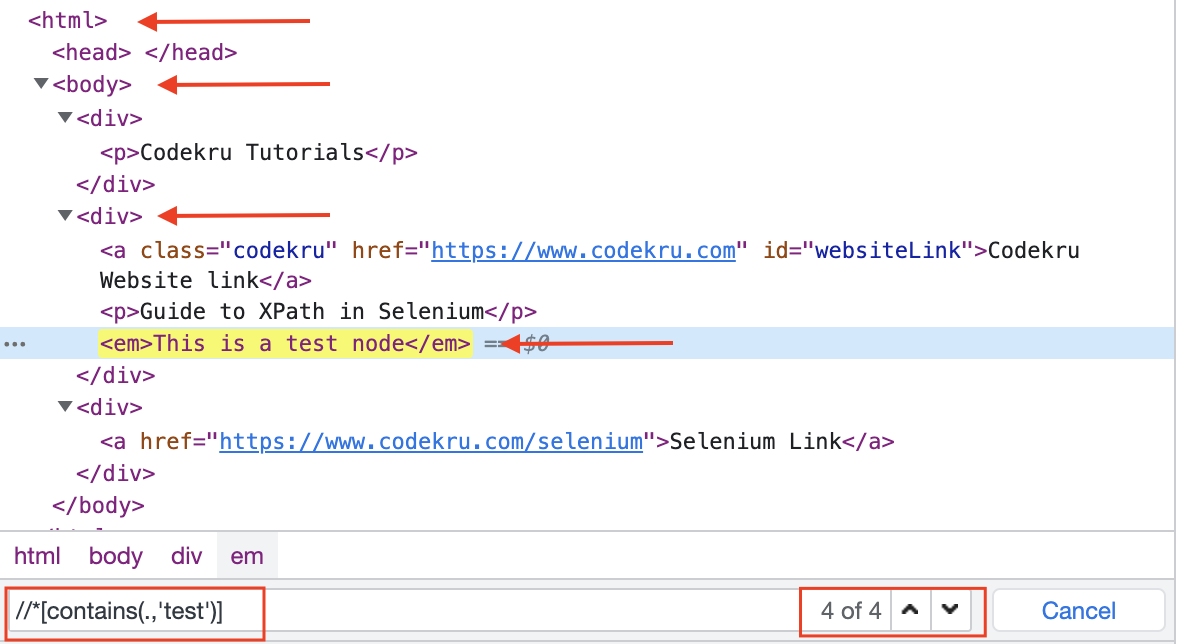
We can see that it selected four nodes leading up to the root node.
Depending on your requirement for locating the elements, you can use text() or dot.
This is it. We hope that you have liked the article. If you have any doubts or concerns, please feel free to write us in the comments or mail us at admin@codekru.com.